使用LiveData简化Android应用程序中的数据交互
发布时间: 2023-12-31 07:59:47 阅读量: 92 订阅数: 24 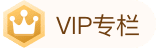
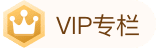
# 引言
## 1.1 介绍LiveData的概念和作用
LiveData是Android官方提供的一个数据持有者类,用于在Android应用程序中观察和响应数据的变化。它可以在数据发生变化时通知相关观察者,并确保观察者在活动状态时才能接收到数据更新。与传统的Observer模式相比,LiveData具有许多优势,可以简化Android应用程序中的数据交互。
## 1.2 解释为什么LiveData可以简化Android应用程序中的数据交互
在传统的Android开发中,数据的交互通常是通过回调接口实现的,这样往往需要在Activity或Fragment中手动管理数据的变化和UI的更新。这样的方式存在一些问题:首先,操作复杂,容易出错;其次,没有统一的管理逻辑,导致代码结构混乱;最重要的是,容易引发内存泄漏问题。
LiveData通过将数据和观察者解耦,将数据的更新与UI的更新自动关联起来,消除了手动管理的繁琐操作。它使用观察者模式,但在实现上更加灵活和安全。LiveData还具有生命周期感知能力,能够根据观察者的生命周期自动开始和停止观察数据的变化,从而有效地避免了内存泄漏的问题。同时,LiveData还支持异步数据处理,使得在后台线程获取数据并更新UI变得更加简单和可靠。
通过使用LiveData,我们可以简化Android应用程序中的数据交互,提高代码的可读性和可维护性,减少bug的产生,提升用户体验。
接下来,我们将详细介绍LiveData的基本用法,以及它在Android应用程序中的优势。
## 2. LiveData的基本用法
LiveData是一种可以感知生命周期并具备观察者模式的数据持有类,它可以用于在Android应用程序中进行数据交互。下面将介绍LiveData的基本用法,包括LiveData的创建和初始化、观察LiveData数据的变化以及更新LiveData数据。
### 2.1 LiveData的创建和初始化
在使用LiveData之前,我们首先需要在项目中引入LiveData库。在build.gradle文件中的dependencies块中添加以下代码:
```java
implementation "androidx.lifecycle:lifecycle-livedata:2.3.1"
implementation "androidx.lifecycle:lifecycle-viewmodel:2.3.1"
```
接下来,我们可以通过LiveData的构造函数创建一个LiveData对象。在通常情况下,我们需要使用一个ViewModel来管理LiveData对象,因为ViewModel会在Activity或Fragment的生命周期发生变化时自动管理LiveData的订阅和取消订阅。
```java
public class MyViewModel extends ViewModel {
private MutableLiveData<String> mLiveData;
public LiveData<String> getLiveData() {
if (mLiveData == null) {
mLiveData = new MutableLiveData<>();
}
return mLiveData;
}
}
```
### 2.2 观察LiveData数据的变化
一旦我们创建了LiveData对象,我们可以通过观察LiveData数据的方式来获取数据的更新。我们可以在Activity或Fragment的onCreate方法中获取ViewModel实例,并使用observe方法观察LiveData数据的变化。
```java
public class MainActivity extends AppCompatActivity {
private MyViewModel mViewModel;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
mViewModel = new ViewModelProvider(this).get(MyViewModel.class);
mViewModel.getLiveData().observe(this, new Observer<String>() {
@Override
public void onChanged(String data) {
// 处理数据变化
// 在这里更新UI界面
}
});
}
}
```
在上面的代码中,我们使用observe方法来观察LiveData对象的数据变化。当LiveData的数据发生变化时,观察者中的onChanged方法将被触发,我们可以在该方法中处理数据的变化并更新UI界面。
### 2.3 更新LiveData数据
要更新LiveData的数据,我们可以通过setValue或postValue方法来更新LiveData对象持有的数据。这两种方法的区别在于,setValue方法必须在主线程中调用,而postValue方法可以在任意线程中调用。
```java
public class MyViewModel extends ViewModel {
private MutableLiveData<String> mLiveData;
public LiveData<String> getLiveData() {
if (mLiveData == null) {
mLiveData = new MutableLiveData<>();
}
return mLiveData;
}
public void updateData(String newData) {
mLiveData.setValue(newData);
// 或者使用 mLiveData.postValue(newData);
}
}
```
在上述代码中,我们通过调用setValue或postValue方法来更新LiveData的数据。当数据发生更新时,观察LiveData的UI界面将自动收到通知并进行更新。
通过上述2.1节到2.3节的介绍,我们可以使用LiveData来创建并观察数据的变化,以及更新数据。在下一节中,我们将讨论LiveData的优势,以及它如何简化Android应用程序中的数据交互。
### 3. LiveData的优势
在Android应用程序中,LiveData具有许多优势,使其成为数据交互的理想选择。下面将详细介绍LiveData的优势及其相关内容。
#### 3.1 自动处理数据更新和UI更新的关系
1. LiveData可以自动感知组件的生命周期,并且只在活跃状态下更新数据,这样可以避免因为组件生命周期不当而导致的空指针异常等问题。
2. 当LiveData中的数据发生变化时,UI组件会自动收到通知并更新显示内容,无需手动编写繁琐的数据刷新逻辑。
#### 3.2 避免常见的内存泄漏问题
1. 使用观察者模式,LiveData能够自动解除组件与数据源之间的绑定,避免了常见的内存泄漏问题。
2. LiveData的设计使得开发者不再需要担心手动管理观察者的解绑,大大简化了代码的编写和维护。
#### 3.3 异步数据处理的支持
1. LiveData可以与异步任务结合使用,例如结合Kotlin的协程或者RxJava,从而使得数据获取和更新可以在后台线程进行,而UI更新部分仍然可以在主线程安全地进行。
2. 通过与ViewModel配合,LiveData还能够在设备旋转等配置改变时保存和恢复数据的状态,提供更加稳定和可靠的异步数据处理能力。
以上就是LiveData的优势部分的详细内容。LiveData的这些特性使得它在Android应用开发中大显身手,提高了数据交互的安全性和可靠性。
接下来,我们将通过实际例子来展示LiveData的优势在实际应用中是如何发挥作用的。
### 4. 使用LiveData简化数据交互的例子
在本章节中,我们将通过具体的例子来演示如何使用LiveData简化Android应用程序中的数据交互过程。包括使用LiveData进行网络请求的数据交互、使用LiveData更新UI界面以及使用LiveData进行跨组件通信。
#### 4.1 使用LiveData进行网络请求的数据交互
在Android应用中,经常需要进行网络请求获取数据,并在获取到数据后更新UI界面。传统的做法是在Activity或者Fragment中执行网络请求的操作,然后在请求成功之后更新UI。这种方式可能会导致内存泄漏,同时也增加了组件之间的耦合度。
使用LiveData可以简化这一流程。我们可以在Repository层发起网络请求并将获取到的数据存储在LiveData对象中,然后在ViewModel中观察这个LiveData对象,最后在UI组件中观察ViewModel中的LiveData数据来更新UI。
下面是一个简单的使用LiveData进行网络请求的示例代码:
```java
// 在Repository中定义一个返回LiveData的方法
public class UserRepository {
private MutableLiveData<User> userData = new MutableLiveData<>();
public LiveData<User> getUserData() {
// 发起网络请求,获取数据
// 省略网络请求的具体实现
User user = fetchDataFromNetwork();
userData.setValue(user);
return userData;
}
}
// 在ViewModel中观察Repository中的LiveData数据
public class UserViewModel extends ViewModel {
private LiveData<User> userLiveData;
private UserRepository userRepository;
public UserViewModel() {
userRepository = new UserRepository();
userLiveData = userRepository.getUserData();
}
public LiveData<User> getUserLiveData() {
return userLiveData;
}
}
// 在Activity或者Fragment中观察ViewModel中的LiveData数据更新UI界面
public class UserProfileActivity extends AppCompatActivity {
private UserViewModel userViewModel;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_user_profile);
userViewModel = ViewModelProviders.of(this).get(UserViewModel.class);
userViewModel.getUserLiveData().observe(this, user -> {
// 更新UI界面
updateUI(user);
});
}
private void updateUI(User user) {
// 更新UI界面的具体操作
}
}
```
通过上述代码,可以看到使用LiveData可以将数据获取与UI更新进行有效地分离,降低了组件之间的耦合度,同时也更容易管理数据和UI的变化。
#### 4.2 使用LiveData更新UI界面
在Android应用开发中,常常需要根据数据的变化来动态更新UI界面。使用LiveData,我们可以很方便地实现这一过程,而无需手动管理数据的变化和UI的更新。
下面是一个使用LiveData更新UI界面的示例代码:
```java
// 在ViewModel中定义一个包含计时器数据的LiveData
public class TimerViewModel extends ViewModel {
private MutableLiveData<Long> timerLiveData = new MutableLiveData<>();
private long initialTime = System.currentTimeMillis();
public TimerViewModel() {
// 使用Handler每隔1秒更新LiveData中的数据
new Handler(Looper.getMainLooper()).postDelayed(new Runnable() {
@Override
public void run() {
long newValue = System.currentTimeMillis() - initialTime;
timerLiveData.setValue(newValue);
new Handler(Looper.getMainLooper()).postDelayed(this, 1000);
}
}, 1000);
}
public LiveData<Long> getTimerLiveData() {
return timerLiveData;
}
}
// 在Activity或者Fragment中观察ViewModel中的LiveData数据更新UI界面
public class TimerActivity extends AppCompatActivity {
private TimerViewModel timerViewModel;
private TextView timerTextView;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_timer);
timerTextView = findViewById(R.id.timerTextView);
timerViewModel = ViewModelProviders.of(this).get(TimerViewModel.class);
timerViewModel.getTimerLiveData().observe(this, time -> {
// 更新UI界面
timerTextView.setText("Timer: " + time);
});
}
}
```
通过上述代码,可以看到使用LiveData可以轻松地实现UI界面根据数据变化而更新的功能,而不需要手动处理数据变化和UI更新的逻辑。
#### 4.3 使用LiveData进行跨组件通信
除了简化网络请求和UI更新的过程,LiveData还可以作为一种有效的跨组件通信方式。多个组件可以观察同一个LiveData对象,从而实现数据共享和通信。
下面是一个使用LiveData进行跨组件通信的示例代码:
```java
// 在ViewModel中定义一个保存用户登录状态的LiveData
public class UserViewModel extends ViewModel {
private MutableLiveData<Boolean> loggedInLiveData = new MutableLiveData<>();
public void setLoggedIn(boolean loggedIn) {
loggedInLiveData.setValue(loggedIn);
}
public LiveData<Boolean> getLoggedInLiveData() {
return loggedInLiveData;
}
}
// 在Fragment A中设置LiveData数据
public class LoginFragment extends Fragment {
private UserViewModel userViewModel;
@Override
public void onViewCreated(View view, @Nullable Bundle savedInstanceState) {
super.onViewCreated(view, savedInstanceState);
userViewModel = ViewModelProviders.of(requireActivity()).get(UserViewModel.class);
// 设置用户登录状态为true
userViewModel.setLoggedIn(true);
}
}
// 在Fragment B中观察LiveData数据
public class ProfileFragment extends Fragment {
private UserViewModel userViewModel;
@Override
public void onViewCreated(View view, @Nullable Bundle savedInstanceState) {
super.onViewCreated(view, savedInstanceState);
userViewModel = ViewModelProviders.of(requireActivity()).get(UserViewModel.class);
userViewModel.getLoggedInLiveData().observe(getViewLifecycleOwner(), loggedIn -> {
if (loggedIn) {
// 执行相应操作,如显示用户信息
showUserInfo();
} else {
// 执行相应操作,如跳转到登录界面
navigateToLogin();
}
});
}
}
```
通过上述代码,可以看到使用LiveData可以很方便地实现不同组件之间的数据共享和通信,从而简化了Android应用程序中跨组件交互的过程。 LiveData的强大功能使得Android应用的开发变得更加简单和高效。
## 5. LiveData的高级用法
在前面的章节中,我们已经了解了LiveData的基本用法,但LiveData还提供了一些高级用法,可以进一步增强其功能和灵活性。
### 5.1 转换和过滤LiveData数据
LiveData提供了一些方法来转换和过滤数据。这些方法可以帮助我们对LiveData中的数据进行处理,以满足不同的需求。
下面介绍一些常用的转换和过滤方法:
- `map`方法:可以将LiveData中的数据转换为另一种类型的数据。例如,将一个整数LiveData转换为字符串LiveData。
```java
LiveData<Integer> sourceLiveData = new MutableLiveData<>();
LiveData<String> transformedLiveData = Transformations.map(sourceLiveData, input -> "Value: " + input);
```
- `switchMap`方法:可以根据某个LiveData的值动态地切换到另一个LiveData。例如,根据用户ID获取用户信息。
```java
LiveData<String> userIdLiveData = new MutableLiveData<>();
LiveData<User> userLiveData = Transformations.switchMap(userIdLiveData, userId -> userRepository.getUserById(userId));
```
- `filter`方法:可以根据条件过滤LiveData中的数据。例如,筛选出大于10的整数。
```java
LiveData<Integer> sourceLiveData = new MutableLiveData<>();
LiveData<Integer> filteredLiveData = Transformations.filter(sourceLiveData, input -> input > 10);
```
通过这些转换和过滤方法,我们可以灵活地处理LiveData中的数据,满足各种业务需求。
### 5.2 使用MediatorLiveData组合多个LiveData
MediatorLiveData是LiveData的一个子类,可以用于组合多个LiveData。通过MediatorLiveData,我们可以将多个LiveData的数据合并或处理后输出。
下面是一个使用MediatorLiveData的示例,假设我们有两个LiveData,分别用于获取用户的姓名和年龄。我们可以通过MediatorLiveData将这两个LiveData合并为一个LiveData,输出用户的姓名和年龄。
```java
LiveData<String> nameLiveData = userRepository.getUserName();
LiveData<Integer> ageLiveData = userRepository.getUserAge();
MediatorLiveData<UserInfo> userInfoLiveData = new MediatorLiveData<>();
userInfoLiveData.addSource(nameLiveData, name -> userInfoLiveData.setValue(new UserInfo(name, ageLiveData.getValue())));
userInfoLiveData.addSource(ageLiveData, age -> userInfoLiveData.setValue(new UserInfo(nameLiveData.getValue(), age)));
class UserInfo {
String name;
int age;
public UserInfo(String name, int age) {
this.name = name;
this.age = age;
}
}
```
在上面的示例中,每当姓名或年龄的LiveData发生变化时,我们都重新生成一个新的UserInfo对象,并通过setValue方法进行输出。
### 5.3 使用Transformations转换LiveData数据类型
Transformations类提供了一些静态方法来进行LiveData数据类型的转换。这些方法可以帮助我们在LiveData之间进行类型转换,以适应不同的需求。
下面是一些常用的Transformations方法:
- `map`方法:将一个LiveData对象转换为另一种类型的LiveData对象。
```java
LiveData<Integer> sourceLiveData = new MutableLiveData<>();
LiveData<String> transformedLiveData = Transformations.map(sourceLiveData, input -> "Value: " + input);
```
- `switchMap`方法:根据某个LiveData的值动态地切换到另一个LiveData对象。
```java
LiveData<String> userIdLiveData = new MutableLiveData<>();
LiveData<User> userLiveData = Transformations.switchMap(userIdLiveData, userId -> userRepository.getUserById(userId));
```
通过使用Transformations类,我们可以轻松地在LiveData之间进行数据类型的转换,提高代码的复用性和灵活性。
在本章节中,我们介绍了LiveData的一些高级用法,包括数据转换和过滤、使用MediatorLiveData组合多个LiveData、使用Transformations转换LiveData数据类型。这些高级用法可以帮助我们更加灵活地使用LiveData,满足复杂的业务需求。
### 6. 总结与展望
在本文中,我们详细介绍了LiveData在Android应用程序中简化数据交互的作用和方法。通过对LiveData的基本用法和优势的讲解,读者可以清晰地了解LiveData的核心概念及其在实际开发中的应用场景。
通过使用LiveData,开发者可以更加轻松地实现数据更新和UI更新之间的关联,避免常见的内存泄漏问题,并且得到了异步数据处理的支持。这些特点使得LiveData成为Android应用程序中不可或缺的组件之一。
未来,随着Android开发技术的不断发展,我们相信LiveData会在更多领域展现其强大的功能,例如在大规模应用中的性能优化、更灵活的数据变换方式等方面。希望开发者们能够充分利用LiveData的特性,从而提升应用的质量和用户体验。
通过本文的阅读,读者可以对LiveData有一个全面的了解,相信在实际的应用开发中会有所帮助。
以上就是对LiveData的总结和展望,希望本文对您有所帮助。
0
0
相关推荐
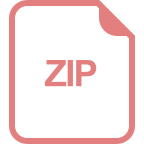
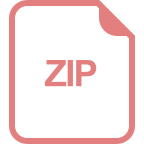
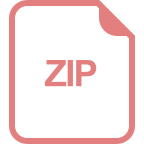
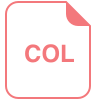
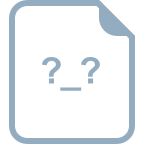
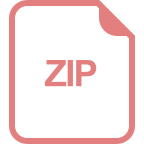
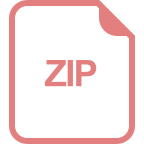
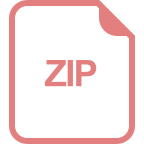
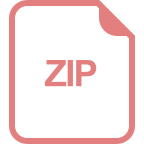