揭秘字符串匹配算法:从Boyer-Moore到KMP的终极指南
发布时间: 2024-08-28 04:22:42 阅读量: 36 订阅数: 22 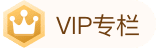
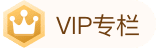
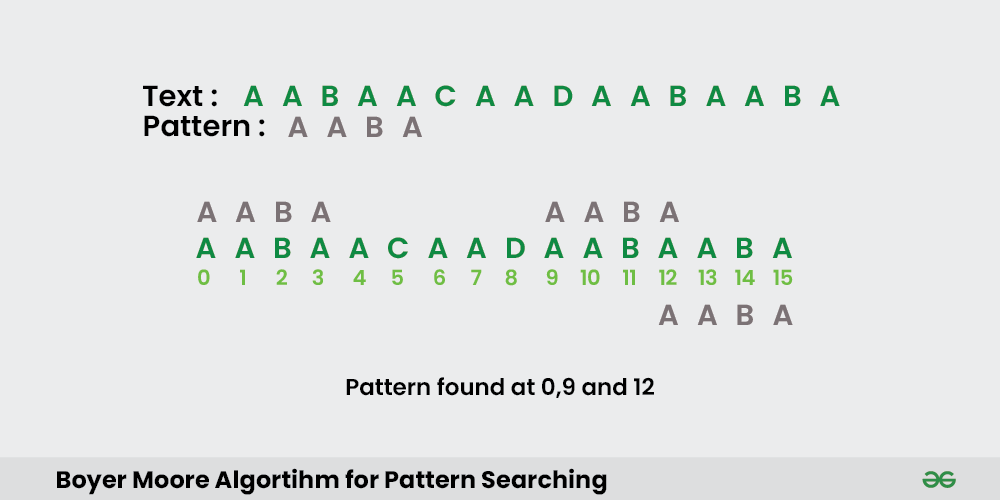
# 1. 字符串匹配算法概述
字符串匹配算法是计算机科学中用来在给定文本中查找特定模式或子串的算法。这些算法在各种应用中至关重要,包括文本搜索、模式识别和数据验证。
字符串匹配算法通常分为两类:朴素算法和高效算法。朴素算法简单易懂,但效率较低。高效算法利用模式的特征来提高匹配速度,例如Boyer-Moore算法和Knuth-Morris-Pratt(KMP)算法。
本章将介绍字符串匹配算法的基本概念,包括模式匹配问题、朴素算法和高效算法的分类。
# 2. Boyer-Moore算法
Boyer-Moore算法是一种字符串匹配算法,它以其高效性和易于实现而闻名。该算法由Robert S. Boyer和J Strother Moore于1977年提出,它通过利用模式字符串中字符的特征来加速匹配过程。
### 2.1 Boyer-Moore算法原理
Boyer-Moore算法的基本原理是:
1. **坏字符规则:**如果模式字符串中的某个字符与文本字符串中当前位置的字符不匹配,则将模式字符串向右移动,移动距离等于该字符在模式字符串中最后出现的位置与当前位置的差值。
2. **好后缀规则:**如果模式字符串中的某个后缀与文本字符串中当前位置的后缀匹配,则将模式字符串向右移动,移动距离等于模式字符串中该后缀的长度。
### 2.2 Boyer-Moore算法的实现
#### 2.2.1 坏字符规则
坏字符规则的实现如下:
```python
def bad_character_rule(pattern, text, i):
"""
实现坏字符规则。
参数:
pattern (str): 模式字符串。
text (str): 文本字符串。
i (int): 文本字符串中当前位置。
返回:
int: 模式字符串向右移动的距离。
"""
if i < len(text) and pattern[len(pattern) - 1] == text[i]:
return 1
last_occurrence = pattern.rfind(text[i], 0, len(pattern) - 1)
return len(pattern) - 1 - last_occurrence
```
**代码逻辑解读:**
* 如果文本字符串中当前位置的字符与模式字符串中的最后一个字符匹配,则移动距离为1。
* 否则,在模式字符串中查找文本字符串中当前位置的字符最后出现的位置,并计算移动距离为模式字符串的长度减去1减去最后出现的位置。
#### 2.2.2 好后缀规则
好后缀规则的实现如下:
```python
def good_suffix_rule(pattern, text, i):
"""
实现好后缀规则。
参数:
pattern (str): 模式字符串。
text (str): 文本字符串。
i (int): 文本字符串中当前位置。
返回:
int: 模式字符串向右移动的距离。
"""
j = len(pattern) - 1
while j >= 0 and pattern[j] == text[i - j]:
j -= 1
if j == -1:
return len(pattern)
return len(pattern) - 1 - j
```
**代码逻辑解读:**
* 从模式字符串的最后一个字符开始,逐个比较模式字符串和文本字符串中当前位置的后缀。
* 如果后缀匹配,则继续比较下一个字符。
* 如果后缀不匹配,则移动距离为模式字符串的长度减去1减去匹配的后缀长度。
# 3. Knuth-Morris-Pratt(KMP)算法
### 3.1 KMP算法原理
Knuth-Morris-Pratt(KMP)算法是一种字符串匹配算法,它利用模式串中的部分信息来加速匹配过程。KMP算法的核心思想是:
- **失配时不回溯:**当模式串和文本串不匹配时,KMP算法不会回溯到模式串的开头,而是根据模式串中已匹配的部分信息,跳过一些字符,继续匹配。
- **利用失配函数:**失配函数是一个数组,其中每个元素表示模式串中某个字符失配后,下一个匹配位置应该从哪里开始。
### 3.2 KMP算法的实现
#### 3.2.1 失效函数的计算
失效函数的计算是KMP算法的关键步骤。它可以预先计算出模式串中每个字符失配后的下一个匹配位置。
```python
def compute_failure_function(pattern):
"""计算失配函数。
Args:
pattern (str): 模式串。
Returns:
list[int]: 失效函数。
"""
m = len(pattern)
failure_function = [0] * m
j = 0
i = 1
while i < m:
if pattern[i] == pattern[j]:
j += 1
failure_function[i] = j
i += 1
else:
if j > 0:
j = failure_function[j - 1]
else:
failure_function[i] = 0
i += 1
return failure_function
```
**代码逻辑逐行解读:**
1. 初始化失效函数数组`failure_function`,长度与模式串相等,第一个元素为0。
2. 设置两个指针`j`和`i`,`j`指向模式串的第一个字符,`i`指向第二个字符。
3. 如果模式串的第`i`个字符与第`j`个字符相等,则`j`和`failure_function[i]`都加1,`i`也加1。
4. 如果模式串的第`i`个字符与第`j`个字符不相等,则判断`j`是否大于0。
- 如果`j`大于0,则将`j`更新为`failure_function[j - 1]`。
- 如果`j`等于0,则将`failure_function[i]`更新为0,并使`i`加1。
5. 重复步骤3和4,直到`i`等于模式串的长度。
#### 3.2.2 字符匹配过程
失效函数计算完成后,就可以进行字符匹配。
```python
def kmp_match(text, pattern):
"""使用KMP算法匹配模式串。
Args:
text (str): 文本串。
pattern (str): 模式串。
Returns:
list[int]: 匹配到的位置索引。
"""
n = len(text)
m = len(pattern)
matches = []
failure_function = compute_failure_function(pattern)
j = 0
i = 0
while i < n:
if pattern[j] == text[i]:
j += 1
i += 1
if j == m:
matches.append(i - j)
j = failure_function[j - 1]
else:
if j > 0:
j = failure_function[j - 1]
else:
i += 1
return matches
```
**代码逻辑逐行解读:**
1. 初始化匹配结果列表`matches`。
2. 设置两个指针`j`和`i`,`j`指向模式串的第一个字符,`i`指向文本串的第一个字符。
3. 如果模式串的第`j`个字符与文本串的第`i`个字符相等,则`j`和`i`都加1。
4. 如果模式串的第`j`个字符与文本串的第`i`个字符不相等,则判断`j`是否大于0。
- 如果`j`大于0,则将`j`更新为`failure_function[j - 1]`。
- 如果`j`等于0,则使`i`加1。
5. 重复步骤3和4,直到`i`等于文本串的长度。
6. 如果`j`等于模式串的长度,则说明匹配成功,将`i - j`添加到`matches`列表中,并更新`j`为`failure_function[j - 1]`。
7. 返回`matches`列表。
### KMP算法与Boyer-Moore算法的比较
KMP算法和Boyer-Moore算法都是字符串匹配算法,但它们有不同的特点:
| 特征 | KMP算法 | Boyer-Moore算法 |
|---|---|---|
| 失配处理 | 利用失配函数,不回溯 | 利用坏字符规则和好后缀规则,部分回溯 |
| 时间复杂度 | O(n + m) | O(nm) |
| 空间复杂度 | O(m) | O(m) |
| 适用场景 | 模式串较长,重复较多 | 模式串较短,重复较少 |
# 4. 字符串匹配算法的比较**
**4.1 Boyer-Moore算法与KMP算法的优缺点**
Boyer-Moore算法和KMP算法是两种最流行的字符串匹配算法,各有优缺点。
| 特征 | Boyer-Moore算法 | KMP算法 |
|---|---|---|
| 时间复杂度 | O(m + n) | O(m + n) |
| 空间复杂度 | O(m) | O(m) |
| 预处理 | 需要 | 需要 |
| 模式长度 | 适用于模式较长的情况 | 适用于模式较短的情况 |
| 文本长度 | 适用于文本较短的情况 | 适用于文本较长的情况 |
| 坏字符规则 | 有助于跳过不匹配的字符 | 没有 |
| 好后缀规则 | 有助于跳过冗余的比较 | 没有 |
| 失效函数 | 没有 | 有 |
**4.2 其他字符串匹配算法(如Rabin-Karp算法)**
除了Boyer-Moore算法和KMP算法,还有其他字符串匹配算法,如Rabin-Karp算法。
Rabin-Karp算法是一种基于哈希函数的字符串匹配算法。它使用一个哈希函数将模式和文本中的子串映射到一个整数。如果两个子串的哈希值相同,则进一步比较它们是否相等。
Rabin-Karp算法的时间复杂度为O(m + n),空间复杂度为O(1)。它适用于模式和文本都较长的情况。
**代码块:**
```python
def rabin_karp(pattern, text):
"""
Rabin-Karp字符串匹配算法
:param pattern: 模式字符串
:param text: 文本字符串
:return: 模式在文本中的起始索引,如果没有匹配则返回-1
"""
# 计算模式和文本的哈希值
pattern_hash = hash(pattern)
text_hash = hash(text[:len(pattern)])
# 滚动哈希值
for i in range(1, len(text) - len(pattern) + 1):
text_hash = (text_hash - hash(text[i - 1])) * 31 + hash(text[i + len(pattern) - 1])
# 比较哈希值
if pattern_hash == text_hash:
if pattern == text[i:i + len(pattern)]:
return i
return -1
```
**代码逻辑分析:**
该代码块实现了Rabin-Karp算法。它首先计算模式和文本的哈希值。然后,它使用一个滚动哈希函数来更新文本的哈希值,以便在每个步骤中比较模式和文本的哈希值。如果哈希值相同,则进一步比较模式和文本的子串是否相等。如果相等,则返回模式在文本中的起始索引。否则,继续滚动哈希值并比较。
# 5. 字符串匹配算法在实践中的应用
### 5.1 文本搜索和替换
字符串匹配算法在文本搜索和替换中发挥着至关重要的作用。文本搜索是指在给定的文本中查找特定模式或子字符串,而文本替换是指将文本中的特定模式替换为另一个字符串。
**代码块 1:使用 Boyer-Moore 算法进行文本搜索**
```python
def boyer_moore_search(text, pattern):
"""
使用 Boyer-Moore 算法在文本中搜索模式。
参数:
text (str): 要搜索的文本。
pattern (str): 要查找的模式。
返回:
int: 模式在文本中出现的位置(如果存在),否则返回 -1。
"""
m = len(pattern)
n = len(text)
# 创建坏字符表
bad_char_table = create_bad_char_table(pattern)
# 创建好后缀表
good_suffix_table = create_good_suffix_table(pattern)
# 偏移量
shift = 0
while shift <= n - m:
# 比较模式和文本中的字符
i = m - 1
while i >= 0 and pattern[i] == text[shift + i]:
i -= 1
# 如果模式匹配成功
if i == -1:
return shift
# 计算偏移量
char = text[shift + m]
shift += max(bad_char_table.get(char, m), good_suffix_table[i])
return -1
```
**逻辑分析:**
该代码块实现了 Boyer-Moore 算法进行文本搜索。它首先创建坏字符表和好后缀表,然后使用这些表来计算偏移量。偏移量表示模式在文本中可能匹配的下一个位置。如果模式匹配成功,函数返回匹配的位置;否则,返回 -1。
### 5.2 模式识别
字符串匹配算法还可用于模式识别,例如图像处理、语音识别和自然语言处理。在图像处理中,字符串匹配算法可用于识别图像中的特定形状或图案。在语音识别中,它们可用于识别语音中的特定单词或短语。在自然语言处理中,它们可用于识别文本中的语法结构或语义模式。
**代码块 2:使用 KMP 算法进行模式识别**
```python
def kmp_pattern_recognition(text, pattern):
"""
使用 KMP 算法进行模式识别。
参数:
text (str): 要搜索的文本。
pattern (str): 要识别的模式。
返回:
list: 模式在文本中出现的所有位置。
"""
m = len(pattern)
n = len(text)
# 计算失效函数
failure_function = compute_failure_function(pattern)
# 匹配模式
i = 0
j = 0
matches = []
while i < n:
if pattern[j] == text[i]:
i += 1
j += 1
if j == m:
matches.append(i - j)
j = failure_function[j - 1]
elif i < n and pattern[j] != text[i]:
if j != 0:
j = failure_function[j - 1]
else:
i += 1
return matches
```
**逻辑分析:**
该代码块实现了 KMP 算法进行模式识别。它首先计算失效函数,然后使用失效函数来匹配模式。失效函数表示模式中每个字符失配时应该跳转到的位置。如果模式匹配成功,函数将匹配的位置添加到结果列表中。
### 5.3 数据验证
字符串匹配算法还可用于数据验证,例如表单验证、输入验证和数据清理。在表单验证中,它们可用于验证用户输入的有效性,例如电子邮件地址、电话号码或邮政编码。在输入验证中,它们可用于验证输入的数据是否符合特定格式或约束。在数据清理中,它们可用于识别和删除数据中的错误或不一致之处。
**代码块 3:使用 Rabin-Karp 算法进行数据验证**
```python
def rabin_karp_data_validation(text, pattern):
"""
使用 Rabin-Karp 算法进行数据验证。
参数:
text (str): 要验证的文本。
pattern (str): 要验证的模式。
返回:
bool: 模式是否在文本中。
"""
m = len(pattern)
n = len(text)
# 计算模式和文本的哈希值
pattern_hash = hash(pattern)
text_hash = hash(text[:m])
# 滚动哈希
for i in range(1, n - m + 1):
text_hash = (text_hash - hash(text[i - 1]) * pow(256, m - 1)) % pow(256, m) + hash(text[i + m - 1]) * pow(256, m - 1) % pow(256, m)
# 比较哈希值
if text_hash == pattern_hash:
return True
return False
```
**逻辑分析:**
该代码块实现了 Rabin-Karp 算法进行数据验证。它首先计算模式和文本的哈希值,然后使用滚动哈希技术来计算文本中每个窗口的哈希值。如果文本中某个窗口的哈希值与模式的哈希值相等,则函数返回 True,表示模式在文本中存在;否则,返回 False。
# 6. 字符串匹配算法的未来发展**
随着字符串匹配算法在各种应用中的广泛使用,其未来发展趋势主要集中在以下两个方面:
**6.1 并行字符串匹配算法**
随着多核处理器和并行计算技术的普及,并行字符串匹配算法应运而生。这些算法通过将字符串匹配任务分配到多个处理器上并行执行,可以显著提高匹配速度。
常见的并行字符串匹配算法包括:
- **基于OpenMP的并行算法:**利用OpenMP编程模型将匹配任务分配到多个线程上。
- **基于CUDA的并行算法:**利用CUDA编程模型将匹配任务分配到GPU上。
- **基于分布式系统的并行算法:**利用分布式系统将匹配任务分配到多个节点上。
**6.2 近似字符串匹配算法**
在某些情况下,精确的字符串匹配并不总是必要的。近似字符串匹配算法可以快速找到与给定模式相似但并不完全匹配的字符串。
常见的近似字符串匹配算法包括:
- **编辑距离算法:**计算两个字符串之间的编辑操作(如插入、删除、替换)次数,从而衡量相似性。
- **Jaccard相似性算法:**计算两个字符串中公共元素占总元素的比例,从而衡量相似性。
- **Cosine相似性算法:**计算两个字符串中公共元素的余弦相似度,从而衡量相似性。
0
0
相关推荐
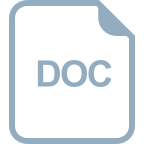
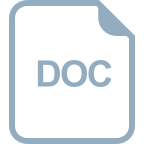
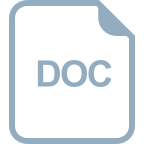
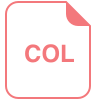
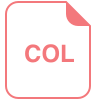
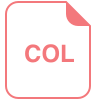
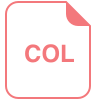
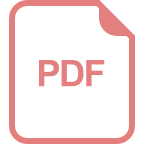
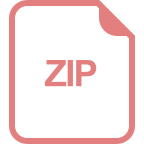