【Python游戏开发实战】:pygame中的面向对象编程技巧揭秘
发布时间: 2024-10-05 13:33:41 阅读量: 2 订阅数: 4 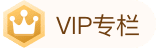
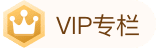

# 1. 面向对象编程基础与pygame概述
## 1.1 面向对象编程概念回顾
面向对象编程(Object-Oriented Programming,OOP)是一种编程范式,以“对象”为程序的基本单位,利用对象的属性和方法来描述事物的行为和状态。它强调封装、继承和多态三个主要特点,对象由数据(属性)和代码(方法)组成,以类为模板创建。
```python
# 示例:定义一个简单的Python类
class Car:
def __init__(self, brand, model):
self.brand = brand
self.model = model
def start(self):
print(f"{self.brand} {self.model} is starting.")
# 创建一个Car类的实例
my_car = Car("Toyota", "Corolla")
my_car.start() # 输出: Toyota Corolla is starting.
```
## 1.2 pygame的简介
pygame是一个开源的Python库,它为游戏开发提供了一系列模块,用于图形渲染、声音播放、事件处理等。它广泛应用于2D游戏的快速开发,支持跨平台操作,是学习面向对象编程和游戏开发的良好平台。
```python
# 示例:pygame的简单初始化和使用
import pygame
import sys
# 初始化pygame
pygame.init()
# 设置窗口大小
size = width, height = 640, 480
screen = pygame.display.set_mode(size)
# 主循环,直到用户发出退出请求
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
```
面向对象编程的原理和pygame库的使用为游戏开发者提供了一个强有力的工具集合,让游戏开发既高效又系统。在后续章节中,我们将深入探讨pygame中的类和对象,以及面向对象编程在游戏开发中的高级应用和设计模式。
# 2. pygame中的类和对象
## 2.1 面向对象编程概念回顾
### 2.1.1 类与对象的关系
面向对象编程(Object-Oriented Programming,OOP)是一种编程范式,其核心思想是数据抽象和对象复用。在面向对象的世界中,类(Class)是创建对象的模板,而对象(Object)则是根据类创建的实例。
类定义了一组属性(数据结构)和方法(行为),对象则是这些属性和方法的具体实现。打个比方,类像是蓝图或食谱,它描述了如何构造一个对象;而对象则是实际的建筑物或蛋糕,它是根据蓝图或食谱制作出来的实体。
### 2.1.2 类的定义和对象的创建
在Python中,定义一个类使用`class`关键字,类的命名通常遵循大驼峰命名法,即每个单词的首字母大写,例如`GameWindow`。
```python
class GameWindow:
def __init__(self, title, width, height):
self.title = title
self.width = width
self.height = height
self.screen = pygame.display.set_mode((width, height))
def run(self):
running = True
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
self.screen.fill((0, 0, 0)) # 清屏操作
pygame.display.flip() # 更新显示
pygame.quit()
```
要创建这个类的实例(即对象),只需调用类名并传入相应的参数:
```python
my_window = GameWindow("My Game", 800, 600)
my_window.run()
```
## 2.2 pygame中的基本类结构
### 2.2.1 游戏循环类Game
游戏循环类负责整个游戏的主要运行逻辑。在pygame中,一个简单游戏循环通常包括初始化游戏环境、游戏主循环、事件处理、游戏更新和渲染这几个部分。
```python
class Game:
def __init__(self):
pygame.init()
# 进行其他初始化设置
self.running = True
def run(self):
while self.running:
self.handle_events()
self.update_game()
self.render()
pygame.quit()
def handle_events(self):
for event in pygame.event.get():
if event.type == pygame.QUIT:
self.running = False
def update_game(self):
# 更新游戏逻辑
pass
def render(self):
# 渲染游戏画面
pass
```
### 2.2.2 事件处理类Event
事件处理类负责处理游戏中的事件,比如按键事件、鼠标点击事件等。pygame使用事件队列来管理事件,开发者需要定期调用`pygame.event.get()`来获取队列中的事件。
```python
class EventManager:
def handle_events(self):
for event in pygame.event.get():
if event.type == pygame.QUIT:
self.quit()
elif event.type == pygame.KEYDOWN:
self.on_key_down(event.key)
# 更多事件处理
def on_key_down(self, key):
# 按键处理逻辑
pass
def quit(self):
# 退出游戏逻辑
pygame.quit()
```
### 2.2.3 碰撞检测类Rect
碰撞检测是游戏开发中的一个基本功能,pygame提供了一个方便的矩形类`pygame.Rect`来实现这个功能。`Rect`对象可以检测点、线或另一个矩形对象的碰撞。
```python
class CollisionDetector:
def __init__(self):
self.player_rect = pygame.Rect(100, 100, 50, 50)
self.enemy_rect = pygame.Rect(150, 100, 50, 50)
def check_collision(self):
return self.player_rect.colliderect(self.enemy_rect)
```
## 2.3 面向对象在pygame中的高级应用
### 2.3.1 继承在游戏开发中的运用
继承是面向对象编程中一种非常强大的特性,它允许我们定义一个类(子类)继承另一个类(父类)的所有属性和方法,并且可以扩展或重写父类的属性和方法。在游戏开发中,继承可以用来创建具有不同行为但共享相同基本属性的对象。
```python
class Character(pygame.sprite.Sprite):
def __init__(self, x, y, image_file):
super().__init__()
self.image = pygame.image.load(image_file)
self.rect = self.image.get_rect()
self.rect.x = x
self.rect.y = y
def update(self):
# 更新角色位置等逻辑
pass
class Player(Character):
def __init__(self, x, y, image_file):
super().__init__(x, y, image_file)
def update(self):
# 扩展更新逻辑,比如跳跃、攻击等
super().update()
# 其他特定玩家行为
```
### 2.3.2 多态与事件处理的结合
多态是面向对象编程中的另一个关键特性,它指的是同一个方法调用,由于对象的不同而产生不同的行为。在事件处理中,多态可以让我们对同一个事件做出不同的响应。
```python
class Game:
# ... 其他方法 ...
def handle_events(self):
```
0
0
相关推荐
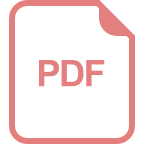
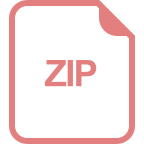
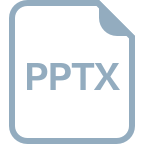
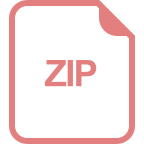
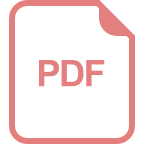
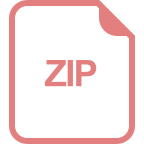
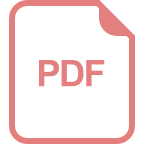
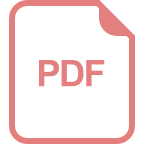
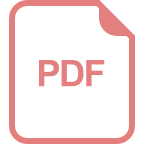