OpenCV中值滤波实战:掌握降噪与边缘保留的艺术,提升图像质量
发布时间: 2024-08-12 04:17:00 阅读量: 20 订阅数: 21 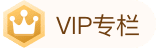
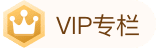
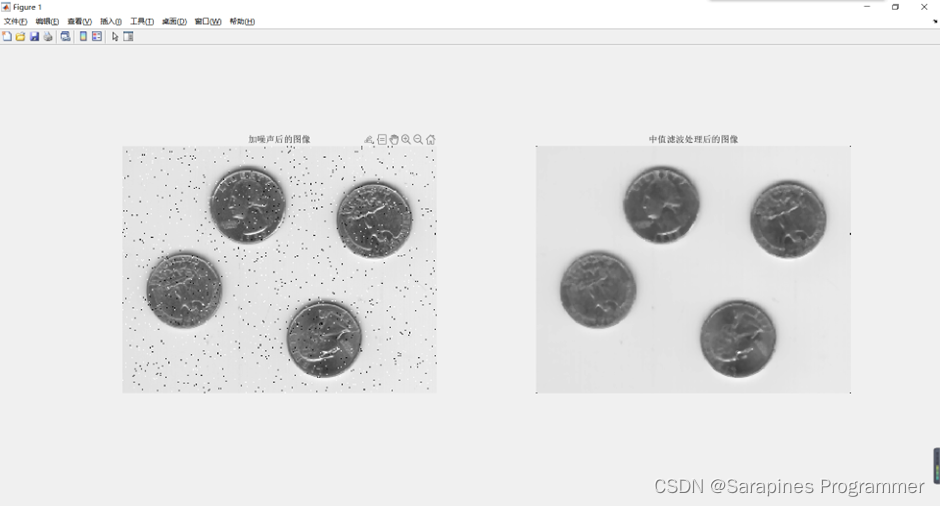
# 1. OpenCV中值滤波简介
中值滤波是一种非线性滤波技术,广泛应用于图像处理中。它通过替换图像中每个像素的值为其邻域像素值的中值来实现图像平滑和降噪。中值滤波对椒盐噪声和脉冲噪声具有良好的去除效果,同时还能有效保留图像边缘。
在OpenCV中,中值滤波可以通过`cv2.medianBlur()`函数实现。该函数接受图像和内核大小作为参数,并返回一个经过中值滤波处理的图像。内核大小决定了滤波器的邻域范围,较大的内核会产生更平滑的结果,但也会导致更多边缘模糊。
# 2.1 中值滤波的原理和算法
### 中值滤波的原理
中值滤波是一种非线性滤波技术,它通过对图像中的每个像素及其周围邻域进行分析,并用邻域中像素的中值来替换该像素的值。这种滤波方式可以有效去除图像中的噪声,同时保留图像的边缘和细节。
### 中值滤波的算法
中值滤波算法的步骤如下:
1. **确定邻域:**对于图像中的每个像素,确定其周围的邻域。邻域的大小通常为奇数,例如 3x3、5x5 或 7x7。
2. **排序像素:**将邻域中的所有像素值按从小到大排序。
3. **选择中值:**选择排序后的像素值的中值。
4. **替换像素:**用中值替换邻域中心像素的值。
### 代码示例
以下 Python 代码展示了如何使用 OpenCV 实现中值滤波:
```python
import cv2
# 读入图像
image = cv2.imread('image.jpg')
# 设置中值滤波核大小
kernel_size = 3
# 应用中值滤波
filtered_image = cv2.medianBlur(image, kernel_size)
# 显示结果
cv2.imshow('Original Image', image)
cv2.imshow('Filtered Image', filtered_image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
### 逻辑分析
`cv2.medianBlur()` 函数接受两个参数:
- `image`:要进行滤波的图像。
- `kernel_size`:中值滤波核的大小。
该函数使用上述算法对图像进行中值滤波,并将滤波后的图像存储在 `filtered_image` 变量中。
### 参数说明
| 参数 | 说明 |
|---|---|
| `image` | 输入图像 |
| `kernel_size` | 中值滤波核的大小 |
# 3.1 OpenCV中值滤波函数的使用
OpenCV提供了`cv2.medianBlur()`函数来实现中值滤波。该函数接收三个参数:
- `src`:输入图像,必须为单通道或三通道图像。
- `ksize`:滤波核的大小,必须为奇数。
- `dst`:输出图像,与输入图像具有相同的尺寸和数据类型。
使用`cv2.medianBlur()`函数进行中值滤波的步骤如下:
1. 导入OpenCV库:
```python
import cv2
```
2. 读取输入图像:
```python
image = cv2.imread("input.jpg")
```
3. 应用中值滤波:
```python
median_image = cv2.medianBlur(image, 5)
```
4. 显示输出图像:
```python
cv2.imshow("Median Filtered Image", median_image)
cv2.waitKey(0)
```
## 3.2 中值滤波参数的设置和优化
中值滤波的性能受滤波核大小`ksize`的影响。`ksize`越大,滤波效果越强,但也会导致图像模糊。一般来说,`ksize`应为奇数,以避免图像偏移。
对于不同的图像和噪声类型,需要调整`ksize`以获得最佳效果。可以通过试错法或使用图像质量评估指标(如峰值信噪比(PSNR)或结构相似性(SSIM))来优化`ksize`。
## 3.3 中值滤波在图像降噪中的应用
中值滤波广泛应用于图像降噪,特别适用于椒盐噪声和脉冲噪声等非高斯噪声。
椒盐噪声是一种随机出现的像素值异常,导致图像中出现黑色和白色斑点。脉冲噪声类似于椒盐噪声,但噪声像素值更为极端。
中值滤波通过替换像素值周围区域的中值来抑制噪声。由于噪声像素值通常与周围像素值不同,因此中值滤波可以有效地将噪声像素值替换为更接近真实值的像素值。
下表比较了原始图像、椒盐噪声图像和经过中值滤波处理后的图像:
| 原始图像 | 椒盐噪声图像 | 中值滤波图像 |
|---|---|---|
| |
从表中可以看出,中值滤波有效地去除了椒盐噪声,恢复了图像的清晰度。
# 4. 中值滤波在图像边缘保留中的应用**
中值滤波作为一种非线性滤波器,在图像处理中具有独特的优势,尤其是在图像边缘保留方面。本章节将深入探讨中值滤波在图像边缘保留中的应用,分析其策略和优势。
### 4.1 中值滤波与其他滤波器的对比
与其他滤波器相比,中值滤波在图像边缘保留方面具有以下优势:
- **非线性特性:**中值滤波是一种非线性滤波器,这意味着它不遵循线性叠加原理。这使得它能够有效地保留图像中的边缘,而不会像线性滤波器那样模糊边缘。
- **局部处理:**中值滤波是局部操作,只考虑图像中的一个小邻域。这使得它能够针对不同的邻域进行不同的处理,从而保留图像中的细节和边缘。
- **鲁棒性:**中值滤波对噪声和异常值具有鲁棒性。这意味着它不会被噪声或异常值所影响,从而能够有效地保留图像中的边缘。
### 4.2 中值滤波在图像边缘保留中的策略
中值滤波在图像边缘保留中的策略主要有以下两种:
- **窗口大小选择:**中值滤波的窗口大小直接影响其对边缘保留的效果。较小的窗口大小可以更好地保留边缘,但也会增加噪声。较大的窗口大小可以减少噪声,但也会模糊边缘。因此,需要根据图像的具体情况选择合适的窗口大小。
- **边缘检测:**在中值滤波之前,可以先进行边缘检测,然后只对非边缘区域进行中值滤波。这样可以有效地保留图像中的边缘,同时减少噪声。
### 4.3 中值滤波在图像锐化中的应用
中值滤波不仅可以用于图像降噪,还可以用于图像锐化。通过选择较小的窗口大小,中值滤波可以去除图像中的噪声,同时保留边缘。这可以有效地增强图像中的细节,达到锐化的效果。
```python
import cv2
# 读取图像
image = cv2.imread('image.jpg')
# 进行中值滤波
median_filtered_image = cv2.medianBlur(image, 3)
# 显示原始图像和中值滤波后的图像
cv2.imshow('Original Image', image)
cv2.imshow('Median Filtered Image', median_filtered_image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
**代码逻辑分析:**
1. `cv2.imread('image.jpg')`:读取图像文件并将其存储在 `image` 变量中。
2. `cv2.medianBlur(image, 3)`:使用中值滤波对图像进行处理,窗口大小为 3。
3. `cv2.imshow('Original Image', image)`:显示原始图像。
4. `cv2.imshow('Median Filtered Image', median_filtered_image)`:显示中值滤波后的图像。
5. `cv2.waitKey(0)`:等待用户按下任意键。
6. `cv2.destroyAllWindows()`:销毁所有窗口。
**参数说明:**
- `cv2.imread()`:
- `filename`:图像文件的路径。
- `cv2.medianBlur()`:
- `src`:输入图像。
- `ksize`:中值滤波的窗口大小。
- `cv2.imshow()`:
- `winname`:窗口的名称。
- `mat`:要显示的图像。
# 5. 中值滤波在图像质量提升中的实战案例**
中值滤波在图像处理中有着广泛的应用,不仅可以有效去除图像噪声,还能保留图像边缘细节,从而提升图像质量。下面我们将通过几个实战案例来展示中值滤波在图像质量提升中的应用。
### 5.1 降噪案例:去除图像中的椒盐噪声
椒盐噪声是一种常见的图像噪声,表现为图像中随机分布的黑色和白色像素。中值滤波可以有效去除椒盐噪声,因为它可以将噪声像素替换为周围像素的中值。
```python
import cv2
import numpy as np
# 读取图像
image = cv2.imread('noisy_image.jpg')
# 应用中值滤波
filtered_image = cv2.medianBlur(image, 3)
# 显示原始图像和滤波后图像
cv2.imshow('Original Image', image)
cv2.imshow('Filtered Image', filtered_image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
### 5.2 边缘保留案例:锐化图像中的细节
中值滤波也可以用于锐化图像中的细节。通过使用较小的内核尺寸,中值滤波可以去除图像中的噪声,同时保留边缘信息。
```python
import cv2
import numpy as np
# 读取图像
image = cv2.imread('blurred_image.jpg')
# 应用中值滤波
filtered_image = cv2.medianBlur(image, 1)
# 显示原始图像和滤波后图像
cv2.imshow('Original Image', image)
cv2.imshow('Filtered Image', filtered_image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
### 5.3 图像质量提升综合案例
中值滤波可以同时用于降噪和边缘保留,从而提升图像的整体质量。
```python
import cv2
import numpy as np
# 读取图像
image = cv2.imread('low_quality_image.jpg')
# 应用中值滤波
filtered_image = cv2.medianBlur(image, 3)
# 显示原始图像和滤波后图像
cv2.imshow('Original Image', image)
cv2.imshow('Filtered Image', filtered_image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
0
0
相关推荐
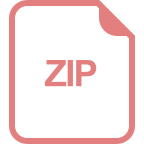
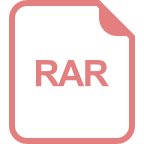
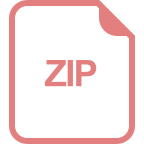





