揭秘OpenCV中值滤波:从原理到实战应用,提升图像处理技能
发布时间: 2024-08-12 04:10:18 阅读量: 37 订阅数: 21 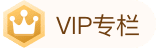
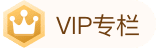
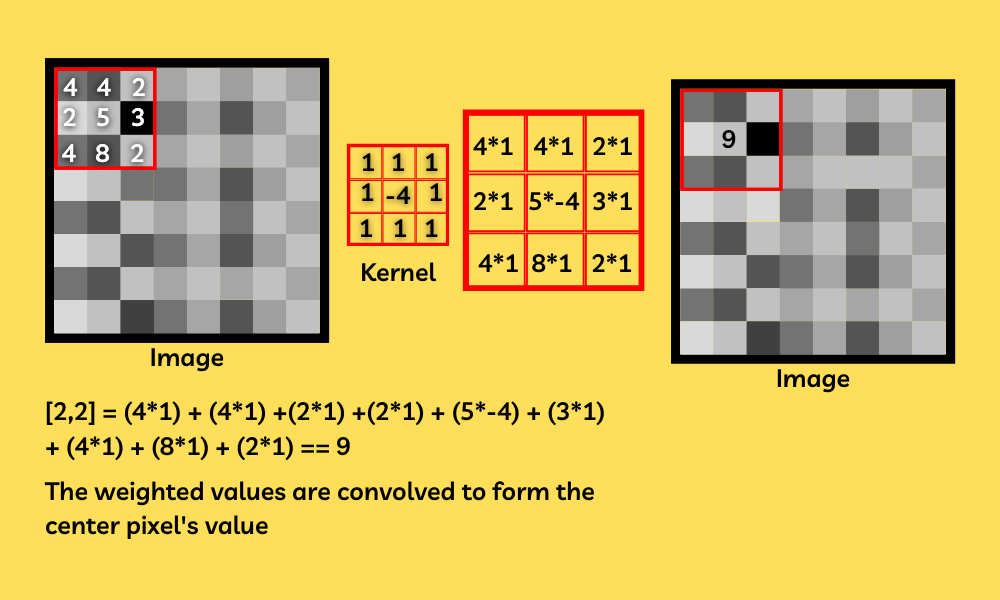
# 1. OpenCV中值滤波基础**
中值滤波是一种非线性图像处理技术,用于消除图像中的噪声。它通过将每个像素替换为其邻域中像素的中值来工作。与线性滤波器(如高斯滤波器)不同,中值滤波器不会模糊图像边缘,使其成为图像降噪的有效选择。
在OpenCV中,中值滤波函数为`cv2.medianBlur()`。它接受三个参数:输入图像、卷积核大小和输出图像。卷积核大小指定滤波器窗口的大小,通常为奇数(例如 3x3 或 5x5)。
# 2. 中值滤波理论与算法**
**2.1 中值滤波的原理**
中值滤波是一种非线性滤波技术,它通过将图像中的每个像素值替换为其邻域内像素值的中值来实现图像降噪。其基本原理如下:
1. **定义邻域:**对于图像中的每个像素,定义一个以该像素为中心的邻域,邻域的大小由窗口尺寸决定。
2. **排序像素值:**将邻域内的所有像素值按升序排列。
3. **选择中值:**取排列后的像素值序列的中值作为该像素的新值。
**2.2 中值滤波的算法实现**
中值滤波算法的实现主要涉及以下步骤:
1. **创建滑动窗口:**创建一个大小为窗口尺寸的滑动窗口。
2. **遍历图像:**遍历图像中的每个像素。
3. **获取邻域像素值:**对于每个像素,将滑动窗口移到该像素上,获取窗口内的所有像素值。
4. **排序像素值:**将窗口内的像素值按升序排列。
5. **选择中值:**取排列后的像素值序列的中值作为该像素的新值。
6. **更新图像:**将更新后的像素值写入图像。
**代码块:**
```python
import cv2
import numpy as np
def median_filter(image, kernel_size):
"""
中值滤波算法实现
Args:
image: 输入图像
kernel_size: 窗口尺寸
Returns:
滤波后的图像
"""
# 创建滑动窗口
window = np.zeros((kernel_size, kernel_size))
# 遍历图像
for i in range(image.shape[0]):
for j in range(image.shape[1]):
# 获取邻域像素值
window[:] = image[i:i+kernel_size, j:j+kernel_size]
# 排序像素值
window = np.sort(window.flatten())
# 选择中值
median_value = window[window.size // 2]
# 更新图像
image[i, j] = median_value
return image
```
**逻辑分析:**
该代码块实现了中值滤波算法。它首先创建一个滑动窗口,然后遍历图像中的每个像素。对于每个像素,它获取窗口内的所有像素值,并按升序排列它们。然后,它选择排列后的像素值序列的中值作为该像素的新值。最后,它将更新后的像素值写入图像。
**参数说明:**
* `image`: 输入图像,类型为 NumPy 数组。
* `kernel_size`: 窗口尺寸,类型为整数。
# 3. OpenCV中值滤波实践**
### 3.1 OpenCV中值滤波函数
OpenCV提供了`medianBlur`函数用于执行中值滤波。该函数的语法如下:
```cpp
void medianBlur(InputArray src, OutputArray dst, Size ksize)
```
其中:
- `src`:输入图像,可以是单通道或多通道图像。
- `dst`:输出图像,与输入图像具有相同的尺寸和类型。
- `ksize`:中值滤波核的大小,必须为奇数。
### 3.2 中值滤波在图像降噪中的应用
中值滤波在图像降噪中非常有效,因为它可以有效去除图像中的椒盐噪声和脉冲噪声。椒盐噪声是指图像中随机分布的白色和黑色像素,而脉冲噪声是指图像中随机分布的异常值像素。
以下代码演示了如何使用OpenCV进行图像降噪:
```cpp
#include <opencv2/opencv.hpp>
using namespace cv;
int main() {
// 读取图像
Mat image = imread("noisy_image.jpg");
// 创建中值滤波核
Size ksize(5, 5);
// 应用中值滤波
Mat denoised_image;
medianBlur(image, denoised_image, ksize);
// 显示降噪后的图像
imshow("Denoised Image", denoised_image);
waitKey(0);
return 0;
}
```
### 代码逻辑分析
该代码首先读取一张图像,然后创建一个大小为5x5的中值滤波核。接下来,它使用`medianBlur`函数将中值滤波应用于图像,并将其结果存储在`denoised_image`中。最后,它显示降噪后的图像。
### 参数说明
- `ksize`:中值滤波核的大小。它是一个`Size`对象,指定核的宽度和高度。
- `src`:输入图像。它是一个`InputArray`对象,可以是`Mat`、`UMat`或其他支持的类型。
- `dst`:输出图像。它是一个`OutputArray`对象,用于存储中值滤波的结果。
# 4.1 中值滤波在图像边缘检测中的应用
中值滤波在图像边缘检测中具有独特的优势,因为它能够有效地去除图像中的噪声,同时保留边缘信息。这对于提高边缘检测算法的准确性和鲁棒性至关重要。
**原理**
中值滤波在边缘检测中的原理是基于这样一个事实:边缘通常是图像中像素值变化最剧烈的地方。因此,通过对图像进行中值滤波,可以有效地平滑图像中非边缘区域的像素值,同时保留边缘处的像素值。
**算法实现**
在OpenCV中,可以使用`cv2.medianBlur()`函数对图像进行中值滤波。该函数接受两个参数:
* `image`:需要进行中值滤波的图像
* `ksize`:中值滤波的内核大小,它是一个奇数,通常取3或5
以下代码示例展示了如何使用`cv2.medianBlur()`函数对图像进行中值滤波:
```python
import cv2
# 读取图像
image = cv2.imread('image.jpg')
# 对图像进行中值滤波
median_filtered_image = cv2.medianBlur(image, 3)
# 显示原始图像和中值滤波后的图像
cv2.imshow('Original Image', image)
cv2.imshow('Median Filtered Image', median_filtered_image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
**代码逻辑分析**
* `cv2.imread('image.jpg')`:读取图像并将其存储在`image`变量中。
* `cv2.medianBlur(image, 3)`:对图像进行中值滤波,内核大小为3。
* `cv2.imshow('Original Image', image)`:显示原始图像。
* `cv2.imshow('Median Filtered Image', median_filtered_image)`:显示中值滤波后的图像。
* `cv2.waitKey(0)`:等待用户按下任意键。
* `cv2.destroyAllWindows()`:销毁所有窗口。
**参数说明**
* `ksize`:中值滤波的内核大小,它是一个奇数,通常取3或5。较大的内核尺寸可以去除更多的噪声,但也会导致边缘模糊。
* `image`:需要进行中值滤波的图像。
* `median_filtered_image`:中值滤波后的图像。
## 4.2 中值滤波在图像分割中的应用
中值滤波在图像分割中也发挥着重要作用,因为它可以有效地去除图像中的噪声,同时保留对象边界。这对于提高图像分割算法的准确性和鲁棒性至关重要。
**原理**
中值滤波在图像分割中的原理是基于这样一个事实:对象边界通常是图像中像素值变化最剧烈的地方。因此,通过对图像进行中值滤波,可以有效地平滑图像中非边界区域的像素值,同时保留边界处的像素值。
**算法实现**
在OpenCV中,可以使用`cv2.medianBlur()`函数对图像进行中值滤波。该函数接受两个参数:
* `image`:需要进行中值滤波的图像
* `ksize`:中值滤波的内核大小,它是一个奇数,通常取3或5
以下代码示例展示了如何使用`cv2.medianBlur()`函数对图像进行中值滤波:
```python
import cv2
# 读取图像
image = cv2.imread('image.jpg')
# 对图像进行中值滤波
median_filtered_image = cv2.medianBlur(image, 3)
# 对图像进行阈值分割
thresholded_image = cv2.threshold(median_filtered_image, 127, 255, cv2.THRESH_BINARY)[1]
# 显示原始图像、中值滤波后的图像和阈值分割后的图像
cv2.imshow('Original Image', image)
cv2.imshow('Median Filtered Image', median_filtered_image)
cv2.imshow('Thresholded Image', thresholded_image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
**代码逻辑分析**
* `cv2.imread('image.jpg')`:读取图像并将其存储在`image`变量中。
* `cv2.medianBlur(image, 3)`:对图像进行中值滤波,内核大小为3。
* `cv2.threshold(median_filtered_image, 127, 255, cv2.THRESH_BINARY)[1]`:对中值滤波后的图像进行阈值分割,阈值设为127。
* `cv2.imshow('Original Image', image)`:显示原始图像。
* `cv2.imshow('Median Filtered Image', median_filtered_image)`:显示中值滤波后的图像。
* `cv2.imshow('Thresholded Image', thresholded_image)`:显示阈值分割后的图像。
* `cv2.waitKey(0)`:等待用户按下任意键。
* `cv2.destroyAllWindows()`:销毁所有窗口。
**参数说明**
* `ksize`:中值滤波的内核大小,它是一个奇数,通常取3或5。较大的内核尺寸可以去除更多的噪声,但也会导致边界模糊。
* `image`:需要进行中值滤波的图像。
* `median_filtered_image`:中值滤波后的图像。
* `thresholded_image`:阈值分割后的图像。
# 5. 中值滤波与其他图像处理技术的比较**
**5.1 中值滤波与高斯滤波的比较**
中值滤波和高斯滤波都是图像处理中常用的降噪滤波器,但它们在原理和效果上存在差异。
**原理:**
* **中值滤波:**通过计算邻域内像素的中值来替换中心像素,从而去除噪声。
* **高斯滤波:**使用高斯核对图像进行加权平均,从而平滑图像并去除噪声。
**效果:**
* **中值滤波:**
* 更有效地去除椒盐噪声(孤立的白色或黑色像素)和脉冲噪声(随机分布的噪声像素)。
* 保留图像边缘和细节,不会产生模糊效果。
* **高斯滤波:**
* 更有效地去除高斯噪声(图像中随机分布的噪声)。
* 会产生一定程度的模糊效果,可能导致图像细节丢失。
**表格:中值滤波与高斯滤波的比较**
| 特征 | 中值滤波 | 高斯滤波 |
|---|---|---|
| 原理 | 计算邻域内像素的中值 | 使用高斯核加权平均 |
| 降噪效果 | 更有效去除椒盐噪声和脉冲噪声 | 更有效去除高斯噪声 |
| 边缘保留 | 保留图像边缘和细节 | 产生模糊效果,可能导致细节丢失 |
**5.2 中值滤波与双边滤波的比较**
中值滤波和双边滤波都是非线性滤波器,但它们在空间域和像素值域上的处理方式不同。
**原理:**
* **中值滤波:**仅考虑空间域,计算邻域内像素的中值。
* **双边滤波:**同时考虑空间域和像素值域,计算邻域内像素的加权平均,权重由空间距离和像素值差异决定。
**效果:**
* **中值滤波:**
* 更有效地去除椒盐噪声和脉冲噪声。
* 保留图像边缘和细节,不会产生模糊效果。
* **双边滤波:**
* 既能去除噪声,又能保留图像边缘和细节。
* 适用于处理纹理复杂或具有噪声的图像。
**表格:中值滤波与双边滤波的比较**
| 特征 | 中值滤波 | 双边滤波 |
|---|---|---|
| 原理 | 仅考虑空间域 | 同时考虑空间域和像素值域 |
| 降噪效果 | 更有效去除椒盐噪声和脉冲噪声 | 既能去除噪声,又能保留细节 |
| 边缘保留 | 保留图像边缘和细节 | 保留图像边缘和细节 |
**代码示例:**
```python
import cv2
# 读取图像
image = cv2.imread('noisy_image.jpg')
# 中值滤波
median_filtered_image = cv2.medianBlur(image, 5)
# 双边滤波
bilateral_filtered_image = cv2.bilateralFilter(image, 9, 75, 75)
# 显示结果
cv2.imshow('Original Image', image)
cv2.imshow('Median Filtered Image', median_filtered_image)
cv2.imshow('Bilateral Filtered Image', bilateral_filtered_image)
cv2.waitKey(0)
```
**逻辑分析:**
* `cv2.medianBlur()`函数使用中值滤波对图像进行降噪,其中5表示滤波器内核的大小。
* `cv2.bilateralFilter()`函数使用双边滤波对图像进行降噪,其中9表示滤波器内核的大小,75表示空间距离权重,75表示像素值差异权重。
# 6.1 图像降噪实战
在实际应用中,图像降噪是中值滤波最常见的应用之一。中值滤波可以有效去除图像中的椒盐噪声和高斯噪声。
**操作步骤:**
1. 导入OpenCV库:
```python
import cv2
```
2. 读取原始图像:
```python
image = cv2.imread('noisy_image.jpg')
```
3. 应用中值滤波:
```python
filtered_image = cv2.medianBlur(image, 3)
```
其中,`3`表示滤波器内核的大小。
4. 显示处理后的图像:
```python
cv2.imshow('Filtered Image', filtered_image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
**代码解释:**
* `cv2.imread()`函数读取原始图像并将其存储在`image`变量中。
* `cv2.medianBlur()`函数应用中值滤波。第一个参数是输入图像,第二个参数是滤波器内核的大小。
* `cv2.imshow()`函数显示处理后的图像。
* `cv2.waitKey(0)`函数等待用户按下任意键。
* `cv2.destroyAllWindows()`函数关闭所有打开的窗口。
**参数说明:**
* `image`: 输入图像。
* `ksize`: 滤波器内核的大小,必须为奇数。
* `filtered_image`: 经过中值滤波处理后的图像。
0
0
相关推荐
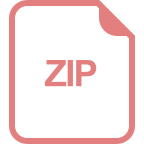
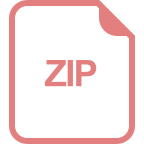
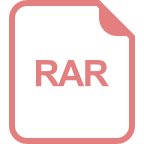





