Python Decorator测试方法:掌握测试装饰器函数的3个步骤
发布时间: 2024-10-17 12:43:49 阅读量: 20 订阅数: 25 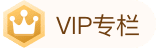
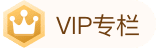
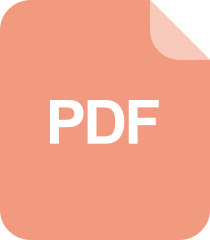
12步入门Python中的decorator装饰器使用方法
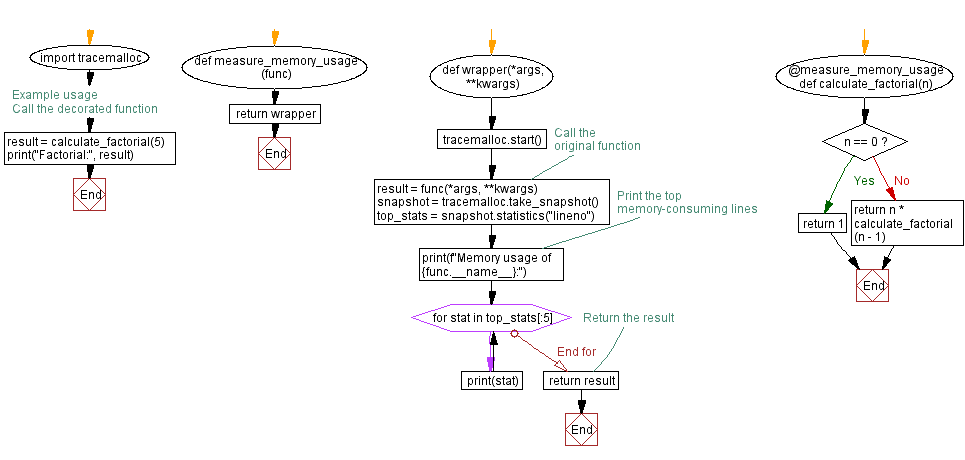
# 1. Python Decorator简介
在Python编程中,Decorator提供了一种优雅的方式来修改或增强函数或类的行为,而无需更改其内部实现。这一概念对于提升代码的复用性、可读性和维护性具有重要意义。本章将对Decorator进行基础介绍,帮助读者理解其定义、工作原理以及在实际开发中的应用。
## Decorator的定义和使用
### Decorator的定义
Decorator是一种设计模式,本质上是一个函数,它接受另一个函数作为参数并返回一个新的函数。这个新函数通常会在原函数的基础上增加额外的功能。
### Decorator的使用方法
Decorator的使用非常简单,只需要在定义函数时,在函数名前加上`@decorator_name`这样的语法糖。例如:
```python
def my_decorator(func):
def wrapper():
print("Something is happening before the function is called.")
func()
print("Something is happening after the function is called.")
return wrapper
@my_decorator
def say_hello():
print("Hello!")
say_hello()
```
在这个例子中,`my_decorator`是一个装饰器,它在`say_hello`函数执行前后分别打印了一些信息。通过使用`@my_decorator`,我们无需修改`say_hello`函数的内部代码,就可以为其增加额外的功能。
# 2. Python Decorator的基础知识
## 2.1 Decorator的定义和使用
### 2.1.1 Decorator的定义
在Python中,Decorator是一种设计模式,用于修改或增强函数、方法或类的行为。它是一种函数,它接受另一个函数作为参数,并返回一个新的函数,这个新函数通常会在原始函数调用前后执行一些额外的操作。
Decorator的核心思想是将函数的定义动态地修改,而不改变函数本身。这样做的好处是,可以在不侵入原有代码逻辑的基础上,增加额外的功能,比如日志记录、性能监控、权限检查等。
### 2.1.2 Decorator的使用方法
使用Decorator非常简单,主要有两种方式:
1. 使用`@decorator`语法糖,这是最常见的方式。例如:
```python
def my_decorator(f):
def wrapper():
print("Something is happening before the function is called.")
f()
print("Something is happening after the function is called.")
return wrapper
@my_decorator
def say_hello():
print("Hello!")
say_hello()
```
在这个例子中,`my_decorator`是一个Decorator,它在`say_hello`函数执行前后打印一些信息。
2. 直接赋值方式,这种方式在某些复杂的情况下更为灵活。例如:
```python
def my_decorator(f):
def wrapper():
print("Something is happening before the function is called.")
f()
print("Something is happening after the function is called.")
return wrapper
def say_hello():
print("Hello!")
say_hello = my_decorator(say_hello)
say_hello()
```
在这个例子中,我们将`say_hello`函数通过`my_decorator`进行装饰,然后重新赋值给`say_hello`。
## 2.2 Decorator的工作原理
### 2.2.1 Decorator的执行过程
当使用`@decorator`语法糖时,Python在函数定义时就会调用Decorator函数,并将函数对象作为参数传递给它。Decorator返回的函数对象将替换原始函数。
例如,以下代码:
```python
@my_decorator
def say_hello():
print("Hello!")
```
等价于:
```python
def say_hello():
print("Hello!")
say_hello = my_decorator(say_hello)
```
在这个过程中,`say_hello`函数对象首先被传递给`my_decorator`函数,然后`my_decorator`返回一个新的函数对象,最后这个新的函数对象被赋值给`say_hello`。
### 2.2.2 Decorator的函数包装
Decorator的核心是函数包装,即将原始函数封装在一个新的函数中,这个新的函数通常会在原始函数调用前后执行一些额外的操作。
例如,以下代码:
```python
def my_decorator(f):
def wrapper():
print("Something is happening before the function is called.")
f()
print("Something is happening after the function is called.")
return wrapper
```
在这个例子中,`my_decorator`返回的`wrapper`函数就是对原始函数`f`的一个包装。当`wrapper`被调用时,它首先打印一条消息,然后调用原始函数`f`,最后再打印一条消息。
## 2.3 Decorator的语法特性
### 2.3.1 @wraps的使用
`@wraps`是`functools`模块中的一个装饰器,它可以帮助我们在创建Decorator时保留原始函数的元数据,比如函数名、文档字符串等。
例如,以下代码:
```python
from functools import wraps
def my_decorator(f):
@wraps(f)
def wrapper(*args, **kwargs):
print("Something is happening before the function is called.")
result = f(*args, **kwargs)
print("Something is happening after the function is called.")
return wrapper
```
在这个例子中,`@wraps(f)`装饰了`wrapper`函数,这样`wrapper`函数就保留了`f`函数的元数据。
### 2.3.2 参数化Decorator
有时,我们希望Decorator能够接收参数,这样就可以创建更加灵活的Decorator。我们可以通过定义一个装饰器工厂来实现这一点。
例如,以下代码:
```python
def repeat(num_times):
def decorator_repeat(f):
@wraps(f)
def wrapper(*args, **kwargs):
for _ in range(num_times):
result = f(*args, **kwargs)
return result
return wrapper
return decorator_repeat
@repeat(num_times=3)
def greet(name):
print(f"Hello {name}")
greet("World")
```
在这个例子中,`repeat`是一个装饰器工厂,它返回一个装饰器`decorator_repeat`,`decorator_repeat`接收一个函数`f`作为参数,并返回一个新的函数`wrapper`。当我们使用`@repeat(num_times=3)`时,实际上就是调用了`decorator_repeat(greet)`,这样就创建了一个重复执行`greet`函数三次的Decorator。
# 3. Python Decorator的测试方法
在本章节中,我们将深入探讨如何对Python Decorator进行测试。无论是简单还是复杂的Decorator,都应当通过一系列的测试案例来确保其正确性和可靠性。本章节将介绍单元测试的基本概念、测试工具和框架的使用,以及通过具体案例分析来展示如何对Decorator进行测试。
## 3.1 Decorator的单元测试
### 3.1.* 单元测试的基本概念
单元测试是软件开发中一个重要的环节,它指的是对代码中的最小可测试部分进行检查和验证。在Python中,单元测试通常是指对一个函数或方法进行测试,确保它在不同情况下都能正确执行。对于Decorator来说,单元测试尤为重要,因为Decorator本质上是一个接收函数作为参数并返回一个新函数的函数,它的行为可能会在不同的使用场景中有所不同。
### 3.1.2 测试Decorator的策略
为了测试Decorator,我们需要关注以下几个方面:
- **功能验证**:确保Decorator正确地修改了被装饰函数的行为。
- **性能评估**:如果Decorator设计用于性能优化,需要评估其对执行时间的影响。
- **异常处理**:
0
0
相关推荐
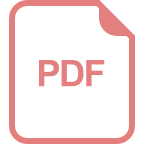
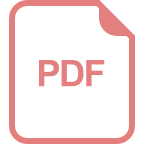
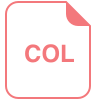
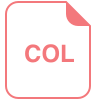
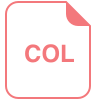
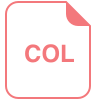
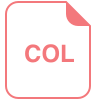
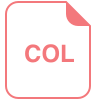