【Python Decorator入门指南】:一步到位掌握函数装饰器的核心
发布时间: 2024-10-17 11:51:04 阅读量: 17 订阅数: 18 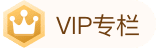
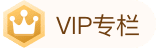
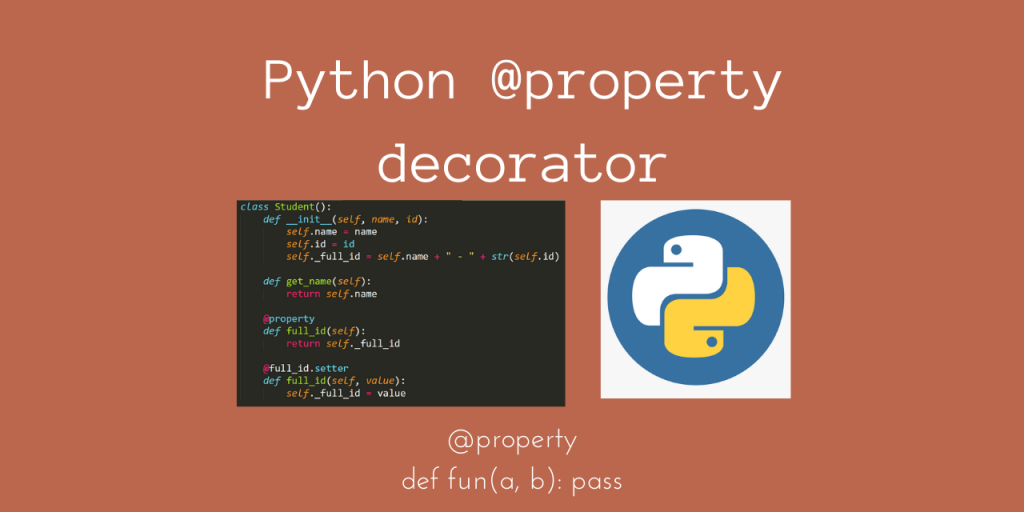
# 1. Python Decorator的简介和基本用法
## 简介
Python Decorator是一种设计模式,允许用户在不修改原有函数定义的情况下增加新的功能。它本质上是一个函数,这个函数可以接收另一个函数作为参数,并且可以返回一个新的函数。
## 基本用法
使用Decorator的基本步骤如下:
1. 定义一个装饰器函数,它接收一个函数作为参数。
2. 在装饰器内部定义一个内部函数,它通常会调用被装饰的函数。
3. 返回内部函数,或者在内部函数中修改并返回结果。
### 示例代码
```python
def my_decorator(func):
def wrapper():
print("Something is happening before the function is called.")
func()
print("Something is happening after the function is called.")
return wrapper
@my_decorator
def say_hello():
print("Hello!")
say_hello()
```
在这个例子中,`my_decorator`是一个装饰器,它在`say_hello`函数调用前后打印了一些信息。使用`@my_decorator`语法糖可以将装饰器应用于函数。
### 执行逻辑
- 当调用`say_hello()`时,实际上调用的是`my_decorator`返回的`wrapper`函数。
- `wrapper`函数首先打印"Something is happening before the function is called."。
- 然后调用`say_hello`函数,打印"Hello!"。
- 最后打印"Something is happening after the function is called."。
### 参数说明
- `func`:被装饰的函数。
- `wrapper`:装饰器内部定义的函数,用于包裹原函数并添加额外功能。
通过这种方式,Decorator提供了一种优雅的方法来增强函数功能,而无需改变原有函数的代码。
# 2. 深入理解Python Decorator的原理
### 2.1 装饰器的底层实现原理
#### 2.1.1 装饰器的函数转换过程
在Python中,装饰器本质上是一个接收函数作为参数并返回一个新函数的高阶函数。这个过程可以通过一个简单的例子来说明。考虑一个基本的装饰器:
```python
def my_decorator(func):
def wrapper():
print("Something is happening before the function is called.")
func()
print("Something is happening after the function is called.")
return wrapper
def say_hello():
print("Hello!")
# 使用装饰器
say_hello = my_decorator(say_hello)
# 调用装饰后的函数
say_hello()
```
在这个例子中,`my_decorator` 接受一个函数 `func` 作为参数,并返回一个新函数 `wrapper`。当 `say_hello` 被装饰后,原来的 `say_hello` 函数被 `wrapper` 函数替代。因此,当我们调用 `say_hello()` 时,实际上是在调用 `wrapper()`。
#### 2.1.2 装饰器和闭包的关系
装饰器和闭包之间有着密切的联系。闭包是一个可以捕获其所在作用域中变量的函数。在上述例子中,`wrapper` 函数就是一个闭包,它捕获了外部的 `func` 变量。当 `wrapper` 被调用时,它可以访问并执行 `func`,即使 `func` 在 `wrapper` 的外部作用域中定义。
闭包的关键特性在于它可以记住其定义时的环境,这使得装饰器能够执行额外的代码,无论是日志记录、性能测试还是其他的横切关注点(cross-cutting concerns)。
### 2.2 装饰器的应用场景
#### 2.2.1 日志记录
装饰器在日志记录方面非常有用,它可以自动记录函数的调用信息而不需要在每个函数内部手动添加日志代码。下面是一个简单的日志记录装饰器的例子:
```python
import functools
def log_decorator(func):
@functools.wraps(func)
def wrapper(*args, **kwargs):
print(f"Calling function {func.__name__}")
result = func(*args, **kwargs)
print(f"Function {func.__name__} returned {result}")
return result
return wrapper
@log_decorator
def add(x, y):
return x + y
add(2, 3)
```
在这个例子中,`log_decorator` 装饰器在被装饰的函数 `add` 调用前后打印出日志信息。
#### 2.2.2 性能测试
装饰器也可以用于性能测试,自动测量函数的执行时间。这是一个性能测试装饰器的例子:
```python
import time
def performance_test_decorator(func):
@functools.wraps(func)
def wrapper(*args, **kwargs):
start_time = time.time()
result = func(*args, **kwargs)
end_time = time.time()
print(f"Function {func.__name__} took {end_time - start_time}s to complete.")
return result
return wrapper
@performance_test_decorator
def slow_function():
time.sleep(2)
return "Done"
slow_function()
```
在这个例子中,`performance_test_decorator` 装饰器在被装饰的函数 `slow_function` 调用前后计算时间差,并打印出来。
### 2.3 装饰器的进阶技巧
#### 2.3.1 带参数的装饰器
有时候,我们可能希望装饰器本身也能够接受参数,这可以通过嵌套定义一个装饰器工厂函数来实现。以下是一个带参数的装饰器的例子:
```python
def repeat(num_times):
def decorator_repeat(func):
@functools.wraps(func)
def wrapper(*args, **kwargs):
for _ in range(num_times):
result = func(*args, **kwargs)
return result
return wrapper
return decorator_repeat
@repeat(num_times=3)
def greet(name):
print(f"Hello {name}")
greet("Alice")
```
在这个例子中,`repeat` 是一个装饰器工厂函数,它接受一个参数 `num_times` 并返回一个装饰器 `decorator_repeat`。`decorator_repeat` 接受一个函数 `func` 并返回一个 `wrapper` 函数,该函数会重复调用 `func` 指定的次数。
#### 2.3.2 装饰器的嵌套使用
装饰器可以被嵌套使用,即一个装饰器被另一个装饰器装饰。这可以用来组合多个装饰器的行为。以下是一个装饰器嵌套使用的例子:
```python
@performance_test_decorator
@log_decorator
def square(x):
return x * x
square(4)
```
在这个例子中,`square` 函数首先被 `log_decorator` 装饰,然后被 `performance_test_decorator` 装饰。这意味着在调用 `square` 时,它会首先记录日志,然后测量性能,最后返回计算结果。
通过本章节的介绍,我们已经深入理解了Python装饰器的原理,包括它的底层实现原理、应用场景以及进阶技巧。这为我们在实际开发中有效地使用装饰器提供了坚实的基础。接下来,我们将探讨装饰器在实践应用中的常见用法,以及它们如何在流行框架如Flask和Django中发挥作用。
# 3. Python Decorator的实践应用
在本章节中,我们将深入探讨Python Decorator在实际编程中的应用,以及如何在流行的Web框架Flask和Django中使用Decorator来增强代码的复用性和可维护性。通过本章节的介绍,你将学会如何构建缓存机制和权限控制逻辑,以及如何在框架中利用Decorator提高开发效率。
## 3.1 常见的Decorator实践
Decorator不仅仅是理论上的概念,它在实际编程中有着广泛的应用。我们将首先讨论如何使用Decorator实现缓存机制和权限控制。
### 3.1.1 缓存机制
在很多情况下,我们希望函数的输出结果能够被缓存起来,以便在下一次调用时直接返回,而不是重新计算。这种做法可以显著提高程序的性能,特别是在处理耗时的计算或者I/O操作时。
```python
import functools
from time import sleep
# 缓存装饰器
def cache_decorator(func):
@functools.wraps(func)
def wrapper(*args, **kwargs):
cache_key = (args, tuple(kwargs.items()))
if cache_key in wrapper.cache:
return wrapper.cache[cache_key]
result = func(*args, **kwargs)
wrapper.cache[cache_key] = result
return result
wrapper.cache = {}
return wrapper
# 示例函数
@cache_decorator
def slow_computation(x):
sleep(2)
return x * x
# 测试缓存效果
print(slow_computation(1)) # 第一次调用,耗时2秒
print(slow_computation(1)) # 第二次调用,几乎立即返回结果
```
在这个例子中,我们定义了一个`cache_decorator`,它会将函数的输入作为键,将结果存储在一个字典中。如果第二次调用同一个函数时输入相同,装饰器会直接返回缓存的结果,而不是再次执行函数体。
### 3.1.2 权限控制
在Web应用中,权限控制是一个常见的需求。我们可以通过Decorator来检查用户是否具有执行某函数的权限。
```python
def check_permission(user_role):
def decorator(func):
@functools.wraps(func)
def wrapper(*args, **kwargs):
if user_role not in kwargs['user'].roles:
raise PermissionError("You don't have permission to access this function.")
return func(*args, **kwargs)
return wrapper
return decorator
# 示例函数
@check_permission("admin")
def delete_user(user):
print(f"User {user.name} has been deleted.")
# 测试权限控制
user = User(name="Alice", roles=["user"])
delete_user(user) # 抛出PermissionError
```
在这个例子中,`check_permission`装饰器接受一个`user_role`参数,并在内部函数`wrapper`中检查用户的角色是否符合要求。如果不符合,抛出`PermissionError`异常。
## 3.2 Decorator在框架中的应用
Decorator在Web框架中的应用非常广泛,特别是在Flask和Django这样的框架中。我们将分别介绍如何在这两个框架中使用Decorator。
### 3.2.1 Flask中的Decorator应用
在Flask框架中,我们可以使用Decorator来定义路由和处理请求。
```python
from flask import Flask, jsonify
app = Flask(__name__)
# 示例装饰器
def json_response(func):
@functools.wraps(func)
def wrapper(*args, **kwargs):
result = func(*args, **kwargs)
return jsonify(result), 200
return wrapper
# 使用Decorator定义路由
@app.route('/api/user/<int:user_id>')
@json_response
def get_user(user_id):
user = User.query.get(user_id)
return {"id": user.id, "name": user.name}
if __name__ == '__main__':
app.run(debug=True)
```
在这个例子中,我们定义了一个`json_response`装饰器,它将函数的返回值转换为JSON格式的响应。然后,我们使用`@app.route`装饰器来定义一个路由,并将其与`get_user`函数关联起来。
### 3.2.2 Django中的Decorator应用
在Django框架中,Decorator常用于视图函数的权限控制和性能优化。
```python
from django.http import JsonResponse
from django.views.decorators.http import require_http_methods
from django.contrib.auth.decorators import login_required
@require_http_methods(["GET", "POST"])
@login_required
def my_view(request):
if request.method == "GET":
return JsonResponse({"message": "This is a GET request"})
else:
return JsonResponse({"message": "This is a POST request"})
# URL配置
urlpatterns = [
path('my_view/', my_view, name='my_view'),
]
```
在这个例子中,我们使用了`require_http_methods`来限制视图函数只接受GET和POST请求,使用`login_required`来要求用户登录后才能访问。这样的组合确保了视图的安全性和访问控制。
### 总结
在本章节中,我们介绍了如何在实际编程中应用Decorator来实现缓存机制和权限控制,以及如何在Flask和Django这样的Web框架中使用Decorator。通过这些例子,你可以看到Decorator的强大功能和灵活性,以及如何将它们应用于不同的场景中。希望这些内容能够帮助你更好地理解和使用Python Decorator,提高你的代码质量和开发效率。
# 4. Python Decorator的高级应用
### 4.1 使用Decorator进行异常处理
在Python中,异常处理是确保程序健壮性的关键部分。Decorator可以用来简化异常处理逻辑,使得代码更加清晰和可重用。本章节将介绍如何使用Decorator进行异常捕获和异常处理的最佳实践。
#### 4.1.1 异常捕获
异常捕获是异常处理的基础,它可以帮助我们捕获运行时错误,并进行相应的处理。使用Decorator进行异常捕获,可以将异常处理逻辑从业务逻辑代码中分离出来,使得代码更加清晰。
```python
def catch_exceptions(func):
def wrapper(*args, **kwargs):
try:
return func(*args, **kwargs)
except Exception as e:
print(f"Exception occurred: {e}")
# 这里可以进行一些异常记录操作
return wrapper
@catch_exceptions
def risky_function():
# 这里是一些可能会抛出异常的代码
raise ValueError("Something went wrong!")
risky_function()
```
在上述代码中,`catch_exceptions`是一个Decorator,它包装了任何函数,并在调用时捕获所有异常。这种方式非常适合用于那些可能会抛出异常但不希望中断程序执行的函数。
#### 4.1.2 异常处理的最佳实践
在实际应用中,我们可能需要根据不同的异常类型进行不同的处理。为了实现这一点,我们可以进一步扩展`catch_exceptions` Decorator。
```python
def catch_exceptions(handler=None):
def decorator(func):
def wrapper(*args, **kwargs):
try:
return func(*args, **kwargs)
except Exception as e:
if handler:
handler(e)
else:
print(f"Exception occurred: {e}")
return wrapper
return decorator
@catch_exceptions()
def risky_function():
# 这里是一些可能会抛出异常的代码
raise ValueError("Something went wrong!")
@catch_exceptions(lambda e: print(f"Error: {e}"))
def risky_function_with_custom_handler():
# 这里是一些可能会抛出异常的代码
raise Exception("Something went terribly wrong!")
```
在这个例子中,`catch_exceptions`可以接受一个可选的`handler`参数,该参数是一个函数,用于处理捕获到的异常。这种方式提供了更高的灵活性,允许调用者定义自己的异常处理逻辑。
### 4.2 Decorator的性能优化
尽管Decorator为Python代码带来了便利和优雅,但它们也可能引入性能损耗。本章节将讨论装饰器的性能损耗原因,并提供一些优化策略。
#### 4.2.1 装饰器的性能损耗
Decorator通过包装原始函数来提供额外的功能,这个包装过程可能会引入额外的性能开销。例如,每次调用被装饰的函数时,装饰器的逻辑都会被执行。
```python
import time
def timer_decorator(func):
def wrapper(*args, **kwargs):
start_time = time.time()
result = func(*args, **kwargs)
end_time = time.time()
print(f"Function {func.__name__} took {end_time - start_time} seconds to run.")
return result
return wrapper
@timer_decorator
def slow_function():
# 模拟一个耗时操作
time.sleep(1)
```
在这个例子中,`timer_decorator`会在每次调用`slow_function`时输出其运行时间。虽然这很有用,但每次调用都会增加时间测量的开销。
#### 4.2.2 优化策略
为了减少性能损耗,我们可以采用一些优化策略。一种常见的策略是使用缓存来存储函数的结果,避免对已经计算过的结果进行重复计算。
```python
from functools import wraps
def memoize(func):
cache = {}
@wraps(func)
def wrapper(*args, **kwargs):
if args in cache:
return cache[args]
result = func(*args, **kwargs)
cache[args] = result
return result
return wrapper
@memoize
def expensive_function(x):
# 模拟一个昂贵的计算
return x * x
```
在这个例子中,`memoize` Decorator使用了一个字典来缓存函数的结果。当一个已缓存的参数再次被传入时,它将直接返回缓存的结果,从而避免了重复计算。
### 4.3 Decorator的调试和测试
Decorator的调试和测试是确保它们正确工作的关键步骤。本章节将介绍一些装饰器的调试技巧和测试方法。
#### 4.3.1 装饰器的调试技巧
调试装饰器可能比较复杂,因为它们通常涉及多层函数调用。为了简化调试过程,我们可以使用Python标准库中的`functools.wraps`装饰器来保留原始函数的元数据。
```python
from functools import wraps
def debug_decorator(func):
@wraps(func)
def wrapper(*args, **kwargs):
print(f"Function {func.__name__} is called with args: {args}, kwargs: {kwargs}")
result = func(*args, **kwargs)
print(f"Function {func.__name__} returned: {result}")
return result
return wrapper
@debug_decorator
def simple_function(a, b):
return a + b
```
在这个例子中,`debug_decorator`使用了`functools.wraps`来确保`simple_function`的调试信息能够正确显示。
#### 4.3.2 装饰器的测试方法
装饰器的测试需要确保它们不仅能够正确执行,而且不会引入不希望的副作用。为了测试装饰器,我们可以使用单元测试框架来模拟函数调用,并验证结果。
```python
import unittest
class TestDecorator(unittest.TestCase):
def test_timer_decorator(self):
@timer_decorator
def test_function():
pass
test_function()
self.assertIn("Function test_function took", output)
if __name__ == "__main__":
unittest.main()
```
在这个例子中,我们使用了Python的`unittest`模块来测试`timer_decorator`装饰器。测试用例确保装饰器能够正确记录函数的执行时间。
### 总结
本章节介绍了使用Decorator进行异常处理、性能优化以及调试和测试的相关技巧。通过这些高级应用,我们可以更好地利用Decorator的优势,同时避免可能引入的问题。在实际开发中,合理地使用和测试Decorator,能够显著提升代码的质量和可维护性。
# 5. Python Decorator的未来展望
## 5.1 装饰器在Python 3中的新特性
在Python 3中,装饰器的应用得到了进一步的增强和完善。Python 3.8版本引入了位置参数装饰器的特性,允许开发者使用`@functools.wraps`来保留函数的元数据。此外,Python 3.9对装饰器的语法进行了优化,使得装饰器的定义更加直观和灵活。
### 5.1.1 Python 3的装饰器新特性
Python 3的装饰器新特性中,最为显著的是`@functools.cached_property`装饰器的引入。这个装饰器提供了一种将属性自动缓存的方法,类似于`@property`装饰器,但它会自动缓存属性的值,使得属性值只在第一次访问时被计算,之后再次访问时直接返回缓存的值。
```python
from functools import cached_property
class Fibonacci:
def __init__(self, n):
self.n = n
@cached_property
def fib(self):
if self.n == 0:
return 0
elif self.n == 1:
return 1
else:
return self.fib(self.n - 1) + self.fib(self.n - 2)
# 使用缓存属性
fib_instance = Fibonacci(50)
print(fib_instance.fib) # 计算速度快,缓存效果显著
```
### 5.1.2 对未来Python版本的展望
随着Python语言的发展,装饰器的语法和功能预计将会得到进一步的改进。例如,Python 4可能会引入更高级的装饰器功能,如装饰器的链式调用、装饰器的参数化和类型提示等。这些改进将使得装饰器在代码组织和抽象方面发挥更大的作用。
## 5.2 装饰器在其他领域的应用
装饰器不仅在Python编程中有着广泛的应用,它们也在其他领域中找到了应用场景,尤其是在Web开发和数据分析这两个领域中。
### 5.2.1 装饰器在Web开发中的应用
在Web开发中,装饰器可以用于权限控制、日志记录、性能监控等多种场景。例如,在Flask框架中,可以使用装饰器来创建路由,而在Django框架中,装饰器可以用于中间件的处理。
```python
# Flask中的装饰器应用
from flask import Flask, jsonify
app = Flask(__name__)
@app.route('/user/<username>')
def get_user(username):
return jsonify({'username': username})
# Django中的装饰器应用
from django.http import HttpResponse
from django.contrib.auth.decorators import login_required
@login_required
def my_view(request):
return HttpResponse("This is a protected view")
```
### 5.2.2 装饰器在数据分析中的应用
在数据分析中,装饰器可以用于性能优化、缓存结果、异常处理等。例如,可以使用装饰器来缓存函数的返回结果,避免重复计算,从而提高数据分析的效率。
```python
import time
from functools import wraps
def timer_decorator(func):
@wraps(func)
def wrapper(*args, **kwargs):
start_time = time.time()
result = func(*args, **kwargs)
end_time = time.time()
print(f"Function {func.__name__} took {end_time - start_time} seconds to run.")
return result
return wrapper
@timer_decorator
def compute数据分析(n):
# 模拟耗时的数据分析操作
time.sleep(2)
return "分析结果"
result = compute数据分析(10)
```
通过上述示例,我们可以看到装饰器在不同领域的应用,它们帮助我们更好地组织代码,提高了代码的可读性和可维护性。随着Python语言的不断发展,装饰器的应用将会更加广泛和深入。
0
0
相关推荐
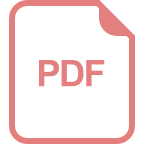
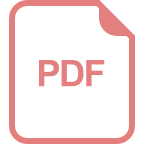
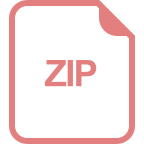
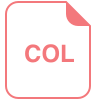
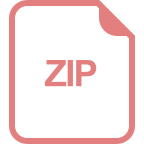
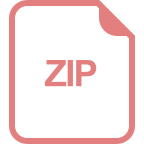
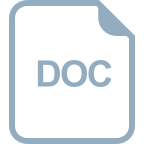
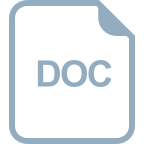
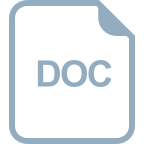