【数据结构新手课】:利用Python列表实现栈和队列基础
发布时间: 2024-09-12 02:50:06 阅读量: 21 订阅数: 26 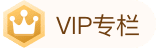
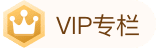
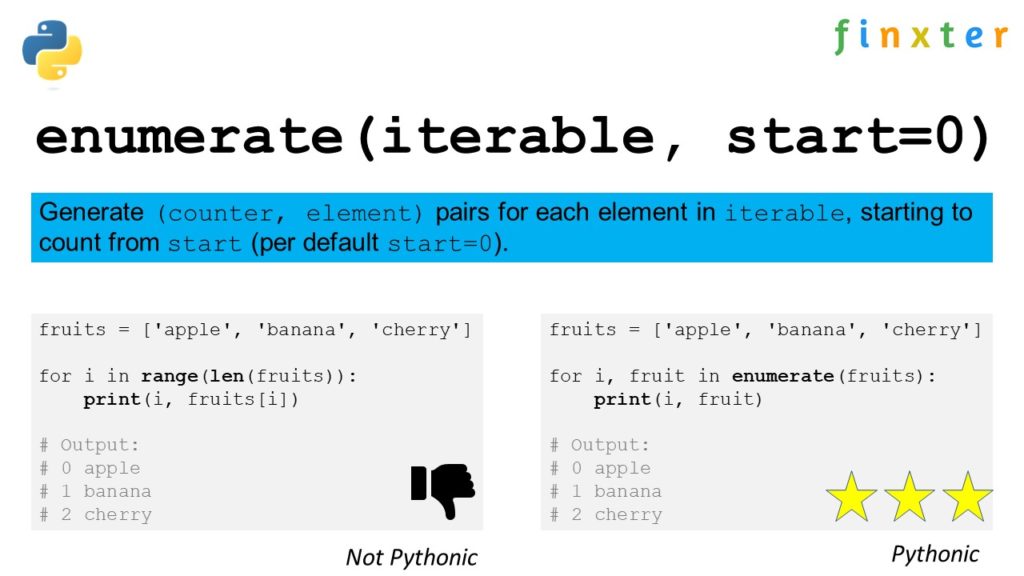
# 1. 数据结构与Python列表基础
在本章中,我们将开始探索计算机科学的基础——数据结构,并介绍Python语言中最常用的数据结构之一:列表。数据结构是存储和组织数据的一种方式,它能够使信息的检索、处理、存储和更新更加高效。我们将深入讨论列表的基本属性和操作,包括创建、添加、删除元素以及如何访问列表中的元素。
首先,我们会介绍列表的定义和特点,列表在Python中是一个有序的、可变的集合,它能够容纳不同类型的数据,并且可以随时进行增删改查操作。我们会演示如何通过简单的代码来创建和初始化列表:
```python
# 创建一个空列表
my_list = []
# 创建一个包含初始值的列表
fruits = ['apple', 'banana', 'cherry']
```
接着,我们会讲解列表索引和切片的概念,这是列表操作中非常重要的基础知识点。索引让我们可以访问列表中的单个元素,而切片允许我们获取列表的一个子集。此外,本章还会涉及列表的常见操作,比如追加元素、插入元素、删除元素以及列表排序等,为接下来的章节打下坚实的基础。
# 2. 栈的实现与应用
## 2.1 栈的概念及其在计算机科学中的作用
栈是一种遵循后进先出(Last In, First Out,LIFO)原则的抽象数据类型。在计算机科学中,栈的概念无处不在,它被用于函数调用、递归算法、表达式求值、括号匹配检测、浏览器后退功能等。栈操作通常只允许在栈顶进行,包括压栈(push)和出栈(pop)。理解栈的原理和操作对于解决实际问题至关重要,因为它提供了一种处理复杂数据的简单而强大的方法。
## 2.2 使用Python列表实现栈
### 2.2.1 栈的基本操作
在Python中,可以非常方便地使用列表(list)数据结构来实现栈的基本操作。以下是一个简单的栈的实现,包括压栈和出栈操作:
```python
class Stack:
def __init__(self):
self.items = []
def is_empty(self):
return len(self.items) == 0
def push(self, item):
self.items.append(item)
def pop(self):
if self.is_empty():
raise IndexError("pop from an empty stack")
return self.items.pop()
def peek(self):
if self.is_empty():
raise IndexError("peek from an empty stack")
return self.items[-1]
```
- `__init__`: 这个方法用于初始化栈,创建一个空列表来存储栈内元素。
- `is_empty`: 这个方法用来检查栈是否为空,如果为空则返回`True`。
- `push`: 这个方法将一个元素添加到栈顶,通过列表的`append`方法实现。
- `pop`: 这个方法移除并返回栈顶元素,使用列表的`pop`方法实现。如果栈为空,则抛出一个`IndexError`异常。
- `peek`: 这个方法返回栈顶元素但不移除它,通过对列表的最后一个元素使用索引`-1`访问实现。
### 2.2.2 栈的应用实例:括号匹配检测
栈常被用于括号匹配检测,这对于编译器和解释器来说是一个基础但极其重要的任务。以下是一个如何使用栈来检测字符串中括号是否正确匹配的示例:
```python
def check_parentheses(input_str):
stack = Stack()
matching = {')': '(', ']': '[', '}': '{'}
for char in input_str:
if char in matching.values():
stack.push(char)
elif char in matching.keys():
if stack.is_empty() or stack.pop() != matching[char]:
return False
return stack.is_empty()
```
- `check_parentheses`: 这个函数接收一个字符串`input_str`作为输入,并使用一个栈来检测其中的括号是否匹配。
- `matching`: 这是一个字典,定义了匹配的括号对。
- 遍历`input_str`中的每个字符,如果字符是一个左括号(`(`、`[`或`{`),则将其压入栈中。
- 如果字符是一个右括号(`)`、`]`或`}`),则检查栈是否为空或栈顶元素是否与之匹配。如果不匹配或栈为空,返回`False`。
- 如果遍历完成并且栈为空,说明所有的括号都正确匹配,返回`True`。
## 2.3 栈的算法问题和解决方案
### 2.3.1 十进制转二进制
使用栈可以高效地实现十进制到二进制的转换,这是一个将数字转换为二进制表示的经典问题。转换过程可以通过反复除以2并收集余数来完成,栈在这里可以用来存储余数。
```python
def decimal_to_binary(n):
stack = Stack()
while n > 0:
remainder = n % 2
stack.push(remainder)
n = n // 2
result = ''
while not stack.is_empty():
result += str(stack.pop())
return result or '0'
```
- `decimal_to_binary`: 这个函数接收一个十进制数`n`并返回其二进制表示。
- 在while循环中,使用`%`操作符获取余数,并使用`//`操作符进行整除。
- 将余数压入栈中,然后继续对`n`进行除以2的操作。
- 当`n`变为0时,while循环结束,使用另一个while循环出栈并将结果拼接到字符串`result`中。
### 2.3.2 迷宫路径问题
栈也可以用于解决迷宫路径问题,即找到从起点到终点的路径。以下是一个简单的算法实现:
```python
def find_path(maze, start, end):
def is_valid_move(maze, position):
x, y = position
return maze and 0 <= x < len(maze) and 0 <= y < len(maze[0]) and maze[x][y] != 'X'
def move(position, move):
x, y = position
return (x + move[0], y + move[1])
stack = Stack()
stack.push((start, [''])) # Start position and path taken to reach it
while not stack.is_empty():
(current, path) = stack.pop()
x, y = current
if current == end:
return path
directions = [(0, 1), (1, 0), (0, -1), (-1, 0)]
for move in directions:
next_position = move(current)
if is_valid_move(maze, next_position):
new_path = path + [move]
stack.push((next_position, new_path))
return "No path found"
```
- `find_path`: 这个函数接收迷宫`maze`、起点`start`和终点`end`作为参数。
- `is_valid_move`: 一个辅助函数,用于判断从当前位置移动到下一个位置是否有效。
- `move`: 一个辅助函数,用于计算从当前位置按指定方向移动后的新位置。
- 初始化栈,并将起点与到达起点的初始路径(只包含起点本身)推入栈中。
- 循环遍历栈,直到找到终点或栈为空(无法到达终点)。
- 对于栈顶的每个位置,尝试所有四个方向的移动,如果移动到的新位置是有效的,将新位置和更新后的路径推入栈中。
- 如果栈为空但没有找到终点,则返回“无路径可走”。
本章节中的实例展示了栈数据结构在算法中的应用,及其在解决实际问题时的高效性。随着数据结构的深入学习,可以进一步探索栈的其他应用和更多高级算法。
# 3. 队列的实现与应用
0
0
相关推荐
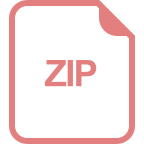
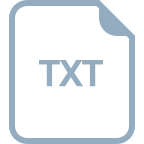
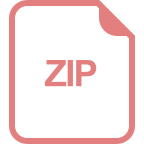
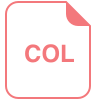




