【数据结构转换术】:从Python字典到集合的转换技巧
发布时间: 2024-09-12 03:24:06 阅读量: 14 订阅数: 26 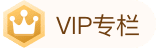
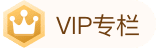

# 1. 数据结构转换的重要性与应用场景
在数据处理的世界里,数据结构的选择至关重要,它直接关系到算法的效率、系统的性能,甚至影响到程序的可读性和可维护性。数据结构转换,即在不同数据类型之间转换数据,是数据处理中的一项基础而核心的操作。理解并掌握数据结构转换的技巧,对于提升数据处理能力、优化代码逻辑有着重要的意义。
## 1.1 数据结构转换的应用场景
数据结构转换的需求往往源自于实际应用中的具体问题。比如,开发者在处理数据时经常需要将字典(Dictionary)中的数据提取出来,形成集合(Set)以便进行快速查找;又或者在数据统计时,需要将列表(List)中的元素整理成字典以方便记录每个元素出现的频率。
举一个简单的例子,在处理日志文件时,我们可能需要将包含相同日志信息的字典转换为集合,以排除重复的条目,从而更高效地对日志进行分析和统计。
```python
log_dict = {"error": 10, "info": 25, "warning": 12, "error": 8}
log_set = set(log_dict.keys())
print(log_set) # 输出: {'info', 'error', 'warning'}
```
在上述代码中,我们从一个字典中创建了一个集合,排除了重复的键(key),这在统计日志事件的种类时十分有用。
## 1.2 数据结构转换的重要性
数据结构转换的重要性可以从以下几个方面体现:
- **数据去重**:集合的不重复特性允许我们快速去除数据中的重复项,而字典允许我们根据键值对存储和检索信息。
- **提高效率**:特定的数据结构对于某些操作来说更加高效,例如,集合可以快速进行并集、交集等集合运算。
- **优化代码**:使用合适的数据结构可以简化代码逻辑,提高代码的可读性和可维护性。
随着对数据结构转换重要性的了解,我们将在后续章节深入探索如何在Python中操作字典和集合,以及如何有效地在二者之间进行转换,以应对复杂的数据处理场景。
# 2. Python字典基础知识
在深入探讨如何将字典转换为集合之前,我们需要先建立对字典这一数据结构的基础认识。Python中的字典是通过键值对存储数据的可变容器模型,它能够存储任意类型的数据,并且在性能上表现出色,特别是在进行查找和更新操作时。本章将详细介绍字典的定义、特性、操作方法以及其高级特性。
## 2.1 字典的定义与特性
### 2.1.1 字典的数据类型与创建
字典在Python中是由大括号`{}`包围起来的数据结构,并且由一系列的键值对组成。每个键值对之间用逗号`,`分隔。键和值之间用冒号`:`来分隔。
```python
# 创建一个空字典
empty_dict = {}
# 创建一个带有初始数据的字典
person = {
'name': 'Alice',
'age': 30,
'country': 'Wonderland'
}
```
字典中的键必须是不可变类型,如字符串、数字或元组。这些键值对是无序的,即它们不会按照添加的顺序排列。
### 2.1.2 字典的操作方法与应用场景
字典提供了丰富的操作方法,比如添加、删除、查找键值对,以及获取字典的键、值和键值对等。
```python
# 添加键值对
person['city'] = 'Wonderland'
# 删除键值对
del person['age']
# 获取字典中的值
print(person['name']) # 输出: Alice
# 获取字典中所有的键
print(list(person.keys())) # 输出: ['name', 'country', 'city']
# 获取字典中所有的值
print(list(person.values())) # 输出: ['Alice', 'Wonderland', 'Wonderland']
# 获取字典中所有的键值对
print(list(person.items())) # 输出: [('name', 'Alice'), ('country', 'Wonderland'), ('city', 'Wonderland')]
```
字典广泛应用于记录和管理数据,例如用户信息、数据库记录等场景。在这些应用中,快速访问和更新数据的需求使字典成为一个理想的选择。
## 2.2 字典的高级特性
### 2.2.1 字典推导式及其实践
字典推导式是一种从其他数据结构中快速生成字典的方式,它的语法和列表推导式相似,但使用了大括号`{}`。
```python
# 使用字典推导式创建字典
squares = {x: x*x for x in range(6)}
print(squares) # 输出: {0: 0, 1: 1, 2: 4, 3: 9, 4: 16, 5: 25}
```
字典推导式适用于数据清洗、数据转换等场景。它能够帮助我们以非常简洁的方式从复杂的数据结构中提取所需的信息并创建字典。
### 2.2.2 字典键的不可变性和哈希性
字典之所以能够提供高效的查找性能,是因为它的键必须是不可变且具有哈希性的对象。这意味着键对象必须能够进行哈希运算,并且在整个字典的生命周期中保持不变。
```python
# 正确的键
my_dict = {tuple([1, 2, 3]): 'OK'}
# 错误的键尝试
try:
my_dict[[1, 2, 3]] = 'Not OK'
except TypeError as e:
print(e) # 输出: unhashable type: 'list'
```
哈希函数将键对象转换为一个整数,用于在内部维护一个哈希表,这使得字典能够快速定位和访问存储的值。因此,确保字典键的不可变性和哈希性对于保证字典的性能至关重要。
在掌握了Python字典的基础知识之后,接下来将深入探讨如何将字典转换为集合,并讨论这种转换在不同场景下的实践和优化。
# 3. 集合的数据结构与操作
## 3.1 集合的创建与基本操作
### 3.1.1 集合的定义和特点
在Python中,集合(set)是一个无序的、不重复的元素集。它主要用于进行成员关系测试和消除重复元素。集合类似于数学中的集合论,因此在集合中进行的操作与数学上的集合操作类似。创建一个集合的基本语法为 `set([iterable])`,其中可迭代对象(iterable)包括列表、元组、字典、字符串等。
集合的定义:
```python
my_set = set([1, 2, 3, 3, 4, 4, 5]) # 创建一个集合
```
上述代码中,集合 `my_set` 包含了不重复的元素 `{1, 2, 3, 4, 5}`。集合的特点包括:
- **唯一性**:集合中的元素都是唯一的,即不允许出现重复元素。
- **无序性**:集合中的元素不具有顺序,即集合是无序的集合。
- **可变性**:集合是可变的,可以向其中添加和删除元素。
### 3.1.2 集合的常用操作方法
集合提供了多种方法来进行操作,以下是一些常用的方法:
- `add()`:为集合添加一个元素
- `update()`:为集合添加多个元素
- `remove()`:移除集合中的一个元素
- `discard()`:移除集合中的一个元素,如果元素不存在,则不进行任何操
0
0
相关推荐
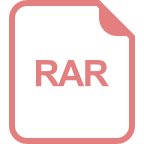
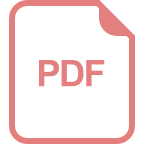
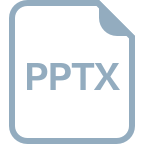





