React Hooks深度解析:掌握状态管理与副作用处理,构建更优雅的前端应用
发布时间: 2024-07-20 02:31:38 阅读量: 36 订阅数: 22 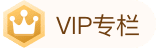
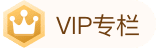
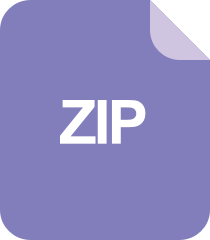
tetris-reacthooks:用React钩子制作的俄罗斯方块俄罗斯方块游戏
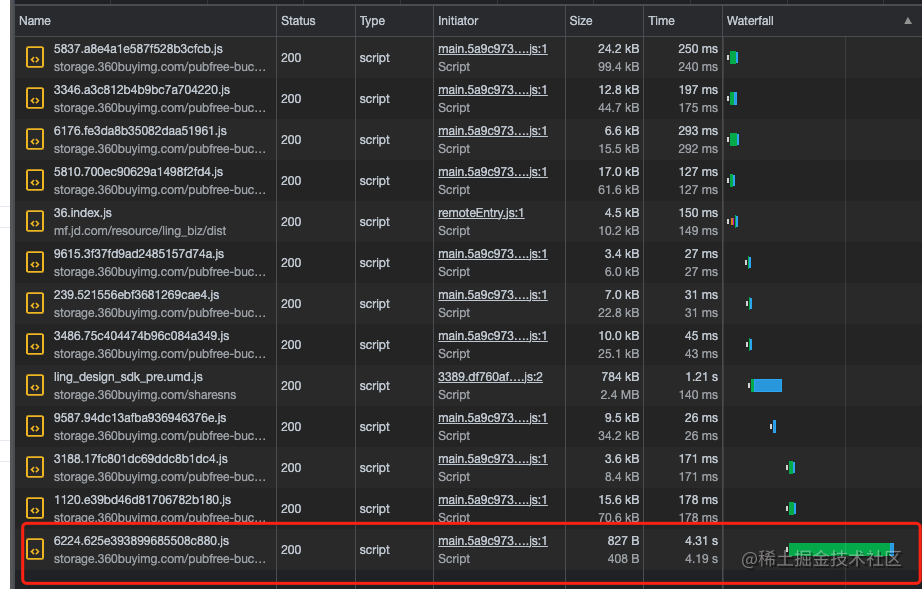
# 1. React Hooks简介**
React Hooks是React 16.8中引入的一组API,用于管理状态和副作用,它们简化了组件的编写和维护。与传统组件中使用生命周期方法不同,Hooks允许我们在函数组件中使用状态和副作用。
Hooks通过提供一组预定义的函数来实现,这些函数以组件的props和state作为参数,并返回一个新的state或副作用。最常用的Hooks包括useState、useEffect和useCallback。
useState Hook用于管理组件的状态,它接受一个初始状态值作为参数,并返回一个包含当前状态值和一个更新状态的函数的数组。useEffect Hook用于处理副作用,它接受一个函数作为参数,该函数将在组件渲染后或特定依赖项发生变化后执行。
# 2. 状态管理与副作用处理
### 2.1 useState Hook
#### 2.1.1 基本用法
`useState` Hook 是 React 中最基本的 Hook,用于管理组件状态。它接受一个初始状态值作为参数,并返回一个数组,数组的第一个元素是当前状态值,第二个元素是一个函数,用于更新状态。
```javascript
const [count, setCount] = useState(0);
```
上述代码中,`count` 是当前状态值,`setCount` 是用于更新状态的函数。要更新状态,只需调用 `setCount` 函数,并传入新的状态值即可。
```javascript
setCount(count + 1);
```
#### 2.1.2 数组和对象状态
`useState` Hook 不仅可以管理基本类型值,还可以管理数组和对象等复杂数据结构。
**数组状态**
```javascript
const [todos, setTodos] = useState([]);
```
**对象状态**
```javascript
const [user, setUser] = useState({ name: '', age: 0 });
```
更新数组或对象状态时,需要使用扩展运算符(...),以避免直接修改原有状态。
```javascript
setTodos([...todos, newTodo]);
setUser({ ...user, age: user.age + 1 });
```
### 2.2 useEffect Hook
#### 2.2.1 副作用的定义
副作用是指在组件生命周期中执行的操作,这些操作会对组件外部产生影响,例如:
* 发送网络请求
* 设置计时器
* 修改 DOM
#### 2.2.2 useEffect Hook 的语法和用法
`useEffect` Hook 用于管理副作用。它接受两个参数:
* **回调函数:**副作用的具体实现。
* **依赖项数组:**当依赖项数组中的值发生变化时,副作用将被重新执行。
```javascript
useEffect(() => {
// 副作用的具体实现
}, [dependency1, dependency2, ...]);
```
**空依赖项数组:**如果依赖项数组为空,则副作用只会在组件挂载时执行一次。
```javascript
useEffect(() => {
// 只在组件挂载时执行一次
}, []);
```
### 2.3 useCallback Hook
#### 2.3.1 优化性能
`useCallback` Hook 用于创建经过优化的回调函数,以避免不必要的重新渲染。
当一个组件依赖于一个回调函数,并且该回调函数在组件每次重新渲染时都会重新创建时,就会发生不必要的重新渲染。`useCallback` Hook 可以通过缓存回调函数来解决这个问题。
```javascript
const memoizedCallback = useCallback(() => {
// 回调函数的具体实现
}, [dependency1, dependency2, ...]);
```
#### 2.3.2 避免不必要的重新渲染
使用 `useCallback` Hook 创建的回调函数,只有在依赖项数组中的值发生变化时才会重新创建。这可以防止不必要的重新渲染,从而提高组件的性能。
```javascript
const MyComponent = () => {
const memoizedCallback = useCallback(() => {
// 回调函数的具体实现
}, [dependency1, dependency2, ...]);
return (
<button onClick={memoizedCallback}>
Click Me
</button>
);
};
```
# 3. 高级Hooks应用
### 3.1 useReducer Hook
#### 3.1.1 状态管理的替代方案
useReducer Hook是一种更高级的状态管理工具,它提供了比useState Hook更强大的功能。它允许你使用一个reducer函数来管理状态,该函数接收当前状态和一个action,并返回一个新的状态。
```javascript
const reducer = (state, action) => {
switch (action.type) {
case 'INCREMENT':
return { count: state.count + 1 };
case 'DECREMENT':
return { count: state.count - 1 };
default:
return state;
}
};
const [state, dispatch] = useReducer(reducer, { count: 0 });
```
在上面的
0
0
相关推荐
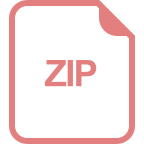
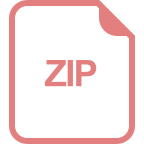
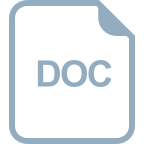
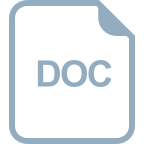
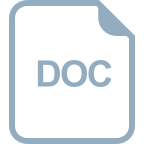
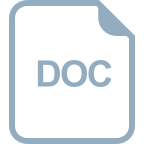
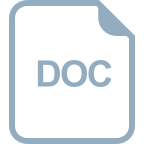
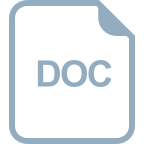