OpenCV图像色彩分析:从颜色直方图到颜色聚类,深入理解图像色彩特征
发布时间: 2024-08-11 09:05:39 阅读量: 61 订阅数: 23 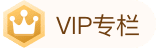
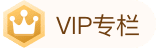
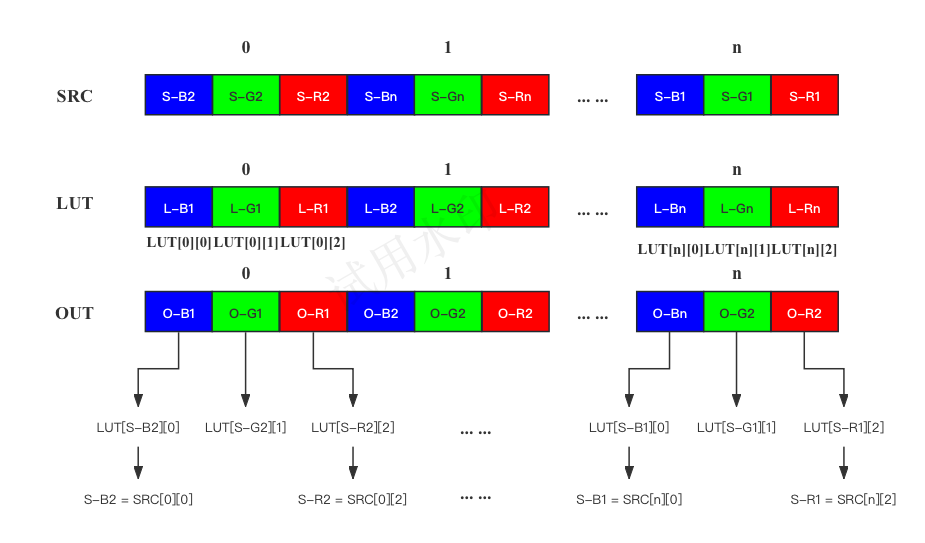
# 1. OpenCV图像色彩分析概述**
图像色彩分析是计算机视觉领域的重要组成部分,它通过提取和分析图像中的颜色信息来了解图像的内容和特性。OpenCV(Open Source Computer Vision Library)是一个强大的开源计算机视觉库,它提供了丰富的函数和算法,用于图像色彩分析。
OpenCV中的图像色彩分析技术主要包括:
- **颜色直方图:**用于提取图像中颜色的分布特征。
- **颜色聚类:**将图像中的颜色分组到不同的类别中。
- **颜色转换:**将图像从一种颜色空间转换到另一种颜色空间,以获得不同的颜色表示。
# 2. 颜色直方图:图像色彩特征提取
### 2.1 颜色直方图的基本原理
#### 2.1.1 颜色空间和量化
颜色直方图是一种图像色彩特征提取技术,它将图像中每个像素的颜色分布统计成一个直方图。为了构建颜色直方图,首先需要将图像中的每个像素的颜色表示为一个向量,通常使用RGB(红、绿、蓝)颜色空间。
为了降低颜色直方图的维度,需要对颜色空间进行量化。量化是指将连续的颜色空间划分为离散的区间,每个区间代表一个颜色桶。例如,对于RGB颜色空间,可以将每个颜色通道量化为8个区间,总共形成256个颜色桶。
#### 2.1.2 直方图的构建
构建颜色直方图的过程如下:
1. **初始化直方图:**创建一个包含所有颜色桶的数组,并将其值初始化为0。
2. **遍历图像像素:**对于图像中的每个像素,将其颜色向量映射到相应颜色桶。
3. **累加像素计数:**将对应颜色桶的值加1。
4. **归一化直方图:**将直方图中每个颜色桶的值除以像素总数,得到归一化直方图。
归一化直方图的每个桶表示该颜色在图像中出现的频率。
### 2.2 颜色直方图的应用
#### 2.2.1 图像相似性比较
颜色直方图可以用来比较图像的相似性。相似图像通常具有相似的颜色分布,因此它们的直方图也相似。为了比较直方图,可以使用以下距离度量:
* **直方图交集(Histogram Intersection):**计算两个直方图中最小值的总和。
* **卡方距离(Chi-Square Distance):**计算两个直方图中每个桶的差值的平方,然后求和。
距离度量越小,图像越相似。
#### 2.2.2 图像分类
颜色直方图还可以用于图像分类。通过将图像的直方图作为特征向量,可以训练机器学习模型来区分不同类别的图像。例如,可以训练一个模型来识别猫和狗的图像,其中猫的图像具有较高的黄色和橙色直方图,而狗的图像具有较高的棕色和灰色直方图。
### 代码示例
以下代码示例演示了如何使用OpenCV计算图像的颜色直方图:
```python
import cv2
import numpy as np
# 加载图像
image = cv2.imread('image.jpg')
# 转换图像到HSV颜色空间
hsv = cv2.cvtColor(image, cv2.COLOR_BGR2HSV)
# 量化颜色空间
h = hsv[:, :, 0]
s = hsv[:, :, 1]
v = hsv[:, :, 2]
# 构建直方图
hist_h = cv2.calcHist([h], [0], None, [256], [0, 256])
hist_s = cv2.calcHist([s], [0], None, [256], [0, 256])
hist_v = cv2.calcHist([v], [0], None, [256], [0, 256])
# 绘制直方图
plt.plot(hist_h, color='r')
plt.plot(hist_s, color='g')
plt.plot(hist_v, color='b')
plt.show()
```
### 逻辑分析
* `cv2.calcHist()`函数用于计算图像的直方图。
* 第一个参数是图像数组,第二个参数是通道索引列表(在本例中,只使用色调通道),第三个参数是掩码(在本例中,没有使用),第四个参数是直方图的bin数(在本例中,使用256个bin),第五个参数是直方图的范围(在本例中,使用[0, 256])。
* `plt.plot()`函数用于绘制直方图。
# 3. 颜色聚类:图像色彩特征分组**
**3.1 K-Means聚类算法**
**3.1.1 算法原理**
K-Means聚类是一种无监督学习算法,用于将数据点分组为K个簇。该算法基于以下原理:
* **簇中心:**每个簇由一个簇中心表示,该簇中心是簇中所有数据点的平均值。
* **距离度量:**数据点与簇中心的距离通常使用欧氏距离或曼哈顿距离来衡量。
* **迭代优化:**算法迭代地执行以下步骤,直到收敛:
* 将每个数据点分配到离它最近的簇中心。
* 更新每个簇的簇中心,使其成为簇中所有数据点的平均值。
**3.1.2 聚类过程**
K-Means聚类算法的步骤如下:
1. **初始化:**随机选择K个数据点作为初始簇中心。
2. **分配:**将每个数据点分配到离它最近的簇中心。
3. **更新:**计算每个簇中所有数据点的平均值,并将其作为新的簇中心。
4. **重复:**重复步骤2和3,直到簇中心不再变化或达到最大迭代次数。
**3.2 颜色聚类的应用**
颜色聚类在图像处理中具有广泛的应用,包括:
**3.2.1 图像分割**
颜色聚类可以将图像分割成具有相似颜色的区域。这在对象检测、背景移除和图像编辑等任务中很有用。
**3.2.2 图像量化**
颜色聚类可以减少图像中使用的颜色数量,同时保持图像的视觉质量。这在图像压缩和显示设备上显示图像时很有用。
**代码示例:**
以下Python代码演示了如何使用OpenCV进行K-Means颜色聚类:
```python
import cv2
import numpy as np
# 读取图像
im
```
0
0
相关推荐
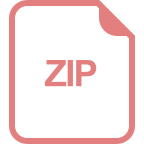
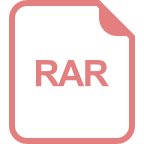






