BeautifulSoup性能优化手册:加速数据处理,提高效率
发布时间: 2024-12-07 05:26:40 阅读量: 10 订阅数: 11 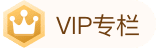
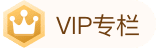
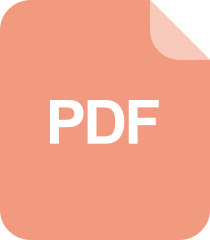
提升网页数据提取效率:PyQuery、lxml和BeautifulSoup的协同作战
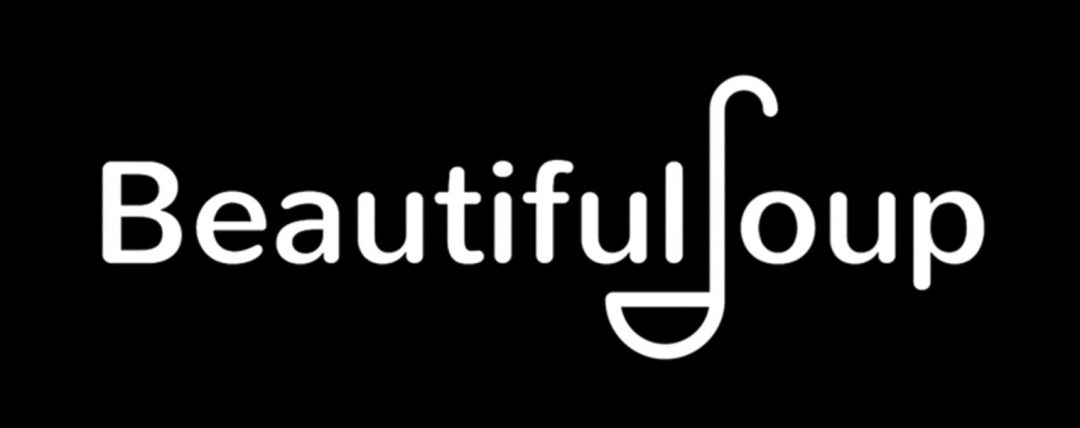
# 1. BeautifulSoup库简介与安装
BeautifulSoup是一个在Python编程语言中常用的库,它能够从HTML或XML文件中提取数据。它是许多数据抓取和网络爬虫应用的基础工具。无论你是数据科学家、开发人员还是网络爬虫爱好者,学习和掌握BeautifulSoup对于提升你的技术栈有着重要的意义。
## 安装BeautifulSoup库
首先,你需要确保你的Python环境已经安装了bs4(BeautifulSoup的包名)。你可以通过以下简单的命令安装它:
```bash
pip install beautifulsoup4
```
如果你需要解析器,比如`lxml`,同样可以使用pip来安装:
```bash
pip install lxml
```
需要注意的是,虽然`lxml`不是必须的,但它比内置的解析器要快,并且错误处理也更为优秀。安装完成后,你就可以开始使用BeautifulSoup了。
```python
from bs4 import BeautifulSoup
# 示例HTML内容
html_doc = """
<html>
<head>
<title>Page Title</title>
</head>
<body>
<div class="container">
<p class="title">Hello World!</p>
</div>
</body>
</html>
# 使用BeautifulSoup解析HTML
soup = BeautifulSoup(html_doc, 'lxml')
```
以上步骤显示了如何在Python环境中安装并开始使用BeautifulSoup库。在后续章节中,我们将深入探讨如何利用BeautifulSoup进行数据提取和网页内容分析。
# 2. BeautifulSoup基础使用技巧
## 2.1 HTML/XML解析入门
### 2.1.1 标签的查找与选择
在使用BeautifulSoup处理HTML或XML文档时,查找与选择特定标签是基础操作之一。BeautifulSoup库提供了一系列查找标签的方法,这些方法不仅简单易用,而且功能强大。
例如,使用`find()`方法可以根据标签名、属性等条件查找第一个匹配的标签:
```python
from bs4 import BeautifulSoup
html_doc = """
<html><head><title>The Dormouse's story</title></head>
<body>
<p class="title"><b>The Dormouse's story</b></p>
<p class="story">Once upon a time there were three little sisters; and their names were
<a href="http://example.com/elsie" class="sister" id="link1">Elsie</a>,
<a href="http://example.com/lacie" class="sister" id="link2">Lacie</a> and
<a href="http://example.com/tillie" class="sister" id="link3">Tillie</a>;
and they lived at the bottom of a well.</p>
soup = BeautifulSoup(html_doc, 'html.parser')
# 查找第一个title标签
first_title = soup.find('title')
print(first_title.text) # 输出: The Dormouse's story
# 查找具有特定类名的p标签
title_class = soup.find('p', class_='title')
print(title_class.text) # 输出: The Dormouse's story
```
### 2.1.2 文本和属性的提取
除了查找标签,BeautifulSoup还提供了方法提取标签内的文本和属性信息。提取文本时,可以使用`.text`或`.get_text()`方法;而获取属性时,则直接通过标签的字典接口访问。
提取文本的示例代码如下:
```python
# 获取title标签内的文本
title_text = first_title.get_text()
print(title_text) # 输出: The Dormouse's story
```
提取属性的示例代码如下:
```python
# 获取a标签的href属性
link = soup.find('a')
href_value = link.get('href')
print(href_value) # 输出: http://example.com/elsie
```
## 2.2 结构化数据提取
### 2.2.1 列表和字典的转换
在处理网页数据时,经常会遇到将数据结构化的需求。BeautifulSoup可以把找到的标签列表转换为Python的列表和字典结构,方便后续的数据处理和分析。
将标签列表转换为列表:
```python
# 查找所有具有类名"title"的p标签
all_titles = soup.find_all('p', class_='title')
titles_list = [title.text for title in all_titles]
print(titles_list) # 输出: ['The Dormouse's story']
```
将标签转换为字典:
```python
# 将单个标签转换为字典形式
title_dict = {title.name: title.get_text() for title in all_titles}
print(title_dict) # 输出: {'p': 'The Dormouse's story'}
```
### 2.2.2 树形结构的遍历与解析
BeautifulSoup支持基于DOM的树形结构遍历和解析。通过遍历节点,可以深入访问文档的层级结构,提取更多的信息。
遍历标签的父节点:
```python
# 获取a标签的父节点
a_parent = link.parent
print(a_parent.name) # 输出: p
```
遍历标签的所有子节点:
```python
# 获取p标签内的所有子节点,并打印其名称
for child in a_parent.children:
print(child.name)
```
通过这些方法,开发者可以灵活地访问和解析HTML/XML文档的各个部分,从而提取所需的数据。
## 2.3 高级节点操作
### 2.3.1 修改和删除节点
在对HTML/XML文档进行解析后,有时需要对文档进行修改,比如修改标签的文本内容、属性或者删除不需要的节点。BeautifulSoup提供了便捷的方法来实现这些操作。
修改节点的文本:
```python
# 修改链接文本
link.string = 'New Elsie'
print(link) # 输出: <a href="http://example.com/elsie" class="sister" id="link1">New Elsie</a>
```
修改节点的属性:
```python
# 修改链接的href属性
link['href'] = 'http://example.com/newelsie'
print(link) # 输出: <a href="http://example.com/newelsie" class="sister" id="link1">New Elsie</a>
```
删除节点:
```python
# 删除链接节点
link.extract()
print(soup.prettify()) # 输出时不会包含已删除的链接
```
### 2.3.2 节点的插入和替换
除了修改和删除节点外,BeautifulSoup还可以在文档中插入新节点或替换现有节点。这些操作是动态网页内容更新和数据清洗的重要手段。
插入节点:
```python
# 在文档底部添加一个新的段落
new_paragraph = soup.new_tag('p', text='This is a new paragraph.')
soup.append(new_paragraph)
print(soup.prettify()) # 输出将包括新添加的段落
```
替换节点:
```python
# 替换已存在的链接
from bs4.element impo
```
0
0
相关推荐
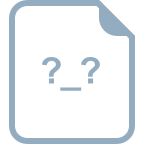
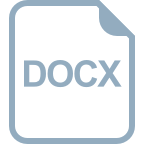
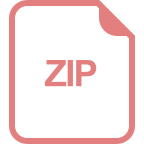
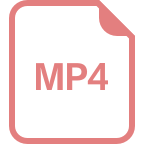
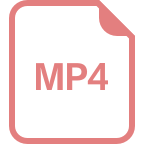
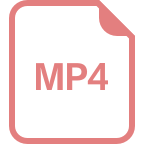
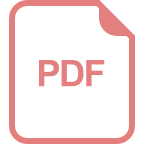