机器人路径规划中的全局规划与局部规划:原理与实践,助你轻松应对复杂环境
发布时间: 2024-08-26 06:06:03 阅读量: 365 订阅数: 22 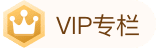
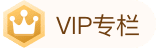

# 1. 机器人路径规划概述
机器人路径规划是机器人学中的一项基本任务,其目的是为机器人生成从起点到目标点的最优路径。它涉及到障碍物检测、路径搜索和运动控制等多个方面。
路径规划算法可以分为全局规划和局部规划。全局规划算法考虑机器人从起点到目标点的整体环境,生成一条全局最优路径。局部规划算法则根据当前传感器数据,生成一条局部最优路径,用于实时避障和运动控制。
全局规划算法和局部规划算法协同工作,可以实现机器人高效、安全的导航。在复杂环境中,机器人需要根据不同的情况切换不同的规划算法,以适应环境的变化。
# 2. 全局规划理论与实践
### 2.1 全局规划算法
全局规划算法旨在为机器人生成从起点到目标点的路径,考虑全局环境信息。常用的全局规划算法包括:
#### 2.1.1 栅格法
栅格法将环境划分为均匀的网格,每个网格代表一个可通行或不可通行的单元格。算法通过搜索网格中的路径来生成机器人路径。
**代码块:**
```python
import numpy as np
def raster_planning(map, start, goal):
"""
栅格法全局规划算法
参数:
map: 环境地图,0表示可通行,1表示不可通行
start: 起点坐标
goal: 目标点坐标
返回:
路径点列表
"""
# 初始化网格
width, height = map.shape
grid = np.zeros((width, height))
grid[map == 1] = 1
# 搜索路径
path = []
current = start
while current != goal:
# 获取当前位置周围的邻居
neighbors = [(current[0] + 1, current[1]), (current[0] - 1, current[1]),
(current[0], current[1] + 1), (current[0], current[1] - 1)]
# 过滤不可通行的邻居
neighbors = [n for n in neighbors if grid[n[0], n[1]] == 0]
# 选择距离目标最近的邻居
next_neighbor = min(neighbors, key=lambda n: np.linalg.norm(n - goal))
# 更新当前位置
current = next_neighbor
# 添加路径点
path.append(current)
return path
```
**逻辑分析:**
* 算法将环境地图划分为网格,并标记不可通行区域。
* 算法从起点开始,逐个搜索网格中的邻居,选择距离目标最近的邻居作为下一个路径点。
* 算法重复此过程,直到到达目标点。
#### 2.1.2 概率路线图法
概率路线图法生成一个连接随机采样点的路线图,然后搜索路线图中的路径。
**代码块:**
```python
import random
import networkx as nx
def probabilistic_roadmap(map, start, goal, num_samples):
"""
概率路线图法全局规划算法
参数:
map: 环境地图,0表示可通行,1表示不可通行
start: 起点坐标
goal: 目标点坐标
num_samples: 采样点数
返回:
路径点列表
"""
# 采样随机点
points = [start]
for _ in range(num_samples):
while True:
point = (random.randint(0, map.shape[0]), random.randint(0, map.shape[1]))
if map[point[0], point[1]] == 0 and point not in points:
points.append(point)
break
# 构建路线图
graph = nx.Graph()
graph.add_nodes_from(points)
for i in range(len(points)):
for j in range(i + 1, len(points)):
if is_path_valid(map, points[i], points[j]):
graph.add_edge(points[i], points[j])
# 搜索路线图
path = nx.shortest_path(graph, start, goal)
return path
```
**逻辑分析:**
* 算法随机采样环境中的点,并构建一个连接这些点的路线图。
* 算法使用路径验证函数检查路线图中的路径是否可通行。
* 算法使用最短路径算法搜索路线图中的路径。
#### 2.1.3 人工势场法
人工势场法将机器人视为带电粒子,环境中的障碍物和目标点分别产生排斥力和吸引力。
**代码块:**
```python
import numpy as np
def artificial_potential_field(map, start, goal, eta, alpha, beta):
"""
人工势场法全局规划算法
参数:
map: 环境地图,0表示可通行,1表示不可通行
start: 起点坐标
goal: 目标点坐标
eta: 吸引力常数
alpha: 排斥力常数
beta: 排斥力指数
返回:
路径点列表
"""
# 初始化势场
potential = np.zeros(map.shape)
potential[goal[0], goal[1]] = eta
# 更新势场
for i in range(map.shape[0]):
for j in range(map.shape[1]):
if map[i, j] == 1:
potential[i, j] = -alpha / (np.linalg.norm(np.array([i, j]) - start) ** beta)
# 梯度下降
current = start
path = []
while current != goal:
# 计算梯度
gradient = np.gradient(potential, axis=0, axis=1)
# 更新当前位置
current -= gradient[current[0], current[1]]
# 添加路径点
path.append(current)
return path
```
**逻辑分析:**
* 算法初始化一个势场,其中目标点产生吸引力,障碍物产生排斥力。
* 算法使用梯度下降算法更新机器人的位置,使其向目标点移动。
* 算法重复此过程,直到机器人到达目标点。
### 2.2 全局规划实践
#### 2.2.1 路径搜索算法的实现
路径搜索算法的实现包括:
* **Dijkstra 算法:**用于在有权重的图中寻找最短路径。
* **A* 算法:**用于在有权重的图中寻找启发式最短路径。
* **RRT 算法:**用于在未知环境中生成路径。
#### 2.2.2 障碍物处理与优化
障碍物处理与优化技术包括:
* **膨胀算法:**扩大障碍物区域,以提高规划的安全性。
* **细化算法:**细化路径,使其更接近障碍物边界。
* **平滑算法:**平滑路径,使其更平缓。
# 3.1 局部规划算法
局部规划算法主要用于机器人对周围环境的感知和实时决策,以实现机器人在局部范围内的安全、高效运动。其主要任务是根据传感器的实时数据,生成机器人从当前位置到目标位置的安全、可行的运动轨迹。
#### 3.1.1 基于激光雷达的扫描匹配
**算法原理:**
扫描匹配算法利用激光雷达采集的环境点云数据,与已知的环境地图或先验地图进行匹配,从而估计机器人的位姿和运动轨迹。算法的核心思想是通过最小化点云数据与地图之间的距离误差,来求解机器人的位姿变换。
**代码示例:**
```python
import numpy as np
import scipy.optimize
def scan_matching(scan_data, map_data):
"""
扫描匹配算法
参数:
scan_data: 激光雷达采集的点云数据
map_data: 已知的环境地图或先验地图
返回:
robot_pose: 机器人的位姿变换
"""
# 定义距离误差函数
def distance_error(robot_pose):
# 将机器人位姿变换应用到点云数据
transformed_scan_data = transform_point_cloud(scan_data, robot_pose)
# 计算点云数据与地图之间的距离误差
error = np.linalg.norm(transformed_scan_data - map_data)
return error
# 使用优化算法求解位姿变换
robot_pose = scipy.optimize.minimize(distance_error, np.zeros(6)).x
return robot_pose
```
**参数说明:**
* `scan_data`:激光雷达采集的点云数据,是一个包含点云坐标的数组。
* `map_data`:已知的环境地图或先验地图,是一个包含地图坐标的数组。
**逻辑分析:**
1. 定义距离误差函数,用于计算点云数据与地图之间的距离误差。
2. 将机器人位姿变换应用到点云数据,得到变换后的点云数据。
3. 计算变换后的点云数据与地图之间的距离误差。
4. 使用优化算法求解机器人位姿变换,使距离误差最小化。
#### 3.1.2 基于视觉的视觉里程计
**算法原理:**
视觉里程计算法利用视觉传感器(如摄像头)采集的图像序列,来估计机器人的运动轨迹。算法通过识别图像中的特征点,并跟踪这些特征点在连续图像中的运动,来计算机器人的位姿变化。
**代码示例:**
```python
import cv2
import numpy as np
def visual_odometry(image1, image2):
"""
视觉里程计算法
参数:
image1: 第一帧图像
image2: 第二帧图像
返回:
robot_pose: 机器人的位姿变换
"""
# 特征点检测和匹配
features1 = cv2.ORB_create().detectAndCompute(image1, None)
features2 = cv2.ORB_create().detectAndCompute(image2, None)
matches = cv2.BFMatcher(cv2.NORM_HAMMING).match(features1.descriptors, features2.descriptors)
# 特征点匹配的几何验证
good_matches = []
for match in matches:
if match.distance < 0.75 * np.min(match.distance):
good_matches.append(match)
# 计算本质矩阵和位姿变换
essential_matrix, _ = cv2.findEssentialMat(np.array([features1[m.queryIdx].pt for m in good_matches]),
np.array([features2[m.trainIdx].pt for m in good_matches]),
camera_matrix)
robot_pose, _ = cv2.recoverPose(essential_matrix, np.array([features1[m.queryIdx].pt for m in good_matches]),
np.array([features2[m.trainIdx].pt for m in good_matches]),
camera_matrix)
return robot_pose
```
**参数说明:**
* `image1`:第一帧图像。
* `image2`:第二帧图像。
* `camera_matrix`:相机的内参矩阵。
**逻辑分析:**
1. 特征点检测和匹配:使用ORB特征检测器和匹配器,在两帧图像中检测和匹配特征点。
2. 特征点匹配的几何验证:通过距离阈值筛选,去除误匹配的特征点。
3. 计算本质矩阵和位姿变换:使用本质矩阵估计算法,计算两帧图像之间的本质矩阵,并恢复机器人的位姿变换。
#### 3.1.3 基于惯性导航的惯性导航
**算法原理:**
惯性导航算法利用惯性传感器(如加速度计和陀螺仪)采集的数据,来估计机器人的运动轨迹。算法通过积分加速度数据,得到速度和位移,并通过积分陀螺仪数据,得到角速度和姿态。
**代码示例:**
```python
import numpy as np
def inertial_navigation(acc_data, gyro_data, dt):
"""
惯性导航算法
参数:
acc_data: 加速度传感器采集的数据
gyro_data: 陀螺仪传感器采集的数据
dt: 时间间隔
返回:
robot_pose: 机器人的位姿变换
"""
# 积分加速度数据得到速度和位移
velocity = np.cumsum(acc_data) * dt
position = np.cumsum(velocity) * dt
# 积分陀螺仪数据得到角速度和姿态
angular_velocity = np.cumsum(gyro_data) * dt
orientation = np.cumsum(angular_velocity) * dt
# 将速度、位移、角速度、姿态组合成位姿变换
robot_pose = np.concatenate((position, orientation), axis=0)
return robot_pose
```
**参数说明:**
* `acc_data`:加速度传感器采集的数据,是一个包含加速度值的数组。
* `gyro_data`:陀螺仪传感器采集的数据,是一个包含角速度值的数组。
* `dt`:时间间隔。
**逻辑分析:**
1. 积分加速度数据,得到速度和位移。
2. 积分陀螺仪数据,得到角速度和姿态。
3. 将速度、位移、角速度、姿态组合成位姿变换。
# 4. 全局规划与局部规划的协同应用
### 4.1 协同规划框架
协同规划将全局规划和局部规划结合起来,以充分利用各自的优势。有两种主要的协同规划框架:层次规划模型和混合规划模型。
**4.1.1 层次规划模型**
层次规划模型将规划过程分解为多个层次。全局规划器在较高层次生成粗略路径,而局部规划器在较低层次生成详细路径。这种方法可以提高规划效率,因为全局规划器无需考虑所有细节。
**4.1.2 混合规划模型**
混合规划模型同时使用全局规划器和局部规划器。全局规划器生成粗略路径,而局部规划器在运行时对路径进行微调。这种方法可以处理动态环境中的不确定性。
### 4.2 协同规划实践
**4.2.1 规划参数的调优**
协同规划的性能取决于规划参数的调优。这些参数包括全局规划器和局部规划器的权重、采样率和障碍物处理策略。通过调整这些参数,可以优化规划性能以满足特定应用的要求。
**4.2.2 复杂环境下的规划性能分析**
在复杂环境中,协同规划器的性能至关重要。可以使用仿真和实验证明规划器的鲁棒性、效率和准确性。通过分析规划器的性能,可以确定其在不同环境下的适用性。
#### 协同规划示例
考虑一个移动机器人需要在仓库中导航的场景。全局规划器生成一个粗略路径,避免仓库中的障碍物。局部规划器使用激光雷达数据微调路径,以避开动态障碍物,如叉车。
#### 代码示例
```python
import numpy as np
import matplotlib.pyplot as plt
# 全局规划器
def global_planner(map, start, goal):
# 使用栅格法生成粗略路径
path = raster_planner(map, start, goal)
return path
# 局部规划器
def local_planner(map, path, robot_pose):
# 使用激光雷达数据微调路径
path = local_planner_with_lidar(map, path, robot_pose)
return path
# 协同规划
def hybrid_planner(map, start, goal, robot_pose):
# 生成粗略路径
global_path = global_planner(map, start, goal)
# 微调路径
local_path = local_planner(map, global_path, robot_pose)
# 返回最终路径
return local_path
# 测试协同规划器
map = np.zeros((100, 100)) # 地图
start = (0, 0) # 起始位置
goal = (99, 99) # 目标位置
robot_pose = (10, 10) # 机器人当前位置
path = hybrid_planner(map, start, goal, robot_pose)
# 绘制路径
plt.imshow(map)
plt.plot(path[:, 0], path[:, 1], color='red')
plt.show()
```
#### 代码逻辑分析
* `global_planner()` 函数使用栅格法生成粗略路径。
* `local_planner()` 函数使用激光雷达数据微调路径。
* `hybrid_planner()` 函数将全局规划和局部规划结合起来,生成最终路径。
* 测试代码生成路径并将其绘制在地图上。
# 5. 机器人路径规划的未来展望
机器人路径规划技术在未来将持续发展,以满足日益复杂的机器人应用需求。以下是一些未来展望:
### 1. 人工智能与机器学习的整合
人工智能(AI)和机器学习(ML)将进一步与机器人路径规划相结合,使机器人能够适应动态环境、学习和优化其规划策略。例如,使用强化学习算法训练的机器人可以从经验中学习最优路径,并适应环境的变化。
### 2. 多机器人协作规划
随着多机器人系统的普及,协作路径规划将变得至关重要。机器人将需要协调其路径,以避免碰撞并有效地完成任务。分布式算法和基于博弈论的方法将用于解决多机器人协作规划问题。
### 3. 感知和规划的融合
感知和规划将变得更加紧密地集成。机器人将能够实时感知其环境,并根据感知信息动态调整其路径规划。这将提高机器人在未知和动态环境中的导航能力。
### 4. 可解释性和可验证性
机器人路径规划的算法将变得更加可解释和可验证。这对于确保机器人的安全性和可靠性至关重要。可解释性算法将使人类能够理解机器人的决策过程,而可验证性算法将使我们能够证明算法的正确性。
### 5. 异构机器人平台
机器人路径规划算法将被设计为适用于各种异构机器人平台,包括地面机器人、空中机器人和水下机器人。这将使机器人能够在更广泛的应用中部署。
### 6. 云计算和边缘计算
云计算和边缘计算将用于支持机器人路径规划。云计算可以提供强大的计算能力,而边缘计算可以实现低延迟和实时响应。这将使机器人能够处理复杂的环境并快速做出决策。
### 7. 标准化和互操作性
机器人路径规划算法和接口将变得更加标准化和互操作性。这将使机器人能够轻松地集成到不同的系统中,并促进算法和技术的共享。
0
0
相关推荐
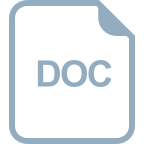
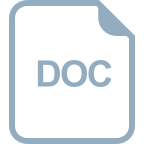
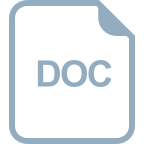
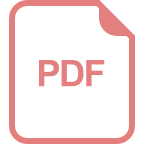
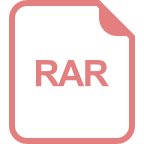
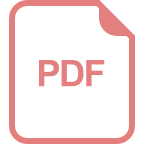
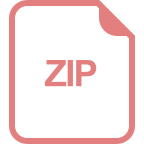
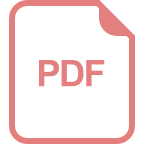
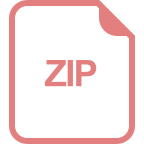