【PHP图片处理宝典】:从入门到精通,全面提升图片处理技能
发布时间: 2024-07-23 18:32:24 阅读量: 16 订阅数: 25 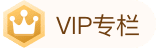
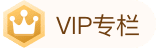
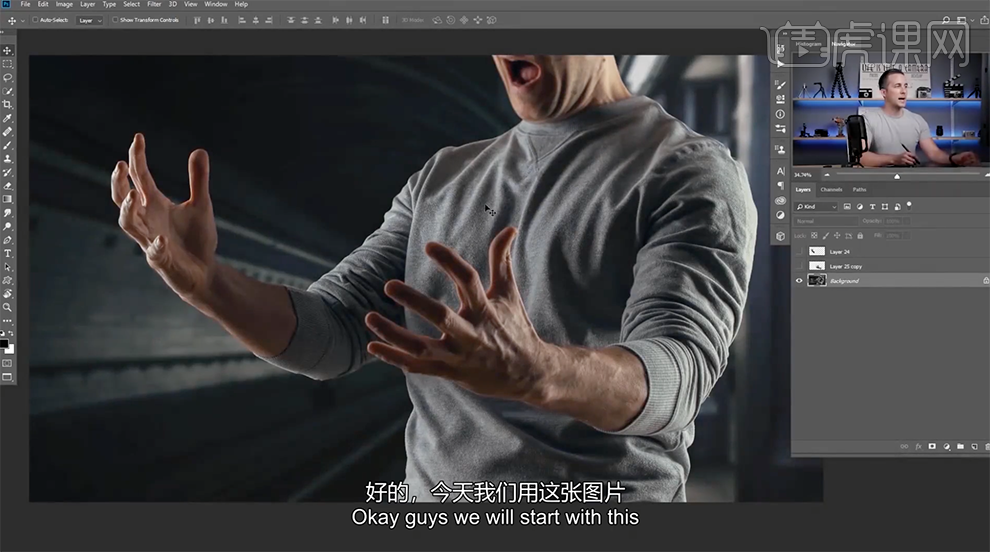
# 1. PHP图片处理基础**
PHP图片处理涉及使用PHP脚本操作和处理图像文件。它提供了一系列函数和类,允许开发人员执行各种图像处理任务,包括格式转换、编辑、优化和分析。
**1.1 图像处理函数**
PHP提供了广泛的图像处理函数,例如`imagecreatefromjpeg()`、`imagecopy()`和`imagefilter()`。这些函数允许开发人员创建、修改和应用效果于图像。
**1.2 图像资源管理**
在PHP中,图像资源表示一个图像文件。开发人员可以使用`imagecreate()`或`imagecreatefromstring()`等函数创建图像资源,并使用`imagedestroy()`销毁它们。
# 2. PHP图片处理进阶技巧
### 2.1 图像格式转换与优化
#### 2.1.1 常用图像格式的特性和转换方法
**特性对比**
| 格式 | 特性 |
|---|---|
| JPEG | 有损压缩,文件大小小,适用于照片和图像 |
| PNG | 无损压缩,文件大小较大,适用于图形和图标 |
| GIF | 无损压缩,支持透明度,适用于动画和简单图像 |
| BMP | 无压缩,文件大小极大,仅用于特殊场合 |
**转换方法**
```php
// 将 JPEG 转换为 PNG
$image = imagecreatefromjpeg('image.jpg');
imagepng($image, 'image.png');
// 将 PNG 转换为 GIF
$image = imagecreatefrompng('image.png');
imagegif($image, 'image.gif');
```
### 2.1.2 图像优化算法和实践
**算法**
| 算法 | 描述 |
|---|---|
| 无损压缩 | 不丢失任何数据,但压缩率较低 |
| 有损压缩 | 丢失部分数据,但压缩率较高 |
| 感知压缩 | 根据人眼视觉特性进行压缩 |
**实践**
- **使用适当的图像格式:**根据图像类型选择合适的格式,如照片使用 JPEG,图标使用 PNG。
- **调整图像质量:**在保存图像时调整质量参数,如 JPEG 的质量范围为 0-100。
- **使用图像优化工具:**如 TinyPNG、ImageOptim 等工具可以进一步优化图像。
### 2.2 图像编辑与特效
#### 2.2.1 图像裁剪、缩放和旋转
```php
// 裁剪图像
$image = imagecreatefromjpeg('image.jpg');
imagecopyresampled($image, $image, 0, 0, 100, 100, 200, 200);
// 缩放图像
$image = imagecreatefromjpeg('image.jpg');
imagecopyresampled($image, $image, 0, 0, 0, 0, 100, 100);
// 旋转图像
$image = imagecreatefromjpeg('image.jpg');
imagerotate($image, 90, 0);
```
#### 2.2.2 图像滤镜、水印和文字添加
```php
// 应用滤镜
$image = imagecreatefromjpeg('image.jpg');
imagefilter($image, IMG_FILTER_GRAYSCALE);
// 添加水印
$image = imagecreatefromjpeg('image.jpg');
$watermark = imagecreatefrompng('watermark.png');
imagecopymerge($image, $watermark, 0, 0, 0, 0, 100, 100, 50);
// 添加文字
$image = imagecreatefromjpeg('image.jpg');
imagettftext($image, 20, 0, 100, 100, 0x000000, 'arial.ttf', 'Hello World');
```
# 3.1 Web应用程序中的图片处理
在Web应用程序中,图片处理是一个常见且至关重要的任务。它涉及各种操作,从验证和处理用户上传的图片到动态生成缩略图和水印。
#### 3.1.1 用户上传图片的验证和处理
当用户上传图片时,Web应用程序需要对图片进行验证,以确保它符合特定标准,例如文件大小、文件类型和内容。验证过程通常包括以下步骤:
```php
// 验证文件大小
if ($_FILES['image']['size'] > 2097152) {
echo '文件大小超过限制';
exit;
}
// 验证文件类型
$allowed_types = array('image/jpeg', 'image/png', 'image/gif');
if (!in_array($_FILES['image']['type'], $allowed_types)) {
echo '文件类型不被允许';
exit;
}
// 验证图片内容
$image_data = getimagesize($_FILES['image']['tmp_name']);
if (!$image_data) {
echo '图片内容无效';
exit;
}
```
验证图片后,应用程序可以将其移动到永久存储位置并对其进行进一步处理,例如调整大小、添加水印或生成缩略图。
#### 3.1.2 动态生成缩略图和水印
缩略图是图片的较小版本,用于在Web页面上快速预览或缩略图画廊中显示。水印是添加到图片上的文本或图像,用于保护版权或识别来源。
在PHP中,可以使用GD库或Imagick扩展来动态生成缩略图和水印。以下示例演示如何使用GD库生成缩略图:
```php
// 创建缩略图
$thumb = imagecreatetruecolor(150, 100);
$source = imagecreatefromjpeg($_FILES['image']['tmp_name']);
imagecopyresampled($thumb, $source, 0, 0, 0, 0, 150, 100, imagesx($source), imagesy($source));
imagejpeg($thumb, 'thumb.jpg');
```
以下示例演示如何使用Imagick扩展添加水印:
```php
// 创建Imagick对象
$image = new Imagick($_FILES['image']['tmp_name']);
// 创建水印对象
$watermark = new Imagick();
$watermark->newImage(100, 100, new ImagickPixel('white'));
$watermark->setImageFormat('png');
// 添加水印
$image->compositeImage($watermark, Imagick::COMPOSITE_OVER, 10, 10);
// 输出水印图片
$image->writeImage('watermarked.jpg');
```
# 4. PHP图片处理高级技术
### 4.1 图像识别与分析
#### 4.1.1 图像特征提取和分类
图像识别是计算机视觉领域的一项重要任务,它涉及从图像中提取特征并将其分类到预定义的类别中。在PHP中,可以使用各种库和算法来实现图像识别。
**特征提取**
特征提取是图像识别过程中的第一步。它涉及从图像中提取有意义的特征,这些特征可以用来区分不同的类别。常用的特征提取方法包括:
- **颜色直方图:**计算图像中不同颜色出现的频率。
- **纹理分析:**分析图像的纹理模式,例如边缘、斑点和纹理。
- **形状描述符:**描述图像的形状,例如周长、面积和凸度。
**分类**
一旦从图像中提取了特征,就可以使用分类算法将其分类到预定义的类别中。常用的分类算法包括:
- **支持向量机 (SVM):**一种线性分类器,可以将数据点分类到不同的类别中。
- **决策树:**一种树状结构,根据一组规则对数据点进行分类。
- **神经网络:**一种受人脑启发的机器学习算法,可以从数据中学习模式并进行分类。
#### 4.1.2 人脸检测和识别
人脸检测和识别是图像识别中的两个特殊应用。
**人脸检测**
人脸检测涉及在图像中找到人脸。它通常使用以下步骤:
1. 将图像转换为灰度。
2. 应用人脸检测算法,例如Haar级联分类器或深度学习模型。
3. 识别图像中的人脸。
**人脸识别**
人脸识别涉及识别图像中的人脸并将其与已知数据库中的面孔进行匹配。它通常使用以下步骤:
1. 提取人脸特征,例如面部特征点、纹理和形状。
2. 将提取的特征与已知数据库中的面孔进行比较。
3. 识别图像中的人脸。
### 4.2 图像合成与增强
#### 4.2.1 图像拼接和全景图生成
图像拼接涉及将多张图像拼接在一起以创建一幅更大的图像。全景图生成是图像拼接的一种特殊应用,它涉及将一系列重叠图像拼接在一起以创建一幅360度的全景图。
**图像拼接**
图像拼接通常使用以下步骤:
1. **图像配准:**将图像对齐以确保它们重叠。
2. **图像融合:**将重叠区域的像素混合在一起以创建平滑的过渡。
3. **图像拼接:**将对齐和融合的图像拼接在一起。
**全景图生成**
全景图生成涉及以下附加步骤:
1. **图像投影:**将图像投影到一个球形表面上。
2. **图像融合:**将投影图像融合在一起以创建平滑的全景图。
#### 4.2.2 图像修复和增强算法
图像修复和增强算法用于改善图像的质量和外观。
**图像修复**
图像修复涉及修复损坏或有缺陷的图像。常见的图像修复算法包括:
- **图像去噪:**去除图像中的噪声。
- **图像修复:**修复图像中的划痕、污点和撕裂。
- **图像复原:**恢复图像的原始外观。
**图像增强**
图像增强涉及改善图像的视觉质量。常见的图像增强算法包括:
- **图像锐化:**增强图像的边缘和细节。
- **图像对比度增强:**增加图像的对比度。
- **图像颜色校正:**调整图像的颜色平衡。
# 5. PHP图片处理最佳实践
### 5.1 性能优化与可扩展性
#### 5.1.1 缓存机制和异步处理
- **缓存机制:**
- 利用缓存机制存储经常访问的图像,减少重复处理和数据库查询。
- 常见的缓存技术包括 Memcached、Redis 和 APC。
- **异步处理:**
- 将图像处理任务分解为多个异步任务,并行执行,提高处理速度。
- 异步处理框架,如 Laravel Queue 或 Symfony Messenger,可以简化异步任务的管理。
#### 5.1.2 分布式处理和云服务利用
- **分布式处理:**
- 将图像处理任务分布到多台服务器上,提高处理能力。
- 使用消息队列或分布式任务管理系统,如 RabbitMQ 或 Celery,协调任务分配。
- **云服务利用:**
- 利用云服务,如 AWS S3 或 Google Cloud Storage,存储和处理图像。
- 云服务提供可扩展的基础设施和按需付费模式,降低成本并提高灵活性。
### 5.2 安全性和隐私保护
#### 5.2.1 图像认证和水印保护
- **图像认证:**
- 使用哈希算法(如 MD5 或 SHA256)对图像进行认证,防止图像篡改。
- 存储图像的哈希值,并定期检查图像的哈希值是否与存储的哈希值匹配。
- **水印保护:**
- 在图像中嵌入不可见的或半透明的水印,以防止未经授权的使用。
- 水印可以包含版权信息或其他标识符,以保护图像的知识产权。
#### 5.2.2 用户隐私和数据保护
- **用户隐私:**
- 遵守隐私法规,如 GDPR,保护用户上传的图像的隐私。
- 仅存储必要的图像数据,并限制对图像的访问。
- **数据保护:**
- 加密存储敏感图像数据,以防止未经授权的访问。
- 定期备份图像数据,以防止数据丢失。
0
0
相关推荐
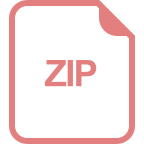
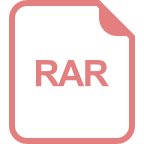
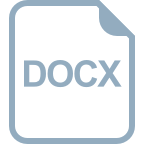
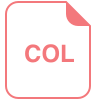
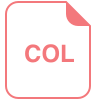
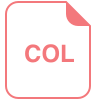
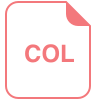

