YOLO算法在农业领域的应用:作物监测与害虫识别,保障农业生产
发布时间: 2024-08-17 18:14:30 阅读量: 116 订阅数: 22 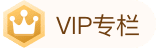
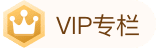
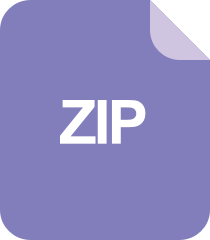
水稻病害基于Yolov8算法优化目标检测识别与AI辅助决策python源码+模型+使用说明.zip
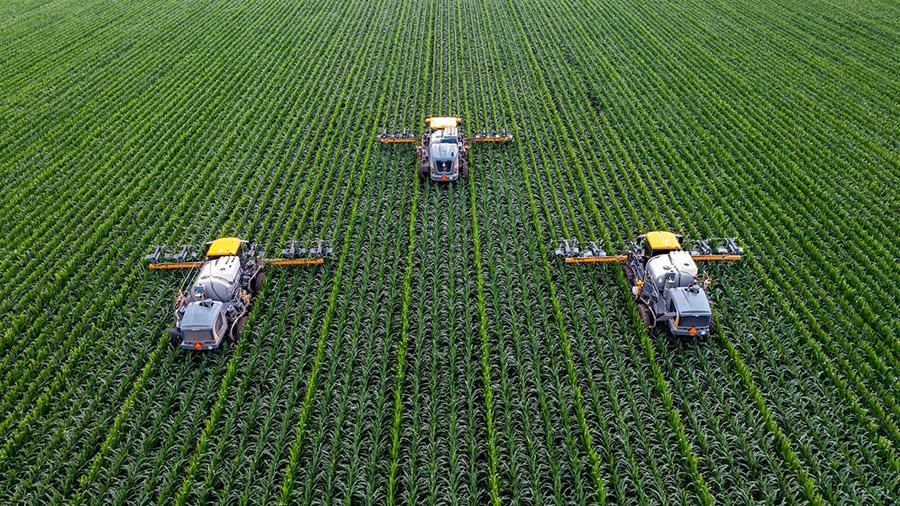
# 1. YOLO算法概述
YOLO(You Only Look Once)算法是一种实时目标检测算法,以其速度快、精度高的特点而闻名。它不同于传统的目标检测算法,后者需要在图像中生成候选区域,然后对每个候选区域进行分类。相反,YOLO算法将目标检测视为一个单一的回归问题,直接预测图像中每个像素的类别和边界框坐标。
YOLO算法的核心思想是将图像划分为一个网格,每个网格负责检测特定区域内的对象。对于每个网格,YOLO算法预测一个条件概率分布,该分布表示该网格中存在对象的可能性以及对象的类别和边界框坐标。通过这种方式,YOLO算法可以一次性检测图像中的所有对象,而无需生成候选区域或进行多次分类。
# 2. YOLO算法在农业领域的理论基础
### 2.1 YOLO算法的原理和特点
YOLO(You Only Look Once)算法是一种单次检测算法,它将目标检测任务转化为回归问题,通过一次前向传播即可获得所有目标的边界框和类别概率。YOLO算法具有以下特点:
- **实时性:**YOLO算法的推理速度非常快,可以达到每秒处理几十到上百帧图像,满足农业领域实时检测的需求。
- **准确性:**YOLO算法在目标检测任务上表现出较高的准确性,可以有效识别和定位目标。
- **鲁棒性:**YOLO算法对图像的尺度、旋转、光照等变化具有较强的鲁棒性,可以在复杂农业环境下稳定工作。
### 2.2 YOLO算法在农业领域的适用性
YOLO算法在农业领域具有广泛的适用性,主要体现在以下几个方面:
- **作物监测:**YOLO算法可以用于作物识别、分类、生长状态监测等任务,帮助农民及时了解作物生长情况,制定科学的管理措施。
- **害虫识别:**YOLO算法可以用于害虫种类识别、数量和分布监测等任务,帮助农民及时发现和控制害虫,减少农业损失。
- **智能温室管理:**YOLO算法可以用于智能温室中的作物管理,通过实时监测作物生长状态,自动调节温室环境,提高作物产量和质量。
- **农田害虫实时监测:**YOLO算法可以用于农田害虫实时监测,通过无人机或地面巡逻机器人搭载摄像头,实时采集图像,并通过YOLO算法进行害虫检测,及时预警害虫发生,指导农民采取防治措施。
**代码块:**
```python
import cv2
import numpy as np
# 加载 YOLO 模型
net = cv2.dnn.readNet("yolov3.weights", "yolov3.cfg")
# 设置输入图像大小
input_width = 416
input_height = 416
# 加载图像
image = cv2.imread("image.jpg")
# 预处理图像
image = cv2.resize(image, (input_width, input_height))
image = image / 255.0
# 将图像输入模型
blob = cv2.dnn.blobFromImage(image, 1 / 255.0, (input_width, input_height), (0, 0, 0), swapRB=True, crop=False)
net.setInput(blob)
# 前向传播
detections = net.forward()
# 解析检测结果
for detection in detections:
# 获取边界框坐标
x, y, w, h = detection[2:6] * np.array([image.shape[1], image.shape[0], image.shape[1], image.shape[0]])
# 获取类别概率
scores = detection[5:]
class_id = np.argmax(scores)
confidence = scores[class_id]
# 绘制边界框和标签
if confidence > 0.5:
cv2.rectangle(image, (int(x - w / 2), int(y - h / 2)), (int(x + w / 2), int(y + h / 2)), (0, 255, 0), 2)
cv2.putText(image, f"{class_id} {confidence:.2f}", (int(x), int(y - 10)), cv2.FONT_HERSHEY_SIMPLEX, 0.5, (0, 255, 0), 2)
# 显示检测结果
cv2.imshow("Detection Result", image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
**代码逻辑分析:**
1. 加载 YOLO 模型并设置输入图像大小。
2. 加载图像并进行预处理。
3. 将图像输入模型并进行前向传播。
4. 解析检测结果,包括边界框坐标和类别概率。
5. 绘制边界框和标签,并显示检测结果。
**参数说明:**
- `input_width` 和 `input_height`:输入图像的大小。
- `image`:输入图像。
- `net`:YOLO 模型。
- `blob`:输入模型的图像数据。
- `detections`:检测结果。
- `x`, `y`, `w`, `h`:边界框坐标。
- `scores`:类别概率。
- `class_id`:类别 ID。
- `confidence`:置信度。
# 3. YOLO算法在农业领域的实践应用
### 3.1 作物监测
#### 3.1.1 作物识别和分类
YOLO算法在作物识别和分类方面表现出色,可快速准确地识别不同作物种类,如小麦、水稻、玉米等。其原理是利用卷积神经网络(CNN)提取作物图像的特征,并通过全连接层进行分类。
```python
import cv2
import numpy as np
# 加载 YOLO 模型
net = cv2.dnn.readNet("yolov3.weights", "yolov3.cfg")
# 加载作物图像
image = cv2.imread("crop.jpg")
# 预处理图像
blob = cv2.dnn.blobFromImage(image, 1 / 255.0, (416, 416), (0, 0, 0), swapRB=True, crop=False)
# 将图像输入模型
net.setInput(blob)
# 前向传播
detections = net.forward()
# 解析检测结果
for detection in detections:
# 获取检测框和类别
box = detection[0:4] * np.array([image.shape[1], image.shape[0], image.shape[1], image.shape[0]])
class_id = int(detection[5])
confidence = detection[2]
# 绘制检测框和标签
cv2.rectangle(image, (int(box[0]), int(box[1])), (int(box[2]), int(box[3])), (0, 255, 0), 2)
cv2.putText(image, f"{class_id}", (int(box[0]), int(box[1] - 10)), cv2.FONT_HERSHEY_SIMPLEX, 0.5, (0, 255, 0), 2)
# 显示结果图像
cv2.imshow("Crop Recognition", image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
**代码逻辑分析:**
* `cv2.dnn.readNet`函数加载 YOLO 模型。
* `cv2.dnn.blobFromImage`函数对图像进行预处理,将其转换为模型输入格式。
* `net.setInput`函数将预处理后的图像输入模型。
* `net.forward`函数进行前向传播,生成检测结果。
* 循环遍历检测结果,获取检测框、类别和置信度。
* 根据检测结果绘制检测框和标签。
#### 3.1.2 作物生长状态监测
YOLO算法还可以用于监测作物生长状态,如叶面积指数(LAI)、冠层高度等。其原理是利用图像分割技术提取作物区域,并通过特征分析评估作物生长状况。
```python
import cv2
import numpy as np
# 加载 YOLO 模型
net = cv2.dnn.readNet("yolov3.weights", "yolov3.cfg")
# 加载作物图像
image = cv2.imread("crop.jpg")
# 预处理图像
blob = cv2.dnn.blobFromImage(image, 1 / 255.0, (416, 416), (0, 0, 0), swapRB=True, crop=False)
# 将图像输入模型
net.setInput(blob)
# 前向传播
detections = net.forward()
# 解析检测结果
for detection in de
```
0
0
相关推荐
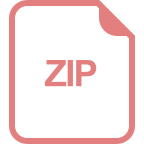
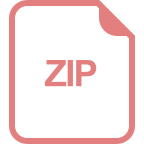
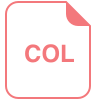
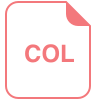
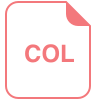
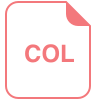
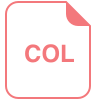
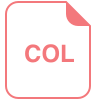