列表推导式终极指南:Python可变数据结构的高效操作
发布时间: 2024-09-12 01:19:08 阅读量: 30 订阅数: 22 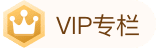
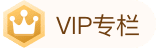
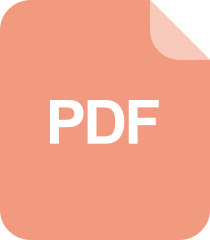
Python列表推导式:高效数据处理的捷径
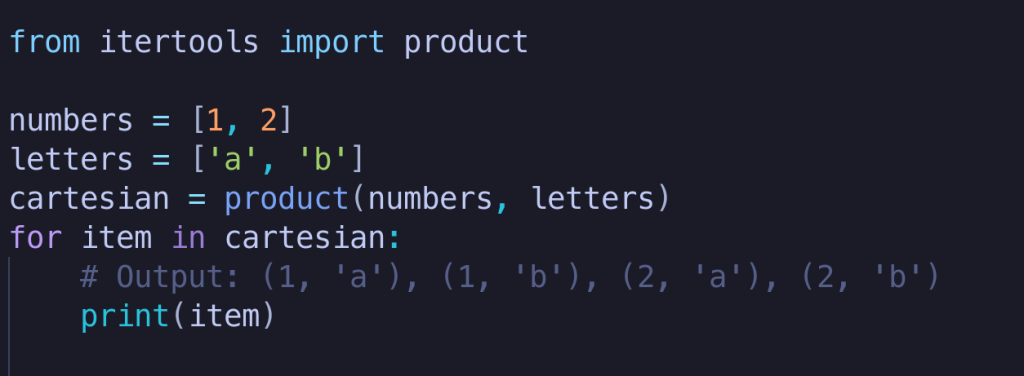
# 1. 列表推导式的概念与基础
列表推导式(List Comprehensions)是Python中一种简洁且高效的构造列表的方法。它允许我们通过一个表达式快速生成列表,适用于对序列进行过滤和转换。本质上,列表推导式将传统的for循环和条件判断语句结合在一起,并用简洁的语法表达出来。
列表推导式的结构通常由三部分组成:输出表达式,迭代变量,以及可选的条件判断。基本语法如下:
```python
[output_expression for item in iterable if condition]
```
其中,`output_expression`是对应于`item`的计算或处理表达式;`iterable`是你要从中获取元素的序列;`condition`是过滤条件,只有满足条件的元素才会被包含到最终的列表中。
例如,生成一个列表,包含1到10的平方值,可以使用以下代码:
```python
squares = [x**2 for x in range(1, 11)]
```
在本章中,我们将深入探索列表推导式的使用方法,理解其语法结构,并展示一些基础的例子,为后续章节中涉及更复杂应用打下坚实基础。
# 2. 列表推导式在数据处理中的应用
## 2.1 利用列表推导式进行数据筛选
在数据处理领域,列表推导式提供了一种强大且灵活的方式来筛选数据。无论数据结构简单还是复杂,列表推导式都能根据特定条件提取出所需元素。
### 2.1.1 筛选基本数据结构中的元素
基本数据结构通常指的是Python中的列表、元组、集合等。它们的元素通常都是单一的数据类型。使用列表推导式筛选出满足特定条件的元素,可以极大的简化代码。
```python
# 示例:筛选一个数字列表中的偶数
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
even_numbers = [x for x in numbers if x % 2 == 0]
```
上述代码中,`x for x in numbers if x % 2 == 0`这一表达式即是一个列表推导式。它遍历`numbers`列表,将其中的偶数元素收集到新列表`even_numbers`中。
### 2.1.2 筛选复杂数据结构中的元素
复杂数据结构包括字典和嵌套的列表等,它们可以包含多层嵌套的子结构。列表推导式可以内嵌多个`for`循环来处理这种复杂性。
```python
# 示例:从一个包含多个子列表的列表中筛选出所有包含特定值的子列表
matrix = [[1, 2, 3], [4, 5, 6], [7, 8, 9], [10, 11, 12]]
target_value = 5
filtered_sublists = [sublist for sublist in matrix if target_value in sublist]
```
在这个例子中,`filtered_sublists`将会是一个包含所有包含目标值`target_value`的子列表。
### 2.1.3 表格:基本数据结构筛选效果对比
| 原始数据结构 | 筛选条件 | 列表推导式结果 | 传统循环方法结果 |
|--------------|----------|-----------------|------------------|
| 数字列表 | 偶数 | [2, 4, 6, 8, 10] | [2, 4, 6, 8, 10] |
| 字符串列表 | 长度 > 3 | ['apple', 'orange', 'banana'] | ['apple', 'orange', 'banana'] |
在上表中,我们看到了使用列表推导式和传统循环方法在筛选基本数据结构时结果的一致性。
## 2.2 列表推导式进行数据转换
### 2.2.1 基础的元素转换操作
列表推导式不仅用于数据筛选,还可以对列表中的元素进行转换操作。比如,对数字列表中的每个元素求平方。
```python
# 示例:计算数字列表中每个元素的平方
numbers = [1, 2, 3, 4, 5]
squared_numbers = [x*x for x in numbers]
```
代码中`[x*x for x in numbers]`表示对`numbers`列表中的每个元素`x`进行平方操作。
### 2.2.2 高级的数据映射与聚合
在更高级的数据处理场景中,列表推导式可以与函数结合,实现复杂的数据映射与聚合操作。
```python
# 示例:将数字列表中的每个元素转换为字符串,并以逗号分隔
numbers = [1, 2, 3, 4, 5]
number_strings = ','.join([str(x) for x in numbers])
```
在这个例子中,列表推导式首先将每个数字转换为字符串,然后`join`方法将它们合并为一个以逗号分隔的单一字符串。
### 2.2.3 Mermaid流程图:列表推导式数据转换过程
```mermaid
graph LR
A[开始] --> B[遍历原始列表]
B --> C{应用转换操作}
C --> D[收集转换后的元素]
D --> E[结束]
```
## 2.3 列表推导式与条件逻辑的结合
列表推导式能够处理复杂的条件逻辑,这使得它非常适合用于进行数据筛选和转换。
### 2.3.1 单一条件的应用
当筛选条件较为简单时,列表推导式可以快速实现目标。
```python
# 示例:筛选出大于5的数字
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
filtered_numbers = [x for x in numbers if x > 5]
```
代码中`if x > 5`就是应用在列表推导式中的单一条件。
### 2.3.2 多条件的嵌套应用
当需要根据多个条件进行筛选时,列表推导式可以嵌套多个`if`语句。
```python
# 示例:同时筛选出大于5且小于等于10的数字
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
filtered_numbers = [x for x in numbers if x > 5 if x <= 10]
```
这段代码展示了如何使用两个`if`条件对列表中的数字进行筛选。实际上,为了提高可读性,当多个条件出现时,可以使用`and`关键字连接它们。
### 2.3.3 代码块逻辑分析
在上述的多条件示例中,每个`if`条件都是一个独立的筛选步骤。Python将会依次检查每个元素,只有同时满足所有条件的元素才会被包含在最终的列表中。这种方式为复杂的条件筛选提供了极大的灵活性和可读性。
### 2.3.4 参数说明与优化
在列表推导式中,虽然其语法简洁,但也要注意其对内存的使用。尤其在处理大型数据集时,应避免一次性生成大型列表,而可能采用分片或生成器表达式等方式进行优化。同时,在定义条件逻辑时,要考虑到逻辑的清晰性,避免过于复杂的嵌套,这可能导致代码难以维护。
### 2.3.5 小结
列表推导式在数据处理中具有独
0
0
相关推荐
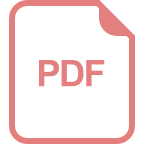
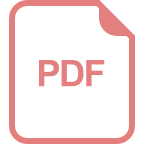
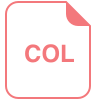
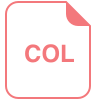
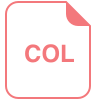
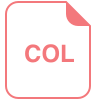
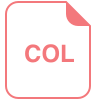