【除法算法的实战攻略】:代码复用、调试技巧与优化策略大揭秘
发布时间: 2024-09-10 08:35:39 阅读量: 149 订阅数: 57 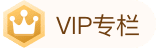
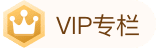
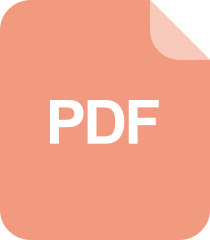
Matlab优化工具箱深度解析:算法概览与代码实战.pdf
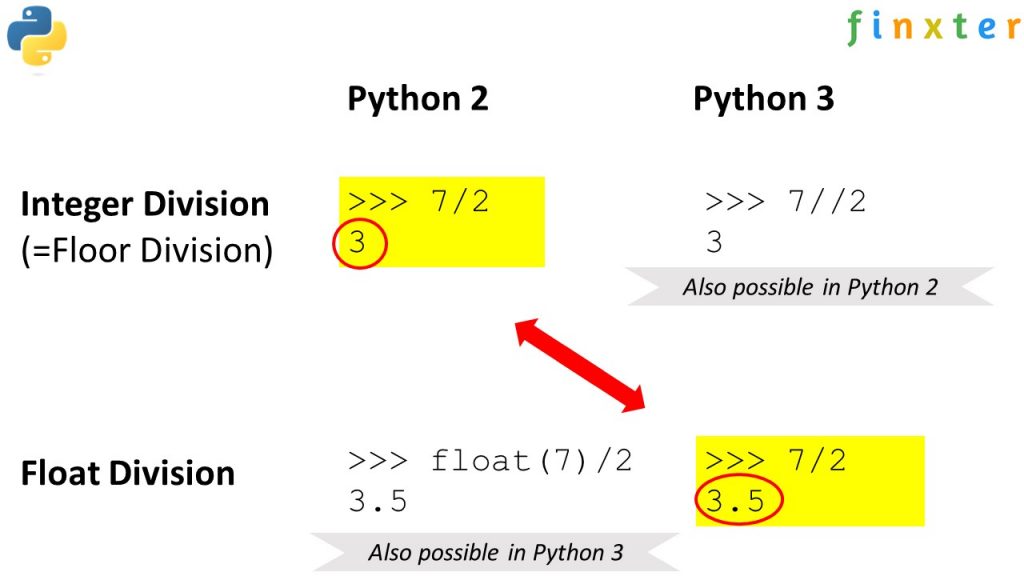
# 1. 除法算法的基础知识回顾
## 算法概念简介
在计算机科学中,算法是解决特定问题的步骤集合,而除法算法是其中最基本的组成部分之一。除法算法通常用于数学运算、数据分析和许多其他计算密集型任务中。理解其基础概念和工作原理对于提高程序效率至关重要。
## 除法的基本数学原理
除法可以视为乘法的逆运算,它将被除数分配成若干个除数大小的部分。在算法层面,整数除法和浮点数除法有所不同,而这些基本的数学原理是算法实现的核心。
## 除法运算的复杂性
除法运算相比加法和乘法来说更为复杂,尤其是在处理大数除法时,可能涉及到的高精度计算、溢出问题和优化方法都是设计高效算法时必须考虑的因素。这节将为读者梳理一些除法算法的基础知识,为后续章节的深入讨论打下基础。
# 2. 代码复用在除法算法中的应用
代码复用是软件工程中的一个核心概念,它可以提高开发效率,减少维护成本,并且有助于保持代码的一致性和质量。本章节将从代码复用的基本概念出发,深入探讨其在除法算法中的具体应用,包括设计模式的运用以及函数式编程的实现方法。
## 2.1 代码复用的基本概念
### 2.1.1 代码复用的定义与重要性
代码复用指的是在开发新软件时,利用已有的代码段、模块、库或组件,而不是从零开始编写所有的代码。这种方法可以显著提高开发效率,降低错误发生的可能性,并且通过重用经过测试的代码,可以提高最终软件的可靠性。
在除法算法的实现中,代码复用可以采取多种形式,如使用已有的数学库函数、封装通用的计算模块或者继承扩展已有的除法类。
### 2.1.2 除法算法中代码复用的实例分析
假设有一个金融应用需要执行大量的除法运算,我们可以考虑实现一个通用的除法计算器类,然后在应用中重用这个类来完成各种除法操作。下面是一个简单示例:
```python
class DivisionCalculator:
def divide(self, numerator, denominator):
if denominator == 0:
raise ValueError("Denominator cannot be zero")
return numerator / denominator
# 在金融应用中重用DivisionCalculator类
calculator = DivisionCalculator()
result1 = calculator.divide(100, 5)
result2 = calculator.divide(200, 10)
```
在这个例子中,`DivisionCalculator`类被创建后,可以被多次实例化并用于不同的除法运算。这减少了为每次除法运算编写独立代码的需要,实现了代码复用。
## 2.2 设计模式在除法算法中的运用
### 2.2.1 设计模式概述
设计模式是软件开发中常见问题的通用解决方案,它们提供了一种在特定情况下应用最佳实践的方法。在除法算法的实现中,可以使用多种设计模式,例如工厂模式、单例模式或策略模式,来优化代码结构和提高代码的复用性。
### 2.2.2 面向对象的除法算法设计
面向对象编程强调将数据和方法封装在对象中,并通过继承和多态等机制来提高代码复用性。在设计一个除法算法时,可以采用继承的概念,创建一个基本的除法运算类,并通过子类来实现特定的除法算法需求。
```python
class BasicDivision:
def divide(self, numerator, denominator):
return numerator / denominator
class SafeDivision(BasicDivision):
def divide(self, numerator, denominator):
if denominator == 0:
raise ValueError("Denominator cannot be zero")
return super().divide(numerator, denominator)
# 使用继承的子类进行除法运算
safe_divider = SafeDivision()
result = safe_divider.divide(100, 0)
```
在这个例子中,`SafeDivision`类继承自`BasicDivision`,并在其`divide`方法中增加了安全性检查。这样的设计模式使得我们可以轻松地扩展或修改除法算法的行为。
## 2.3 函数式编程与除法算法
### 2.3.1 函数式编程简介
函数式编程是一种编程范式,它强调使用纯函数来构建软件。纯函数指的是没有副作用、输入决定输出的函数,这使得函数式编程代码易于测试和维护,并且更适合并行处理。
在除法算法中,我们可以利用函数式编程的思想,将算法设计为纯函数,这样可以更容易地进行复用。
### 2.3.2 函数式方法实现除法算法
在Python中,我们可以使用`functools`模块中的`reduce`函数来实现一个函数式风格的除法算法。
```python
from functools import reduce
def division(numerator, denominator):
if denominator == 0:
raise ValueError("Denominator cannot be zero")
return reduce(lambda x, y: x / y, [numerator] + [denominator] * (numerator // denominator))
# 使用reduce实现的除法函数
result = division(100, 4)
```
在这个实现中,`division`函数通过`reduce`来重复执行除法操作,模拟了整数除法的行为。这是一个纯函数式的方法,因为它不产生任何副作用,并且对于相同的输入总是产生相同的输出。
通过以上二级章节内容,我们深入地了解了代码复用在除法算法中的应用,涵盖了设计模式、函数式编程等高级概念,并通过代码示例展示了如何将这些概念运用到实际的除法算法实现中。接下来,我们将探索除法算法的调试技巧,以确保算法的准确性和效率。
# 3. 除法算法的调试技巧
## 3.1 调试的基本方法与工具
### 3.1.1 常用的调试工具和方法
在软件开发过程中,调试是一个不可或缺的环节,尤其是对于复杂数学算法如除法算法。有效的调试工具和方法能够帮助开发者快速定位和解决问题,减少程序中的缺陷,提高开发效率。
调试工具分为静态分析和动态调试两大类。静态分析工具不运行程序,通过分析代码来预测可能出现的问题。例如ESLint、Pylint等,可以在代码编写阶段就发现潜在的问题。
动态调试工具则是在程序运行时使用的。常见的动态调试工具有GDB、LLDB等,它们可以在程序执行到特定行时暂停,允许开发者检查程序状态、变量值和调用堆栈等。而集成开发环境(IDE)如IntelliJ IDEA和Visual Studio通常内置了强大的动态调试功能,包括断点、变量观察和步进执行等。
代码调试时,一个关键的步骤是设置断点。断点可以是在代码中特定行,程序运行到该行时自动停止。开发者可以检查程序运行的上下文环境,对变量进行修改和重新执行代码,以便观察不同情况下的行为。
### 3.1.2 除法算法的常见错误与调试
除法算法可能会遇到的常见错误包括除以零的错误、精度问题以及溢出错误。除以零是最直接的错误,往往因为边界条件处理不当而产生。在调试除法算法时,首先需要确保除数不为零。
精度问题则涉及到浮点数计算,由于浮点数无法精确表示所有实数,会导致计算结果与预期有微小差异。调试这类问题需要理解浮点数的内部表示,以及如何使用库函数来控制精度。
溢
0
0
相关推荐







