Java中的IO操作详解与最佳实践
发布时间: 2024-01-02 10:12:45 阅读量: 14 订阅数: 14 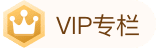
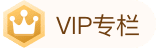
# 第一章:IO操作的基础知识
## 1.1 Java中的IO操作概述
在Java编程中,IO操作是一项非常重要的功能。IO操作主要用于数据的输入和输出,可以从文件、网络、键盘等不同的源获取数据,或将数据发送到文件、网络、屏幕等不同的目的地。
Java提供了丰富的IO类库,包括输入流和输出流两大类。输入流用于从外部获取数据,而输出流用于将数据发送到外部。在具体的使用过程中,需要根据具体需求选择合适的输入流和输出流类。
## 1.2 输入输出流的类型和区别
在Java中,输入流和输出流分别对应了不同的输入和输出操作的类。常见的输入流类包括FileInputStream、BufferedInputStream、InputStreamReader等,而常见的输出流类包括FileOutputStream、BufferedOutputStream、OutputStreamWriter等。
输入流用于读取数据,而输出流用于写入数据。输入流从数据源读取数据,输出流将数据写入目的地。输入流和输出流的作用是相反的。
## 1.3 字符流和字节流的选择
在Java中,IO操作可分为字符流和字节流两种类型。字符流主要用于处理字符数据,而字节流则用于处理二进制数据。
字符流以字符为单位进行读写操作,适用于处理文本文件和文本数据。字符流可以处理字符的Unicode编码,因此在处理中文等特殊字符时非常方便。
字节流则以字节为单位进行读写操作,适用于处理二进制文件和二进制数据。字节流直接读写文件中的字节,适用于处理图像、音频和视频等非文本数据。
在选择字符流和字节流时,需要根据具体的数据类型和处理逻辑来选择合适的流类型。
以上就是Java中IO操作的基础知识,下面将详细介绍Java中不同类型的输入流操作。
## 第二章:Java中的输入流操作
输入流操作主要涉及对外部数据源的读取操作,包括文件输入流、缓冲输入流和字符输入流的使用。
### 2.1 文件输入流(FileInputStream)的使用
文件输入流用于从文件中获取输入字节。可以通过以下示例代码演示文件输入流的基本使用:
```java
import java.io.*;
public class FileInputStreamExample {
public static void main(String[] args) {
try {
FileInputStream fis = new FileInputStream("input.txt");
int data;
while ((data = fis.read()) != -1) {
System.out.print((char) data);
}
fis.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
**代码说明:**
- 使用`FileInputStream`打开名为`input.txt`的文件,并逐字节读取文件内容,直至文件末尾。
- 读取的字节通过`read()`方法返回,并在控制台上以字符形式输出。
**结果说明:**
- 如果`input.txt`中包含文本内容,将被打印到控制台上。
### 2.2 缓冲输入流(BufferedInputStream)的使用
缓冲输入流提供了缓冲功能,能够一次读取一批数据,而不是每次只读取一个字节。
```java
import java.io.*;
public class BufferedInputStreamExample {
public static void main(String[] args) {
try {
BufferedInputStream bis = new BufferedInputStream(new FileInputStream("input.txt"));
int data;
while ((data = bis.read()) != -1) {
System.out.print((char) data);
}
bis.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
**代码说明:**
- 使用`BufferedInputStream`包装`FileInputStream`,实现了对文件内容的缓冲读取。
- 通过`read()`方法逐字节读取文件内容,并以字符形式输出。
**结果说明:**
- 与直接使用`FileInputStream`不同的是,`BufferedInputStream`在读取和输出文件内容时会进行缓冲,提高了读取效率。
### 2.3 字符输入流(InputStreamReader)的使用
字符输入流可以直接读取字符,而不是字节,并且支持指定字符集。
```java
import java.io.*;
public class InputStreamReaderExample {
public static void main(String[] args) {
try {
InputStreamReader reader = new InputStreamReader(new FileInputStream("input.txt"), "UTF-8");
int data;
while ((data = reader.read()) != -1) {
System.out.print((char) data);
}
reader.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
**代码说明:**
- 使用`InputStreamReader`包装`FileInputStream`,并指定字符集为`UTF-8`,以实现对文件内容的字符流读取。
- 通过`read()`方法逐字符读取文件内容,并以字符形式输出。
**结果说明:**
- 与字节流不同的是,字符流可以直接读取字符,并且能够按指定字符集解码文件内容,适用于文本文件的读取操作。
### 第三章:Java中的输出流操作
在Java中,输出流用于向外部设备写入数据,例如文件、网络连接等。输出流可以帮助我们将数据从程序发送到其他地方。本章将介绍Java中常用的输出流操作,包括文件输出流、缓冲输出流和字符输出流。
#### 3.1 文件输出流(FileOutputStream)的使用
文件输出流用于向文件中写入数据,其使用方式如下:
```java
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
public class FileOutputExample {
public static void main(String[] args) {
String data = "Hello, this is a file output example.";
try {
File file = new File("output.txt");
FileOutputStream fos = new FileOutputStream(file);
fos.write(data.getBytes());
fos.close();
System.out.println("Data has been written to the file.");
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
**代码说明:**
- 首先创建一个File对象,指定要写入数据的文件名。
- 创建FileOutputStream对象,并将File对象作为参数传入。
- 使用write方法将数据写入文件。
- 关闭输出流。
**代码总结:**
通过FileOutputStream将数据写入文件,需要注意在使用完毕后及时关闭输出流,以释放资源。
**结果说明:**
运行该程序后,将在当前目录下生成一个名为output.txt的文件,其中包含了"Hello, this is a file output example."的文本内容。
#### 3.2 缓冲输出流(BufferedOutputStream)的使用
缓冲输出流可以提高写入数据的性能,其使用方式如下:
```java
import java.io.BufferedOutputStream;
import java.io.FileOutputStream;
import java.io.IOException;
public class BufferedOutputExample {
public static void main(String[] args) {
String data = "Hello, this is a buffered output example.";
try {
FileOutputStream fos = new FileOutputStream("output.txt");
BufferedOutputStream bos = new BufferedOutputStream(fos);
bos.write(data.getBytes());
bos.close();
System.out.println("Data has been written to the file using buffered output stream.");
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
**代码说明:**
- 创建FileOutputStream对象并传入文件名。
- 创建BufferedOutputStream对象,将FileOutputStream对象作为参数传入。
- 使用write方法将数据写入文件。
- 关闭缓冲输出流。
**代码总结:**
通过BufferedOutputStream将数据写入文件时,可以提高写入性能,尤其是对于大量数据的写入操作。
**结果说明:**
运行该程序后,将在当前目录下生成一个名为output.txt的文件,其中包含了"Hello, this is a buffered output example."的文本内容。
#### 3.3 字符输出流(OutputStreamWriter)的使用
字符输出流用于向文件中写入字符数据,其使用方式如下:
```java
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.OutputStreamWriter;
import java.io.Writer;
public class OutputStreamWriterExample {
public static void main(String[] args) {
String data = "Hello, this is an output stream writer example.";
try {
FileOutputStream fos = new FileOutputStream("output.txt");
Writer writer = new OutputStreamWriter(fos, "UTF-8");
writer.write(data);
writer.close();
System.out.println("Data has been written to the file using output stream writer.");
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
**代码说明:**
- 创建FileOutputStream对象并传入文件名。
- 创建OutputStreamWriter对象,将FileOutputStream对象和字符编码格式作为参数传入。
- 使用write方法将字符数据写入文件。
- 关闭输出流。
**代码总结:**
通过OutputStreamWriter将字符数据写入文件时,可以指定字符编码格式,确保写入的字符数据按照指定编码格式进行写入。
**结果说明:**
运行该程序后,将在当前目录下生成一个名为output.txt的文件,其中包含了"Hello, this is an output stream writer example."的文本内容。
以上是Java中的输出流操作的示例代码和解释。在实际编程中,根据具体场景和性能要求,选择合适的输出流进行数据写入操作。
### 第四章:Java中的字符流操作
#### 4.1 字符流的概念和特点
字符流是用来处理字符数据的IO流,以字符为单位进行读写操作。与字节流不同的是,字符流可以直接读写字符,而不需要先将字符转换成字节。字符流通常用于处理文本文件,能够更方便地读写文本数据。
#### 4.2 字符流的读写操作
Java中常用的字符流类包括FileReader和FileWriter,它们可以方便地对文本文件进行读写操作。下面是一个示例代码,演示了如何使用FileReader读取文本文件中的内容,并使用FileWriter向文件中写入内容。
```java
import java.io.FileReader;
import java.io.FileWriter;
import java.io.IOException;
public class CharacterStreamExample {
public static void main(String[] args) {
try {
// 使用FileReader读取文件内容
FileReader reader = new FileReader("input.txt");
int data;
while ((data = reader.read()) != -1) {
System.out.print((char) data);
}
reader.close();
// 使用FileWriter向文件中写入内容
FileWriter writer = new FileWriter("output.txt");
writer.write("Hello, this is a test.");
writer.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
**代码总结:** 上面的示例代码演示了如何使用字符流进行文件的读取和写入操作。通过FileReader读取文件内容,并通过FileWriter向文件中写入内容。
**结果说明:** 通过FileReader读取的文件内容会被打印输出,同时使用FileWriter向文件中写入了一句话"Hello, this is a test."。
#### 4.3 字符编码和解码
在字符流操作中,字符编码和解码是非常重要的概念。Java中的字符流与字节流相比,更容易处理字符编码的转换,能够方便地读写不同字符编码的文本文件。常用的字符编码包括UTF-8、GBK等,Java提供了相应的编解码类来处理不同字符编码的文件操作。
### 第五章:Java中的字节流操作
在Java中,字节流是以字节为单位进行输入输出的。字节流主要用于处理二进制数据,如图像、音频、视频等文件。下面我们将详细介绍Java中的字节流操作。
#### 5.1 字节流的概念和特点
- 字节流以字节为单位进行读写操作,适用于处理二进制数据。
- 字节流通常通过InputStream和OutputStream类以及它们的子类来实现。
#### 5.2 字节流的读写操作
下面是一个使用字节流读取文件的示例代码:
```java
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
public class ByteStreamExample {
public static void main(String[] args) {
try (FileInputStream fis = new FileInputStream("input.bin");
FileOutputStream fos = new FileOutputStream("output.bin")) {
int data;
while ((data = fis.read()) != -1) {
fos.write(data);
}
} catch (IOException e) {
System.out.println("IOException: " + e.getMessage());
}
}
}
```
代码说明:
- 使用FileInputStream读取文件,并使用FileOutputStream写入文件。
- 通过循环逐个字节读取并写入文件,直到文件结束。
- 使用try-with-resources语句自动关闭资源,在发生异常时也会自动关闭资源。
#### 5.3 字节流的转换操作
在Java中,可以使用InputStreamReader和OutputStreamWriter类来进行字节流和字符流之间的转换。下面是一个示例代码:
```java
import java.io.*;
public class ByteToCharStreamExample {
public static void main(String[] args) {
try (FileInputStream fis = new FileInputStream("input.txt");
InputStreamReader isr = new InputStreamReader(fis, "UTF-8");
FileOutputStream fos = new FileOutputStream("output.txt");
OutputStreamWriter osw = new OutputStreamWriter(fos, "UTF-8")) {
int data;
while ((data = isr.read()) != -1) {
osw.write(data);
}
} catch (IOException e) {
System.out.println("IOException: " + e.getMessage());
}
}
}
```
代码说明:
- 使用InputStreamReader将字节流转换为字符流,并指定字符编码为UTF-8。
- 使用OutputStreamWriter将字符流转换为字节流,并指定字符编码为UTF-8。
- 通过循环逐个字符读取并写入文件,直到文件结束。
- 使用try-with-resources语句自动关闭资源,在发生异常时也会自动关闭资源。
通过本章的学习,我们详细了解了Java中的字节流操作及转换操作,掌握了字节流的读写方法以及字节流与字符流之间的转换技巧。
### 第六章:Java中的IO操作最佳实践
在Java中进行IO操作时,我们可以采用一些最佳实践来提高代码的可读性和性能。本章将介绍一些常用的IO最佳实践。
#### 6.1 使用try-with-resources语句自动关闭资源
在进行IO操作时,为了避免资源泄漏,我们通常需要手动关闭打开的文件流或网络连接等资源。为了简化代码,我们可以使用try-with-resources语句来自动关闭资源。
例如,使用try-with-resources语句关闭一个文件输入流:
```java
try (FileInputStream fis = new FileInputStream("example.txt")) {
// IO操作代码
} catch (IOException e) {
e.printStackTrace();
}
```
使用try-with-resources语句可以省去手动关闭资源的繁琐操作。当try块执行完毕或发生异常时,Java会自动关闭try括号中声明的资源。这样可以确保资源及时释放,避免资源泄漏。
#### 6.2 缓冲流的使用注意事项
在进行IO操作时,使用缓冲流(如BufferedInputStream和BufferedOutputStream)可以提高IO的效率。然而,在使用缓冲流时,需要注意一些事项。
1. 在创建缓冲流之前,要先创建底层的输入流或输出流。
```java
// 创建底层的文件输入流
FileInputStream fis = new FileInputStream("example.txt");
// 将文件输入流包装成缓冲输入流
BufferedInputStream bis = new BufferedInputStream(fis);
```
2. 在数据写入缓冲区后,要记得调用flush()方法刷新缓冲区,确保数据被写入到目标设备中。
```java
BufferedOutputStream bos = new BufferedOutputStream(new FileOutputStream("example.txt"));
bos.write("Hello World".getBytes());
bos.flush(); // 刷新缓冲区,确保数据被写入到文件中
```
3. 在使用完缓冲流后,要调用close()方法关闭流,以确保缓冲区中的数据被刷新到目标设备并释放资源。
```java
try (BufferedInputStream bis = new BufferedInputStream(new FileInputStream("example.txt"))) {
// IO操作代码
} catch (IOException e) {
e.printStackTrace();
}
```
#### 6.3 使用NIO进行高效的IO操作
Java的NIO(New Input/Output)提供了一套基于Channel和Buffer的高性能IO框架。相比传统的IO操作,NIO可以更高效地处理大量的并发IO请求。
使用NIO进行文件读取的示例代码如下:
```java
try (FileChannel channel = FileChannel.open(Paths.get("example.txt"), StandardOpenOption.READ)) {
ByteBuffer buffer = ByteBuffer.allocate(1024);
int bytesRead = channel.read(buffer);
while (bytesRead != -1) {
buffer.flip();
while (buffer.hasRemaining()) {
System.out.print((char) buffer.get());
}
buffer.clear();
bytesRead = channel.read(buffer);
}
} catch (IOException e) {
e.printStackTrace();
}
```
使用NIO时,我们可以使用Channel来代替传统的InputStream和OutputStream,使用Buffer来代替传统的数组,从而更高效地进行IO操作。
总结:
在Java中进行IO操作时,我们可以采用一些最佳实践来提高代码的可读性和性能。使用try-with-resources语句可以自动关闭资源,使用缓冲流可以提高IO效率,使用NIO可以实现高效的IO操作。通过合理地使用这些技巧,可以编写出更高质量的IO代码。
以上是第六章的内容,介绍了Java中的IO操作最佳实践,包括使用try-with-resources语句自动关闭资源、缓冲流的使用注意事项以及使用NIO进行高效的IO操作。这些最佳实践可以帮助开发者编写更加高效和可靠的IO代码。
0
0
相关推荐
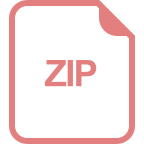
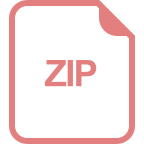





