Java并发集合框架深度剖析:ConcurrentHashMap与CopyOnWriteArrayList的高效使用
发布时间: 2024-08-29 15:37:33 阅读量: 29 订阅数: 30 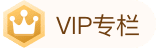
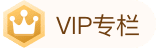
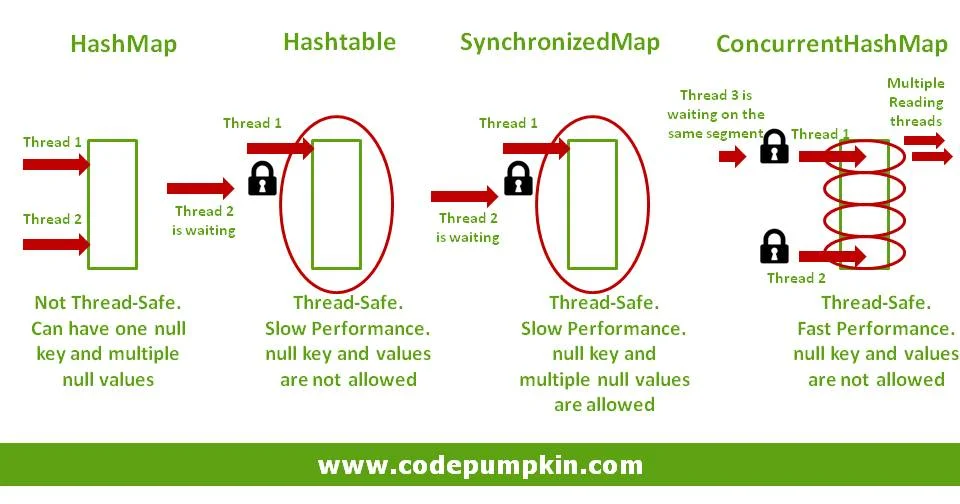
# 1. Java并发集合框架概述
在现代软件开发中,随着多核处理器和高并发系统的普及,对并发集合的需求日益增加。Java并发集合框架应运而生,它提供了一系列线程安全的集合实现,这些集合允许在多线程环境下进行高效的并发操作,同时保证线程安全。Java并发集合框架不仅减少了多线程编程的复杂性,而且通过优化数据结构和并发策略,显著提升了性能。
## 并发集合框架的核心组件
Java并发集合框架的核心组件包括`ConcurrentHashMap`、`CopyOnWriteArrayList`和`ConcurrentSkipListMap`等。它们各自解决了不同的并发问题,并为特定的并发场景提供了高效的解决方案。例如,`ConcurrentHashMap`以其分段锁机制提供了极高的并发读写性能,而`CopyOnWriteArrayList`则为读多写少的环境提供了快速一致的迭代体验。
## 应用并发集合的必要性
在构建可扩展的高并发应用时,正确使用并发集合至关重要。不当的并发集合使用可能导致线程安全问题,如数据不一致、死锁或性能瓶颈。因此,理解和掌握Java并发集合框架是提升并发编程能力的关键。本章将为读者提供并发集合框架的入门知识,为进一步深入学习打下坚实基础。
# 2. 深入理解ConcurrentHashMap
### ConcurrentHashMap的工作原理
#### 分段锁机制与同步映射
ConcurrentHashMap是Java.util.concurrent包中的重要成员,它是一个线程安全的哈希表,在多线程环境下,相比传统的HashMap提供了更优的并发性能。其核心设计理念是通过分段锁机制,对不同的数据段分别进行加锁,以此来减少锁竞争,提升并发度。
分段锁机制,即通过把整个映射分为若干个段(Segment),每个段独立加锁,当一个线程占用锁访问一个Segment时,其他段的访问可以不受影响。这种方式极大地提高了并发性。
```java
ConcurrentHashMap<String, Integer> map = new ConcurrentHashMap<>();
map.put("key", 1);
map.get("key");
```
这段代码中,`put`和`get`操作是线程安全的。ConcurrentHashMap内部通过CAS(Compare-And-Swap)操作,以及一些特殊的设计,比如volatile关键字的使用,确保了操作的原子性。
#### put和get操作的线程安全机制
`put`操作在添加键值对时,首先通过键的哈希值计算得到它所在的段,然后只对该段加锁,操作完成后释放锁。对于`get`操作,通常情况下可以直接通过volatile变量的可见性来获取数据,不需要加锁。
对于冲突比较激烈的哈希码,ConcurrentHashMap会通过链表和红黑树的结构来提高操作的效率。当链表长度超过阈值后,会转化为红黑树以减少搜索时间。
```java
public V put(K key, V value) {
return segmentFor(key).put(key, value, false);
}
public V get(Object key) {
int hash = hash(key.hashCode());
return segmentFor(hash).get(key, hash);
}
```
以上代码展示了ConcurrentHashMap如何通过分段锁的机制来保证`put`和`get`操作的线程安全。每个操作首先定位到对应的Segment,然后由Segment内部的方法来完成操作。
### ConcurrentHashMap的高级特性
#### 弱一致性迭代器
ConcurrentHashMap的一个显著特点是,它不保证映射的迭代器具有强一致性,而是提供弱一致性保证,这意味着迭代器可能不会在迭代过程中反映出映射的最新修改。这种设计在多个线程修改集合时,允许迭代器快速跳过未处理的更新,减少迭代器操作的延迟。
```java
Iterator<Integer> iterator = map.values().iterator();
while (iterator.hasNext()) {
Integer value = iterator.next();
System.out.println(value);
}
```
迭代器的这种设计意味着在并发环境下使用时,需要理解并接受其弱一致性的行为。在高并发系统中,这一点尤为重要,因为可能会读到脏数据。
#### 并发度的调整与优化
ConcurrentHashMap允许通过构造函数或者方法`concurrencyLevel`来设置其并发级别(concurrencyLevel),这个值指的是能够独立进行并发写操作的段(Segment)的数量。合理设置并发级别可以有效提高并发性能。
```java
ConcurrentHashMap<String, Integer> map = new ConcurrentHashMap<>(16, 0.75f, 2);
```
在上述代码中,我们创建了一个并发级别为2的ConcurrentHashMap实例。理论上,这将允许2个线程同时进行写操作而不会互相干扰。
### 实践中的ConcurrentHashMap
#### 高效读写操作的案例分析
在高并发读写场景下,例如一个用于缓存热点数据的系统,ConcurrentHashMap可以提供高效的线程安全支持。例如在缓存系统中,我们可能会大量地进行`get`和`put`操作。
```java
ConcurrentHashMap<String, String> cache = new ConcurrentHashMap<>();
cache.put("key1", "value1");
String value = cache.get("key1");
```
在上述代码中,缓存操作可以非常高效,因为读操作几乎不需要加锁,而写操作只对数据段加锁,这大大提升了性能。
#### 性能测试与调优策略
为了确保系统性能,对于使用的ConcurrentHashMap,应当进行性能测试,观察在特定的并发级别下,读写操作的效率。根据测试结果进行调优,比如修改初始容量、加载因子或并发级别。
```java
void testConcurrentHashMapPerformance() {
// 测试代码,假设并发执行多次put和get操作
// 捕获并分析执行时间,CPU和内存使用情况
}
```
在性能测试过程中,应该考虑到不同并发级别下的读写性能,以及在不同系统负载下的表现。调优策略应当基于实际的测试结果来决定,以达到最佳的性能表现。
在下一章节中,我们将探讨另一个并发集合CopyOnWriteArrayList,看看它在并发环境下是如何提供线程安全的迭代与修改操作的。
# 3. 深入探究CopyOnWriteArrayList
## 3.1 CopyOnWriteArrayList的工作机制
### 3.1.1 写时复制(Copy-On-Write)技术解析
CopyOnWriteArrayList是Java中一种线程安全的List集合,其核心机制是“写时复制”,在每次修改集合时,都会创建并复制底层数组的副本,在副本上进行修改,然后将原集合引用指向这个修改后的副本。这种方法确保了读操作不会被写操作阻塞,从而提高了并发读取的性能。
Copy-On-Write 的关键优势在于:
- 读操作可以无锁,因为它们总是在当前快照上执行。
- 写操作成本较高,因为需要复制底层数组,但因为写操作通常较少,因此整体性能影响较小。
下面是实现写时复制的代码示例:
```java
public class CopyOnWriteArrayList<E> {
private transient volatile Object[] array;
public CopyOnWriteArrayList() {
array = new Object[0];
}
public void add(E e) {
final ReentrantLock lock = this.lock;
lock.lock();
try {
Object[] elements = getArray();
int len = elements.length;
Object[] newElements = Arrays.copyOf(elements, len + 1);
newElements[len] = e;
setArray(newElements);
} finally {
lock.unlock();
}
}
// ... 其他方法 ...
}
```
在`add`方法中,我们首先通过锁保证写操作的互斥性,然后复制底层数组,并在副本上添加新元素,最后用新数组替换旧数组。使用`finally`确保锁总是被释放。
###
0
0
相关推荐
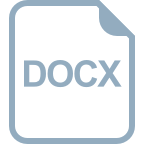
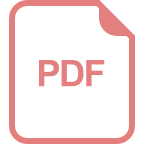
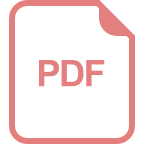
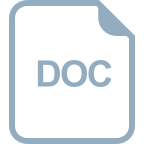
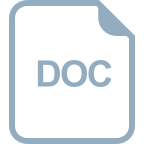
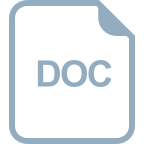
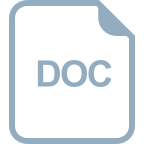
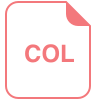
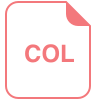