Java泛型类型参数化:设计原则与实践详解
发布时间: 2024-09-11 05:25:15 阅读量: 74 订阅数: 46 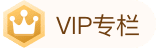
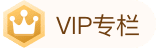

# 1. Java泛型概述
## 1.1 泛型的引入背景
Java泛型是在JDK 5版本中引入的一个重要的语言特性。泛型的引入主要是为了解决类型安全问题,以及减少强制类型转换带来的错误和代码的可读性。在没有泛型之前,Java的集合框架(如List和Map)会把它们的元素当做Object类型处理,这需要程序员在使用时对对象进行显式的类型转换。
## 1.2 泛型的定义和好处
泛型可以简单理解为“参数化类型”,允许在定义类、接口和方法时使用类型参数,这些类型参数在使用时会被具体化。泛型的好处主要体现在:
- **类型安全**:泛型确保了在编译时进行类型检查,从而避免了类型转换异常。
- **减少类型转换**:通过泛型,可以在编译时自动推断和确认类型,减少了运行时的类型转换。
- **代码复用**:泛型方法和类可以在多种不同数据类型上复用,无需重复编写相似代码。
例如,`List<Integer>`可以声明一个只能包含整数的列表,这使得代码在编译阶段就保证了列表中的元素类型一致,无需在运行时进行类型检查和转换。
泛型的引入极大地提高了Java代码的安全性和可读性,并且通过类型参数化,为Java的集合框架带来了质的飞跃。在接下来的章节中,我们将进一步探讨泛型的设计原则、高级用法以及其在集合框架中的应用,深入理解Java泛型的真正力量。
# 2. 泛型设计原则
## 2.1 泛型的基本概念
### 2.1.1 泛型的定义和目的
在Java中,泛型提供了编译时类型安全检测机制,这种机制允许在编译时检测到非法的类型。泛型的本质是参数化类型,也就是说,所操作的数据类型被指定为一个参数。这种参数在使用时会被具体的类型所替换,即所谓的类型参数化。
泛型的关键优点包括:
1. **类型安全**:泛型允许在编译时提供类型检查,减少了运行时的类型转换错误。
2. **消除强制类型转换**:泛型的使用避免了使用强制类型转换。
3. **代码复用**:通过泛型,可以创建可重用的代码库,因为它们可以适应多种数据类型。
泛型的定义通常在类、接口和方法中使用。例如,我们可以定义一个泛型类`Box<T>`,其中`T`代表类型参数:
```java
public class Box<T> {
private T t;
public void set(T t) {
this.t = t;
}
public T get() {
return t;
}
}
```
在本节中,我们重点介绍类型擦除的概念,即在运行时泛型信息是如何被处理的。
### 2.1.2 类型擦除与泛型数组
Java的泛型是通过类型擦除实现的,这意味着在运行时,泛型信息实际上并不存在。编译器通过类型擦除来移除类型和方法的泛型信息,并在相应的地方插入类型转换。
类型擦除是Java虚拟机(JVM)实现泛型的一种方式。在编译期间,Java编译器会处理所有的泛型信息。编译器会进行类型检查和类型推断,然后擦除类型变量,使用其边界(最常用的边界是类型本身的上界)替换这些变量。这之后,代码被编译成普通的字节码。
例如,编译器在处理上面的`Box<T>`类时,会将其转换成类似下面的非泛型类:
```java
public class Box {
private Object t;
public void set(Object t) {
this.t = t;
}
public Object get() {
return t;
}
}
```
**类型擦除会导致一些限制**,比如无法创建具体类型的泛型数组:
```java
Box<Integer>[] intBoxes = new Box<Integer>[10]; // 会出现编译错误
```
上述代码会导致编译错误,因为擦除之后的类型是`Box[]`,它是一个原始类型的数组。由于Java不支持创建原始类型的泛型数组,这是为了防止在运行时出现类型转换异常。
因此,当需要使用泛型数组时,可以使用`Object`数组或者使用`ArrayList`等集合类。
## 2.2 泛型类与接口
### 2.2.1 定义泛型类和接口
泛型类和接口在Java中扮演着重要的角色,它们能够定义多种数据类型,增强代码的复用性和类型安全。
#### 泛型类定义
泛型类是在类名后用尖括号`< >`括起来的类型参数来定义的。这里是一个简单的泛型类的例子:
```java
public class GenericClass<T> {
private T data;
public GenericClass(T data) {
this.data = data;
}
public T getData() {
return data;
}
}
```
`<T>`定义了一个类型参数,它可以在类的定义中被自由使用。
#### 泛型接口定义
泛型接口的定义与泛型类类似,也可以包含类型参数:
```java
public interface GenericInterface<T> {
void set(T t);
T get();
}
```
在实现泛型接口时,需要提供具体的类型:
```java
public class GenericInterfaceImpl<T> implements GenericInterface<T> {
private T data;
@Override
public void set(T t) {
this.data = t;
}
@Override
public T get() {
return data;
}
}
```
### 2.2.2 泛型类的实例化和使用
泛型类的实例化非常直接。在创建对象时,只需指定具体的类型即可。例如,使用上面定义的`GenericClass`类:
```java
GenericClass<String> stringObject = new GenericClass<>("Hello Generic");
```
如果要创建一个没有具体类型参数的泛型类实例,可以使用通配符`?`:
```java
GenericClass<?> unknownType = new GenericClass<>(new Object());
```
对于泛型接口的实现,创建实例的方式也与普通接口相似,但在实现接口时需要指定类型参数:
```java
GenericInterfaceImpl<Integer> integerObject = new GenericInterfaceImpl<>();
integerObject.set(123);
int value = integerObject.get();
```
## 2.3 泛型方法与构造器
### 2.3.1 泛型方法的定义和规则
泛型方法是定义在类中但操作类型参数的方法。泛型方法可以存在于普通类中,也可以存在于泛型类中。泛型方法拥有自己的类型参数,这些参数在方法声明时指定,与类的类型参数无关。
泛型方法的定义格式如下:
```java
public <T> void.genericMethod(T t) {
// 方法体
}
```
这里的`<T>`是泛型方法的类型参数,它可以与类中定义的泛型参数相同或不同。
泛型方法有几个重要规则:
1. **泛型方法可以定义在任何类中**:无论是泛型类还是非泛型类。
2. **泛型方法可以调用其他泛型方法**:只要在调用时提供必要的类型参数。
3. **泛型方法可以使用类的类型参数**:如果它们是在泛型类中定义的。
### 2.3.2 泛型构造器的使用和限制
泛型构造器是指在构造器声明中定义的类型参数。它们允许在创建对象实例时提供具体的类型参数。
泛型构造器的定义格式如下:
```java
public class GenericConstructor<T> {
private T data;
public GenericConstructor(T data) {
this.data = data;
}
// 泛型构造器
public <U extends Number> GenericConstructor(U u) {
this.data = (T) u;
}
}
```
在这个例子中,`GenericConstructor`类有一个泛型构造器,它接受一个实现了`Number`接口的类型`U`作为参数。
使用泛型构造器时需要注意以下几点:
1. **构造器的类型参数必须在实例化对象时确定**。
2. **类型参数可以使用类的类型
0
0
相关推荐
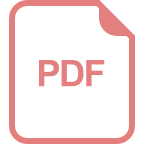
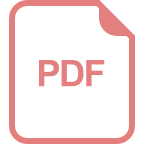
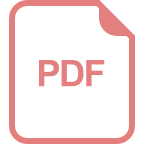





