扩展Spring Batch:自定义任务和步骤
发布时间: 2023-12-17 12:20:07 阅读量: 47 订阅数: 24 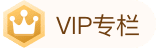
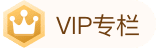
# 1. 简介
## 1.1 Spring Batch概述
Spring Batch是一个轻量级的批处理框架,提供了一套支持大规模、高性能的批量作业处理的工具和API。它可以简化批量作业的开发,并且提供了事务管理、并发控制、错误处理和重试机制等功能。
Spring Batch的核心概念包括Job(作业)、Step(步骤)、ItemReader(读取器)、ItemProcessor(处理器)和ItemWriter(写入器)。使用这些组件,可以将一个复杂的批量作业拆分为多个步骤,并为每个步骤指定读取、处理和写入的逻辑。
## 1.2 扩展Spring Batch的需求
在使用Spring Batch开发批量作业时,可能会遇到一些特殊的业务需求,标准的组件无法满足要求。此时,我们需要扩展Spring Batch,实现自定义任务和步骤,以及定制化的错误处理和重试机制。
本文将介绍如何自定义任务和步骤,并通过示例代码演示Spring Batch的执行器内部机制和错误处理机制。
# 2. 自定义任务
在Spring Batch中,任务是一个可以由JobLauncher运行的Job实例。一个Job可以有一个或多个步骤(Step),每个步骤包含一个或多个任务项(Item)。如果你需要扩展Spring Batch以满足特定需求,你可以自定义任务。
### 2.1 创建新的任务类
要创建一个新的任务类,你需要实现Spring Batch提供的接口。在Java中,你可以创建一个类并实现Job接口,例如:
```java
import org.springframework.batch.core.Job;
import org.springframework.batch.core.JobExecution;
import org.springframework.batch.core.JobParameters;
import org.springframework.batch.core.Step;
import org.springframework.batch.core.StepContribution;
import org.springframework.batch.core.configuration.annotation.JobBuilderFactory;
import org.springframework.batch.core.configuration.annotation.StepBuilderFactory;
import org.springframework.batch.core.launch.JobLauncher;
import org.springframework.batch.core.launch.support.RunIdIncrementer;
import org.springframework.batch.core.scope.context.ChunkContext;
import org.springframework.batch.repeat.RepeatStatus;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
@Configuration
public class CustomJob {
@Autowired
private JobBuilderFactory jobBuilderFactory;
@Autowired
private StepBuilderFactory stepBuilderFactory;
@Autowired
private JobLauncher jobLauncher;
@Bean
public Job customJob() {
return jobBuilderFactory.get("customJob")
.incrementer(new RunIdIncrementer())
.start(customStep())
.build();
}
@Bean
public Step customStep() {
return stepBuilderFactory.get("customStep")
.tasklet((contribution, chunkContext) -> {
System.out.println("Executing custom task...");
return RepeatStatus.FINISHED;
})
.build();
}
}
```
在上面的示例中,我们创建了一个名为`customJob`的Job,并添加了一个名为`customStep`的步骤。在`customStep`步骤中,我们使用了`tasklet`方法来执行自定义的任务逻辑。
### 2.2 配置任务
要使用自定义的任务,你需要在Spring Batch的配置文件中添加相应的配置。例如,在XML配置中,你可以添加如下配置:
```xml
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:batch="http://www.springframework.org/schema/batch"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/batch http://www.springframework.org/schema/batch/spring-batch.xsd">
<batch:job id="customJob" incrementer="runIdIncrementer">
<batch:step id="customStep">
<
```
0
0
相关推荐








