揭秘模糊逻辑系统在决策支持中的作用:案例分析与最佳实践
发布时间: 2024-08-21 12:40:36 阅读量: 47 订阅数: 29 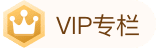
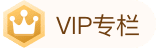
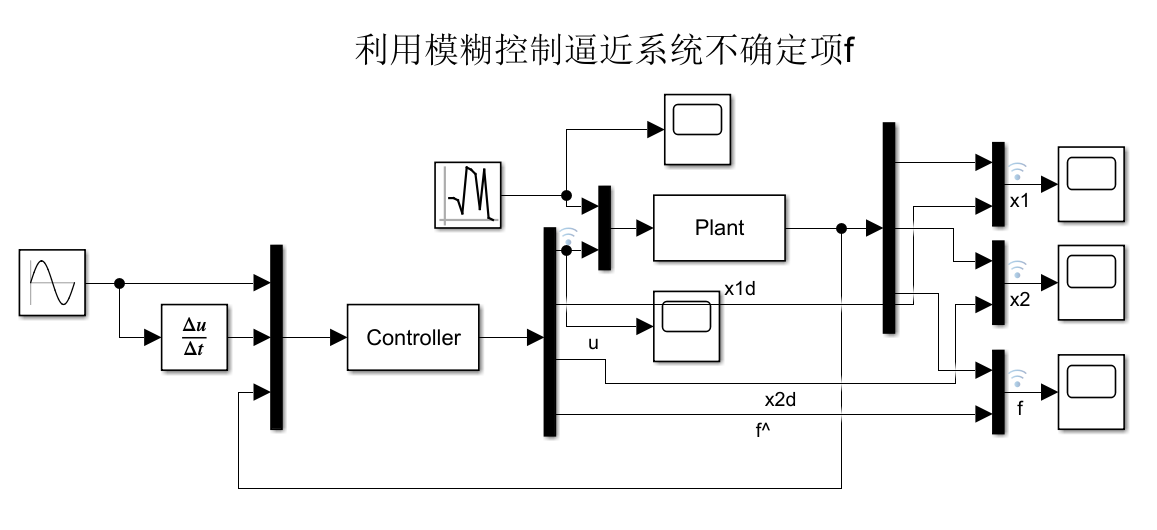
# 1. 模糊逻辑系统概述**
模糊逻辑系统是一种处理不确定性和模糊性的计算模型。它基于模糊集合理论,该理论允许元素具有部分成员资格。模糊逻辑系统由以下组件组成:
- 模糊化器:将输入变量转换为模糊集合。
- 模糊规则库:包含一系列模糊规则,定义了系统如何根据输入做出决策。
- 模糊推理机:使用模糊规则库对输入变量进行推理,产生模糊输出。
- 解模糊化器:将模糊输出转换为清晰输出。
# 2. 模糊逻辑系统在决策支持中的应用
### 2.1 模糊逻辑系统在决策中的优势
模糊逻辑系统在决策支持中具有以下优势:
- **处理不确定性和模糊性:**模糊逻辑系统可以处理不确定性和模糊性,这在现实世界决策中很常见。它允许决策者使用模糊变量和规则来表达他们的知识和经验。
- **模拟人类推理:**模糊逻辑系统使用类似于人类推理的规则和推理机制,使其能够模拟人类决策者的思维过程。
- **鲁棒性和适应性:**模糊逻辑系统对输入数据的变化具有鲁棒性,并且可以随着新信息的出现而适应。
- **可解释性:**模糊逻辑系统的规则和推理过程易于理解和解释,这有助于决策者理解系统的决策。
### 2.2 模糊逻辑系统在决策支持中的实际案例
模糊逻辑系统已成功应用于各种决策支持领域,包括:
- **医疗诊断:**模糊逻辑系统用于诊断疾病,如癌症和心脏病,利用模糊规则将患者症状映射到疾病概率。
- **金融预测:**模糊逻辑系统用于预测股票价格和市场趋势,通过模糊规则整合经济指标和市场情绪。
- **制造业控制:**模糊逻辑系统用于控制制造过程,如机器人操作和过程优化,利用模糊规则将传感器数据映射到控制动作。
- **交通管理:**模糊逻辑系统用于管理交通流量,如信号灯控制和路线规划,利用模糊规则优化交通流和减少拥堵。
**示例:模糊逻辑系统在医疗诊断中的应用**
以下是一个使用模糊逻辑系统进行医疗诊断的示例:
```python
# 导入必要的库
import numpy as np
import skfuzzy as fuzz
# 定义模糊变量
symptoms = ['发烧', '咳嗽', '喉咙痛', '流鼻涕']
disease = ['流感', '普通感冒', '肺炎']
# 定义模糊集
fever = fuzz.trimf(symptoms, [0, 38, 40])
cough = fuzz.trimf(symptoms, [0, 5, 10])
sore_throat = fuzz.trimf(symptoms, [0, 3, 6])
runny_nose = fuzz.trimf(symptoms, [0, 2, 4])
flu = fuzz.trimf(disease, [0, 0.5, 1])
cold = fuzz.trimf(disease, [0, 0.5, 1])
pneumonia = fuzz.trimf(disease, [0, 0.5, 1])
# 定义模糊规则
rules = [
fuzz.Rule(fever['high'] & cough['high'] & sore_throat['high'] & runny_nose['high'], flu['high']),
fuzz.Rule(fever['medium'] & cough['medium'] & sore_throat['medium'] & runny_nose['medium'], cold['high']),
fuzz.Rule(fever['low'] & cough['low'] & sore_throat['low'] & runny_nose['low'], pneumonia['high'])
]
# 输入患者症状
patient_symptoms = [39, 7, 5, 3]
# 模糊化输入
fever_level = fuzz.interp_membership(symptoms, fever, patient_symptoms[0])
cough_level = fuzz.interp_membership(symptoms, cough, patient_symptoms[1])
sore_throat_level = fuzz.interp_membership(symptoms, sore_throat, patient_symptoms[2])
runny_nose_level = fuzz.interp_membership(symptoms, runny_nose, patient_symptoms[3])
# 应用模糊规则
activated_rules = []
for rule in rules:
firing_strength = rule.antecedent.membership_grade(fever_level, cough_level, sore_throat_level, runny_nose_level)
if firing_strength > 0:
activated_rules.append((rule, firing_strength))
# 聚合模糊输出
aggregated_output = np.zeros_like(disease)
for rule, firing_strength in activated_rules:
aggregated_output = np.fmax(aggregated_output, firing_strength * rule.consequent)
# 反模糊化输出
diagnosis = fuzz.defuzz(disease, aggregated_output, 'centroid')
# 打印诊断结果
print(f"诊断结果:{diagnosis}")
```
**代码逻辑分析:**
1. 导入必要的库。
2. 定义模糊变量:症状和疾病。
3. 定义模糊集:使用三角形隶属函数定义症状和疾病的模糊集。
4. 定义模糊规则:使用模糊规则将症状映射到疾病概率。
5. 输入患者症状:获取患者的症状值。
6. 模糊化输入:将患者症状模糊化为模糊变量。
7. 应用模糊规则:根据模糊规则计算每个规则的激发强度。
8. 聚合模糊输出:聚合所有激发规则的模糊输出。
9. 反模糊化输出:将聚合模糊输出反模糊化为疾病概率。
10. 打印诊断结果:输出疾病诊断。
# 3. 模糊逻辑系统设计与实现
### 3.1 模糊逻辑系统的架构和组件
模糊逻辑系统通常由以下组件组成:
- **模糊化器:**将输入变量转换为模糊变量。
- **模糊规则库:**包含模糊规则,这些规则定义了输入变量和输出变量之间的关系。
- **推理引擎:**使用模糊规则和模糊推理方法来推导出输出变量。
- **去模糊化器:**将模糊输出变量转换为清晰输出变量。
### 3.2 模糊逻辑系统的建模与仿真
模糊逻辑系统的建模和仿真是一个迭代过程,涉及以下步骤:
1. **定义输入和输出变量:**确定模糊逻辑系统将处理的输入和输出变量。
2. **创建模糊集合:**为每个输入和输出变量定义模糊集合,这些集合代表变量的不同值。
3. **建立模糊规则库:**根据专家知识或数据分析,制定模糊规则。
4. **选择推理方法:**选择一种模糊推理方法,例如 Mamdani 或 Sugeno 方法。
5. **仿真系统:**使用输入数据仿真模糊逻辑系统,并观察输出。
6. **调整模型:**根据仿真结果,调整模糊集合、规则或推理方法,以提高系统性能。
### 代码块示例
以下 Python 代码展示了如何使用 Mamdani 推理方法实现模糊逻辑系统:
```python
import numpy as np
import skfuzzy as fuzz
# 定义输入变量
input_var = np.arange(0, 101, 1)
# 定义模糊集合
low = fuzz.trimf(input_var, [0, 0, 50])
medium = fuzz.trimf(input_var, [0, 50, 100])
high = fuzz.trimf(input_var, [50, 100, 100])
# 定义输出变量
output_var = np.arange(0, 101, 1)
# 定义模糊集合
low_output = fuzz.trimf(output_var, [0, 0, 50])
medium_output = fuzz.trimf(output_var, [0, 50, 100])
high_output = fuzz.trimf(output_var, [50, 100, 100])
# 定义模糊规则
rules = [
fuzz.Rule(low, low_output),
fuzz.Rule(medium, medium_output),
fuzz.Rule(high, high_output)
]
# 仿真系统
input_value = 75
output = fuzz.centroid(output_var, fuzz.interp_membership(input_var, low, input_value))
print(output)
```
### 逻辑分析和参数说明
- **模糊化器:** `fuzz.trimf()` 函数用于创建三角形模糊集合,它需要三个参数:左边界、峰值和右边界。
- **推理引擎:** Mamdani 推理方法使用最小-最大推理来计算输出隶属度。
- **去模糊化器:** `fuzz.centroid()` 函数用于计算输出变量的质心,它返回一个清晰值。
- **仿真:** `input_value` 是输入变量的值,`output` 是模糊逻辑系统输出的清晰值。
# 4. 模糊逻辑系统优化与评估
### 4.1 模糊逻辑系统的参数优化方法
模糊逻辑系统的性能受其参数的影响,包括模糊集的定义、规则库和推理机制。参数优化旨在调整这些参数以提高系统的性能。常用的优化方法包括:
- **梯度下降法:**一种迭代算法,通过计算性能指标的梯度并沿着梯度方向调整参数来优化系统。
```python
import numpy as np
def gradient_descent(params, learning_rate, num_iterations):
for i in range(num_iterations):
gradient = compute_gradient(params)
params -= learning_rate * gradient
```
- **遗传算法:**一种受进化论启发的算法,通过选择、交叉和变异操作来优化参数。
```python
import random
def genetic_algorithm(params, population_size, num_generations):
population = generate_population(params, population_size)
for i in range(num_generations):
population = select_parents(population)
population = crossover(population)
population = mutate(population)
```
- **粒子群优化:**一种受鸟群行为启发的算法,通过粒子之间的信息共享来优化参数。
```python
import numpy as np
def particle_swarm_optimization(params, num_particles, num_iterations):
particles = generate_particles(params, num_particles)
for i in range(num_iterations):
for particle in particles:
particle.update_velocity()
particle.update_position()
```
### 4.2 模糊逻辑系统的性能评估指标
评估模糊逻辑系统的性能至关重要,以确定其有效性和准确性。常用的评估指标包括:
- **准确率:**预测值与实际值之间的匹配程度。
- **召回率:**系统正确识别正例的比例。
- **F1 分数:**准确率和召回率的加权平均值。
- **均方根误差(RMSE):**预测值与实际值之间的平均平方差。
```python
from sklearn.metrics import accuracy_score, recall_score, f1_score, mean_squared_error
def evaluate_fuzzy_system(y_true, y_pred):
accuracy = accuracy_score(y_true, y_pred)
recall = recall_score(y_true, y_pred)
f1 = f1_score(y_true, y_pred)
rmse = np.sqrt(mean_squared_error(y_true, y_pred))
return accuracy, recall, f1, rmse
```
### 4.3 模糊逻辑系统优化与评估的交互
模糊逻辑系统的优化和评估是相互关联的过程。通过评估系统的性能,可以识别需要优化的参数。优化后,系统性能得到提高,评估指标也相应提高。这种迭代过程有助于创建具有最佳性能的模糊逻辑系统。
```mermaid
graph LR
subgraph 优化
A[参数优化] --> B[性能评估]
end
subgraph 评估
C[性能评估] --> D[优化需求]
end
A --> C
D --> B
```
# 5. 模糊逻辑系统在决策支持中的最佳实践
### 5.1 模糊逻辑系统在决策支持中的应用场景
模糊逻辑系统在决策支持中具有广泛的应用场景,包括但不限于:
- **风险评估:**评估投资、项目或决策的潜在风险,并根据模糊变量(如市场不确定性、竞争强度)做出明智的决定。
- **故障诊断:**分析复杂系统中的故障模式,并根据模糊症状(如振动、噪音)确定可能的故障原因。
- **预测建模:**预测未来事件或趋势,例如销售额、客户流失或经济增长,基于模糊输入(如消费者偏好、市场条件)。
- **决策优化:**优化决策变量,例如资源分配、生产计划或投资组合管理,以最大化目标函数,同时考虑模糊约束(如预算限制、时间限制)。
- **知识管理:**捕获和表示专家知识,以便在决策过程中使用,例如医疗诊断、法律咨询或财务规划。
### 5.2 模糊逻辑系统在决策支持中的实施指南
实施模糊逻辑系统进行决策支持时,应遵循以下指南:
1. **明确决策问题:**定义决策目标、约束和模糊变量。
2. **收集数据:**收集有关模糊变量和决策结果的历史数据或专家意见。
3. **建立模糊逻辑模型:**使用模糊规则和模糊推理机制创建模糊逻辑模型,将模糊输入映射到决策输出。
4. **验证和优化模型:**使用验证数据测试模型的准确性和鲁棒性,并根据需要调整模糊规则和参数。
5. **集成到决策支持系统:**将模糊逻辑模型集成到现有的决策支持系统中,提供模糊推理功能。
6. **持续监控和评估:**定期监控模型的性能,并根据需要进行调整或重新训练,以确保持续的准确性和有效性。
0
0
相关推荐
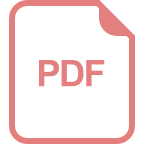
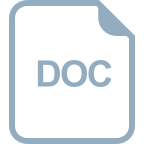
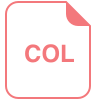
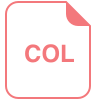
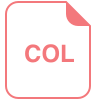
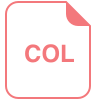
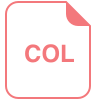
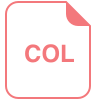