Practical PyCharm Python Version Switching: Resolving Common Issues and Troubleshooting
发布时间: 2024-09-15 15:44:55 阅读量: 55 订阅数: 36 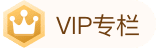
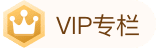
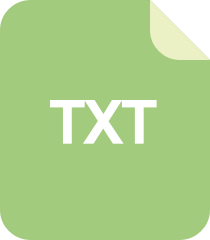
PyCharm配置Python环境:快速入门指南.txt
# PyCharm Python Version Switching: Solving Common Issues and Troubleshooting
PyCharm is a popular Python IDE that allows users to easily switch between different Python versions. This is incredibly useful for developers who work on multiple Python versions, for instance, when they need to support legacy code or utilize the latest Python features.
## 1. Basic Python Version Switching in PyCharm
Switching Python versions in PyCharm is a straightforward process. First, open the "Settings" dialog box (on macOS, this is "Preferences"). Then, navigate to the "Project: Interpreter" settings page. Here, you can see the currently selected Python version and a list of all available versions. To switch versions, simply select the desired version from the list.
## 2. Practical Python Version Switching
### 2.1 Detailed Steps for Switching Versions
**Step 1: Install Multiple Python Versions**
* Use the official Python website or package managers (like pip) to install the desired Python versions.
* Ensure each version is correctly installed and configured in the PATH environment variable.
**Step 2: Open PyCharm**
* Start PyCharm and create a new project.
**Step 3: Configure Project Interpreter**
* In PyCharm, go to "File" > "Settings" > "Project" > "Project Interpreter".
* Select the desired Python version from the dropdown list.
**Step 4: Create a Virtual Environment (Optional)**
* If you need to isolate your project environment, you can create a virtual environment.
* In PyCharm, go to "File" > "Settings" > "Project" > "Project Interpreter".
* Click the "Add" button and select "Virtual Environment".
* Specify the name and location of the virtual environment.
**Step 5: Verify Version Switching**
* Open a terminal or command prompt window in PyCharm.
* Run the following command to verify the switched Python version:
```
python --version
```
### 2.2 Common Problems and Solutions
**Problem 1: Unable to See All Installed Python Versions in PyCharm**
***Solution:** Ensure that all Python versions have been correctly added to the PATH environment variable.
**Problem 2: Errors After Switching Versions**
***Solution:** Check if the Python version is compatible with project dependencies. Update dependencies or use a compatible Python version.
**Problem 3: Virtual Environment Cannot Be Activated**
***Solution:** Ensure that the virtual environment has been correctly created and added to the PyCharm project interpreter.
**Problem 4: Unable to Install Dependencies in the Virtual Environment**
***Solution:** Ensure the virtual environment is activated. Run the following command in the terminal or command prompt window:
```
source activate <virtualenv_name>
```
**Problem 5: PyCharm Fails to Recognize Installed Python Versions**
***Solution:** Restart PyCharm and reconfigure the project interpreter.
## 3. Advanced Python Version Switching
### 3.1 Coexistence of Multiple Python Versions
In some cases, you may need to have multiple Python versions coexist on the same computer. For instance, you might need to use different Python versions for various projects, or you might need to use a specific version of Python to run old code.
In PyCharm, you can install and manage multiple Python versions on the same computer by following these steps:
1. Open PyCharm and go to "File" > "Settings" > "Project" > "Python Interpreter".
2. Click the "Add" button and select the Python version you wish to install.
3. Repeat step 2 until you have installed all the required Python versions.
4. Select the Python version you want to use for your current project.
**Code Block:**
```python
import sys
print(sys.version)
```
**Logical Analysis:**
This code block uses the `sys.version` variable to print detailed information about the current Python version.
**Argument Explanation:**
* `sys.version`: A string containing information about the current Python version.
### 3.2 Virtual Environment Management
Virtual environments are a way to isolate Python installations. This allows you to install and manage different Python versions and packages without affecting the system-wide Python installation.
In PyCharm, you can create and manage virtual environments by following these steps:
1. Open PyCharm and go to "File" > "Settings" > "Project" > "Python Interpreter".
2. Click the "Create Virtual Environment" button.
3. Select the path where you want to create the virtual environment.
4. Select the Python version you want to use for the virtual environment.
5. Click the "Create" button.
**Code Block:**
```python
import venv
venv.create("my_venv")
```
**Logical Analysis:**
This code block uses the `venv` module to create a virtual environment named "my_venv".
**Argument Explanation:**
* `venv.create()`: Creates a virtual environment.
* `my_venv`: The name of the virtual environment.
**Table: Virtual Environment Management in PyCharm**
| Operation | Shortcut |
|---|---|
| Create Virtual Environment | Ctrl+Alt+R |
| Activate Virtual Environment | Ctrl+Shift+A |
| Deactivate Virtual Environment | Ctrl+Shift+D |
| Delete Virtual Environment | Ctrl+Alt+Shift+D |
**Mermaid Flowchart: Advanced Python Version Switching**
```mermaid
graph LR
subgraph Coexistence of Multiple Python Versions
A[Install Multiple Python Versions] --> B[Choose Python Version]
end
subgraph Virtual Environment Management
C[Create Virtual Environment] --> D[Activate Virtual Environment]
D --> E[Deactivate Virtual Environment]
D --> F[Delete Virtual Environment]
end
```
## 4. Troubleshooting Python Version Switching
### 4.1 Unable to Switch Versions
**Problem Description:**
When attempting to switch Python versions in PyCharm, you encounter an inability to switch.
**Possible Causes:**
- **Python Interpreter Not Installed:** Ensure that the desired Python version is installed on your system.
- **Incorrect PyCharm Settings:** Check the "Project Interpreter" settings in PyCharm to ensure that the desired Python version has been correctly configured.
- **Damaged Project Files:** The project files in the .idea directory might be damaged, causing version switching issues.
- **Permission Problems:** Ensure you have permission to change the project interpreter.
**Solutions:**
- **Install Python Interpreter:** Download and install the desired Python version from the official website.
- **Check PyCharm Settings:** In "Settings" > "Project Interpreter," select the correct Python version.
- **Delete .idea Directory:** Close PyCharm, delete the .idea directory in the project folder, then reopen the project.
- **Check Permissions:** Ensure you have permission to change the project interpreter, and if necessary, run PyCharm with administrative privileges.
### 4.2 Errors After Switching Versions
**Problem Description:**
After switching Python versions, errors occur when running the project.
**Possible Causes:**
- **Incompatible Libraries:** The switched Python version might be incompatible with existing dependency libraries.
- **Code Incompatibility:** The code might rely on specific features of a particular Python version, causing incompatibility after switching versions.
- **Environment Variables Not Updated:** After switching versions, environment variables might not be updated, leading to an inability to find the correct Python interpreter.
**Solutions:**
- **Update Dependency Libraries:** Use pip or conda to update the dependency libraries used in the project to ensure compatibility with the new Python version.
- **Modify Code:** Check the code and modify any parts that rely on specific features of a particular Python version.
- **Update Environment Variables:** Update the PYTHONPATH variable in system environment variables to point to the new Python interpreter installation directory.
**Code Example:**
```python
# Python 3.7 code
print("Hello, Python 3.7!")
# Python 3.8 code
print("Hello, Python 3.8!")
```
**Logical Analysis:**
The above code examples demonstrate potential incompatibility issues that might arise when running code on different Python versions. Running Python 3.8 code on Python 3.7 will result in a syntax error because Python 3.8 introduced f-string syntax.
**Argument Explanation:**
- `print()`: Used to output information to the console.
- `PYTHONPATH`: An environment variable that specifies the search path for the Python interpreter.
## 5.1 Version Management Strategy
**Version Management Principles**
***Priority on Stability:** For production environments, use stable and verified Python versions.
***Compatibility Considerations:** Ensure Python versions are compatible with project dependencies and operating systems.
***Performance Optimization:** Choose a Python version that matches project requirements to optimize performance.
***Security Assurance:** Keep Python versions updated to fix security vulnerabilities and enhance security.
**Version Management Methods**
***Centralized Management:** Use version control systems (such as Git) to centrally manage Python versions, allowing team members to share and collaborate.
***Version Tagging:** Create tags for different Python versions for quick switching and identification.
***Documentation:** Record the Python version used in the project and regularly update the documentation.
**Version Switching Process**
1. **Determine Required Version:** Based on project needs and compatibility considerations, determine the Python version to switch to.
2. **Backup Project:** Back up project code and data before switching versions, as a precaution.
3. **Update Virtual Environment:** Use virtual environment management tools (such as virtualenv) to update the Python version in the virtual environment.
4. **Test Code:** After switching versions, thoroughly test the code to ensure compatibility and correctness.
5. **Deploy Update:** If tests pass, deploy the update to the production environment.
## 5.2 Prevention of Troublesome Issues
**Common Troublesome Issues**
***Unable to Switch Versions:** Ensure the virtual environment is correctly configured and path settings are accurate.
***Errors After Switching Versions:** Check if dependencies are compatible with the new version and update or install the required dependencies.
***Code Compatibility Issues:** Carefully review the code, identify, and resolve segments incompatible with the new version.
**Preventative Measures**
***Regular Updates:** Keep Python versions updated to fix security vulnerabilities and enhance stability.
***Test-Driven:** Validate code compatibility through unit tests and integration tests before switching versions.
***Monitor Errors:** Use logs and monitoring tools to monitor errors, allowing for quick identification and resolution of issues arising from version switching.
***Rollback Strategy:** Develop a rollback strategy to quickly revert to a previous Python version in case of problems.
0
0
相关推荐







