C++ OpenCV人脸跟踪算法深度解析:原理、实现与应用,解锁人脸识别新境界
发布时间: 2024-08-08 07:05:06 阅读量: 28 订阅数: 36 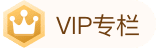
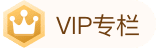
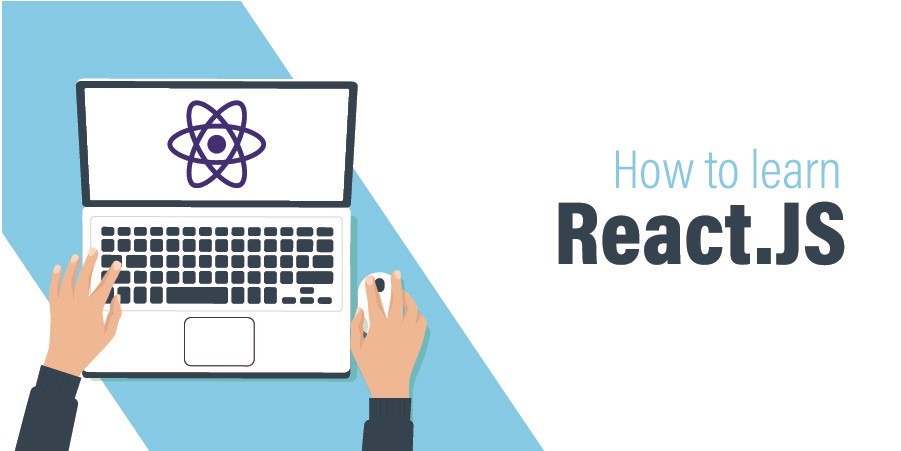
# 1. 人脸跟踪算法概述**
人脸跟踪算法是一种计算机视觉技术,用于实时定位和跟踪图像或视频序列中的人脸。它在各种应用中至关重要,例如人脸识别、视频监控和增强现实。
人脸跟踪算法通常基于概率模型,如卡尔曼滤波或粒子滤波。这些模型利用运动模型和观测模型来估计人脸的位置和状态。运动模型描述人脸的运动模式,而观测模型描述人脸在图像或视频中的外观。
通过结合运动模型和观测模型,人脸跟踪算法可以根据先前的帧中的信息预测人脸在当前帧中的位置。然后,它使用观测模型将预测与当前帧中的实际观测进行比较,并更新其状态估计。
# 2. OpenCV人脸跟踪算法原理**
人脸跟踪算法是计算机视觉领域中至关重要的技术,它能够在视频序列中实时定位和跟踪人脸。OpenCV作为计算机视觉领域的领先库,提供了强大的算法和函数来实现人脸跟踪。本章将深入解析OpenCV人脸跟踪算法的原理,包括卡尔曼滤波和粒子滤波。
**2.1 卡尔曼滤波**
卡尔曼滤波是一种线性动态系统状态估计算法,它广泛应用于跟踪问题中。在人脸跟踪中,卡尔曼滤波用于预测人脸的位置和速度,并根据观测结果更新估计值。
**2.1.1 理论基础**
卡尔曼滤波基于以下状态空间模型:
```
x_k = A * x_{k-1} + B * u_k + w_k
y_k = C * x_k + v_k
```
其中:
* x_k:时刻k的状态向量(例如,人脸位置和速度)
* u_k:时刻k的控制输入(通常为0)
* y_k:时刻k的观测值(例如,人脸检测框)
* A、B、C:状态转移矩阵、控制矩阵和观测矩阵
* w_k、v_k:过程噪声和观测噪声(假设为高斯分布)
卡尔曼滤波通过两个步骤更新状态估计值:
* **预测步骤:**根据前一时刻的状态估计值和控制输入,预测当前时刻的状态。
* **更新步骤:**根据当前时刻的观测值,更新状态估计值。
**2.1.2 OpenCV实现**
OpenCV提供了`KalmanFilter`类来实现卡尔曼滤波。该类包含以下关键参数:
* **状态转移矩阵A:**描述状态如何随时间变化。
* **观测矩阵C:**描述观测值如何与状态相关。
* **过程噪声协方差矩阵Q:**描述过程噪声的协方差。
* **观测噪声协方差矩阵R:**描述观测噪声的协方差。
以下代码示例展示了如何使用OpenCV的`KalmanFilter`类进行人脸跟踪:
```cpp
// 创建卡尔曼滤波器对象
KalmanFilter kf(4, 2, 0);
// 初始化状态转移矩阵A
Mat A = (Mat_<float>(4, 4) <<
1, 0, 1, 0,
0, 1, 0, 1,
0, 0, 1, 0,
0, 0, 0, 1);
// 初始化观测矩阵C
Mat C = (Mat_<float>(2, 4) <<
1, 0, 0, 0,
0, 1, 0, 0);
// 初始化过程噪声协方差矩阵Q
Mat Q = (Mat_<float>(4, 4) <<
0.0001, 0, 0, 0,
0, 0.0001, 0, 0,
0, 0, 0.0001, 0,
0, 0, 0, 0.0001);
// 初始化观测噪声协方差矩阵R
Mat R = (Mat_<float>(2, 2) <<
0.0001, 0,
0, 0.0001);
// 设置卡尔曼滤波器参数
kf.transitionMatrix = A;
kf.measurementMatrix = C;
kf.processNoiseCov = Q;
kf.measurementNoiseCov = R;
// 设置初始状态估计值
Mat state = (Mat_<float>(4, 1) <<
0, 0, 0, 0);
kf.statePost = state;
// ...(人脸检测和跟踪代码)
```
**2.2 粒子滤波**
粒子滤波是一种蒙特卡罗方法,用于非线性非高斯系统状态估计。在人脸跟踪中,粒子滤波通过维护一组粒子(代表可能的状态)来估计人脸的位置和速度。
**2.2.1 理论基础**
粒子滤波基于以下原理:
* **粒子表示:**粒子是状态空间中的随机采样点,其权重表示该点的概率。
* **粒子传播:**粒子根据状态转移概率分布传播到新的位置。
* **粒子权重更新:**粒子权重根据观测值和状态转移概率分布更新。
* **粒子重采样:**权重较低的粒子被淘汰,权重较高的粒子被复制,以保持粒子的多样性。
**2.2.2 OpenCV实现**
OpenCV提供了`ParticleFilter`类来实现粒子滤波。该类包含以下关键参数:
* **粒子数量:**粒子滤波器中粒子的数量。
* **状态转移模型:**描述状态如何随时间变化的模型。
* **观测模型:**描述观测值如何与状态相关。
* **重采样策略:**用于重采样的策略(例如,系统重采样或残差重采样)。
以下代码示例展示了如何使用OpenCV的`ParticleFilter`类进行人脸跟踪:
```cpp
// 创建粒子滤波器对象
ParticleFilter pf(100, 4, 2);
// 初始化状态转移模型
Mat A = (Mat_<float>(4, 4) <<
1, 0, 1, 0,
0, 1, 0, 1,
0, 0, 1, 0,
0, 0, 0, 1);
// 初始化观测模型
Mat C = (Mat_<float>(2, 4) <<
1, 0, 0, 0,
0, 1, 0, 0);
// 设置粒子滤波器参数
pf.transitionModel = A;
pf.measurementModel = C;
pf.resamplingMethod = ParticleFilter::RESAMPLING_METHOD_SYSTEMATIC;
// 设置初始粒子
Mat state = (Mat_<float>(4, 1) <<
0, 0, 0, 0);
pf.setParticles(state);
// ...(人脸检测和跟踪代码)
```
# 3. OpenCV人脸跟踪算法实现**
### 3.1 卡尔曼滤波跟踪
**3.1.1 代码框架**
```cpp
#include <opencv2/opencv.hpp>
using namespace cv;
int main() {
// 初始化视频捕获器
VideoCapture cap("video.mp4");
// 检测第一帧的人脸
Mat frame;
```
0
0
相关推荐
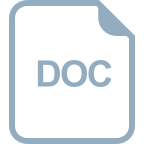
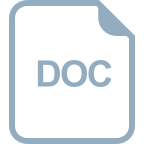
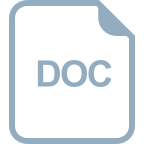
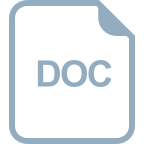
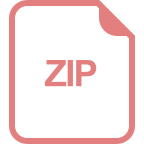
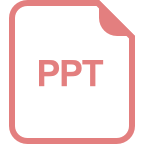
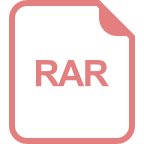
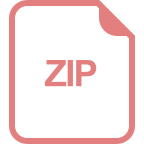