STM32单片机固件更新全攻略:掌握固件升级与维护,保障系统稳定
发布时间: 2024-07-03 13:00:45 阅读量: 169 订阅数: 51 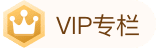
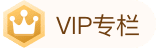
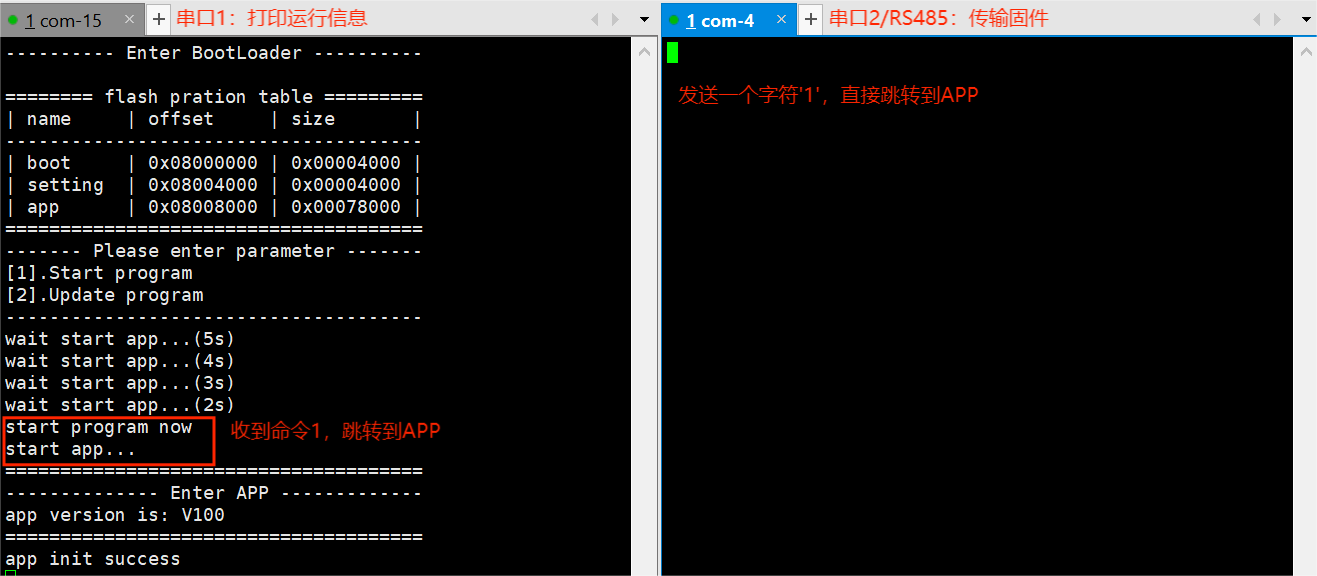
# 1. 固件更新概述
固件更新是嵌入式系统维护中的关键任务,它涉及将新固件映像加载到设备中以增强功能、修复缺陷或提高安全性。在STM32单片机中,固件更新通常通过串口、USB或无线连接进行。
固件更新过程包括以下步骤:
- **准备工作:**收集必要的工具和资源,包括固件映像、编程器和连接电缆。
- **固件烧录:**使用编程器将固件映像写入设备的闪存。
- **验证升级结果:**检查设备是否已成功升级,并确保新固件正常运行。
# 2. 固件升级方式
### 2.1 串口升级
串口升级是一种通过串口通信接口对固件进行更新的方式,具有成本低、操作简单等优点。
**操作步骤:**
1. 准备升级工具:串口编程器、升级软件
2. 连接串口编程器与单片机
3. 打开升级软件,选择固件文件
4. 设置串口参数(波特率、数据位、停止位、校验位)
5. 开始升级,等待升级完成
**代码示例:**
```c
#include <stdio.h>
#include <stdlib.h>
int main()
{
// 打开串口
int fd = open("/dev/ttyUSB0", O_RDWR);
if (fd < 0) {
perror("open serial port failed");
return -1;
}
// 设置串口参数
struct termios options;
tcgetattr(fd, &options);
options.c_cflag |= (CLOCAL | CREAD);
options.c_cflag &= ~PARENB;
options.c_cflag &= ~CSTOPB;
options.c_cflag &= ~CSIZE;
options.c_cflag |= CS8;
options.c_cc[VTIME] = 0;
options.c_cc[VMIN] = 1;
tcsetattr(fd, TCSANOW, &options);
// 发送升级数据
FILE *fp = fopen("firmware.bin", "rb");
if (fp == NULL) {
perror("open firmware file failed");
return -1;
}
while (!feof(fp)) {
char buf[1024];
int len = fread(buf, 1, sizeof(buf), fp);
if (len > 0) {
write(fd, buf, len);
}
}
fclose(fp);
// 等待升级完成
sleep(1);
// 关闭串口
close(fd);
return 0;
}
```
**逻辑分析:**
* 打开串口并设置串口参数
* 从固件文件中读取数据
* 通过串口发送数据
* 等待升级完成
* 关闭串口
### 2.2 USB升级
USB升级是一种通过USB接口对固件进行更新的方式,具有传输速度快、操作方便等优点。
**操作步骤:**
1. 准备升级工具:USB编程器、升级软件
2. 连接USB编程器与单片机
3. 打开升级软件,选择固件文件
4. 设置USB参数(VID、PID)
5. 开始升级,等待升级完成
**代码示例:**
```c
#include <stdio.h>
#include <stdlib.h>
#include <libusb.h>
int main()
{
// 初始化libusb
int ret = libusb_init(NULL);
if (ret < 0) {
perror("libusb_init failed");
return -1;
}
// 打开USB设备
libusb_device **devs;
libusb_device_handle *dev_handle;
ret = libusb_get_device_list(NULL, &devs);
if (ret < 0) {
perror("libusb_get_device_list failed");
return -1;
}
for (int i = 0; i < ret; i++) {
libusb_device *dev = devs[i];
struct libusb_device_descriptor desc;
ret = libusb_get_device_descriptor(dev, &desc);
if (ret < 0) {
perror("libusb_get_device_descriptor failed");
continue;
}
if (desc.idVendor == 0x0483 && desc.idProduct == 0x5740) {
dev_handle = libusb_open(dev, NULL);
if (dev_handle == NULL) {
perror("libusb_open failed");
continue;
}
}
}
// 发送升级数据
FILE *fp = fopen("firmware.bin", "rb");
if (fp == NULL) {
perror("open firmware file failed");
return -1;
}
while (!feof(fp))
```
0
0
相关推荐







