【CAD二次开发中文文档指南】:深入解析2025版CAD二次开发文档,助你轻松入门
发布时间: 2024-07-21 23:10:09 阅读量: 218 订阅数: 31 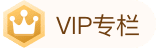
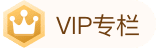
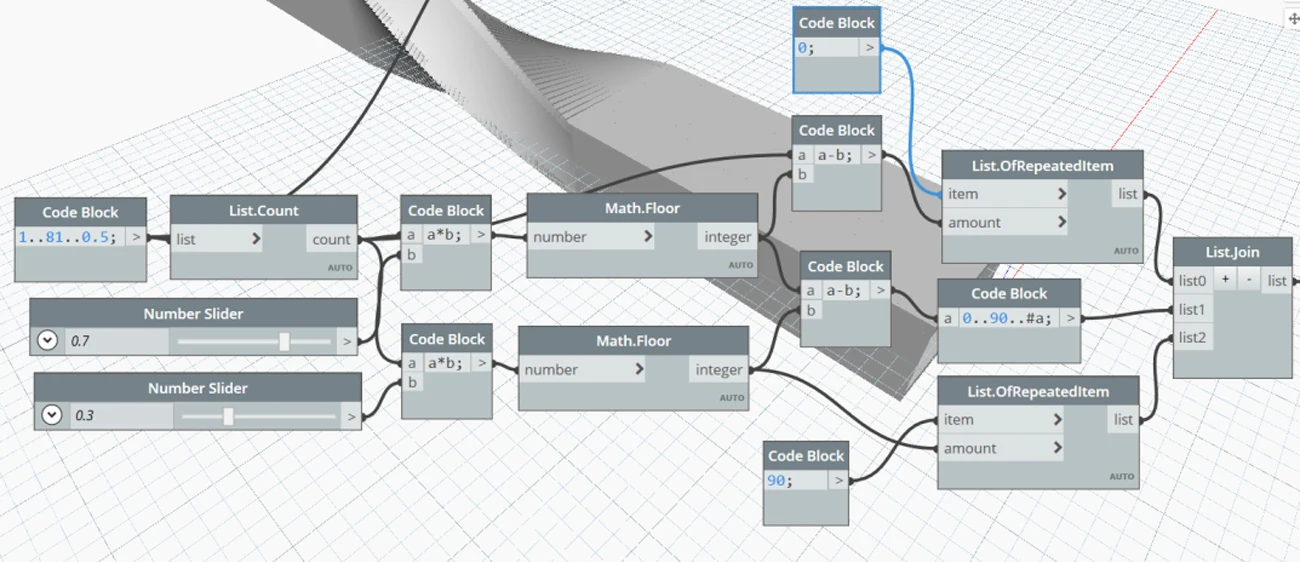
# 1. CAD二次开发概述**
CAD二次开发是指在现有CAD软件的基础上,通过编程技术扩展其功能,满足特定需求。它涉及到一系列理论基础和实践指南,包括平台介绍、编程语言选择、开发工具使用、核心技术应用等。
CAD二次开发的好处包括:
- 提高效率:自动化重复性任务,减少人工操作时间。
- 扩展功能:增加CAD软件无法实现的特定功能。
- 定制化:根据具体需求定制CAD软件,满足个性化需求。
CAD二次开发适用于广泛的行业,包括建筑、机械、制造等。它为工程师、设计师和技术人员提供了强大的工具,帮助他们提高工作效率,解决复杂问题,并创造创新解决方案。
# 2. CAD二次开发理论基础
### 2.1 CAD二次开发平台介绍
#### 2.1.1 AutoCAD平台架构
AutoCAD是一个计算机辅助设计(CAD)软件,它基于一个模块化平台,允许开发人员创建自定义应用程序和扩展功能。AutoCAD平台架构主要包括以下组件:
- **图形引擎:**负责渲染和显示几何图形,包括线、圆、多边形和3D模型。
- **数据库:**存储和管理CAD图纸中包含的所有数据,包括几何实体、属性和元数据。
- **应用程序编程接口(API):**提供一组函数和类,允许开发人员与AutoCAD平台交互,创建自定义应用程序。
- **用户界面:**提供用户与AutoCAD交互的图形界面,包括菜单、工具栏和命令行。
#### 2.1.2 .NET和COM技术
AutoCAD二次开发主要使用两种技术:
- **.NET:**一种面向对象的编程语言,用于创建在Microsoft Windows平台上运行的应用程序。.NET提供了一系列类库,简化了开发人员创建复杂应用程序的任务。
- **COM(组件对象模型):**一种跨语言和平台的二进制接口标准,允许应用程序相互通信。AutoCAD通过COM公开其API,使开发人员可以使用.NET和其他编程语言访问AutoCAD功能。
### 2.2 CAD二次开发编程语言
#### 2.2.1 C#语言基础
C#是一种面向对象的编程语言,是.NET平台的主要语言。C#具有以下特点:
- **类型安全:**C#是一个类型安全的语言,这意味着在编译时检查变量和表达式的类型,以防止类型不匹配错误。
- **面向对象:**C#支持面向对象编程范式,允许开发人员创建类和对象来表示现实世界中的实体。
- **垃圾回收:**C#具有自动垃圾回收功能,可以自动释放不再使用的内存,从而简化了内存管理。
#### 2.2.2 Visual Studio集成开发环境
Visual Studio是Microsoft开发的集成开发环境(IDE),用于创建和管理.NET应用程序。Visual Studio提供了一系列工具和功能,包括:
- **代码编辑器:**用于编写、调试和维护C#代码。
- **调试器:**用于调试应用程序,查找和修复错误。
- **项目管理:**用于管理应用程序的项目文件和依赖项。
- **版本控制:**用于与其他开发人员协作并管理代码更改。
# 3.1 AutoCAD二次开发入门
### 3.1.1 创建第一个CAD插件
**步骤:**
1. 打开Visual Studio,新建一个C#类库项目。
2. 在解决方案资源管理器中,右键单击项目,选择“添加”>“新建项”。
3. 在“添加新项”对话框中,选择“AutoCAD插件模板”,然后单击“添加”。
4. 在“插件信息”对话框中,输入插件名称、描述和作者信息。
5. 单击“确定”创建插件。
**代码块:**
```csharp
using Autodesk.AutoCAD.Runtime;
namespace MyPlugin
{
public class MyPlugin : IExtensionApplication
{
public void Initialize()
{
// 插件初始化代码
}
public void Terminate()
{
// 插件终止代码
}
}
}
```
**逻辑分析:**
* `Initialize()`方法在加载插件时调用,用于执行插件初始化代码。
* `Terminate()`方法在卸载插件时调用,用于执行插件终止代码。
**参数说明:**
* `Autodesk.AutoCAD.Runtime`命名空间包含CAD二次开发所需的类和接口。
* `IExtensionApplication`接口定义了插件的基本功能。
### 3.1.2 绘制几何图形
**步骤:**
1. 在插件代码中,使用`Database`类访问当前CAD文档。
2. 使用`Transaction`类开启一个事务,以修改文档。
3. 使用`Entity`类创建几何图形实体。
4. 将实体添加到文档中。
5. 提交事务,以保存更改。
**代码块:**
```csharp
using Autodesk.AutoCAD.DatabaseServices;
namespace MyPlugin
{
public class MyPlugin : IExtensionApplication
{
public void Initialize()
{
// 获取当前文档的数据库
Database db = HostApplicationServices.WorkingDatabase;
// 开启一个事务
using (Transaction trans = db.TransactionManager.StartTransaction())
{
// 创建一个圆
Circle circle = new Circle();
circle.Center = new Point3d(0, 0, 0);
circle.Radius = 10;
// 将圆添加到文档中
db.ModelSpace.AddEntity(circle);
// 提交事务
trans.Commit();
}
}
public void Terminate()
{
// 插件终止代码
}
}
}
```
**逻辑分析:**
* `DatabaseServices`命名空间包含CAD文档中实体和事务的类。
* `Transaction`类用于管理对文档的修改。
* `Entity`类是所有CAD实体的基类。
* `ModelSpace`属性表示文档中的模型空间,用于添加实体。
**参数说明:**
* `HostApplicationServices.WorkingDatabase`属性获取当前CAD文档的数据库。
* `TransactionManager.StartTransaction()`方法开启一个事务。
* `Circle`类表示一个圆形实体。
* `Center`属性设置圆的中心点。
* `Radius`属性设置圆的半径。
* `AddEntity()`方法将实体添加到文档中。
* `Commit()`方法提交事务,保存更改。
# 4.1 CAD二次开发扩展技术
### 4.1.1 图形引擎扩展
图形引擎扩展是CAD二次开发中一项重要的技术,它允许开发人员扩展CAD软件的图形功能,创建更加复杂和交互式的图形应用。常用的图形引擎扩展技术包括:
- **OpenGL和DirectX:**这些API提供了低级别的图形编程接口,允许开发人员直接控制图形渲染过程。
- **OpenSceneGraph:**这是一个开源的图形库,提供了高级别的图形编程接口,简化了复杂场景的创建和管理。
- **ParaView:**这是一个开源的可视化和分析工具,提供了交互式的数据可视化和处理功能。
### 4.1.2 云端服务集成
云端服务集成使CAD二次开发能够利用云计算的强大功能,扩展CAD软件的功能和可用性。常见的云端服务集成技术包括:
- **云存储:**将CAD文件和数据存储在云端,实现跨设备和平台的访问和协作。
- **云计算:**利用云端服务器的计算能力,执行复杂和耗时的任务,例如渲染和仿真。
- **云端协作:**使用云端平台进行团队协作,实现多人同时编辑和修改CAD文件。
### 4.2 CAD二次开发行业应用
CAD二次开发在各个行业都有着广泛的应用,为特定领域的专业人员提供了定制化的解决方案。
### 4.2.1 建筑设计
在建筑设计行业,CAD二次开发可以:
- **自动化建筑设计流程:**创建自定义工具,自动化重复性任务,例如生成平面图、立面图和剖面图。
- **优化建筑性能:**集成仿真和分析工具,优化建筑物的能源效率、结构稳定性和空间利用率。
- **增强协作:**开发云端协作平台,实现建筑师、工程师和承包商之间的无缝协作。
### 4.2.2 机械工程
在机械工程行业,CAD二次开发可以:
- **创建定制化机械零件:**开发工具生成复杂和精确的机械零件,满足特定的设计要求。
- **自动化机械装配:**创建工具自动化机械装配过程,提高效率和准确性。
- **仿真和分析:**集成仿真和分析工具,评估机械设计的性能和可靠性。
# 5.1 AutoCAD 2025版二次开发文档结构
AutoCAD 2025版二次开发文档主要分为两部分:开发者指南和API参考。
### 5.1.1 开发者指南
开发者指南提供了AutoCAD二次开发的基础知识和指导,包括:
- CAD二次开发概述
- CAD二次开发平台介绍
- CAD二次开发编程语言
- CAD二次开发实践指南
- CAD二次开发进阶应用
开发者指南是入门AutoCAD二次开发的必读文档,为开发者提供了全面的理论基础和实践指导。
### 5.1.2 API参考
API参考提供了AutoCAD 2025版中所有API的详细描述,包括:
- 类和接口
- 方法和属性
- 常量和枚举
- 事件和委托
API参考是开发者开发AutoCAD二次开发应用程序时不可或缺的参考文档,为开发者提供了详细的技术信息。
0
0
相关推荐
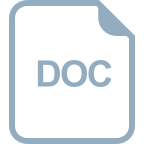
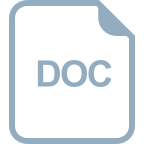
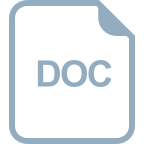
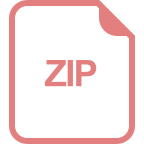
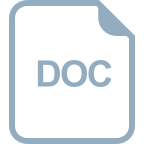
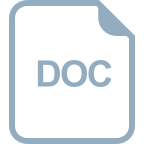
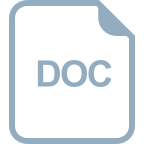
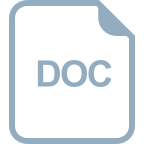
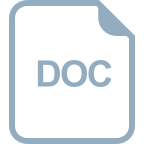