揭秘PHP数据库插入性能优化:瓶颈分析与提速秘籍
发布时间: 2024-07-24 09:50:25 阅读量: 29 订阅数: 29 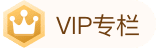
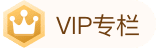
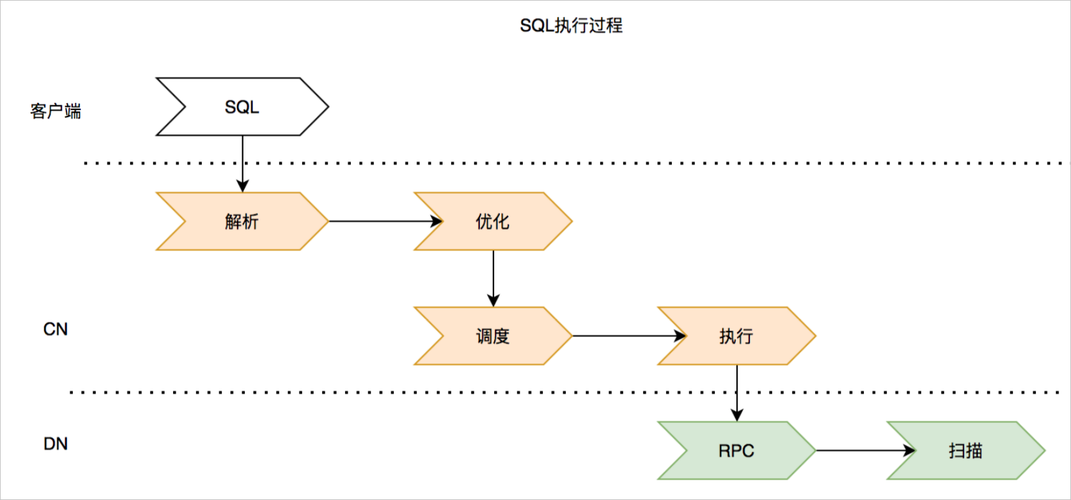
# 1. PHP数据库插入性能瓶颈分析
**1.1 数据库配置瓶颈**
* 数据库连接数不足导致并发插入受限
* 缓冲池大小过小,导致频繁磁盘IO
* 日志记录级别过高,影响插入速度
**1.2 索引瓶颈**
* 缺少必要的索引,导致全表扫描
* 索引不合理,导致索引覆盖度低
* 索引维护不当,导致索引碎片化
# 2. PHP数据库插入性能优化实践
### 2.1 服务器端优化
#### 2.1.1 数据库配置优化
**参数调整:**
- **innodb_flush_log_at_trx_commit:**控制事务提交时是否立即将日志写入磁盘。设置为`2`可以提高性能,但会增加数据丢失的风险。
- **innodb_buffer_pool_size:**指定缓冲池大小。增加缓冲池大小可以减少磁盘IO,提高性能。
- **innodb_log_file_size:**指定日志文件大小。较大的日志文件可以减少日志切换的频率,提高性能。
**示例代码:**
```bash
# 调整 innodb_flush_log_at_trx_commit 参数
mysql -e "SET GLOBAL innodb_flush_log_at_trx_commit=2"
# 调整 innodb_buffer_pool_size 参数
mysql -e "SET GLOBAL innodb_buffer_pool_size=1G"
# 调整 innodb_log_file_size 参数
mysql -e "SET GLOBAL innodb_log_file_size=512M"
```
#### 2.1.2 索引优化
**创建适当的索引:**
为经常查询的列创建索引,可以大大提高查询速度。
**示例代码:**
```sql
CREATE INDEX idx_name ON table_name (column_name);
```
**删除不必要的索引:**
不必要的索引会增加数据库维护开销,降低性能。
**示例代码:**
```sql
DROP INDEX idx_name ON table_name;
```
#### 2.1.3 查询优化
**避免不必要的连接:**
使用`JOIN`连接表时,只连接必要的表。
**示例代码:**
```sql
SELECT * FROM table1 JOIN table2 ON table1.id = table2.id;
```
**使用子查询:**
将复杂查询分解成子查询,可以提高性能。
**示例代码:**
```sql
SELECT * FROM table1 WHERE id IN (SELECT id FROM table2 WHERE name = 'John');
```
### 2.2 客户端优化
#### 2.2.1 代码优化
**使用批量插入:**
一次性插入多条记录,可以减少数据库连接次数,提高性能。
**示例代码:**
```php
$stmt = $conn->prepare("INSERT INTO table_name (column1, column2) VALUES (?, ?)");
$stmt->bind_param("ss", $column1, $column2);
for ($i = 0; $i < 100; $i++) {
$stmt->execute();
}
```
**避免不必要的查询:**
只查询必要的字段,避免不必要的数据库交互。
**示例代码:**
```php
$stmt = $conn->prepare("SELECT id, name FROM table_name WHERE id = ?");
$stmt->bind_param("i", $id);
```
#### 2.2.2 缓存优化
**使用缓存:**
将经常查询的数据缓存起来,可以减少数据库访问次数,提高性能。
**示例代码:**
```php
// 使用 Memcached 缓存
$cache = new Memcached();
$cache->add("key", $value, 3600);
```
#### 2.2.3 并发控制
**使用事务:**
在需要保证数据一致性的操作中,使用事务可以防止并发冲突。
**示例代码:**
```php
$conn->begin_transaction();
try {
// 执行操作
$conn->commit();
} catch (Exception $e) {
$conn->rollback();
}
```
**使用锁:**
在需要防止并发修改数据的操作中,使用锁可以保证数据完整性。
**示例代码:**
```php
$conn->query("LOCK TABLE table_name WRITE");
// 执行操作
$conn->query("UNLOCK TABLE table_name");
```
# 3. PHP数据库插入性能测试与监控
### 3.1 性能测试工具介绍
性能测试工具主要分为两类:基准测试工具和压力测试工具。
#### 3.1.1 基准测试工具
基准测试工具用于测量系统在特定负载下的性能,帮助确定系统的性能极限和瓶颈。常用的基准测试工具包括:
- **PHPBench:** 一个用于 PHP 基准测试的开源框架。
- **Siege:** 一个命令行工具,用于对 Web 服务器进行基准测试。
- **JMeter:** 一个开源的负载测试工具,可以模拟大量并发用户。
#### 3.1.2 压力测试工具
压力测试工具用于模拟真实世界的负载,测试系统在高并发和高负载下的稳定性和可靠性。常用的压力测试工具包括:
- **wrk:** 一个命令行工具,用于对 HTTP 服务器进行压力测试。
- **Locust:** 一个 Python 框架,用于编写分布式压力测试脚本。
- **Vegeta:** 一个 Go 语言编写的压力测试工具,支持多种协议。
### 3.2 性能监控方法
性能监控对于识别和解决性能瓶颈至关重要。常见的性能监控方法包括:
#### 3.2.1 数据库监控
数据库监控工具可以监控数据库的性能指标,例如:
- **查询时间:** 每个查询执行所需的时间。
- **连接数:** 当前与数据库建立的连接数。
- **I/O 吞吐量:** 数据库读写数据的速率。
常用的数据库监控工具包括:
- **MySQLTuner:** 一个用于 MySQL 数据库性能调优的开源工具。
- **pgAdmin:** 一个用于 PostgreSQL 数据库管理和监控的工具。
- **MongoDB Compass:** 一个用于 MongoDB 数据库管理和监控的工具。
#### 3.2.2 应用监控
应用监控工具可以监控应用程序的性能指标,例如:
- **响应时间:** 应用程序处理请求所需的时间。
- **错误率:** 应用程序中发生的错误数量。
- **资源利用率:** 应用程序使用的 CPU、内存和网络资源。
常用的应用监控工具包括:
- **New Relic:** 一个商业应用监控平台,提供全面的性能监控和分析功能。
- **AppDynamics:** 一个商业应用监控平台,专注于应用程序性能管理。
- **Prometheus:** 一个开源监控系统,提供灵活的指标收集和查询功能。
# 4. PHP数据库插入性能调优案例
### 4.1 电商网站订单插入优化
#### 4.1.1 瓶颈分析
**数据库配置问题:**
- **innodb_buffer_pool_size** 设置过小,导致频繁的磁盘IO。
- **innodb_flush_log_at_trx_commit** 设置为 1,导致每条插入都会触发日志刷新,降低性能。
**索引问题:**
- 订单表缺少主键索引,导致每次插入都需要全表扫描。
- 缺少组合索引,导致查询效率低下。
**代码优化问题:**
- 使用 `INSERT` 语句逐条插入,效率低下。
- 未使用事务,导致每次插入都触发一次数据库连接。
#### 4.1.2 优化方案
**数据库配置优化:**
- 调整 **innodb_buffer_pool_size** 为服务器物理内存的 70-80%,以减少磁盘IO。
- 将 **innodb_flush_log_at_trx_commit** 设置为 2,以减少日志刷新频率。
**索引优化:**
- 为订单表添加主键索引,以加快查询速度。
- 添加组合索引,以优化查询效率。
**代码优化:**
- 使用批量插入语句,一次性插入多条数据。
- 使用事务,以减少数据库连接次数。
### 4.2 社交平台评论插入优化
#### 4.2.1 瓶颈分析
**缓存问题:**
- 评论内容未缓存,导致每次查询都需要从数据库中读取。
**数据库设计问题:**
- 评论表设计不合理,导致查询效率低下。
**并发控制问题:**
- 未对并发插入进行控制,导致数据竞争和死锁。
#### 4.2.2 优化方案
**缓存优化:**
- 使用缓存机制,将评论内容缓存起来,以减少数据库查询次数。
**数据库设计优化:**
- 优化评论表设计,以提高查询效率。
- 添加适当的索引,以加快查询速度。
**并发控制:**
- 使用锁机制,以控制并发插入,避免数据竞争和死锁。
# 5. PHP数据库插入性能优化最佳实践
### 5.1 优化原则
#### 5.1.1 避免不必要的插入
* 避免插入重复数据,使用唯一索引或主键约束。
* 考虑使用缓存或其他机制来减少对数据库的写入操作。
* 对于不需要持久存储的数据,可以使用内存数据库或临时表。
#### 5.1.2 批量插入
* 使用批量插入语句(如 `INSERT INTO ... VALUES ...`)一次插入多行数据。
* 批量插入可以减少与数据库的交互次数,提高性能。
* 优化批量插入大小,找到最佳平衡点以最大化吞吐量。
#### 5.1.3 异步插入
* 使用消息队列或其他异步机制将插入操作与主应用程序解耦。
* 异步插入可以防止写入操作阻塞主应用程序,提高响应时间。
* 确保异步插入机制具有可靠性,以避免数据丢失。
### 5.2 优化工具
#### 5.2.1 ORM框架
* 使用对象关系映射(ORM)框架,如 Doctrine 或 Eloquent,可以简化数据库交互。
* ORM框架可以自动生成批量插入语句,简化代码并提高性能。
#### 5.2.2 数据库连接池
* 使用数据库连接池可以减少创建和销毁数据库连接的开销。
* 连接池可以提高性能,尤其是在并发量大的情况下。
* 优化连接池大小,找到最佳平衡点以最大化吞吐量和资源利用率。
0
0
相关推荐
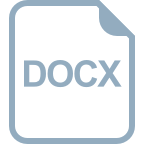
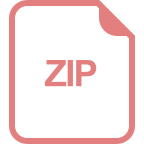