【PHP数据插入数据库实战指南】:从入门到精通的详细教程
发布时间: 2024-07-24 09:48:40 阅读量: 25 订阅数: 27 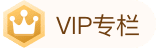
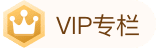

# 1. PHP数据库操作基础
PHP是一种广泛使用的脚本语言,它提供了强大的数据库操作功能。在本章中,我们将介绍PHP数据库操作的基础知识,包括连接数据库、执行查询、处理结果以及插入、更新和删除数据的基本操作。这些基础知识对于任何希望使用PHP进行数据库操作的开发人员都是至关重要的。
# 2. PHP数据库连接与查询
### 2.1 连接数据库
#### 2.1.1 mysqli_connect() 函数
mysqli_connect() 函数用于建立与 MySQL 数据库的连接。其语法如下:
```php
mysqli_connect(host, username, password, database, port, socket);
```
**参数说明:**
- host:数据库服务器地址,默认为 "localhost"。
- username:数据库用户名。
- password:数据库密码。
- database:要连接的数据库名称。
- port:数据库端口号,默认为 3306。
- socket:数据库套接字文件,默认为 "/tmp/mysql.sock"。
**示例:**
```php
$mysqli = mysqli_connect("localhost", "root", "password", "my_database");
```
#### 2.1.2 mysqli_connect_error() 函数
mysqli_connect_error() 函数用于获取连接错误信息。
**语法:**
```php
mysqli_connect_error();
```
**示例:**
```php
if (mysqli_connect_error()) {
echo "连接失败:" . mysqli_connect_error();
exit;
}
```
### 2.2 执行查询语句
#### 2.2.1 mysqli_query() 函数
mysqli_query() 函数用于执行 SQL 查询语句。其语法如下:
```php
mysqli_query(connection, query);
```
**参数说明:**
- connection:数据库连接句柄。
- query:要执行的 SQL 查询语句。
**示例:**
```php
$result = mysqli_query($mysqli, "SELECT * FROM users");
```
#### 2.2.2 mysqli_fetch_array() 函数
mysqli_fetch_array() 函数用于获取查询结果中的一行数据。其语法如下:
```php
mysqli_fetch_array(result);
```
**参数说明:**
- result:查询结果集。
**示例:**
```php
while ($row = mysqli_fetch_array($result)) {
echo $row["name"] . " " . $row["email"] . "<br>";
}
```
### 2.3 处理查询结果
#### 2.3.1 mysqli_num_rows() 函数
mysqli_num_rows() 函数用于获取查询结果集中行的数量。其语法如下:
```php
mysqli_num_rows(result);
```
**参数说明:**
- result:查询结果集。
**示例:**
```php
$num_rows = mysqli_num_rows($result);
```
#### 2.3.2 mysqli_close() 函数
mysqli_close() 函数用于关闭数据库连接。其语法如下:
```php
mysqli_close(connection);
```
**参数说明:**
- connection:数据库连接句柄。
**示例:**
```php
mysqli_close($mysqli);
```
# 3. PHP数据插入操作实战
### 3.1 准备数据
在执行插入操作之前,需要准备要插入的数据。数据可以是数组、对象或字符串。
**数组格式:**
```php
$data = [
'name' => 'John Doe',
'email' => 'john.doe@example.com',
'age' => 30
];
```
**对象格式:**
```php
class User {
public $name;
public $email;
public $age;
public function __construct($name, $email, $age) {
$this->name = $name;
$this->email = $email;
$this->age = $age;
}
}
$user = new User('John Doe', 'john.doe@example.com', 30);
```
**字符串格式:**
```php
$data = 'John Doe,john.doe@example.com,30';
```
### 3.2 执行插入语句
使用 `INSERT INTO` 语句插入数据。语法如下:
```sql
INSERT INTO table_name (column1, column2, ...) VALUES (value1, value2, ...)
```
**使用数组数据:**
```php
$sql = "INSERT INTO users (name, email, age) VALUES (:name, :email, :age)";
$stmt = $conn->prepare($sql);
$stmt->bindParam(':name', $data['name']);
$stmt->bindParam(':email', $data['email']);
$stmt->bindParam(':age', $data['age']);
$stmt->execute();
```
**使用对象数据:**
```php
$sql = "INSERT INTO users (name, email, age) VALUES (:name, :email, :age)";
$stmt = $conn->prepare($sql);
$stmt->bindParam(':name', $user->name);
$stmt->bindParam(':email', $user->email);
$stmt->bindParam(':age', $user->age);
$stmt->execute();
```
**使用字符串数据:**
```php
$sql = "INSERT INTO users (name, email, age) VALUES ('$data')";
$stmt = $conn->prepare($sql);
$stmt->execute();
```
### 3.3 处理插入结果
执行插入语句后,需要处理插入结果。
**获取插入的 ID:**
```php
$last_id = $conn->lastInsertId();
```
**获取受影响的行数:**
```php
$affected_rows = $stmt->rowCount();
```
**检查错误:**
```php
if ($stmt->errorCode() != 0) {
// 发生错误
}
```
# 4. PHP数据更新与删除操作
### 4.1 更新数据
更新操作用于修改数据库中已有的数据。PHP提供了`mysqli_query()`函数来执行更新语句。
**语法:**
```php
mysqli_query($conn, "UPDATE table_name SET column1 = value1, column2 = value2 WHERE condition");
```
**参数:**
* `$conn`: 数据库连接对象
* `table_name`: 要更新的表名
* `column1`, `column2`: 要更新的列名
* `value1`, `value2`: 要更新的值
* `condition`: 更新条件
**示例:**
```php
$conn = mysqli_connect("localhost", "root", "password", "database");
// 更新表中 id 为 1 的记录
$sql = "UPDATE users SET name = 'John Doe' WHERE id = 1";
if (mysqli_query($conn, $sql)) {
echo "Record updated successfully";
} else {
echo "Error updating record: " . mysqli_error($conn);
}
mysqli_close($conn);
```
### 4.2 删除数据
删除操作用于从数据库中删除数据。PHP提供了`mysqli_query()`函数来执行删除语句。
**语法:**
```php
mysqli_query($conn, "DELETE FROM table_name WHERE condition");
```
**参数:**
* `$conn`: 数据库连接对象
* `table_name`: 要删除数据的表名
* `condition`: 删除条件
**示例:**
```php
$conn = mysqli_connect("localhost", "root", "password", "database");
// 删除表中 id 为 1 的记录
$sql = "DELETE FROM users WHERE id = 1";
if (mysqli_query($conn, $sql)) {
echo "Record deleted successfully";
} else {
echo "Error deleting record: " . mysqli_error($conn);
}
mysqli_close($conn);
```
# 5. PHP数据库安全与优化
### 5.1 防范SQL注入
**什么是SQL注入?**
SQL注入是一种网络安全攻击,攻击者通过在用户输入中注入恶意SQL语句,从而控制数据库服务器。
**如何防范SQL注入?**
* **使用参数化查询:**使用参数化查询可以将用户输入与SQL语句分开,防止恶意代码被注入。
* **转义特殊字符:**在将用户输入插入数据库之前,对特殊字符(如单引号、双引号)进行转义。
* **使用白名单验证:**只允许用户输入预定义的合法值,防止恶意输入。
* **使用安全框架:**使用如OWASP ESAPI等安全框架,提供预先构建的防SQL注入功能。
### 5.2 优化数据库性能
**优化查询性能**
* **使用索引:**为经常查询的列创建索引,可以显著提高查询速度。
* **避免全表扫描:**使用WHERE子句过滤数据,避免对整个表进行扫描。
* **优化查询语句:**使用正确的连接符(如JOIN、UNION),避免子查询。
**优化数据结构**
* **选择合适的表类型:**根据数据特性选择合适的表类型,如InnoDB、MyISAM。
* **规范化数据:**将数据分解到多个表中,避免冗余和数据不一致。
* **设置合适的字段类型:**选择合适的字段类型,如整型、字符串、日期,以优化存储空间和查询性能。
**优化数据库服务器**
* **调整缓冲池大小:**根据系统负载调整缓冲池大小,以提高缓存命中率。
* **优化连接池:**使用连接池管理数据库连接,避免频繁创建和销毁连接。
* **定期维护:**定期进行数据库维护,如优化表、重建索引,以保持数据库性能。
**代码示例**
```php
// 使用参数化查询防范SQL注入
$stmt = $conn->prepare("SELECT * FROM users WHERE username = ?");
$stmt->bind_param("s", $username);
$stmt->execute();
// 使用索引优化查询性能
$stmt = $conn->prepare("SELECT * FROM users WHERE username = ?");
$stmt->bind_param("s", $username);
$stmt->execute();
$stmt->bind_result($id, $username, $email);
```
**逻辑分析**
* 使用参数化查询,将用户输入`$username`与SQL语句分开,防止恶意代码注入。
* 使用索引,通过`username`列快速查找数据,提高查询速度。
# 6.1 事务管理
事务管理是数据库中一种重要的概念,它允许将多个操作组合成一个原子单元。这意味着,要么所有操作都成功执行,要么所有操作都失败回滚。事务管理在确保数据一致性方面至关重要,尤其是在涉及多个数据库表或操作时。
### 事务的特性
事务具有以下四个特性,称为 ACID 特性:
- **原子性 (Atomicity)**:事务中的所有操作要么全部成功,要么全部失败。
- **一致性 (Consistency)**:事务执行前后的数据库状态都必须满足所有完整性约束。
- **隔离性 (Isolation)**:事务与其他并发事务隔离,不会相互影响。
- **持久性 (Durability)**:一旦事务提交,其对数据库所做的更改将永久生效,即使发生系统故障。
### PHP 中的事务管理
PHP 中使用 `PDO` 类进行事务管理。以下代码示例演示了如何使用事务:
```php
$pdo = new PDO(...);
try {
$pdo->beginTransaction();
// 执行多个数据库操作
$pdo->commit();
} catch (PDOException $e) {
$pdo->rollBack();
}
```
在事务块中执行的所有操作都会被视为一个原子单元。如果其中任何一个操作失败,整个事务将回滚,数据库状态将恢复到事务开始前的状态。
### 事务的应用场景
事务管理在以下场景中非常有用:
- **确保数据一致性**:当需要同时更新多个表或操作时,事务可以确保所有操作要么全部成功,要么全部失败,从而保证数据的一致性。
- **防止并发冲突**:事务的隔离性可以防止并发事务之间的冲突,例如当多个事务同时尝试更新同一行数据时。
- **提高性能**:在某些情况下,使用事务可以提高性能,因为数据库可以将多个操作合并为一个操作来执行。
0
0
相关推荐
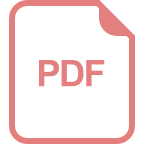
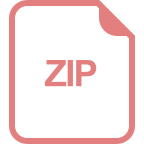
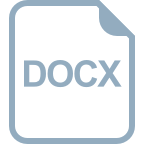
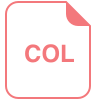
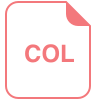
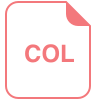
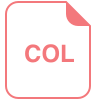
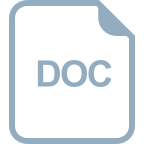
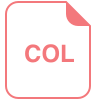