使用多线程_多进程加速爬虫数据处理过程
发布时间: 2024-04-16 12:00:09 阅读量: 8 订阅数: 16 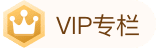
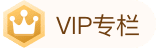
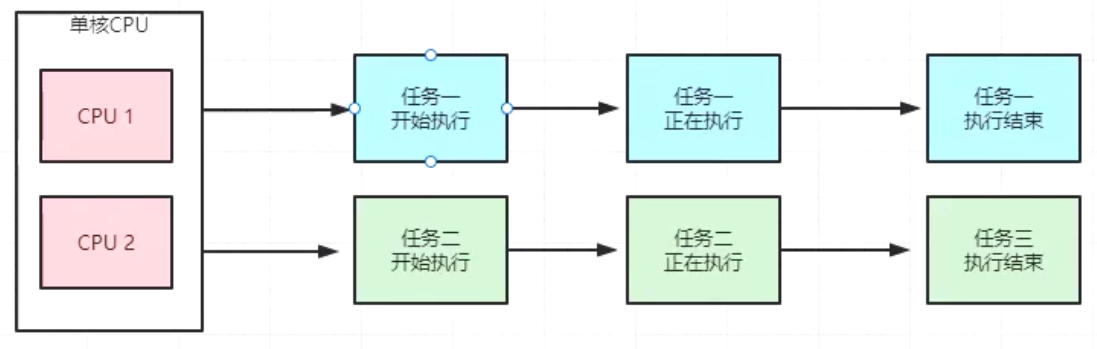
# 1. 理解多线程与多进程
在计算机领域,线程和进程是重要的概念。一个进程可以包含多个线程,各线程共享进程的资源,但拥有各自的执行流。线程轻量级且创建销毁速度快,能提高程序的效率。而进程拥有独立的内存空间,更为稳定可靠。
多线程适用于I/O密集型任务,能提高程序的响应速度。然而,多线程编程需要考虑线程安全和同步问题。多进程则适用于CPU密集型任务,通过多个进程并行处理数据,提高系统的整体性能。但进程之间通信相对复杂,需要考虑数据一致性与同步。
理解多线程与多进程的优势有助于选择合适的并发编程方式,提高程序的效率和性能。
# 2. Python 中的多线程编程
Python 中的多线程编程是利用线程在同一应用程序中并发执行代码,实现多个任务同时执行的目的。本章将重点介绍Python 中多线程的概念以及如何使用 threading 模块创建多线程。
#### 2.1 Python 中多线程概念
在 Python 中,多线程是指在同一进程中同时运行多个线程,每个线程都有自己的执行序列。然而,Python 的全局解释器锁(Global Interpreter Lock,GIL)会限制多线程效率。
##### 2.1.1 理解 Python GIL
GIL 是 Python 解释器自带的一个全局锁,限制同一时刻只有一个线程在解释器中运行。这会导致多线程在 CPU 密集型任务上无法充分利用多核 CPU 的优势。
```python
# 示例:演示 Python GIL 对多线程性能的影响
import threading
def count_up():
count = 0
while count < 10000000:
count += 1
# 创建两个线程
thread1 = threading.Thread(target=count_up)
thread2 = threading.Thread(target=count_up)
# 启动线程
thread1.start()
thread2.start()
# 等待两个线程结束
thread1.join()
thread2.join()
```
##### 2.1.2 Python 中多线程的局限性与解决方法
为了克服 GIL 的限制,可以使用更多线程的方式模拟并发,或者通过使用 multiprocessing 模块创建多个进程来实现并行化计算任务。
#### 2.2 使用 threading 模块创建多线程
在 Python 中,可以使用 threading 模块来创建和管理多线程,实现并发执行任务。
##### 2.2.1 线程的创建与启动
通过 threading 模块的 Thread 类可以创建新的线程,并通过 start() 方法启动线程。
```python
# 示例:使用 threading 模块创建并启动线程
import threading
def print_numbers():
for i in range(1, 6):
print(f"Number: {i}")
# 创建线程
thread = threading.Thread(target=print_numbers)
# 启动线程
thread.start()
```
##### 2.2.2 线程同步与通信
在多线程编程中,可能会涉及到多个线程之间的数据共享和通信。可以使用锁(Lock)、条件变量(Condition)、信号量(Semaphore)等机制实现线程同步。
```python
# 示例:使用锁实现线程同步
import threading
x = 0
lock = threading.Lock()
def increment():
global x
for _ in range(1000000):
lock.acquire()
x += 1
lock.release()
thread1 = threading.Thread(target=increment)
thread2 = threading.Thread(target=increment)
thread1.start()
thread2.start()
thread1.join()
thread2.join()
print(f"Final value of x: {x}")
```
##### 2.2.3 线程的关闭与管理
在 Python 中,可以通过设置线程的 daemon 属性将线程设置为守护线程,当主线程结束时守护线程也会随之结束。
```python
# 示例:设置线程为守护线程
import threading
import time
def count_down():
for i in range(5, 0, -1):
print(f"Counting down: {i}")
time.sleep(1)
thread = threading.Thread(target=count_down)
thread.daemon = True # 设置为守护线程
thread.start()
print("Main thread ends")
```
# 3. Python 中的多进程编程
3.1 Python 中多进程概念
多进程是一种并行处理的方式,允许同时运行多个任务,每个任务运行在独立的进程中。进程之间相互独立,拥有独立的内存空间,数据不共享。
0
0
相关推荐
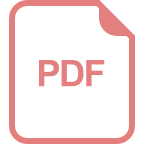
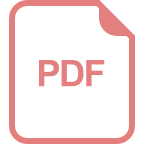
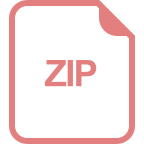





