Python爬虫进阶技巧:解锁高级功能,提升爬虫效能
发布时间: 2024-06-18 02:57:21 阅读量: 12 订阅数: 17 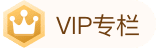
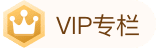
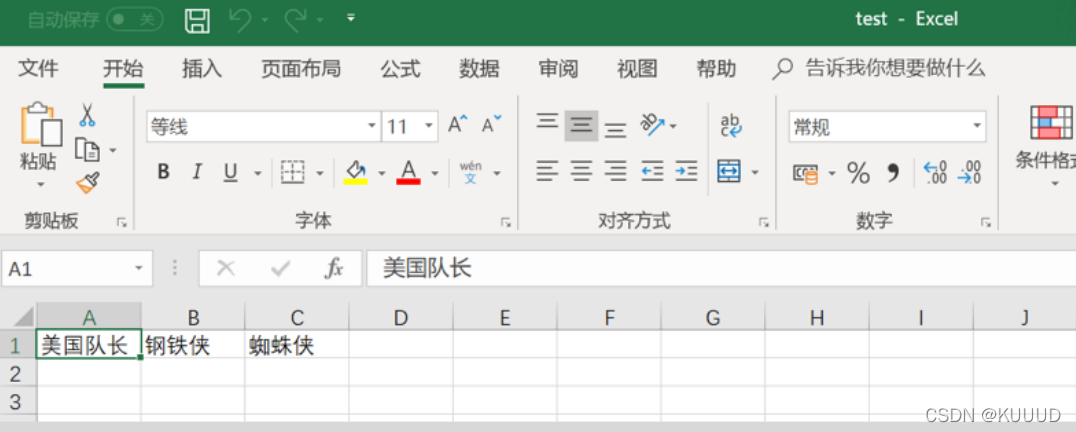
# 1. Python爬虫基础回顾**
**1.1 爬虫的基本原理**
- 理解爬虫的工作流程:请求、解析、存储
- 掌握HTTP协议和HTML结构
**1.2 爬虫工具库简介**
- 介绍Requests、BeautifulSoup等基本爬虫库
- 了解库的功能和使用场景
# 2. 高级爬虫技巧
### 2.1 并发和异步编程
#### 2.1.1 线程和进程
**线程**
* 线程是操作系统中的轻量级进程,与进程共享相同的内存空间。
* 线程创建和销毁成本低,可以提高程序的并发性。
* 在 Python 中,使用 `threading` 模块创建和管理线程。
**进程**
* 进程是操作系统中的独立执行单元,拥有自己的内存空间。
* 进程创建和销毁成本较高,但隔离性更好。
* 在 Python 中,使用 `multiprocessing` 模块创建和管理进程。
**示例代码:**
```python
import threading
def thread_function(arg):
print(f"Thread {threading.current_thread().name} is running with argument {arg}")
if __name__ == "__main__":
threads = []
for i in range(5):
thread = threading.Thread(target=thread_function, args=(i,))
threads.append(thread)
thread.start()
for thread in threads:
thread.join()
```
**逻辑分析:**
* 创建一个线程函数 `thread_function`,它打印线程名称和参数。
* 在主线程中创建 5 个线程,每个线程都运行 `thread_function`。
* 使用 `join()` 方法等待所有线程完成。
#### 2.1.2 协程和事件循环
**协程**
* 协程是一种轻量级的并发机制,允许函数暂停和恢复执行。
* 协程与线程类似,但更轻量级,并且不占用系统资源。
* 在 Python 中,使用 `async` 和 `await` 关键字创建和管理协程。
**事件循环**
* 事件循环是一个无限循环,它处理协程中的事件。
* 当协程暂停时,事件循环会执行其他协程,直到该协程可以继续执行。
* 在 Python 中,使用 `asyncio` 模块创建和管理事件循环。
**示例代码:**
```python
import asyncio
async def coroutine_function(arg):
print(f"Coroutine {arg} is running")
await asyncio.sleep(1)
print(f"Coroutine {arg} is done")
async def main():
tasks = [coroutine_function(i) for i in range(5)]
await asyncio.gather(*tasks)
if __name__ == "__main__":
asyncio.run(main())
```
**逻辑分析:**
* 创建一个协程函数 `coroutine_function`,它打印协程名称并暂停 1 秒。
* 在主协程 `main` 中创建 5 个协程任务。
* 使用 `asyncio.gather()` 同时执行所有协程。
* 使用 `asyncio.run()` 运行事件循环。
# 3. 数据处理与分析
### 3.1 数据清洗和转换
数据清洗和转换是数据处理中的关键步骤,它确保了数据的完整性、一致性和可分析性。
#### 3.1.1 数据类型转换
数据类型转换涉及将数据从一种数据类型转换为另一种数据类型。Python提供了多种内置函数来执行此操作,例如 `int()`, `float()`, `str()`, `bool()`。
```python
# 将字符串转换为整数
age = "25"
age_int = int(age)
print(age_int) # 输出:25
```
#### 3.1.2 数据标准化
数据标准化包括将数据转换为一致的格式,以便于比较和分析。这可能涉及删除重复项、标准化日期格式、处理空值等。
```python
import pandas as pd
# 创建一个包含重复值的数据框
df = pd.DataFrame({
"name": ["John", "Jane", "John", "Ja
```
0
0
相关推荐
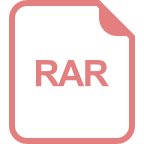
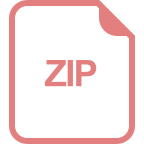
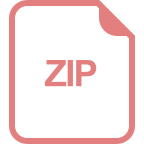





