如何选择合适的约束优化算法:全面对比算法优缺点
发布时间: 2024-08-26 20:31:31 阅读量: 16 订阅数: 17 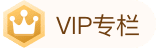
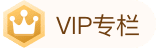
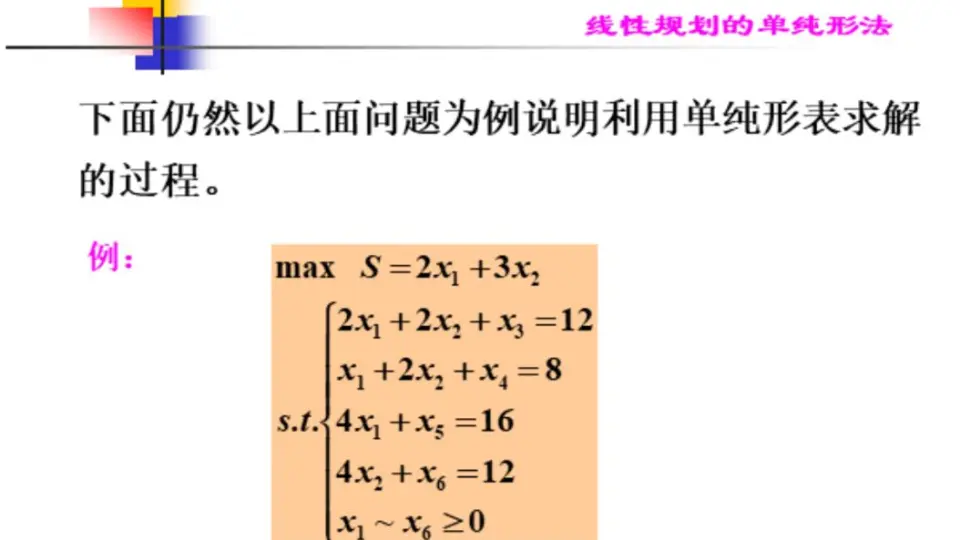
# 1. 约束优化算法概述
约束优化算法是一种用于解决具有约束条件的优化问题的数学方法。它在各个领域都有着广泛的应用,例如工程、经济学和运筹学。约束优化算法通过在满足约束条件的情况下,找到目标函数的最佳值。
约束优化问题通常可以表示为:
```
min/max f(x)
subject to:
g_i(x) <=/>= b_i, i = 1, ..., m
h_j(x) = b_j, j = 1, ..., p
```
其中:
* f(x) 是目标函数
* g_i(x) 和 h_j(x) 是约束函数
* b_i 和 b_j 是约束常数
# 2. 约束优化算法理论基础
约束优化算法理论基础是约束优化算法的基础,它为算法的实现和应用提供了理论支撑。本章节将介绍线性规划、非线性规划和整数规划的理论基础,为后续章节的算法实践和选择指南奠定基础。
### 2.1 线性规划
**2.1.1 线性规划模型**
线性规划(LP)是一种数学优化问题,其目标函数和约束条件都是线性的。线性规划模型可以表示为:
```
min/max f(x) = c^T x
s.t.
Ax ≤ b
x ≥ 0
```
其中:
* f(x) 是目标函数,需要最小化或最大化
* x 是决策变量向量
* c 是目标函数系数向量
* A 是约束矩阵
* b 是约束值向量
**2.1.2 线性规划算法**
解决线性规划问题的算法有很多,其中最著名的算法是单纯形法。单纯形法是一种迭代算法,通过不断寻找可行解和最优解之间的差异,逐步逼近最优解。
### 2.2 非线性规划
**2.2.1 非线性规划模型**
非线性规划(NLP)是一种数学优化问题,其目标函数或约束条件是非线性的。非线性规划模型可以表示为:
```
min/max f(x)
s.t.
g(x) ≤ 0
h(x) = 0
```
其中:
* f(x) 是目标函数,需要最小化或最大化
* x 是决策变量向量
* g(x) 是不等式约束条件
* h(x) 是等式约束条件
**2.2.2 非线性规划算法**
解决非线性规划问题的算法有很多,其中常用的算法包括:
* 梯度下降法
* 牛顿法
* 共轭梯度法
### 2.3 整数规划
**2.3.1 整数规划模型**
整数规划(IP)是一种数学优化问题,其决策变量必须取整数。整数规划模型可以表示为:
```
min/max f(x)
s.t.
Ax ≤ b
x ∈ Z^n
```
其中:
* f(x) 是目标函数,需要最小化或最大化
* x 是决策变量向量
* A 是约束矩阵
* b 是约束值向量
* Z^n 表示 n 维整数空间
**2.3.2 整数规划算法**
解决整数规划问题的算法有很多,其中常用的算法包括:
* 分支定界法
* 割平面法
# 3.1 线性规划算法实践
**3.1.1 Simplex算法**
Simplex算法是一种求解线性规划问题的经典算法,其基本思想是通过迭代的方式,不断寻找可行解并逐步优化目标函数。
**代码块:**
```python
import numpy as np
from scipy.optimize import linprog
# 定义线性规划模型
c = np.array([1, 2]) # 目标函数系数
A = np.array([[2, 1], [1, 2]]) # 约束矩阵
b = np.array([4, 6]) # 约束值
bounds = [(0, None), (0, None)] # 变量范围
# 求解线性规划问题
res = linprog(c, A_ub=A, b_ub=b, bounds=bounds)
# 输出求解结果
print("最优解:", res.x)
print("最优值:", res.fun)
```
**逻辑分析:**
* `linprog`函数用于求解线性规划问题,其参数包括目标函数系数、约束矩阵、约束值和变量范围。
* `bounds`参数指定变量的范围,`(0, None)`表示变量只能取非负值。
* `res`对象包含求解结果,包括最优解和最优值。
**3.1.2 内点法**
内点法是一种求解线性规划问题的现代算法,其基本思想是通过迭代的方式,保持可行解和最优解之间的接近度,逐步逼近最优解。
**代码块:**
```python
import numpy as np
from cvxopt import matrix, solvers
# 定义线性规划模型
c = np.array([1, 2]) # 目标函数系数
A = np.array([[2, 1], [1, 2]]) # 约束矩阵
b = np.array([4, 6]) # 约束值
# 转换为 CVXOPT 格式
c = matrix(c)
A = matrix(A)
b = matrix(b)
# 求解线性规划问题
solvers.lp(c, A, b)
# 输出求解结果
print("最优解:", sol.get_primal_dual()['x'])
print("最优值:", sol.get_primal_dual()['primal objective'])
```
**逻辑分析:**
* `cvxopt`库提供了求解线性规划问题的函数。
* `matrix`函数将 NumPy 数组转换为 CVXOPT 矩阵。
* `solvers.lp`函数求解线性规划问题,返回一个包含求解结果的对象。
* `get_primal_dual`方法获取求解结果,包括最优解和最优值。
# 4. 约束优化算法选择指南
### 4.1 算法选择原则
在选择约束优化算法时,需要考虑以下原则:
- **问题类型:**首先确定问题的类型,是线性规划、非线性规划还是整数规划。
- **问题规模:**考虑问题的规模,即变量和约束的数量。大规模问题可能需要专门的算法。
- **精度要求:**确定所需的解精度。某些算法可能提供更精确的解,但计算成本更高。
- **计算资源:**考虑可用的计算资源,包括时间和内存。
- **算法特性:**了解不同算法的特性,例如收敛速度、鲁棒性和易用性。
### 4.2 算法优缺点对比
下表总结了不同约束优化算法的优缺点:
| 算法 | 优点 | 缺点 |
|---|---|---|
| Simplex算法 | 适用于大规模线性规划问题 | 可能会出现数值不稳定性 |
| 内点法 | 适用于大规模线性规划问题,数值稳定性好 | 计算成本可能较高 |
| 梯度下降法 | 适用于非线性规划问题,收敛速度快 | 可能陷入局部最优 |
| 牛顿法 | 适用于非线性规划问题,收敛速度快 | 计算成本较高,可能出现数值不稳定性 |
| 分支定界法 | 适用于整数规划问题,保证找到全局最优解 | 计算成本较高 |
| 割平面法 | 适用于整数规划问题,收敛速度快 | 可能陷入局部最优 |
### 4.3 算法适用场景分析
根据不同的问题类型和特性,可以将约束优化算法应用于以下场景:
- **线性规划:**库存管理、生产调度、物流配送。
- **非线性规划:**工程设计、化学反应优化、金融投资优化。
- **整数规划:**人员排班、网络优化、设施选址。
例如,在生产调度优化中,线性规划算法(如 Simplex算法)可以用于分配资源和安排生产顺序,以最大化产量或最小化成本。在金融投资优化中,非线性规划算法(如梯度下降法)可以用于优化投资组合,以最大化收益或最小化风险。
# 5.1 生产调度优化
约束优化算法在生产调度优化中扮演着至关重要的角色,通过优化生产计划,提高产能利用率,降低生产成本。
**5.1.1 线性规划模型**
线性规划模型可以用来优化生产调度问题,其中决策变量表示生产数量,目标函数表示总生产成本,约束条件包括产能限制、需求限制和库存限制。
```python
import pulp
# 创建线性规划模型
model = pulp.LpProblem("生产调度优化", pulp.LpMinimize)
# 决策变量:生产数量
x1 = pulp.LpVariable("产品1数量", 0, None, pulp.LpInteger)
x2 = pulp.LpVariable("产品2数量", 0, None, pulp.LpInteger)
# 目标函数:总生产成本
model += 10 * x1 + 15 * x2
# 约束条件:产能限制
model += x1 + x2 <= 100
# 需求限制
model += x1 >= 50
model += x2 >= 30
# 库存限制
model += x1 <= 200
model += x2 <= 150
# 求解模型
model.solve()
```
**5.1.2 非线性规划模型**
当生产调度问题涉及非线性目标函数或约束条件时,需要采用非线性规划模型。例如,目标函数可以表示为产出与成本的非线性关系。
```python
import scipy.optimize
# 非线性规划模型
def objective_function(x):
return x[0]**2 + x[1]**2
# 约束条件
cons = ({'type': 'ineq', 'fun': lambda x: x[0] + x[1] - 1},
{'type': 'ineq', 'fun': lambda x: -x[0] + x[1] - 1})
# 求解模型
result = scipy.optimize.minimize(objective_function, [0, 0], constraints=cons)
```
0
0
相关推荐
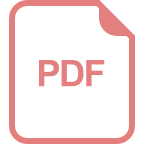
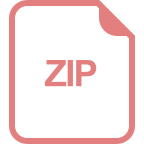
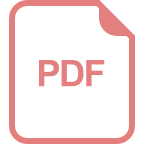





