PHP获取数据库ID的性能优化秘籍:提升效率,优化代码
发布时间: 2024-07-28 14:33:07 阅读量: 22 订阅数: 38 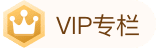
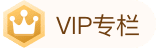
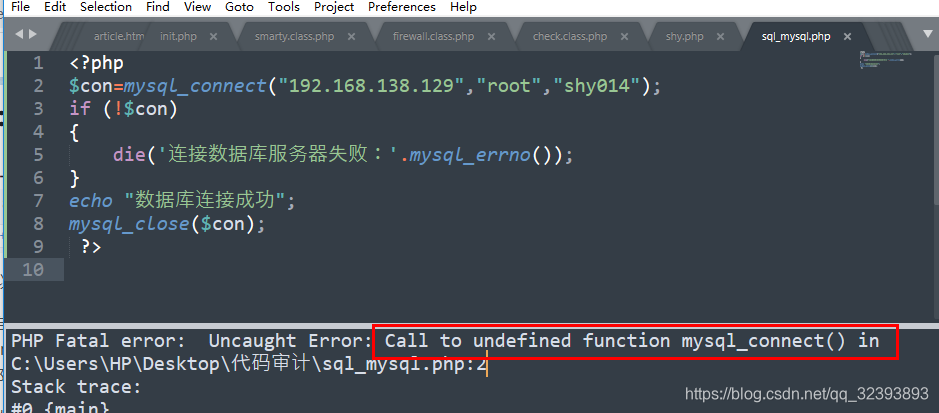
# 1. PHP数据库ID获取的性能瓶颈**
PHP中数据库ID获取的性能瓶颈主要源于以下几个方面:
- **频繁的数据库查询:**每次需要获取ID时都需要向数据库发送查询,这会消耗大量的数据库资源,尤其是当并发量较大时。
- **主键冲突:**数据库中的主键通常是自增的,这意味着在高并发场景下,可能会出现主键冲突,导致插入失败或数据不一致。
- **索引效率低下:**如果没有为ID字段创建适当的索引,数据库在查找ID时需要扫描整个表,这会严重影响查询性能。
# 2. PHP数据库ID获取的优化策略
### 2.1 缓存机制
缓存机制是一种将频繁访问的数据存储在快速访问的内存中,以避免每次从数据库中检索数据。这对于经常需要获取ID的场景非常有效。
#### 2.1.1 使用Redis缓存
Redis是一个开源的内存数据结构存储系统,以其高性能和低延迟而闻名。它非常适合缓存ID,因为ID通常是小型且经常访问的数据。
```php
// 使用Redis缓存ID
$redis = new Redis();
$redis->connect('127.0.0.1', 6379);
$id = $redis->get('product_id');
if (!$id) {
// 从数据库中获取ID
$id = $database->query('SELECT id FROM products WHERE name = "Product A"')->fetchColumn();
$redis->set('product_id', $id);
}
```
**参数说明:**
* `connect()`: 连接到Redis服务器。
* `get()`: 从Redis缓存中获取ID。
* `set()`: 将ID存储在Redis缓存中。
**代码逻辑分析:**
1. 连接到Redis服务器。
2. 从Redis缓存中获取ID。
3. 如果ID不存在,则从数据库中查询并存储在Redis缓存中。
4. 返回ID。
#### 2.1.2 使用Memcached缓存
Memcached也是一个开源的内存数据结构存储系统,具有类似于Redis的功能。它也适用于缓存ID。
```php
// 使用Memcached缓存ID
$memcached = new Memcached();
$memcached->addServer('127.0.0.1', 11211);
$id = $memcached->get('product_id');
if (!$id) {
// 从数据库中获取ID
$id = $database->query('SELECT id FROM products WHERE name = "Product A"')->fetchColumn();
$memcached->set('product_id', $id);
}
```
**参数说明:**
* `addServer()`: 添加Memcached服务器。
* `get()`: 从Memcached缓存中获取ID。
* `set()`: 将ID存储在Memcached缓存中。
**代码逻辑分析:**
1. 添加Memcached服务器。
2. 从Memcached缓存中获取ID。
3. 如果ID不存在,则从数据库中查询并存储在Memcached缓存中。
4. 返回ID。
### 2.2 索引优化
索引是数据库中一种特殊的数据结构,用于快速查找数据。为ID列创建索引可以显著提高获取ID的性能。
#### 2.2.1 创建主键索引
主键索引是数据库中的一种特殊索引,用于唯一标识表中的每一行。为ID列创建主键索引可以确保快速查找ID。
```sql
CREATE TABLE products (
id INT NOT NULL AUTO_INCREMENT,
name VARCHAR(255) NOT NULL,
PRIMARY KEY (id)
);
```
**参数说明:**
* `NOT NULL`: 指定列不能为NULL。
* `AUTO_INCREMENT`: 指定列的值自动递增。
* `PRIMARY KEY`: 指定主键索引。
**代码逻辑分析:**
1. 创建`products`表。
2. 将`id`列指定为主键索引。
3. 这将确保`id`列中的值唯一且自动递增。
#### 2.2.2 创建唯一索引
唯一索引是一种索引,确保表中每一行中的列值都是唯一的。为ID列创建唯一索引可以防止重复ID。
```sql
CREATE TABLE products (
id INT NOT NULL AUTO_INCREMENT,
name VARCHAR(255) NOT NULL,
UNIQUE INDEX (id)
);
```
**参数说明:**
* `NOT NULL`: 指定列不能为NULL。
* `AUTO_INCREMENT`: 指定列的值自动递增。
* `UNIQUE INDEX`: 指定唯一索引。
**代码逻辑分析:**
1. 创建`products`表。
2. 将`id`列指定为唯一索引。
3. 这将确保`id`列中的值唯一且自动递增。
### 2.3 查询优化
查询优化涉及优化SQL查询以提高性能。对于获取ID,可以使用以下技术进行优化:
#### 2.3.1 使用LIMIT子句
`LIMIT`子句用于限制查询返回的行数。这对于获取单个ID非常有用,因为它可以防止查询返回不必要的行。
```sql
SELECT id FROM products WHERE name = "Product A" LIMIT 1;
```
**参数说明:**
* `LIMIT 1`: 限制查询返回一行。
**代码逻辑分析:**
1. 查询`products`表。
2. 过滤结果以获取名称为“Product A”的产品。
3. 限制查询返回一行。
#### 2.3.2 使用JOIN优化
`JOIN`子句用于将来自多个表的行组合在一起。这对于获取与其他表相关联的ID非常有用。
```sql
SELECT p.id, o.order_id
FROM products AS p
JOIN orders AS o ON p.id = o.product_id
WHERE o.customer_id = 123;
```
**参数说明:**
* `JOIN`: 将`products`表(别名`p`)与`orders`表(别名`o`)连接。
* `ON`: 指定连接条件(`p.id = o.product_id`)。
* `WHERE`: 过滤结果以获取与客户ID为123的订单关联的产品ID。
**代码逻辑分析:**
1. 连接`products`表和`orders`表。
2. 过滤结果以获取与客户ID为123的订单关联的产品ID。
# 3. PHP数据库ID获取的实践案例
### 3.1 优化电商网站的产品ID获取
#### 3.1.1 使用Redis缓存优化
在电商网站中,产品ID是一个经常需要获取的关键信息。为了优化产品ID的获取性能,可以使用Redis缓存。
**步骤:**
1. 在Redis中设置一个哈希表,键为产品名称,值为产品ID。
2. 当需要获取产品ID时,先从Redis中查询。如果命中,直接返回产品ID。
3. 如果Redis中没有命中,则从数据库中查询产品ID,并将结果存入Redis中。
**代码块:**
```php
// 连接Redis
$redis = new Redis();
$redis->connect('127.0.0.1', 6379);
// 从Redis中获取产品ID
$productId = $redis->hGet('products', $productName);
// 如果Redis中没有命中,则从数据库中查询
if ($productId === false) {
// 从数据库中查询产品ID
$productId = $db->query("SELECT id FROM products WHERE name = '$productName'")->fetchColumn();
// 将结果存入Redis
$redis->hSet('products', $productName, $productId);
}
```
**逻辑分析:**
* 连接Redis并获取产品ID。
* 如果Redis中没有命中,则从数据库中查询并存入Redis。
* 返回产品ID。
#### 3.1.2 使用索引优化
除了使用缓存外,还可以通过优化数据库索引来提升产品ID的获取性能。
**步骤:**
1. 在产品表上创建主键索引。
2. 在产品名称字段上创建唯一索引。
**代码块:**
```sql
// 创建主键索引
ALTER TABLE products ADD PRIMARY KEY (id);
// 创建唯一索引
ALTER TABLE products ADD UNIQUE INDEX (name);
```
**逻辑分析:**
* 主键索引可以快速定位到指定的产品记录。
* 唯一索引可以防止插入重复的产品名称。
### 3.2 优化博客网站的评论ID获取
#### 3.2.1 使用Memcached缓存优化
在博客网站中,评论ID也是一个经常需要获取的信息。为了优化评论ID的获取性能,可以使用Memcached缓存。
**步骤:**
1. 在Memcached中设置一个哈希表,键为评论内容,值为评论ID。
2. 当需要获取评论ID时,先从Memcached中查询。如果命中,直接返回评论ID。
3. 如果Memcached中没有命中,则从数据库中查询评论ID,并将结果存入Memcached中。
**代码块:**
```php
// 连接Memcached
$memcached = new Memcached();
$memcached->addServer('127.0.0.1', 11211);
// 从Memcached中获取评论ID
$commentId = $memcached->get($commentContent);
// 如果Memcached中没有命中,则从数据库中查询
if ($commentId === false) {
// 从数据库中查询评论ID
$commentId = $db->query("SELECT id FROM comments WHERE content = '$commentContent'")->fetchColumn();
// 将结果存入Memcached
$memcached->set($commentContent, $commentId);
}
```
**逻辑分析:**
* 连接Memcached并获取评论ID。
* 如果Memcached中没有命中,则从数据库中查询并存入Memcached。
* 返回评论ID。
#### 3.2.2 使用JOIN优化
除了使用缓存外,还可以通过优化数据库查询来提升评论ID的获取性能。
**步骤:**
1. 使用JOIN查询将评论表与文章表连接起来。
2. 在JOIN查询中指定文章ID作为过滤条件。
**代码块:**
```sql
// 使用JOIN查询获取评论ID
$commentId = $db->query("SELECT comments.id FROM comments JOIN articles ON comments.article_id = articles.id WHERE articles.id = $articleId")->fetchColumn();
```
**逻辑分析:**
* JOIN查询将评论表与文章表连接起来,并过滤出指定文章ID的评论。
* 直接返回评论ID,避免了额外的查询。
# 4. PHP数据库ID获取的进阶优化
### 4.1 分布式ID生成
在高并发系统中,单一数据库服务器可能无法满足系统对ID生成的需求。此时,需要采用分布式ID生成机制来解决ID生成瓶颈。
#### 4.1.1 Snowflake算法
Snowflake算法是一种分布式ID生成算法,它将ID划分为多个字段,每个字段代表不同的信息,例如时间戳、机器ID和序列号。通过这种方式,可以保证ID的唯一性和有序性。
```php
// 使用雪花算法生成ID
$snowflake = new Snowflake(1, 1);
$id = $snowflake->generate();
// 解析ID
$timestamp = $id >> 22;
$machineId = ($id >> 12) & 0x3FF;
$sequence = $id & 0xFFF;
```
#### 4.1.2 UUID算法
UUID(Universally Unique Identifier)是一种通用唯一标识符算法,它生成一个128位的随机ID。UUID算法可以保证ID的唯一性,但它不具有顺序性。
```php
// 使用UUID算法生成ID
$uuid = UUID::uuid4();
// 解析UUID
$timestamp = $uuid->getTime();
$clockSeqAndNode = $uuid->getClockSeqAndNode();
```
### 4.2 数据库分片
当数据库数据量过大时,单一数据库服务器可能无法承受高并发访问的压力。此时,需要采用数据库分片技术来将数据分布到多个数据库服务器上。
#### 4.2.1 水平分片
水平分片是指将数据表中的数据按行进行分片,每个分片存储部分数据。水平分片可以有效降低单一数据库服务器的负载,提高系统并发处理能力。
#### 4.2.2 垂直分片
垂直分片是指将数据表中的数据按列进行分片,每个分片存储部分列的数据。垂直分片可以优化数据查询性能,因为只需要查询相关分片即可获取所需数据。
```
**水平分片示例:**
| 分片 | 数据范围 |
|---|---|
| 分片1 | 用户ID 1-10000 |
| 分片2 | 用户ID 10001-20000 |
| 分片3 | 用户ID 20001-30000 |
**垂直分片示例:**
| 分片 | 列 |
|---|---|
| 分片1 | 用户ID、用户名、邮箱 |
| 分片2 | 地址、电话号码、邮政编码 |
```
# 5.1 性能测试和监控
### 5.1.1 使用 Apache JMeter 进行性能测试
Apache JMeter 是一款开源的性能测试工具,可用于模拟大量并发用户对数据库进行 ID 获取操作。通过 JMeter,可以测试不同优化策略对 ID 获取性能的影响,并找出性能瓶颈。
**步骤:**
1. 安装 Apache JMeter。
2. 创建一个测试计划,包括线程组、HTTP 请求和断言。
3. 配置线程组,设置并发用户数和持续时间。
4. 配置 HTTP 请求,指定数据库 ID 获取的 URL。
5. 添加断言,验证 ID 获取的正确性。
6. 运行测试,收集性能数据。
### 5.1.2 使用 Prometheus 进行性能监控
Prometheus 是一款开源的监控系统,可用于监控数据库 ID 获取的性能指标。通过 Prometheus,可以实时监控 ID 获取的延迟、吞吐量和错误率等指标,并及时发现性能问题。
**步骤:**
1. 安装 Prometheus。
2. 配置 Prometheus 监控数据库 ID 获取的指标。
3. 安装 Grafana,用于可视化 Prometheus 收集的指标。
4. 配置 Grafana 仪表盘,显示 ID 获取的性能指标。
5. 定期监控仪表盘,及时发现性能异常。
通过性能测试和监控,可以持续跟踪数据库 ID 获取的性能,并及时发现和解决性能问题,确保 ID 获取的稳定性和高效性。
0
0
相关推荐
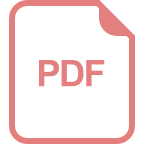
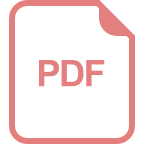
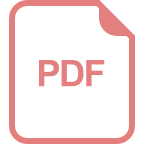
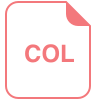
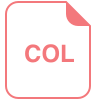
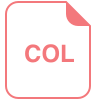
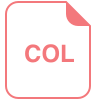
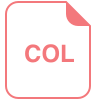
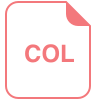