YOLO算法性能评估秘籍:全面衡量算法表现
发布时间: 2024-08-14 13:53:11 阅读量: 30 订阅数: 35 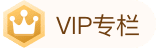
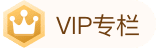
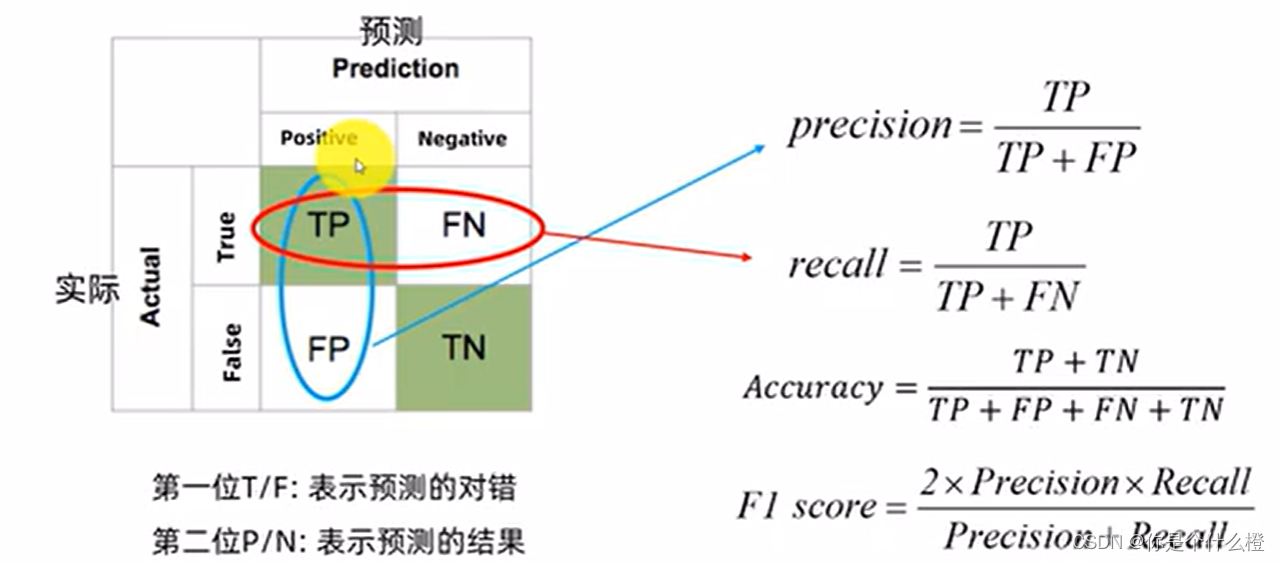
# 1. YOLO算法简介
**1.1 YOLO算法概述**
YOLO(You Only Look Once)算法是一种单次卷积神经网络(CNN),用于实时目标检测。它将目标检测任务视为回归问题,直接预测目标的边界框和类别概率。与传统的目标检测算法相比,YOLO算法具有速度快、精度高的特点。
**1.2 YOLO算法架构**
YOLO算法的架构主要包括以下组件:
- **主干网络:**用于提取图像特征,通常采用预训练的卷积神经网络,如ResNet或Darknet。
- **检测头:**负责预测目标的边界框和类别概率,由多个卷积层和全连接层组成。
- **损失函数:**衡量预测结果与真实标签之间的差异,指导模型的训练。
# 2. YOLO算法性能评估指标
### 2.1 定量指标
#### 2.1.1 平均精度(mAP)
平均精度(mAP)是YOLO算法性能评估中最常用的定量指标。它衡量算法在不同置信度阈值下的平均精度,反映了算法检测目标的准确性和召回率。
**计算公式:**
```
mAP = (AP_0.5 + AP_0.55 + ... + AP_0.95) / 10
```
其中,AP_x表示置信度阈值为x时的平均精度。
**代码实现:**
```python
import numpy as np
def calculate_mAP(gt_boxes, pred_boxes, iou_threshold=0.5):
"""计算平均精度(mAP)。
参数:
gt_boxes (list): 真实目标框列表。
pred_boxes (list): 预测目标框列表。
iou_threshold (float): 交并比阈值。
返回:
mAP (float): 平均精度。
"""
# 计算所有目标框的交并比
ious = np.array([iou(gt_box, pred_box) for gt_box in gt_boxes for pred_box in pred_boxes])
# 对于每个置信度阈值,计算平均精度
mAP = 0
for iou_threshold in np.arange(0.5, 1.0, 0.05):
tp, fp, fn = 0, 0, 0
for iou in ious:
if iou >= iou_threshold:
tp += 1
else:
fp += 1
fn = len(gt_boxes) - tp
precision = tp / (tp + fp)
recall = tp / (tp + fn)
AP = calculate_AP(precision, recall)
mAP += AP
return mAP / 10
def calculate_AP(precision, recall):
"""计算平均精度(AP)。
参数:
precision (list): 精度列表。
recall (list): 召回率列表。
返回:
AP (float): 平均精度。
"""
# 计算插值后的精度-召回率曲线
interpolated_precision = np.interp(np.linspace(0, 1, 11), recall, precision)
# 计算平均精度
AP = np.sum(interpolated_precision) / 11
return AP
```
#### 2.1.2 交并比(IoU)
交并比(IoU)衡量预测目标框与真实目标框的重叠程度。它用于确定预测是否正确。
**计算公式:**
```
IoU = (Area of Intersection) / (Area of Union)
```
其中,Area of Intersection表示预测目标框与真实目标框的重叠面积,Area of Union表示预测目标框与真实目标框的并集面积。
**代码实现:**
```python
def iou(box1, box2):
"""计算两个目标框的交并比。
参数:
box1 (list): 第一个目标框。
box2 (list): 第二个目标框。
返回:
iou (float): 交并比。
"""
# 获取两个目标框的坐标
x1, y1, x2, y2 = box1
x3, y3, x4, y4 = box2
# 计算交集面积
intersection_area = max(0, min(x2, x4) - max(x1, x3)) * max(0, min(y2, y4) - max(y1, y3))
# 计算并集面积
union_area = (x2 - x1) * (y2 - y1) + (x4 - x3) * (y4 - y3) - intersection_area
# 计算交并比
iou = intersection_area / union_area
return iou
```
### 2.2 定性指标
#### 2.2.1 可视化结果
可视化结果可以直观地展示YOLO算法的检测效果。它可以帮助我们识别算法的错误和改进方向。
**代码实现:**
```python
import matplotlib.pyplot as p
```
0
0
相关推荐
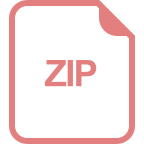
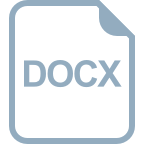
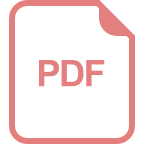





