Python字符串处理大全:从基础到进阶,玩转字符串
发布时间: 2024-06-25 15:23:07 阅读量: 91 订阅数: 48 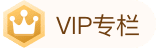
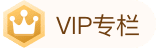
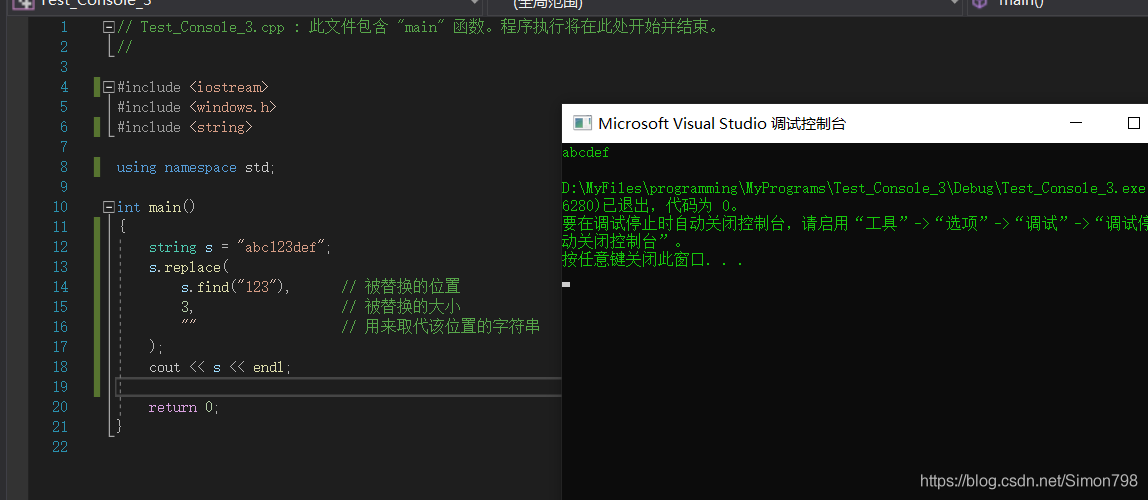
# 1. Python字符串处理基础
Python字符串是表示文本数据的不可变序列。它们提供了广泛的方法和函数来处理和操作文本数据。本节将介绍Python字符串处理的基础知识,包括字符串的创建、访问、拼接和切片。
### 1.1 字符串创建和访问
字符串可以使用单引号(')或双引号(")创建。字符串中的每个字符都表示为一个Unicode码点。可以使用方括号([])访问字符串中的单个字符,索引从0开始。
```python
my_string = 'Hello World'
first_character = my_string[0] # 'H'
```
# 2. Python字符串处理进阶技巧
### 2.1 字符串的切片和拼接
#### 2.1.1 切片操作
字符串切片是一种提取字符串中特定部分的方法。它使用方括号 [] 和冒号 : 运算符。语法如下:
```python
string[start:end:step]
```
* `start`:切片的起始索引(包含)
* `end`:切片的结束索引(不包含)
* `step`:切片步长(默认为 1)
**示例:**
```python
string = "Hello, world!"
# 从索引 0 开始,到索引 5 结束,步长为 1
substring = string[0:5] # 'Hello'
# 从索引 6 开始,到结尾,步长为 2
substring = string[6::2] # 'olr!'
```
#### 2.1.2 拼接操作
字符串拼接是将两个或多个字符串连接在一起。可以使用加号 (+) 运算符或 `join()` 方法。
**加号拼接:**
```python
string1 = "Hello"
string2 = "world!"
new_string = string1 + string2 # 'Helloworld!'
```
**`join()` 拼接:**
```python
separator = "-"
new_string = separator.join([string1, string2]) # 'Hello-world!'
```
`join()` 方法将一个可迭代对象(如列表或元组)中的元素连接在一起,使用指定的分隔符。
### 2.2 字符串的查找和替换
#### 2.2.1 `find()` 和 `rfind()` 函数
`find()` 和 `rfind()` 函数用于在字符串中查找子字符串。
* `find(substring)`:从字符串的开头开始查找子字符串,返回其第一个匹配项的索引。如果未找到,返回 -1。
* `rfind(substring)`:从字符串的末尾开始查找子字符串,返回其最后一个匹配项的索引。如果未找到,返回 -1。
**示例:**
```python
string = "Hello, world!"
index = string.find("world") # 7
index = string.rfind("world") # 7
```
#### 2.2.2 `replace()` 和 `sub()` 函数
`replace()` 和 `sub()` 函数用于在字符串中替换子字符串。
* `replace(old, new)`:将字符串中的所有 `old` 子字符串替换为 `new` 子字符串。
* `sub(pattern, repl, count)`:使用正则表达式 `pattern` 匹配字符串,并将匹配项替换为 `repl`。`count` 指定替换的最大次数(默认为所有匹配项)。
**示例:**
```python
string = "Hello, world!"
new_string = string.replace("world", "Python") # 'Hello, Python!'
new_string = string.sub(r"(\w+), (\w+)", r"\2, \1", 1) # 'world, Hello!'
```
### 2.3 字符串的格式化和转换
#### 2.3.1 格式化字符串
字符串格式化允许将变量嵌入到字符串中。使用 `f-strings` 或 `str.format()` 方法。
**`f-strings`:**
```python
name = "John"
age = 30
formatted_string = f"Hello, {name}! You are {age} years old."
```
**`str.format()`:**
```python
formatted_string = "Hello, {}! You are {} years old.".format(name, age)
```
#### 2.3.2 转换字符串
字符串转换将字符串从一种数据类型转换为另一种数据类型。
* **转换为数字:** `int()`, `float()`, `complex()`
* **转换为布尔值:** `bool()`
* **转换为列表:** `list()`
* **转换为元组:** `tuple()`
* **转换为字典:** `dict()`
**示例:**
```pytho
```
0
0
相关推荐







