单片机C语言中断与实时系统:深入理解单片机实时响应机制,3个实战案例
发布时间: 2024-07-10 08:18:22 阅读量: 68 订阅数: 30 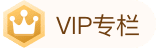
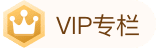
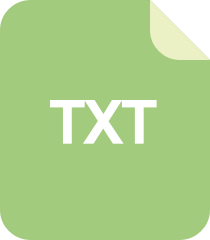
单片机C语言Proteus仿真实例按键识别
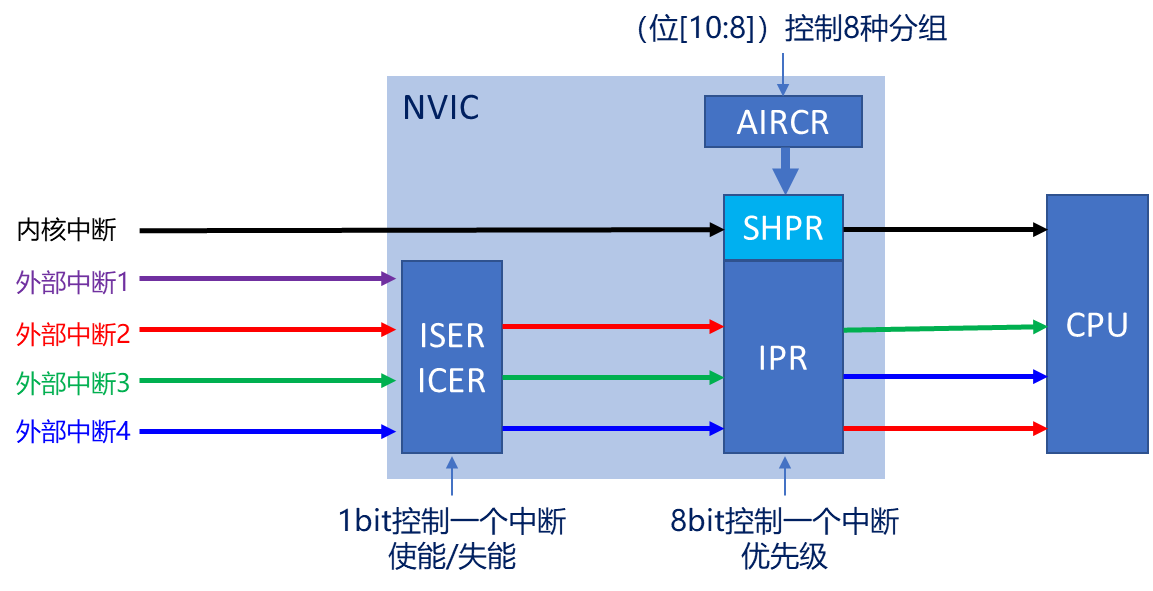
# 1. 单片机C语言中断基础**
中断是一种硬件机制,当发生特定事件时,它会暂停当前正在执行的程序并跳转到一个特定的函数(称为中断服务程序)来处理该事件。在单片机C语言编程中,中断机制至关重要,因为它允许系统在不中断主程序执行的情况下响应外部事件。
本章将介绍单片机C语言中断的基础知识,包括中断的概念、中断类型、中断服务程序的编写以及中断优先级和嵌套。通过对这些基础知识的理解,读者可以掌握中断机制的使用,为开发更复杂、更可靠的单片机系统奠定基础。
# 2.1 中断服务程序的编写
### 中断服务程序的基本结构
中断服务程序(ISR)是响应中断请求而执行的代码段。其基本结构如下:
```c
void ISR_name() interrupt [interrupt_number]
{
// 中断处理代码
}
```
其中:
* `ISR_name`:中断服务程序的名称,由用户自定义。
* `interrupt_number`:中断号,表示中断源。
* `中断处理代码`:响应中断请求时执行的代码。
### 中断服务程序的编写原则
编写中断服务程序时,应遵循以下原则:
* **简洁高效:**中断服务程序应尽可能简洁,避免执行耗时的操作。
* **原子性:**中断服务程序应是原子的,即不可被其他中断打断。
* **保存和恢复寄存器:**中断服务程序应保存和恢复受中断影响的寄存器,以保证程序的正确执行。
* **避免死锁:**中断服务程序中应避免死锁,如等待一个被其他中断锁定的资源。
### 中断服务程序的示例
以下是一个中断服务程序的示例,用于响应定时器 0 中断:
```c
void ISR_Timer0() interrupt 1
{
// 清除定时器 0 中断标志位
TF0 = 0;
// 执行中断处理代码
// ...
}
```
在该示例中,中断服务程序 ISR_Timer0() 响应定时器 0 中断(中断号为 1)。它首先清除定时器 0 中断标志位,然后执行中断处理代码。
# 3.1 实时系统的概念和特点
**3.1.1 实时系统的概念**
实时系统是一种计算机系统,其正确性不仅取决于其逻辑功能的正确性,还取决于其对时间约束的满足。换句话说,实时系统必须在指定的时间内对外部事件做出响应,否则将导致系统故障。
**3.1.2 实时系统的特点**
实时系统具有以下几个特点:
- **时间确定性:**实时系统必须能够在指定的时间内对外部事件做出响应,否则将导致系统故障。
- **可靠性:**实时系统必须高度可靠,因为任何故障都可能导致系统故障。
- **并发性:**实时系统通常需要处理多个并发事件,因此必须能够同时处理多个任务。
- **可预测性:**实时系统必须能够预测其行为,以便能够设计出满足时间约束的系统。
### 3.2 实时操作系统的结构和功能
**3.2.1 实时操作系统的结构**
实时操作系统(RTOS)是一种专门为实时系统设计的操作系统。RTOS通常由以下几个组件组成:
- **内核:**内核是RTOS的核心组件,负责管理系统资源和调度任务。
- **任务调度器:**任务调度器负责根据任务的优先级和时间约束调度任务。
- **中断处理程序:**中断处理程序负责处理外部事件并将其转换为任务。
- **内存管理单元:**内存管理单元负责管理系统内存。
- **文件系统:**文件系统负责管理系统文件。
**3.2.2 实时操作系统的功能**
RTOS提供以下功能:
- **任务管理:**RTOS负责创建、管理和调度任务。
- **中断处理:**RTOS负责处理外部事件并将其转换为任务。
- **内存管理:**RTOS负责管理系统内存。
- **文件系统管理:**RTOS负责管理系统文件。
- **通信管理:**RTOS负责管理系统中的通信。
### 3.3 实时任务调度算法
**3.3.1 实时任务调度算法的分类**
实时任务调度算法可分为以下几类:
- **非抢占式调度算法:**非抢占式调度算法不会中断正在运行的任务,直到任务完成或阻塞。
- **抢占式调度算法:**抢占式调度算法可以中断正在运行的任务,以调度具有更高优先级的任务。
**3.3.2 常用的实时任务调度算法**
以下是一些常用的实时任务调度算法:
- **先到先服务(FCFS):**FCFS是一种非抢占式调度算法,它根据任务到达的时间调度任务。
- **最近最少使用(LRU):**LRU是一种非抢占式调度算法,它根据任务最近使用的时间调度任务。
- **最短作业优先(SJF):**SJF是一种抢占式调度算法,它根据任务的执行时间调度任务。
- **最短剩余时间优先(SRTF):**SRTF是一种抢占式调度算法,它根据任务剩余的执行时间调度任务。
- **速率单调调度(RMS):**RMS是一种抢占式调度算法,它专门为具有周期性任务的实时系统设计。
# 4. 单片机C语言实时系统实践
### 4.1 实时任务的创建和管理
在实时系统中,任务是执行特定功能的独立实体。任务的创建和管理对于确保系统的实时性至关重要。
**任务创建**
在单片机C语言中,可以通过调用 `os_task_create()` 函数创建任务。该函数需要指定任务的以下属性:
- 任务名称
0
0
相关推荐
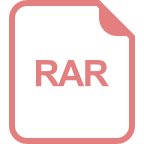
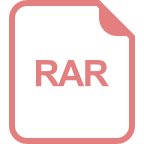
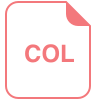
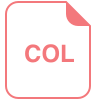
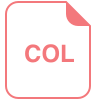
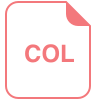
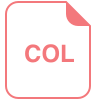
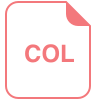