单片机C语言I2C通信:10个深入解析I2C总线的原理与应用的实战案例
发布时间: 2024-07-06 13:44:24 阅读量: 168 订阅数: 25 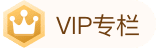
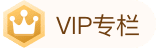
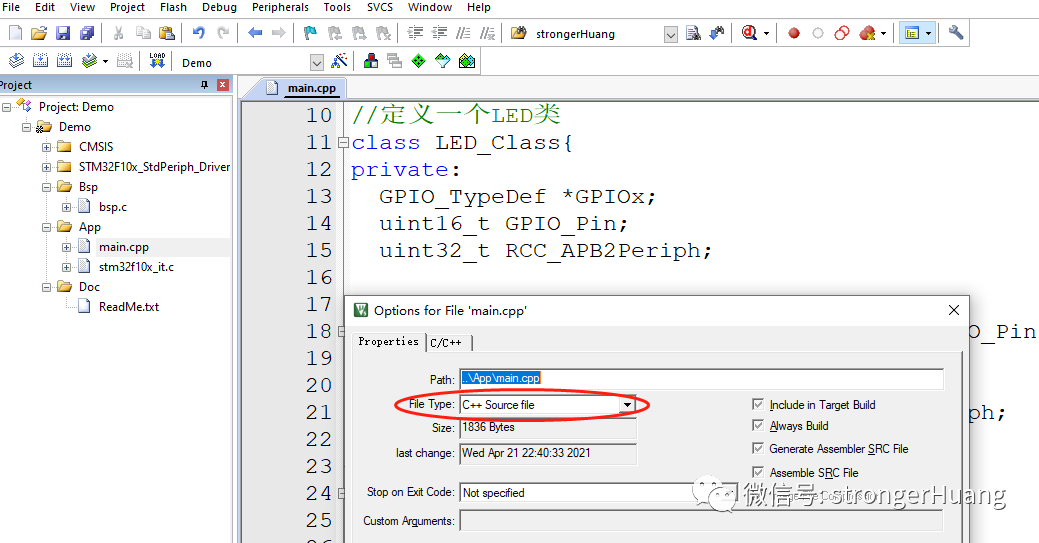
# 1. 单片机C语言I2C通信概述
I2C(Inter-Integrated Circuit)是一种串行通信协议,广泛应用于单片机、微控制器和各种外围设备之间的数据交换。它具有布线简单、成本低廉、易于实现等优点,在嵌入式系统中得到了广泛应用。
本章将介绍I2C通信的基本概念、通信原理和硬件接口,为后续的单片机C语言I2C通信编程实践奠定基础。
# 2. I2C总线原理深入解析
### 2.1 I2C总线协议和通信机制
#### 2.1.1 I2C总线通信流程
I2C总线采用主从式通信模式,通信流程如下:
1. **起始信号(Start):** 主设备发送起始信号,表示通信开始。
2. **从机地址(Slave Address):** 主设备发送从机地址,指定要通信的从机。
3. **读/写位(R/W):** 主设备发送读/写位,表示要进行读操作还是写操作。
4. **应答(Acknowledge):** 从机收到地址和读/写位后,发送应答信号,表示已收到并准备通信。
5. **数据传输:** 主设备和从机交换数据,主设备可以向从机发送数据(写操作),也可以从从机读取数据(读操作)。
6. **停止信号(Stop):** 主设备发送停止信号,表示通信结束。
#### 2.1.2 I2C总线数据格式
I2C总线数据格式为 8 位,包括:
- **起始位(Start Bit):** 低电平,表示通信开始。
- **从机地址(7 位):** 指定要通信的从机地址。
- **读/写位(1 位):** 0 表示写操作,1 表示读操作。
- **应答位(1 位):** 从机发送应答信号,表示已收到并准备通信。
- **数据(8 位):** 主设备和从机交换的数据。
- **停止位(Stop Bit):** 高电平,表示通信结束。
### 2.2 I2C总线硬件接口和电路设计
#### 2.2.1 I2C总线物理层接口
I2C总线采用两线制接口,包括:
- **SCL(时钟线):** 主设备控制时钟信号,从机跟随时钟信号进行通信。
- **SDA(数据线):** 主设备和从机交换数据。
#### 2.2.2 I2C总线驱动电路和器件选择
I2C总线驱动电路需要满足以下要求:
- **开漏输出:** 允许多个器件连接到同一总线上。
- **上拉电阻:** 将总线拉高至高电平。
- **速率控制:** 控制总线通信速率。
常用的 I2C总线驱动器件包括:
- **PCF8574:** I2C总线缓冲器,提供开漏输出和上拉电阻。
- **MAX3000:** I2C总线扩展器,支持多主设备通信。
- **PCA9548:** I2C总线多路复用器,允许多个从机连接到同一总线上。
# 3. 单片机C语言I2C通信编程实践
### 3.1 I2C总线通信初始化和配置
在进行I2C总线通信之前,需要对I2C总线进行初始化和配置,主要包括时钟频率设置和地址分配。
#### 3.1.1 I2C总线时钟频率设置
I2C总线通信速率由时钟频率决定,不同的应用场景对时钟频率有不同的要求。一般情况下,时钟频率越高,通信速度越快,但功耗也越大。常用的I2C总线时钟频率范围为100kbps~400kbps。
在单片机中,I2C总线时钟频率通常通过设置I2C总线控制寄存器中的时钟分频系数来实现。例如,在STM32系列单片机中,可以通过设置I2C_CR2寄存器的PRESC[3:0]位来设置时钟分频系数。
```c
// 设置I2C总线时钟频率为100kbps
I2C_CR2 |= (100000 / I2C_PCLK1_FREQ) << 8;
```
#### 3.1.2 I2C总线地址分配
I2C总线上的每个设备都有一个唯一的7位或10位地址。在初始化阶段,需要为单片机分配一个地址,以便其他设备可以识别和访问它。
在单片机中,I2C总线地址通常通过设置I2C总线控制寄存器中的OWNADDR[6:0]位来分配。例如,在STM32系列单片机中,可以通过设置I2C_OAR1寄存器的ADD0[6:0]位来设置自己的地址。
```c
// 设置单片机I2C总线地址为0x5A
I2C_OAR1 |= 0x5A;
```
### 3.2 I2C总线数据读写操作
I2C总线数据读写操作分为主设备读写和从设备读写两种情况。
#### 3.2.1 I2C总线主设备读写操作
主设备读写操作是指主设备向从设备发送或接收数据。主设备读写操作流程如下:
1. 主设备发送起始信号。
2. 主设备发送从设备地址和读/写标志位。
3. 从设备响应。
4. 主设备发送或接收数据。
5. 主设备发送停止信号。
在单片机中,I2C总线主设备读写操作通常通过调用HAL库函数实现。例如,在STM32系列单片机中,可以使用HAL_I2C_Master_Transmit和HAL_I2C_Master_Receive函数进行主设备读写操作。
```c
// 主设备向从设备地址为0x5A的寄存器0x10写入数据0xAB
uint8_t data = 0xAB;
HAL_I2C_Master_Transmit(&hi2c, 0x5A, &data, 1, 100);
// 主设备从从设备地址为0x5A的寄存器0x10读取数据
uint8_t data;
HAL_I2C_Master_Receive(&hi2c, 0x5A, &data, 1, 100);
```
#### 3.2.2 I2C总线从设备读写操作
从设备读写操作是指从设备向主设备发送或接收数据。从设备读写操作流程如下:
1. 从设备接收起始信号。
2. 从设备接收自己的地址和读/写标志位。
3. 从设备响应。
4. 从设备发送或接收数据。
5. 从设备接收停止信号。
在单片机中,I2C总线从设备读写操作通常通过调用HAL库函数实现。例如,在STM32系列单片机中,可以使用
0
0
相关推荐
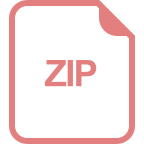





