单片机C语言函数进阶:10个函数指针、可变参数、递归的实战解析
发布时间: 2024-07-06 13:31:27 阅读量: 88 订阅数: 42 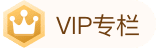
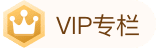
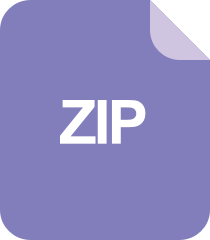
YOLO算法-城市电杆数据集-496张图像带标签-电杆.zip
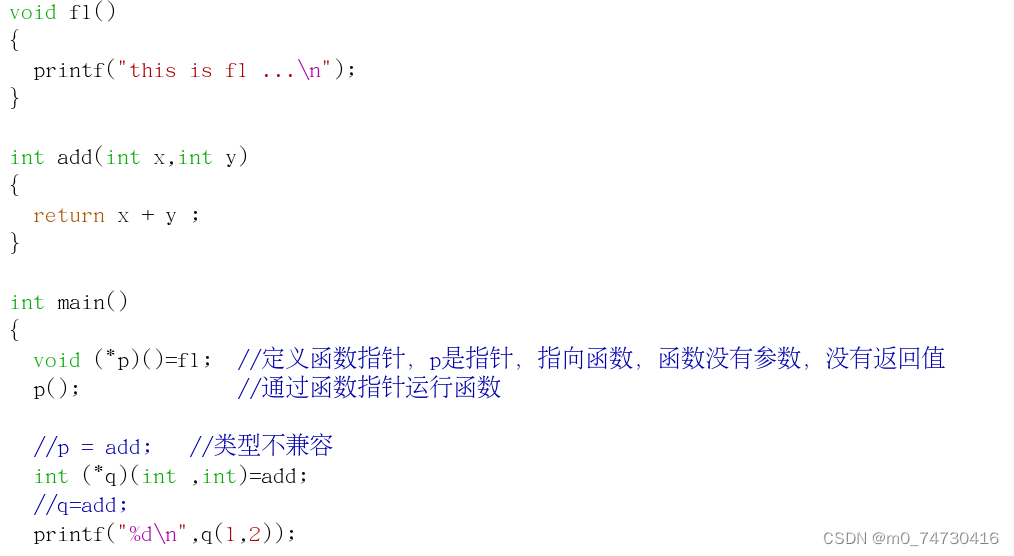
# 1. 单片机C语言函数进阶概述
单片机C语言函数进阶是学习单片机C语言的重要内容,它不仅能够提高代码的可读性、可维护性,而且能够提升代码的执行效率。本章将深入探讨单片机C语言函数的进阶知识,包括函数指针、可变参数函数和递归函数,并结合实战案例进行讲解,帮助读者全面掌握单片机C语言函数的进阶应用。
# 2. 函数指针的深入解析
### 2.1 函数指针的定义和类型
函数指针是一种指向函数的指针变量。它存储的是函数的地址,而不是函数本身。函数指针的类型与它所指向的函数的类型相同。
在 C 语言中,函数指针的语法如下:
```
<返回类型> (*函数指针名)(<参数列表>);
```
例如:
```
int (*func_ptr)(int, int);
```
这个函数指针指向一个接收两个 int 参数并返回一个 int 的函数。
### 2.2 函数指针的传递和使用
函数指针可以像普通指针一样传递和使用。
传递函数指针:
```
void pass_func_ptr(int (*func_ptr)(int, int)) {
// 使用函数指针
}
```
使用函数指针:
```
int result = func_ptr(10, 20);
```
### 2.3 函数指针的应用场景
函数指针在许多场景中都有应用,包括:
- **回调函数:**将函数指针作为参数传递给另一个函数,以便在特定事件发生时调用。
- **事件处理:**使用函数指针注册事件处理程序,以便在事件发生时执行特定的操作。
- **动态函数调用:**通过函数指针动态调用函数,实现代码的灵活性和可扩展性。
- **数据结构:**使用函数指针作为数据结构中的成员,实现复杂的数据处理和算法。
#### 代码示例:回调函数
```c
#include <stdio.h>
// 回调函数
void print_number(int num) {
printf("%d\n", num);
}
// 使用回调函数
void pass_callback(int (*callback)(int)) {
callback(10);
}
int main() {
// 传递回调函数
pass_callback(print_number);
return 0;
}
```
#### 代码逻辑分析
- `print_number` 函数是一个回调函数,它接收一个 int 参数并打印该数字。
- `pass_callback` 函数接收一个回调函数指针,并调用该函数指针传递给它的参数。
- 在 `main` 函数中,将 `print_number` 函数的地址传递给 `pass_callback` 函数。
- `pass_callback` 函数调用 `print_number` 函数,并打印数字 10。
#### 参数说明
- `callback`:指向回调函数的函数指针。
# 3. 可变参数函数的实战应用
### 3.1 可变参数函数的语法和特点
可变参数函数是一种允许函数接受数量可变的参数的函数。在 C 语言中,可变参数函数的语法如下:
```c
int printf(const char *format, ...);
```
其中,`format` 参数是一个格式化字符串,指定输出的格式。`...` 表示可变参数列表,可以传递任意数量的参数。
可变参数函数的特点如下:
* 可接受数量可变的参数。
* 可变参数列表必须放在参数列表的最后。
* 可变参数列表中的参数类型必须相同。
### 3.2 可变参数函数的实现原理
可变参数函数的实现原理是利用可变参数列表的地址。在函数调用时,可变参数列表的地址被压入堆栈。函数内部通过一个特殊的指针(通常称为 `va_list`)来访问可变参数列表。
### 3.3 可变参数函数的应用实例
可变参数函数在实际应用中非常广泛,下面列举几个常见的应用实例:
* **日志记录:** 可变参数函数可以方便地记录日志信息,例如:
```c
void log(const char *format, ...)
{
va_list args;
va_start(args, format);
vprintf(format, args);
va_end(args);
}
```
* **错误处理:** 可变参数函数可以方便地输出错误信息,例如:
```c
void error(const char *format, ...)
{
va_list args;
va_start(args, format);
vfprintf(stderr, format, args);
va_end(args);
}
```
* **数据处理:** 可变参数函数可以方便地处理数量可变的数据,例如:
```c
int sum(int count, ...)
{
va_list args;
va_start(args, count);
int sum = 0;
for (int i = 0; i < count; i++) {
sum += va_arg(args, int);
}
va_end(args);
return sum;
}
```
### 3.3.1 可变参数函数的代码示例
以下是一个可变参数函数的代码示例:
```c
#include <stdarg.h>
#include <stdio.h>
// 可变参数求和函数
int sum(int count, ...)
{
va_list args;
va_start(args, count);
int sum
```
0
0
相关推荐
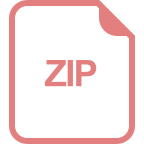
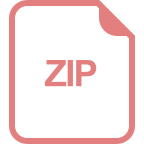
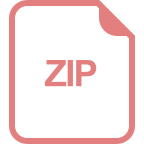
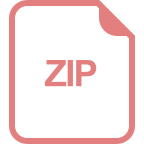