单片机C语言指针详解:15个深入理解指针本质与应用的实战案例
发布时间: 2024-07-06 13:24:34 阅读量: 67 订阅数: 25 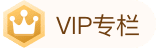
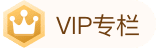
# 1. 指针基础
指针是一种特殊变量,它存储另一个变量的地址。指针变量的类型由它所指向的变量的类型决定。指针变量可以用于访问和修改其他变量的值,而无需直接引用变量本身。
指针变量的声明语法如下:
```c
<数据类型> *<指针变量名>;
```
例如,声明一个指向整数变量的指针变量:
```c
int *ptr;
```
# 2.1 指针变量的定义和初始化
### 2.1.1 指针变量的声明
在 C 语言中,指针变量用于存储另一个变量的地址。指针变量的声明语法如下:
```c
数据类型 *指针变量名;
```
其中,`数据类型` 是指针指向的变量的数据类型,`指针变量名` 是指针变量的名称。例如:
```c
int *ptr;
char *str;
```
### 2.1.2 指针变量的赋值
指针变量可以通过取地址运算符 `&` 获取变量的地址,也可以通过解引用运算符 `*` 访问指针指向的变量。
**取地址运算符 `&`**
`&` 运算符用于获取变量的地址。语法如下:
```c
&变量名
```
例如:
```c
int num = 10;
int *ptr = #
```
此时,`ptr` 指向变量 `num` 的地址。
**解引用运算符 `*`**
`*` 运算符用于访问指针指向的变量。语法如下:
```c
*指针变量名
```
例如:
```c
int num = 10;
int *ptr = #
int value = *ptr;
```
此时,`value` 的值为 10,因为 `ptr` 指向变量 `num`,`*ptr` 等同于 `num`。
# 3. 指针在单片机C语言中的应用
### 3.1 指针与数组
#### 3.1.1 指针访问数组元素
在单片机C语言中,数组名本身就是一个常量指针,指向数组首元素的地址。因此,我们可以使用指针运算符(*)来解引用数组名,访问数组中的元素。
```c
int arr[] = {1, 2, 3, 4, 5};
int *ptr = arr;
// 访问数组元素
printf("arr[0] = %d\n", arr[0]); // 输出 1
printf("*(ptr + 0) = %d\n", *(ptr + 0)); // 输出 1
```
#### 3.1.2 数组名作为指针
数组名作为指针时,其类型为指向数组首元素类型的指针。因此,我们可以将数组名直接赋值给一个指针变量。
```c
int arr[] = {1, 2, 3, 4, 5};
int *ptr = arr;
// arr 名作为指针赋值给 ptr
ptr = arr;
// 访问数组元素
printf("arr[0] = %d\n", arr[0]); // 输出 1
printf("*(ptr + 0) = %d\n", *(ptr + 0)); // 输出 1
```
### 3.2 指针与函数
#### 3.2.1 函数参数传递
在单片机C语言中,函数参数可以按值传递或按引用传递。按值传递会将参数值复制一份传递给函数,而按引用传递会将参数的地址传递给函数。
```c
// 按值传递
void swap_by_value(int a, int b) {
int temp = a;
a = b;
b = temp;
}
// 按引用传递
void swap_by_reference(int *a, int *b) {
int temp = *a;
*a = *b;
*b = temp;
}
int main() {
int x = 1, y = 2;
// 按值传递
swap_by_value(x, y);
printf("x = %d, y = %d\n", x, y); // 输出 x = 1, y = 2
// 按引用传递
swap_by_reference(&x, &y);
printf("x = %d, y = %d\n", x, y); // 输出 x = 2, y = 1
}
```
#### 3.2.2 函数返回值
函数的返回值也可以是按值传递或按引用传递。按值传递会将返回值复制一份返回给调用函数,而按引用传递会将返回值的地址返回给调用函数。
```c
// 按值传递
int get_max_by_value(int a, int b) {
if (a > b) {
return a;
} else {
return b;
}
}
// 按引用传递
int *get_max_by_reference(int *a, int *b) {
if (*a > *b) {
return a;
} else {
return b;
}
}
int main() {
int x = 1, y = 2;
// 按值传递
int max = get_max_by_value(x, y);
printf("max = %d\n", max); // 输出 max = 2
// 按引用传递
int *max_ptr = get_max_by_reference(&x, &y);
printf("max = %d\n", *max_ptr); // 输出 max = 2
}
```
# 4.1 指针与结构体
### 4.1.1 指针访问结构体成员
结构体是一种数据类型,它允许我们将相关数据项组合在一起。指针可以用来访问结构体的成员,就像访问数组元素一样。
```c
struct student {
int id;
char name[20];
float gpa;
};
int main() {
struct student s1;
s1.id = 12345;
strcpy(s1.name, "John Doe");
s1.gpa = 3.5;
// 使用指针访问结构体成员
struct student *ptr = &s1;
printf("Student ID: %d\n", ptr->id);
printf("Student Name: %s\n", ptr->name);
printf("Student GPA: %.2f\n", ptr->gpa);
return 0;
}
```
**逻辑分析:**
* `struct student` 定义了一个名为 `student` 的结构体,其中包含三个成员:`id`、`name` 和 `gpa`。
* `s1` 是 `student` 结构体的实例。
* `ptr` 是一个指向 `s1` 的指针。
* `ptr->id`、`ptr->name` 和 `ptr->gpa` 使用指针运算符 `->` 访问 `student` 结构体的成员。
### 4.1.2 结构体数组的遍历
指针可以用来遍历结构体数组。
```c
struct student {
int id;
char name[20];
float gpa;
};
int main() {
struct student students[] = {
{12345, "John Doe", 3.5},
{23456, "Jane Smith", 4.0},
{34567, "Bob Jones", 3.2}
};
// 使用指针遍历结构体数组
struct student *ptr = students;
for (int i = 0; i < 3; i++) {
printf("Student ID: %d\n", ptr->id);
printf("Student Name: %s\n", ptr->name);
printf("Student GPA: %.2f\n\n", ptr->gpa);
ptr++;
}
return 0;
}
```
**逻辑分析:**
* `students` 是一个 `student` 结构体的数组。
* `ptr` 是一个指向 `students` 数组第一个元素的指针。
* `for` 循环使用指针运算符 `++` 遍历 `students` 数组。
* 在循环中,使用指针运算符 `->` 访问 `student` 结构体的成员。
## 4.2 指针与动态内存分配
### 4.2.1 malloc()和free()函数
`malloc()` 和 `free()` 函数用于动态分配和释放内存。
```c
#include <stdlib.h>
int main() {
// 分配 10 个整数的内存
int *ptr = (int *)malloc(10 * sizeof(int));
// 使用分配的内存
for (int i = 0; i < 10; i++) {
ptr[i] = i;
}
// 释放分配的内存
free(ptr);
return 0;
}
```
**逻辑分析:**
* `malloc()` 函数分配 10 个整数所需的内存,并返回指向分配内存的指针。
* `ptr` 是一个指向分配内存的指针。
* `for` 循环使用指针运算符 `[]` 访问分配的内存。
* `free()` 函数释放分配的内存。
### 4.2.2 指针与链表
链表是一种数据结构,它使用指针将元素连接在一起。
```c
struct node {
int data;
struct node *next;
};
int main() {
// 创建链表的第一个节点
struct node *head = (struct node *)malloc(sizeof(struct node));
head->data = 10;
head->next = NULL;
// 创建链表的第二个节点
struct node *second = (struct node *)malloc(sizeof(struct node));
second->data = 20;
second->next = NULL;
// 将第二个节点连接到第一个节点
head->next = second;
// 遍历链表
struct node *ptr = head;
while (ptr != NULL) {
printf("%d\n", ptr->data);
ptr = ptr->next;
}
// 释放链表的内存
while (head != NULL) {
struct node *next = head->next;
free(head);
head = next;
}
return 0;
}
```
**逻辑分析:**
* `node` 结构体定义了链表节点。
* `head` 是指向链表第一个节点的指针。
* `second` 是指向链表第二个节点的指针。
* `head->next` 将链表的第一个节点连接到第二个节点。
* `while` 循环使用指针运算符 `->` 和 `next` 成员遍历链表。
* `while` 循环释放链表中每个节点的内存。
# 5.1 指针错误的类型
指针错误是指在使用指针时发生的错误,主要分为两类:空指针和野指针。
### 5.1.1 空指针
空指针是指指向一个未分配内存地址的指针。当指针指向空指针时,任何对该指针的解引用操作都会导致程序崩溃。
**产生原因:**
* 未初始化指针变量
* 指针变量被释放后再次使用
* 指针变量被赋值为 NULL(空指针常量)
**危害:**
* 程序崩溃
* 数据损坏
* 系统不稳定
### 5.1.2 野指针
野指针是指指向一个已释放或无效内存地址的指针。当指针指向野指针时,任何对该指针的解引用操作都会导致未定义的行为,可能导致程序崩溃、数据损坏或系统不稳定。
**产生原因:**
* 指针变量指向已释放的内存
* 指针变量指向超出数组或结构体范围的内存
* 指针变量指向未分配的内存
**危害:**
* 程序崩溃
* 数据损坏
* 系统不稳定
## 5.2 指针调试技巧
指针错误的调试是一个具有挑战性的任务,需要使用专门的工具和技巧。以下是一些常见的指针调试技巧:
### 5.2.1 GDB 调试
GDB(GNU 调试器)是一个强大的调试工具,可以帮助调试指针错误。GDB 提供了以下功能:
* 设置断点以在特定代码行处停止执行
* 检查变量的值,包括指针变量
* 检查内存内容
* 单步执行代码以跟踪指针操作
### 5.2.2 printf() 调试
printf() 函数可以用于调试指针错误。通过在代码中添加 printf() 语句,可以打印指针变量的值和指向的内存内容。这有助于识别空指针或野指针。
**示例:**
```c
int *ptr;
// ...
printf("Pointer value: %p\n", ptr);
printf("Memory content: %d\n", *ptr);
```
### 5.2.3 其他技巧
除了 GDB 和 printf() 调试之外,还有其他指针调试技巧:
* 使用 valgrind 等内存调试工具
* 使用指针检查器工具,例如 Clang 的 -fsanitize=address 选项
* 仔细检查指针操作的代码,寻找潜在的错误
* 使用单元测试来测试指针操作的正确性
# 6. 指针在单片机C语言中的实战案例
指针在单片机C语言中有着广泛的应用,以下列举几个实战案例:
### 6.1 字符串处理
指针可以方便地对字符串进行操作。例如,以下代码使用指针遍历字符串并打印每个字符:
```c
#include <stdio.h>
int main() {
char str[] = "Hello, world!";
char *ptr = str;
while (*ptr != '\0') {
printf("%c", *ptr);
ptr++;
}
return 0;
}
```
### 6.2 链表管理
指针可以用于创建和管理链表。例如,以下代码创建一个简单的链表并插入一个新节点:
```c
#include <stdlib.h>
struct node {
int data;
struct node *next;
};
int main() {
struct node *head = NULL;
// 创建一个新节点
struct node *new_node = (struct node *)malloc(sizeof(struct node));
new_node->data = 10;
new_node->next = NULL;
// 将新节点插入链表
if (head == NULL) {
head = new_node;
} else {
struct node *ptr = head;
while (ptr->next != NULL) {
ptr = ptr->next;
}
ptr->next = new_node;
}
// 打印链表
ptr = head;
while (ptr != NULL) {
printf("%d ", ptr->data);
ptr = ptr->next;
}
return 0;
}
```
### 6.3 数据结构实现
指针可以用于实现各种数据结构,例如栈、队列和树。例如,以下代码使用指针实现一个栈:
```c
#include <stdlib.h>
struct stack {
int *data;
int top;
int size;
};
int main() {
struct stack *stack = (struct stack *)malloc(sizeof(struct stack));
stack->data = (int *)malloc(sizeof(int) * 10);
stack->top = -1;
stack->size = 10;
// 入栈
stack->top++;
stack->data[stack->top] = 10;
// 出栈
int popped_value = stack->data[stack->top];
stack->top--;
return 0;
}
```
### 6.4 外设控制
指针可以用于控制单片机的外设。例如,以下代码使用指针访问并控制GPIO寄存器:
```c
#include <stdint.h>
volatile uint32_t *GPIO_BASE = (volatile uint32_t *)0x40000000;
int main() {
// 设置GPIO引脚为输出
*GPIO_BASE |= (1 << 12);
// 设置GPIO引脚为高电平
*GPIO_BASE |= (1 << 13);
return 0;
}
```
### 6.5 系统优化
指针可以用于优化单片机的代码性能。例如,以下代码使用指针避免了数组复制,从而提高了效率:
```c
#include <string.h>
int main() {
char str1[] = "Hello, world!";
char str2[strlen(str1) + 1];
// 使用指针避免数组复制
char *ptr = str1;
while (*ptr != '\0') {
*ptr++ = *ptr;
}
return 0;
}
```
0
0
相关推荐
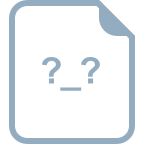
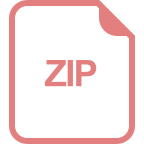
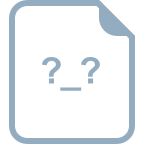





