单片机C语言I2C通信解析:低速串行通信的精髓,让代码更可靠
发布时间: 2024-07-07 05:33:47 阅读量: 67 订阅数: 31 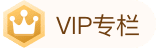
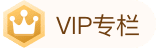
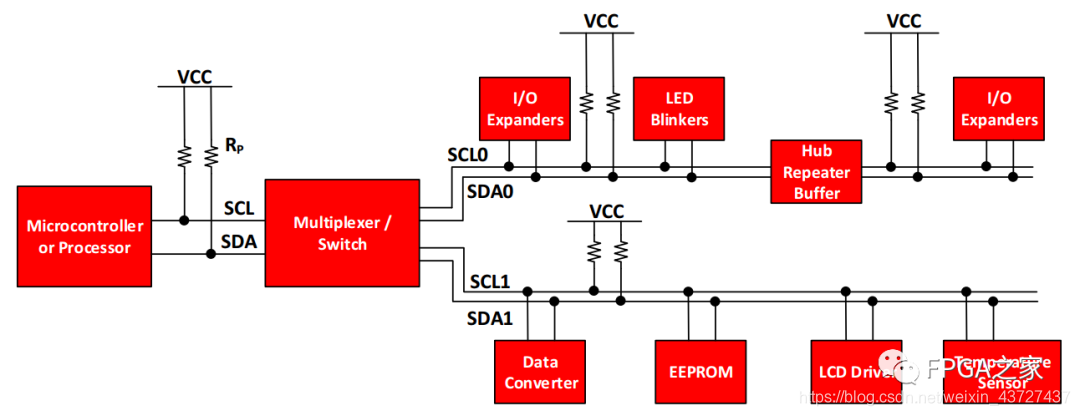
# 1. 单片机C语言I2C通信概述
I2C(Inter-Integrated Circuit)是一种串行通信协议,用于在集成电路(IC)之间进行数据传输。它以其简单易用、低成本和高可靠性而著称,广泛应用于各种电子设备中。
本篇文章将深入探讨单片机C语言中的I2C通信技术,从协议解析、硬件接口初始化、数据收发函数实现到故障诊断和优化,全面阐述I2C通信的原理、编程方法和应用场景。通过循序渐进的讲解,帮助读者深入理解和掌握I2C通信技术在单片机项目中的应用。
# 2. I2C通信协议解析
### 2.1 I2C总线架构和信号定义
#### 2.1.1 I2C总线拓扑结构
I2C总线采用多主从结构,支持多台主设备和多台从设备同时连接在同一条总线上。总线拓扑结构如下图所示:
```mermaid
graph LR
subgraph 主设备
A[主设备1]
B[主设备2]
end
subgraph 从设备
C[从设备1]
D[从设备2]
E[从设备3]
end
A --> C
A --> D
A --> E
B --> C
B --> D
B --> E
```
#### 2.1.2 I2C信号传输原理
I2C总线采用两条信号线进行通信:
- **SCL(时钟线):**由主设备控制,用于同步总线上的数据传输。
- **SDA(数据线):**用于传输数据,由主设备和从设备共同使用。
I2C信号传输采用开漏输出方式,即当总线上没有设备发送数据时,信号线处于高电平;当有设备发送数据时,信号线被拉低。
### 2.2 I2C通信过程和数据格式
#### 2.2.1 I2C通信时序图
I2C通信过程由以下几个阶段组成:
- **起始条件:**主设备将SCL和SDA同时拉低,表示通信开始。
- **设备地址阶段:**主设备发送从设备的地址,从设备响应。
- **读/写位:**主设备发送读/写位,表示要进行读操作还是写操作。
- **数据传输阶段:**主设备和从设备交换数据。
- **停止条件:**主设备将SCL和SDA同时拉高,表示通信结束。
下图展示了I2C通信时序图:
[图片展示I2C通信时序图]
#### 2.2.2 I2C数据帧结构
I2C数据帧由以下部分组成:
- **起始位:**SCL和SDA同时拉低。
- **设备地址:**7位或10位,用于标识从设备。
- **读/写位:**0表示写操作,1表示读操作。
- **数据:**8位或16位,具体长度由通信协议规定。
- **应答位:**从设备在接收数据后发送应答信号,表示数据接收成功。
- **停止位:**SCL和SDA同时拉高。
下表总结了I2C数据帧的格式:
| 字段 | 长度 | 描述 |
|---|---|---|
| 起始位 | 1位 | SCL和SDA同时拉低 |
| 设备地址 | 7位或10位 | 标识从设备 |
| 读/写位 | 1位 | 0表示写操作,1表示读操作 |
| 数据 | 8位或16位 | 数据内容 |
| 应答位 | 1位 | 从设备发送的应答信号 |
| 停止位 | 1位 | SCL和SDA同时拉高 |
# 3. 单片机C语言I2C通信编程
### 3.1 I2C硬件接口初始化
#### 3.1.1 I2C时钟和数据引脚配置
在单片机中,I2C通信需要使用两根引脚:一根作为时钟线(SCL),另一根作为数据线(SDA)。在初始化I2C硬件接口时,需要首先配置这两根引脚为复用功能,并设置其为开漏输出模式。
```c
// I2C时钟和数据引脚配置
void I2C_GPIO_Init(void)
{
// 使能I2C时钟
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOB, ENABLE);
// 配置I2C时钟引脚
GPIO_InitTypeDef GPIO_InitStructure;
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_6;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_OD;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOB, &GPIO_InitStructure);
// 配置I2C数据引脚
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_7;
GPIO_Init(GPIOB, &GPIO_InitStructure);
}
```
**参数说明:**
* `RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOB, ENABLE)`:使能GPIOB时钟。
* `GPIO_InitStructure.GPIO_Pin = GPIO_Pin_6`:配置GPIOB第6位为I2C时钟引脚。
* `GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_OD`:配置为开漏输出模式。
* `GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz`:配置引脚速率为50MHz。
#### 3.1.2 I2C中断配置
在单片机中,I2C通信可以使用中断方式来提高效率。在初始化I2C硬件接口时,需要配置I2C中断,包括中断向量、中断优先级和中断使能。
```c
// I2C中断配置
void I2C_NVIC_Init(void)
{
NVIC_InitTypeDef NVIC_InitStructure;
// 配置I2C中断向量
NVIC_InitStructure.NVIC_IRQChannel = I2C1_EV_IRQn;
NVIC_InitStructure.NVIC_IRQChannelPreemptionPriority = 1;
NVIC_InitStructure.NVIC_IRQChannelSubPriority = 0;
NVIC_InitStructure.NVIC_IRQChannelCmd = ENABLE;
NVIC_Init(&NVIC_InitStructure);
// 配置I2C错误中断向量
NVIC_InitStructure.NVIC_IRQChannel = I2C1_ER_IRQn;
NVIC_Init(&NVIC_InitStructure);
}
```
**参数说明
0
0
相关推荐
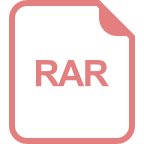
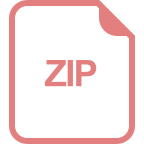
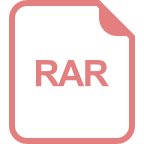
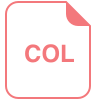
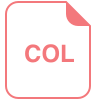
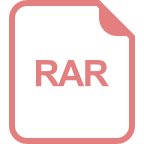
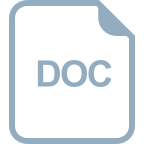
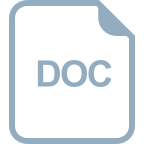
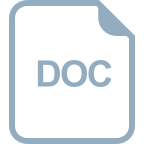