红黑树的搜索算法及效率分析
发布时间: 2024-02-16 06:11:57 阅读量: 32 订阅数: 29 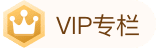
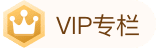
# 1. 红黑树概述
## 1.1 二叉搜索树的特点
二叉搜索树(Binary Search Tree,BST)是一种特殊的二叉树结构,它具有以下特点:
- 每个节点最多有两个子节点,分别称为左子节点和右子节点;
- 对于每个节点,其左子树中的所有节点的值都小于该节点的值,右子树中的所有节点的值都大于该节点的值;
- 中序遍历二叉搜索树可以得到一个有序的节点值序列。
## 1.2 红黑树的定义和性质
红黑树是一种自平衡的二叉搜索树,它具有以下性质:
1. 每个节点要么是红色,要么是黑色;
2. 根节点是黑色;
3. 每个叶子节点(NIL节点,空节点)是黑色;
4. 如果一个节点是红色,则它的子节点都是黑色;
5. 对于每个节点,从该节点出发到所有叶子节点的路径上包含相同数目的黑色节点。
## 1.3 红黑树的应用场景
红黑树广泛应用于各种编程语言的内部实现,例如Java中的`TreeMap`和`TreeSet`,C++中的`std::map`和`std::set`等。它在需要动态插入、删除,同时保持有序性的场景下表现优异,如文件系统,数据库索引等。
# 2. 红黑树的基本操作
### 2.1 插入操作的实现及效率分析
插入操作是红黑树的基本操作之一,在保持红黑树性质的前提下将新节点插入到树中的适当位置。下面我们将详细介绍红黑树插入操作的实现及其效率分析。
#### 插入操作的代码实现(Python版本)
```python
class RedBlackTree:
def __init__(self):
self.root = None
def insert(self, key):
new_node = Node(key)
self._insert_helper(self.root, new_node)
self._insert_fixup(new_node)
def _insert_helper(self, root, node):
if root is None:
self.root = node
elif node.key < root.key:
if root.left is None:
root.left = node
node.parent = root
else:
self._insert_helper(root.left, node)
else:
if root.right is None:
root.right = node
node.parent = root
else:
self._insert_helper(root.right, node)
def _insert_fixup(self, node):
while node.parent is not None and node.parent.color == 'red':
if node.parent == node.parent.parent.left:
uncle = node.parent.parent.right
if uncle is not None and uncle.color == 'red':
node.parent.color = 'black'
uncle.color = 'black'
node.parent.parent.color = 'red'
node = node.parent.parent
else:
if node == node.parent.right:
node = node.parent
self._left_rotate(node)
node.parent.color = 'black'
node.parent.parent.color = 'red'
self._right_rotate(node.parent.parent)
else:
uncle = node.parent.parent.left
if uncle is not None and uncle.color == 'red':
node.parent.color = 'black'
uncle.color = 'black'
node.parent.parent.color = 'red'
node = node.parent.parent
else:
if node == node.parent.left:
node = node.parent
self._right_rotate(node)
node.parent.color = 'black'
node.parent.parent.color = 'red'
self._left_rotate(node.parent.parent)
self.root.color = "black"
def _left_rotate(self, node):
right_child = node.right
node.right = right_child.left
if right_child.left is not None:
right_child.left.parent = node
right_child.parent = node.parent
if node.parent is None:
self.root = right_child
else:
if node == node.parent.left:
node.parent.left = right_child
else:
node.parent.right = right_child
right_child.left = node
node.parent = right_child
def _right_rotate(self, node):
left_child = node.left
node.left = left_child.right
if left_child.right is not None:
left_child.right.parent = node
left_child.parent = node.parent
if node.parent is None:
self.root = left_child
else:
if node == node.parent.right:
node.parent.right = left_child
else:
node.parent.left = left_child
left_child.right = node
node.parent = left_child
# 以下省略其他代码
```
#### 插入操作的效率分析
红黑树的插入操作的时间复杂度为O(log n),其中n为红黑树中已有节点的数量。由于红黑树具有自平衡的特性,插入一个节点后通过旋转和颜色变换来保持树的平衡,使得树的高度保持在O(log n)的范围内。插入操作需要进行一次搜索找到插入位置,然后执行旋转和颜色变换操作,每次插入操作的时间复杂度为O(1)。
但是,红黑树的插入操作并不完全是O(1)的时间复杂度,因为在执行插入操作后还需要进行一次修复操作。修复操作包括旋转和颜色变换,具体的操作次数取决于插入节点的位置以及其祖父节点和叔父节点的颜色。在最坏情况下,可能需要进行O(log n)次修复操作,但是这种情况非常罕见,大部分情况下只需进行O(1)次修复操作。
因此,红黑树的插入操作在平均情况下的时间复杂度为O(log n),在最坏情况下的时间复杂度为O(log n)。这使得红黑树成为一种高效的搜索树结构。
### 2.2 删除操作的实现及效率分析
删除操作是红黑树的基本操作之一,它将一个节点从树中移除,并保持红黑树的性质。下面我们将详细介绍红黑树删除操作的实现及其效率分析。
#### 删除操作的代码实现(Python版本)
```python
class RedBlackTree:
# 省略其他代码
def delete(self, key):
node = self._search(key)
if node is None:
return
self._delete_node(node)
def _delete_node(self, node):
# case 1: 如果待删除节点没有子节点
if node.left is None and node.right is None:
if node == self.root:
self.root = None
else:
if node.color == 'black':
self._delete_fixup(node)
if node == node.parent.left:
node.parent.left = None
else:
no
```
0
0
相关推荐
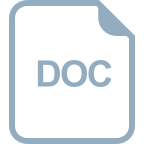
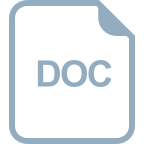
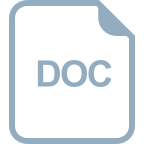
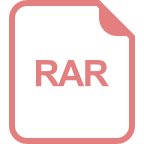
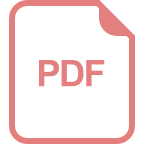
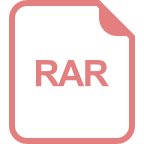
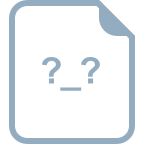
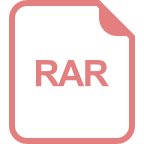
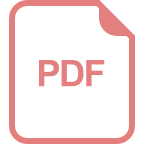