Java算法面试题:栈与队列的实际应用分析与7个实用案例
发布时间: 2024-08-30 03:26:17 阅读量: 59 订阅数: 43 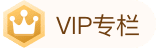
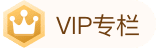
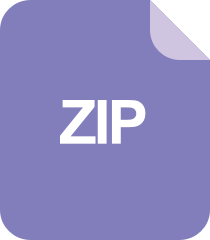
java+sql server项目之科帮网计算机配件报价系统源代码.zip
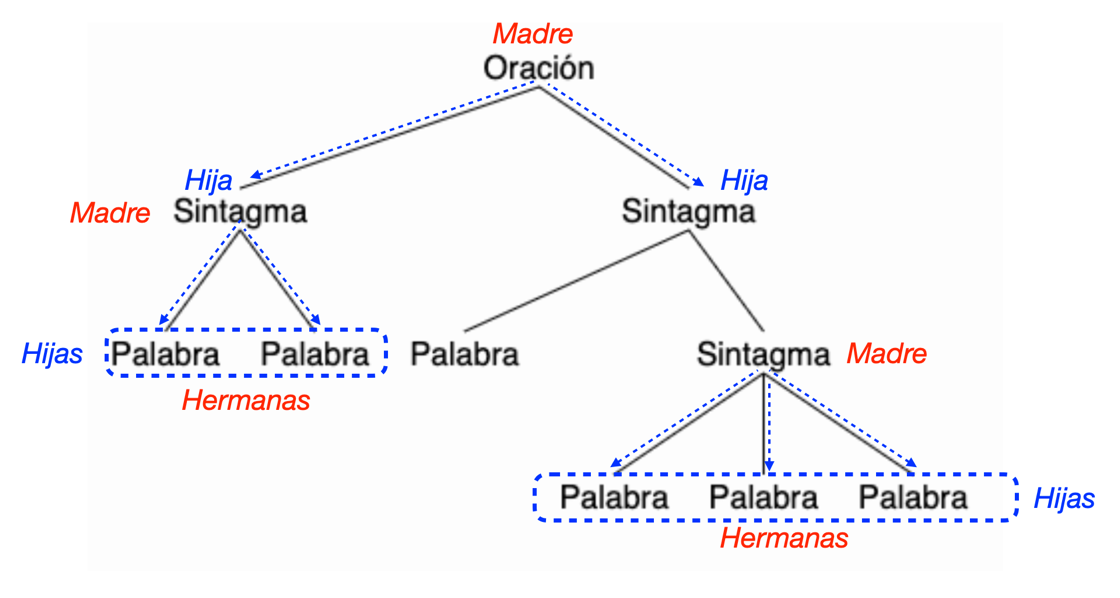
# 1. 栈与队列的数据结构基础
## 简介
在数据结构的世界里,栈(Stack)和队列(Queue)是最基础且广泛应用的线性数据结构之一。它们各自具有独特的特点:栈是一个后进先出(LIFO)的结构,而队列则是一个先进先出(FIFO)的结构。这两个数据结构在解决实际问题中扮演着关键角色,从简单的撤销操作到复杂的算法实现,栈和队列都是不可或缺的工具。
## 栈的基本概念
栈被形象地比喻为一摞盘子,只能从顶部添加或移除元素。添加操作称为“push”,移除操作称为“pop”。栈的这两个操作可以类比为一组“堆叠”任务,最后一个添加进去的将是第一个被处理的。
## 队列的基本概念
队列则像是一条等待服务的队伍,新元素总是在队尾加入,而处理元素则从队首开始。这个数据结构的典型操作包括“enqueue”(入队)和“dequeue”(出队)。队列在处理请求、事件以及在多线程编程中的线程调度等方面应用广泛。
通过本章内容,我们将深入理解栈与队列的核心概念,并准备进入更高级的应用场景和实践案例。
# 2. 栈与队列在算法中的应用
栈和队列是两种基础的数据结构,它们在算法中的应用非常广泛,因为它们能有效地处理各种场景下的数据集合。在这一章节中,我们将详细探讨栈和队列在不同算法中的具体应用,理解它们如何帮助解决实际问题。
## 2.1 栈的应用
栈(Stack)是一种后进先出(LIFO, Last In First Out)的数据结构,它有两个主要操作:push(入栈)和pop(出栈)。在算法中,栈的应用场景非常多样,包括但不限于以下内容。
### 2.1.1 栈在表达式求值中的应用
在表达式求值问题中,栈可以用来处理运算符的优先级和括号。例如,表达式求值通常包括两个栈:一个用于操作数(数字栈),另一个用于运算符(操作符栈)。处理一个中缀表达式(如 3 + 4 * 2 / ( 1 - 5 ) ^ 2 ^ 3)时,通过以下步骤可将其转换为后缀表达式(3 4 2 * 1 5 - 2 3 ^ ^ / +):
```python
import operator
def precedence(op):
precedences = {'+': 1, '-': 1, '*': 2, '/': 2, '^': 3}
return precedences[op]
def apply_operator(operators, values):
operator = operators.pop()
right = values.pop()
left = values.pop()
if operator == '+': values.append(left + right)
elif operator == '-': values.append(left - right)
elif operator == '*': values.append(left * right)
elif operator == '/': values.append(left / right)
elif operator == '^': values.append(left ** right)
def infix_to_postfix(expression):
precedence = {'+': 1, '-': 1, '*': 2, '/': 2, '^': 3}
operators = []
values = []
i = 0
while i < len(expression):
if expression[i].isdigit():
j = i
while j < len(expression) and expression[j].isdigit():
j += 1
values.append(int(expression[i:j]))
i = j
elif expression[i] == '(':
operators.append(expression[i])
elif expression[i] == ')':
while operators[-1] != '(':
apply_operator(operators, values)
operators.pop()
else:
while operators and operators[-1] != '(' and precedence[operators[-1]] >= precedence[expression[i]]:
apply_operator(operators, values)
operators.append(expression[i])
i += 1
while operators:
apply_operator(operators, values)
return values[0]
# 示例:将中缀表达式转换为后缀表达式并计算结果
print(infix_to_postfix("3 + 4 * 2 / ( 1 - 5 ) ^ 2 ^ 3"))
```
上述代码中,我们首先定义了`precedence`函数来判断运算符的优先级,然后定义`apply_operator`函数来执行栈顶的两个操作数与栈顶运算符的运算。最后定义`infix_to_postfix`函数将中缀表达式转换为后缀表达式。
### 2.1.2 栈在括号匹配问题中的应用
括号匹配问题是一个经典的栈的应用场景。通过栈可以方便地判断一个字符串中的括号是否匹配,比如在字符串 "((a+b)*(c-d)/e)" 中,所有的括号是否正确地匹配。
```python
def is_parentheses_balanced(expression):
parentheses_map = {')': '(', ']': '[', '}': '{'}
stack = []
for char in expression:
if char in parentheses_map.values():
stack.append(char)
elif char in parentheses_map.keys():
if not stack or parentheses_map[char] != stack.pop():
return False
return not stack
# 示例:检查括号是否匹配
print(is_parentheses_balanced("((a+b)*(c-d)/e)")) # 输出 True
print(is_parentheses_balanced("((a+b)*(c-d)/e")) # 输出 False
```
在`is_parentheses_balanced`函数中,我们使用一个栈来存储遇到的左括号,每当遇到一个右括号时,我们检查它是否与栈顶的左括号匹配。如果不匹配,或者在遍历完整个字符串后栈不为空,则说明括号不匹配。
## 2.2 队列的应用
队列是一种先进先出(FIFO, First In First Out)的数据结构,它有两个主要操作:enqueue(入队列)和dequeue(出队列)。队列在算法中的应用同样十分丰富,例如用于任务调度、广度优先搜索等场景。
### 2.2.1 队列在任务调度中的应用
在操作系统中,队列被用于任务调度,比如先来先服务(FCFS, First-Come, First-Served)调度算法。队列模拟了任务的执行顺序,最先入队的任务最先被执行。
### 2.2.2 队列在广度优先搜索中的应用
广度优先搜索(BFS, Breadth-First Search)是一种用于图的遍历或搜索树结构的算法。队列在BFS中用于存储即将访问的节点,以确保按照从近到远的顺序搜索图中的节点。
```python
from collections import deque
def bfs(graph, start):
visited, queue = set(), deque([start])
while queue:
vertex = queue.popleft()
if vertex not in visited:
print(vertex, end=" ")
visited.add(vertex)
queue.extend([n for n in graph[vertex] if n not in visited])
# 示例:使用队列进行图的广度优先搜索
graph = {
'A': ['B', 'C'],
'B': ['D', 'E'],
'C': ['F'],
'D': [],
'E': ['F'],
'F': []
}
print("广度优先遍历结果:")
bfs(graph, 'A')
```
在上述代码中,我们首先导入了Python的`deque`来创建队列,然后定义了`bfs`函数来遍历图。我们使用队列来维护待访问的节点,并使用集合`visited`来记录已经访问过的节点。
通过以上各个小节,我们介绍了栈与队列在算法中应用的典型例子,每一部分都提供了具体的操作步骤、代码实现以及算法分析。在后续章节中,我们将继续深入探讨栈与队列的高级数据结构,及其在实际项目中的应用案例,并最终引向面试准备策略。
# 3. 栈与队列的高级数据结构
## 3.1 双端队列(Deque)
双端队列(Deque)是一种允许我们同时在两端进行插入和删除操作的线性数据结构。这种灵活性让Deque在算法设计中显得特别有用,特别是在需要频繁在两端进行操作的场景下。
### 3.1.1 双端队列的基本操作
在高级数据结构中,Deque提供了以下几种基本操作:
- **push_front(x)**: 在队列前端添加一个元素 x。
- **push_back(x)**: 在队列后端添加一个元素 x。
- **pop_front()**: 移除并返回队列前端的元素。
- **pop_back()**: 移除并返回队列后端的元素。
- **front()**: 返回队列前端的元素。
- **back()**: 返回队列后端的元素。
双端队列可以支持栈和队列的特性,即可以像栈一样进行后进先出的操作,也可以像队列一样进行先进先出的操作。
### 3.1.2 双端队列在算法中的应用
0
0
相关推荐
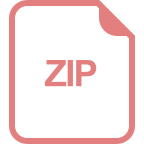
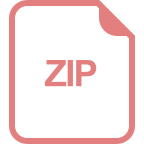
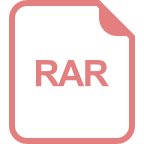
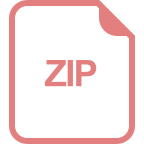
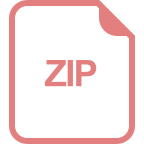