Java算法面试题:字符串匹配与编辑距离的实战分析
发布时间: 2024-08-30 03:18:18 阅读量: 59 订阅数: 22 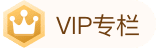
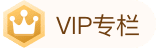
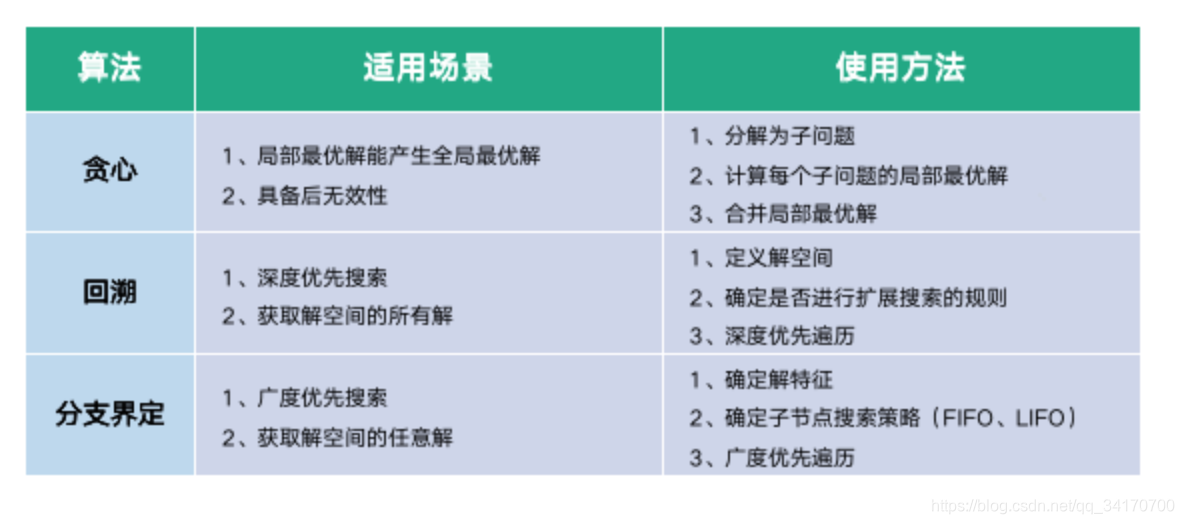
# 1. 字符串匹配与编辑距离概述
字符串匹配与编辑距离是计算机科学中两个基础且重要的概念。它们不仅在理论研究中占有重要地位,而且在实际应用,比如文本编辑、生物信息学、语音识别以及自然语言处理等领域中扮演着核心角色。本章旨在为读者提供一个全面的入门级介绍,涵盖基本定义、应用场景,并为接下来的深入探讨奠定基础。
在进一步学习之前,让我们先理解这两个术语:
- **字符串匹配**:在一段文本(也称为“主串”)中,寻找与另一段较短的字符串(称为“模式串”)相匹配的所有位置的过程。
- **编辑距离**:衡量将一个字符串转换成另一个字符串所需的最少编辑操作数,包括插入、删除和替换字符。
接下来的章节将详细讨论这些概念,并引入常见的算法以及它们在不同场景下的应用和优化。
# 2. 字符串匹配算法理论与实践
## 2.1 字符串匹配基础概念
### 2.1.1 字符串匹配的定义和应用场景
字符串匹配是在一个文本字符串中查找某个模式(pattern)字符串的位置的过程。这在计算机科学中是一个基本的问题,在文本编辑、生物信息学、数据压缩、网络安全、数据库和自然语言处理等领域有着广泛的应用。
在文本编辑器中,查找和替换功能利用字符串匹配来定位需要编辑的文本片段。在网络安全领域,字符串匹配技术被用于入侵检测系统,通过匹配特定的攻击签名来检测恶意流量。
### 2.1.2 简单的匹配算法:暴力匹配
暴力匹配算法(Brute Force)是最直观的字符串匹配方法。它通过逐个比较文本字符串(text)和模式字符串(pattern)中的字符来工作。
该算法从文本的第一个字符开始,尝试逐个匹配模式字符串。如果在某个位置发现不匹配的字符,算法会将模式字符串整体向右滑动一位,然后从模式字符串的第一个字符开始重新匹配。
虽然暴力匹配算法简单易懂,但在最坏情况下时间复杂度为O(n*m),其中n是文本字符串的长度,m是模式字符串的长度。因此,在实际应用中通常会寻找更高效的算法来处理大规模数据。
```python
def brute_force_match(text, pattern):
n = len(text)
m = len(pattern)
for i in range(n - m + 1):
for j in range(m):
if text[i + j] != pattern[j]:
break
else: # 此处else与for循环对应,表示循环正常结束,没有执行break
return i # 匹配成功返回模式字符串在文本中的起始索引
return -1 # 没有匹配到返回-1
```
在上面的Python代码中,暴力匹配算法通过两层循环来比较文本和模式字符串。如果字符匹配,则内层循环继续;如果字符不匹配,则跳出内层循环。如果内层循环正常结束(没有被break打断),则表示找到了匹配,返回当前匹配的起始索引。
## 2.2 高级字符串匹配算法
### 2.2.1 KMP算法的原理和实现
KMP算法(Knuth-Morris-Pratt)是一种改进的字符串匹配算法,主要解决暴力匹配算法在遇到不匹配时需要从头开始匹配的问题。KMP算法通过预处理模式字符串来找到部分匹配,使得在不匹配时可以跳过一些不必要的比较。
KMP算法的关键在于构建一个部分匹配表(也称为“失配函数”或“前缀函数”),该表记录了模式字符串中每个位置之前的子串的最长相同前后缀的长度。当发生不匹配时,可以根据部分匹配表将模式字符串滑动到正确的位置继续匹配。
```python
def kmp_table(pattern):
m = len(pattern)
table = [0] * m
table[0] = -1
j = 0
for i in range(1, m):
while j > 0 and pattern[i] != pattern[j]:
j = table[j]
if pattern[i] == pattern[j]:
j += 1
table[i] = j
return table
def kmp_match(text, pattern):
n = len(text)
m = len(pattern)
if m == 0:
return 0
table = kmp_table(pattern)
j = 0
for i in range(n):
while j > 0 and text[i] != pattern[j]:
j = table[j]
if text[i] == pattern[j]:
j += 1
if j == m:
return i - m + 1 # 匹配成功
return -1 # 匹配失败
```
在上述代码中,`kmp_table`函数用于构建部分匹配表,而`kmp_match`函数则实现了KMP算法的字符串匹配过程。
### 2.2.2 Rabin-Karp算法的原理和实现
Rabin-Karp算法是一种用于多模式匹配的字符串匹配算法。它通过散列函数将模式字符串和文本字符串中的子串转换成数值,从而进行快速的匹配检测。该算法特别适合在文本中查找多个模式字符串。
Rabin-Karp算法的核心在于利用哈希表来避免重复的字符串比较。在模式字符串和文本字符串的每次比较中,算法计算一个哈希值来快速判断是否匹配。当发现潜在的匹配时,再进行详细的字符比较来确认是否真的匹配。
```python
def rabin_karp(text, pattern):
d = 256 # 字符集大小
q = 101 # 一个质数
m = len(pattern)
n = len(text)
if m == 0:
return 0
if m > n:
return -1
p = 0
t = 0
h = 1
for i in range(m - 1):
h = (h * d) % q
for i in range(m):
p = (d * p + ord(pattern[i])) % q
t = (d * t + ord(text[i])) % q
for s in range(n - m + 1):
if p == t:
if text[s:s + m] == pattern:
return s
if s < n - m:
```
0
0
相关推荐
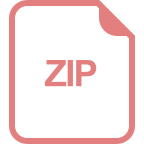
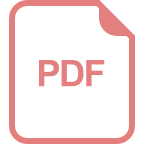
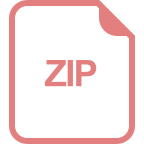





