PHP数据库分页与设计模式:应用设计模式,优化分页代码
发布时间: 2024-07-22 22:27:32 阅读量: 37 订阅数: 33 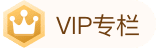
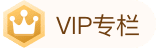
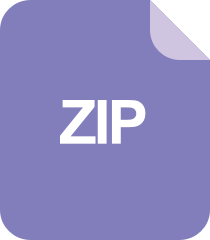
基于Java多线程与设计模式的2310服务架构优化源码
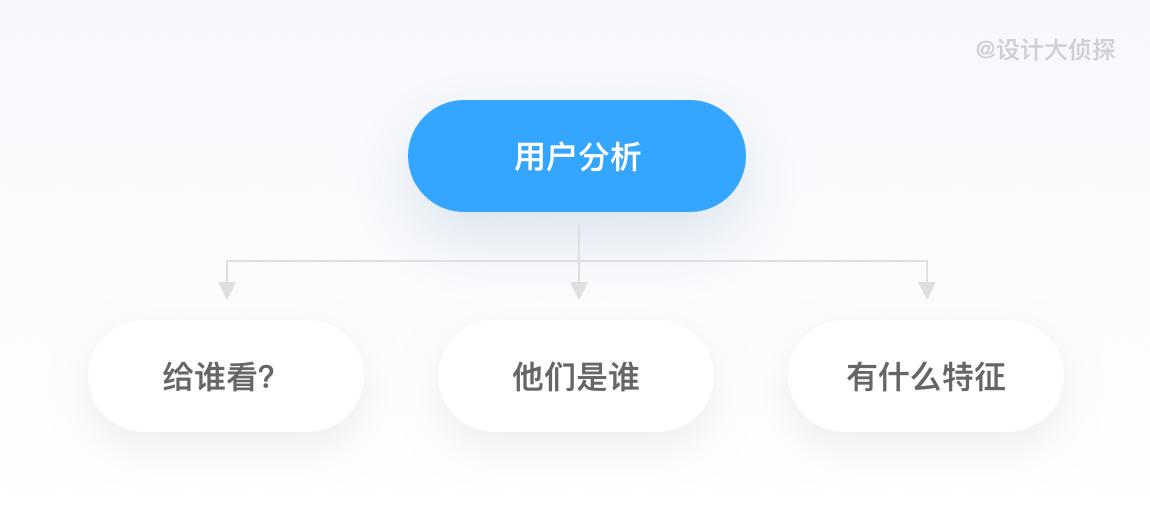
# 1. PHP数据库分页概述
**1.1 分页的必要性**
在处理海量数据时,分页是将数据分成较小的、可管理的块的过程。它可以提高性能、增强用户体验并简化数据管理。
**1.2 分页的原理**
分页通过以下步骤实现:
1. 确定要显示的数据总量(总记录数)。
2. 指定每页要显示的记录数(每页记录数)。
3. 计算总页数(总记录数 / 每页记录数)。
4. 根据当前页码计算要检索的记录的偏移量(偏移量 = (当前页码 - 1)* 每页记录数)。
5. 使用偏移量和每页记录数从数据库中检索数据。
# 2. PHP数据库分页设计模式
### 2.1 分页设计模式的类型
在PHP中,实现数据库分页有三种主要的设计模式:
#### 2.1.1 游标分页
游标分页通过使用数据库游标来实现分页。游标是一个指向数据库中特定记录集的指针。通过移动游标,可以逐行遍历记录集。游标分页的优点是它可以高效地处理大数据集,因为不需要一次性加载所有记录。
#### 2.1.2 偏移量分页
偏移量分页通过使用SQL的LIMIT和OFFSET子句来实现分页。LIMIT子句指定要返回的记录数,OFFSET子句指定要跳过的记录数。偏移量分页的优点是它易于实现,并且适用于大多数数据库系统。
#### 2.1.3 链接分页
链接分页通过在页面上显示链接来实现分页。每个链接代表一个页面,单击链接将加载该页面的记录。链接分页的优点是它易于使用,并且允许用户轻松地在页面之间导航。
### 2.2 分页设计模式的优缺点
#### 2.2.1 游标分页的优缺点
**优点:**
* 高效处理大数据集
* 可以逐行遍历记录集
**缺点:**
* 某些数据库系统不支持游标
* 可能难以实现
#### 2.2.2 偏移量分页的优缺点
**优点:**
* 易于实现
* 适用于大多数数据库系统
**缺点:**
* 处理大数据集时效率较低
* 可能导致性能问题
#### 2.2.3 链接分页的优缺点
**优点:**
* 易于使用
* 允许用户轻松导航页面
**缺点:**
* 可能会生成大量页面
* 对于大数据集,可能导致性能问题
**选择分页设计模式时,需要考虑以下因素:**
* 数据集大小
* 数据库系统
* 性能要求
* 用户体验
# 3.1 游标分页的实现
#### 3.1.1 游标分页的原理
游标分页是一种通过使用数据库游标来实现分页的技术。游标是一个指向数据库中特定记录集的指针,它允许应用程序逐行访问记录。游标分页的原理是:
1. 打开一个游标,指向要分页的数据集。
2. 使用游标的 `fetch()` 方法逐行获取记录。
3. 当游标到达当前页的最后一行时,使用 `seek()` 方法将游标移动到下一页的第一行。
4. 重复步骤 2 和 3,直到获取到所有页面的数据。
游标分页的优点是:
* 效率高:游标分页只需要一次数据库查询,因此效率很高。
* 灵活:游标分页可以轻松实现各种分页场景,例如正向分页、反向分页和随机分页。
游标分页的缺点是:
* 占用资源:游标需要占用服务器资源,因此不适合处理大数据集。
* 不支持并发:游标分页不支持并发访问,因此不适合高并发场景。
#### 3.1.2 游标分页的代码示例
```php
<?php
// 打开游标
$cursor = $db->query('SELECT * FROM users')->fetchCursor();
// 设置每页记录数
$pageSize = 10;
// 获取当前页码
$currentPage = isset($_GET['page']) ? $_GET['page'] : 1;
// 计算偏移量
$offset = ($currentPage - 1) * $pageSize;
// 移动游标到指定偏移量
$cursor->seek($offset);
// 获取当前页的数据
$users = [];
for ($i = 0; $i < $pageSize; $i++) {
$user = $cursor->fetch();
if (!$user) {
break;
}
$users[] = $user;
}
// 输出当前页的数据
echo json_encode($users);
```
**代码逻辑分析:**
1. 打开一个指向 `users` 表的游标。
2. 设置每页记录数为 10。
3. 获取当前页码,默认为 1。
4. 计算偏移量,根据当前页码和每页记录数计算出偏移量。
5. 将游标移动到指定偏移量,跳过前几页的数据。
6. 使用循环逐行获取当前页的数据,直到达到每页记录数或游标到达末尾。
7. 输出当前页的数据。
# 4. PHP数据库分页优化
### 4.1 分页性能优化
#### 4.1.1 索引优化
索引是数据库中用于快速查找数据的结构。为分页查询中涉及的字段添加索引可以显著提高查询性能。
**示例代码:**
```php
CREATE INDEX idx_name ON table_name (column_name);
```
**逻辑分析:**
该代码创建了一个名为 `idx_name` 的索引,用于对 `table_name` 表中的 `column_name` 字段进行快速查找。
#### 4.1.2 缓存优化
缓存是一种存储数据的临时区域,可以减少对数据库的访问次数。将分页查询结果缓存起来可以提高后续查询的性能。
**示例代码:**
```php
$cache = new Cache();
$cache->set('page_results', $results, 3600);
```
**逻辑分析:**
该代码使用 `Cache` 类将分页查询结果存储在缓存中,并设置缓存时间为 3600 秒(1 小时)。
#### 4.1.3 查询优化
优化分页查询本身也可以提高性能。以下是一些优化技巧:
- 使用 `LIMIT` 子句限制返回的行数。
- 使用 `OFFSET` 子句跳过不需要的行。
- 避免使用 `SELECT *`,只选择需要的字段。
- 使用连接查询代替多次查询。
### 4.2 分页代码优化
#### 4.2.1 代码重构
重构分页代码可以提高可读性和可维护性。将分页逻辑提取到单独的函数或类中,可以使代码更易于理解和管理。
**示例代码:**
```php
function paginate($query, $page, $limit) {
$offset = ($page - 1) * $limit;
$query->limit($limit)->offset($offset);
return $query->get();
}
```
**逻辑分析:**
该函数接受一个查询对象、当前页码和每页显示的行数,并返回分页后的查询结果。
#### 4.2.2 错误处理
分页代码中应包含适当的错误处理机制,以处理可能发生的异常。例如,当页码超出范围或每页显示的行数无效时,应返回错误消息或异常。
**示例代码:**
```php
try {
$results = paginate($query, $page, $limit);
} catch (Exception $e) {
echo $e->getMessage();
}
```
**逻辑分析:**
该代码使用 `try-catch` 块来捕获分页函数中可能发生的异常,并输出错误消息。
#### 4.2.3 安全性增强
分页代码应防止 SQL 注入攻击。使用预处理语句或参数化查询可以防止攻击者通过恶意输入修改查询。
**示例代码:**
```php
$stmt = $db->prepare("SELECT * FROM table_name WHERE id = ? LIMIT ? OFFSET ?");
$stmt->bind_param('iii', $id, $limit, $offset);
$stmt->execute();
```
**逻辑分析:**
该代码使用预处理语句来执行分页查询,并使用 `bind_param()` 方法将参数绑定到查询中,从而防止 SQL 注入攻击。
# 5. PHP数据库分页案例
### 5.1 基于游标分页的案例
#### 5.1.1 案例需求
假设我们有一个包含用户数据的表 `users`,并希望实现一个分页功能,每页显示 10 条记录。
#### 5.1.2 案例实现
```php
<?php
// 连接数据库
$conn = new PDO('mysql:host=localhost;dbname=database', 'username', 'password');
// 获取当前页码
$page = isset($_GET['page']) ? (int) $_GET['page'] : 1;
// 计算偏移量
$offset = ($page - 1) * 10;
// 查询数据
$stmt = $conn->prepare('SELECT * FROM users ORDER BY id ASC LIMIT 10 OFFSET :offset');
$stmt->bindParam(':offset', $offset, PDO::PARAM_INT);
$stmt->execute();
$users = $stmt->fetchAll(PDO::FETCH_ASSOC);
// 计算总页数
$stmt = $conn->prepare('SELECT COUNT(*) AS total FROM users');
$stmt->execute();
$total = $stmt->fetchColumn();
$totalPages = ceil($total / 10);
// 生成分页链接
$pagination = '<ul class="pagination">';
for ($i = 1; $i <= $totalPages; $i++) {
$active = $i == $page ? 'active' : '';
$pagination .= "<li class='$active'><a href='?page=$i'>$i</a></li>";
}
$pagination .= '</ul>';
?>
<!DOCTYPE html>
<html>
<head>
<title>游标分页案例</title>
</head>
<body>
<h1>用户列表</h1>
<table border="1">
<thead>
<tr>
<th>ID</th>
<th>用户名</th>
<th>邮箱</th>
</tr>
</thead>
<tbody>
<?php foreach ($users as $user): ?>
<tr>
<td><?= $user['id'] ?></td>
<td><?= $user['username'] ?></td>
<td><?= $user['email'] ?></td>
</tr>
<?php endforeach; ?>
</tbody>
</table>
<?= $pagination ?>
</body>
</html>
```
**逻辑分析:**
* 连接数据库并获取当前页码。
* 根据当前页码计算偏移量。
* 使用游标分页查询数据,并限制每页显示 10 条记录。
* 查询总记录数并计算总页数。
* 生成分页链接,并根据当前页码高亮显示当前页。
### 5.2 基于偏移量分页的案例
#### 5.2.1 案例需求
假设我们有一个包含产品数据的表 `products`,并希望实现一个分页功能,每页显示 20 条记录。
#### 5.2.2 案例实现
```php
<?php
// 连接数据库
$conn = new PDO('mysql:host=localhost;dbname=database', 'username', 'password');
// 获取当前页码
$page = isset($_GET['page']) ? (int) $_GET['page'] : 1;
// 计算偏移量
$offset = ($page - 1) * 20;
// 查询数据
$stmt = $conn->prepare('SELECT * FROM products ORDER BY id ASC LIMIT 20 OFFSET :offset');
$stmt->bindParam(':offset', $offset, PDO::PARAM_INT);
$stmt->execute();
$products = $stmt->fetchAll(PDO::FETCH_ASSOC);
// 计算总页数
$stmt = $conn->prepare('SELECT COUNT(*) AS total FROM products');
$stmt->execute();
$total = $stmt->fetchColumn();
$totalPages = ceil($total / 20);
// 生成分页链接
$pagination = '<ul class="pagination">';
for ($i = 1; $i <= $totalPages; $i++) {
$active = $i == $page ? 'active' : '';
$pagination .= "<li class='$active'><a href='?page=$i'>$i</a></li>";
}
$pagination .= '</ul>';
?>
<!DOCTYPE html>
<html>
<head>
<title>偏移量分页案例</title>
</head>
<body>
<h1>产品列表</h1>
<table border="1">
<thead>
<tr>
<th>ID</th>
<th>产品名称</th>
<th>价格</th>
</tr>
</thead>
<tbody>
<?php foreach ($products as $product): ?>
<tr>
<td><?= $product['id'] ?></td>
<td><?= $product['name'] ?></td>
<td><?= $product['price'] ?></td>
</tr>
<?php endforeach; ?>
</tbody>
</table>
<?= $pagination ?>
</body>
</html>
```
**逻辑分析:**
* 连接数据库并获取当前页码。
* 根据当前页码计算偏移量。
* 使用偏移量分页查询数据,并限制每页显示 20 条记录。
* 查询总记录数并计算总页数。
* 生成分页链接,并根据当前页码高亮显示当前页。
### 5.3 基于链接分页的案例
#### 5.3.1 案例需求
假设我们有一个包含订单数据的表 `orders`,并希望实现一个分页功能,每页显示 15 条记录。
#### 5.3.2 案例实现
```php
<?php
// 连接数据库
$conn = new PDO('mysql:host=localhost;dbname=database', 'username', 'password');
// 获取当前页码
$page = isset($_GET['page']) ? (int) $_GET['page'] : 1;
// 计算偏移量
$offset = ($page - 1) * 15;
// 查询数据
$stmt = $conn->prepare('SELECT * FROM orders ORDER BY id ASC LIMIT 15 OFFSET :offset');
$stmt->bindParam(':offset', $offset, PDO::PARAM_INT);
$stmt->execute();
$orders = $stmt->fetchAll(PDO::FETCH_ASSOC);
// 计算总页数
$stmt = $conn->prepare('SELECT COUNT(*) AS total FROM orders');
$stmt->execute();
$total = $stmt->fetchColumn();
$totalPages = ceil($total / 15);
// 生成分页链接
$pagination = '<ul class="pagination">';
for ($i = 1; $i <= $totalPages; $i++) {
$active = $i == $page ? 'active' : '';
$pagination .= "<li class='$active'><a href='?page=$i'>$i</a></li>";
}
$pagination .= '</ul>';
?>
<!DOCTYPE html>
<html>
<head>
<title>链接分页案例</title>
</head>
<body>
<h1>订单列表</h1>
<table border="1">
<thead>
<tr>
<th>ID</th>
<th>订单号</th>
<th>总金额</th>
</tr>
</thead>
<tbody>
<?php foreach ($orders as $order): ?>
<tr>
<td><?= $order['id'] ?></td>
<td><?= $order['order_number'] ?></td>
<td><?= $order['total_amount'] ?></td>
</tr>
<?php endforeach; ?>
</tbody>
</table>
<?= $pagination ?>
</body>
</html>
```
**逻辑分析:**
* 连接数据库并获取当前页码。
* 根据当前页码计算偏移量。
* 使用链接分页查询数据,并限制每页显示 15 条记录。
* 查询总记录数并计算总页数。
* 生成分页链接,并根据当前页码高亮显示当前页。
# 6. PHP数据库分页总结
### 6.1 分页设计模式的选择
在选择分页设计模式时,需要考虑以下因素:
- **数据量:**数据量越大,游标分页的性能优势越明显。
- **查询复杂度:**查询越复杂,链接分页的性能优势越明显。
- **并发访问:**偏移量分页在并发访问下容易出现数据不一致问题。
- **开发成本:**链接分页的开发成本较高,游标分页的开发成本较低。
### 6.2 分页性能优化技巧
- **索引优化:**为分页查询涉及的字段建立索引,可以显著提高查询效率。
- **缓存优化:**将分页结果缓存起来,可以避免重复查询数据库。
- **查询优化:**使用高效的查询语句,减少不必要的查询操作。
- **分页大小:**合理设置分页大小,避免一次性加载过多数据。
- **异步分页:**使用异步技术,将分页查询操作放到后台执行,避免影响页面响应时间。
### 6.3 分页代码优化要点
- **代码重构:**将分页代码模块化,提高代码的可维护性。
- **错误处理:**完善错误处理机制,避免分页操作出现异常。
- **安全性增强:**防止SQL注入等安全漏洞,保证分页代码的安全性。
0
0
相关推荐
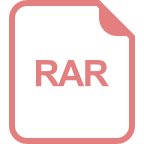
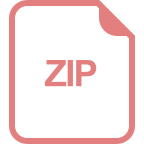
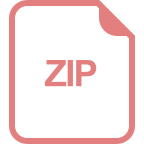
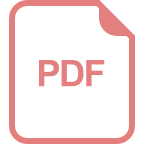
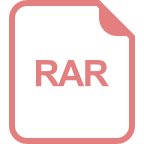
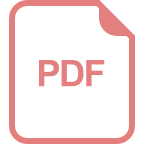
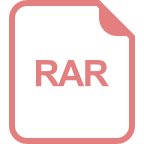
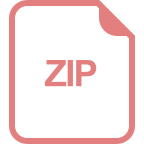