【PHP数据库分页指南】:掌握分页技术,从基础到实战
发布时间: 2024-07-22 21:44:35 阅读量: 30 订阅数: 29 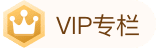
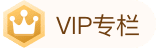
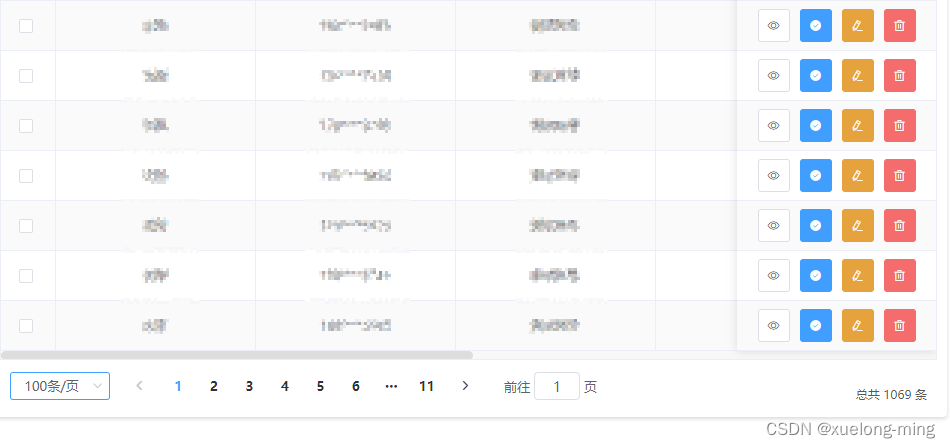
# 1. PHP数据库分页的理论基础**
PHP数据库分页是将大型数据集分解成较小的、可管理的页面,以提高Web应用程序的性能和用户体验。其核心原理是:
* **查询限制:**通过LIMIT子句限制每次查询返回的行数。
* **偏移量:**指定查询从数据集中的哪个位置开始返回行。
* **页码:**表示当前正在查看的页面。
* **总页数:**根据数据集的大小和每页的行数计算得出。
# 2. PHP数据库分页的编程技巧
### 2.1 分页算法与实现
#### 2.1.1 分页算法的原理
分页算法的核心思想是将庞大的数据集划分为较小的、易于管理的页面。最常用的分页算法是偏移量限制算法,其原理如下:
* **计算总页数:**总页数 = 总记录数 / 每页记录数
* **计算当前页的偏移量:**偏移量 = (当前页码 - 1) * 每页记录数
* **获取当前页的数据:**SELECT * FROM 表名 LIMIT 偏移量, 每页记录数
#### 2.1.2 PHP中实现分页算法
```php
<?php
// 获取总记录数
$total_records = $db->query("SELECT COUNT(*) FROM table_name")->fetchColumn();
// 每页记录数
$per_page = 10;
// 计算总页数
$total_pages = ceil($total_records / $per_page);
// 获取当前页码
$current_page = isset($_GET['page']) ? (int) $_GET['page'] : 1;
// 计算当前页的偏移量
$offset = ($current_page - 1) * $per_page;
// 获取当前页的数据
$query = $db->prepare("SELECT * FROM table_name LIMIT ?, ?");
$query->execute([$offset, $per_page]);
$results = $query->fetchAll();
?>
```
### 2.2 分页参数的处理
#### 2.2.1 获取分页参数
分页参数通常通过GET请求传递,例如:
```
?page=2
```
在PHP中,可以使用`$_GET`超级全局变量获取分页参数:
```php
$current_page = isset($_GET['page']) ? (int) $_GET['page'] : 1;
```
#### 2.2.2 参数验证与处理
获取分页参数后,需要进行验证和处理,以防止恶意输入:
* **类型检查:**确保分页参数是整数类型。
* **范围检查:**确保分页参数在有效范围内(1到总页数)。
* **默认值:**如果分页参数无效,则使用默认值(通常为1)。
```php
if (!isset($_GET['page']) || !is_numeric($_GET['page'])) {
$current_page = 1;
} else {
$current_page = (int) $_GET['page'];
if ($current_page < 1 || $current_page > $total_pages) {
$current_page = 1;
}
}
```
### 2.3 分页查询的优化
#### 2.3.1 索引的使用
索引是数据库中用于快速查找记录的特殊数据结构。在分页查询中,可以使用索引来优化查询速度。
```sql
CREATE INDEX index_name ON table_name (column_name);
```
#### 2.3.2 查询语句的优化
优化分页查询语句可以减少数据库的负载:
* **使用LIMIT子句:**LIMIT子句指定要返回的记录数。
* **使用ORDER BY子句:**ORDER BY子句指定要按哪个列排序。
* **避免使用SELECT *:**只选择需要的列,减少数据传输量。
* **使用子查询:**将分页逻辑放在子查询中,提高查询效率。
# 3. PHP数据库分页的实践应用
### 3.1 基本分页功能实现
**3.1.1 简单的分页代码示例**
以下是一个实现基本分页功能的简单代码示例:
```php
<?php
// 获取分页参数
$page = isset($_GET['page']) ? (int)$_GET['page'] : 1;
$limit = 10; // 每页显示的记录数
// 计算偏移量
$offset = ($page - 1) * $limit;
// 查询数据
$sql = "SELECT * FROM table LIMIT $offset, $limit";
$result = $conn->query($sql);
// 获取总记录数
$total_records = $conn->query("SELECT COUNT(*) FROM table")->fetchColumn();
// 计算总页数
$total_pages = ceil($total_records / $limit);
?>
<!-- 分页导航栏 -->
<ul class="pagination">
<?php
for ($i = 1; $i <= $total_pages; $i++) {
if ($i == $page) {
echo "<li class='active'><a href='?page=$i'>$i</a></li>";
} else {
echo "<li><a href='?page=$i'>$i</a></li>";
}
}
?>
</ul>
```
**代码逻辑分析:**
1. 获取分页参数 `page`,默认为 1。
2. 定义每页显示的记录数 `limit` 为 10。
3. 计算偏移量 `offset`,用于限制查询结果的范围。
4. 查询数据,并获取总记录数 `total_records`。
5. 计算总页数 `total_pages`。
6. 生成分页导航栏,显示页码链接。
### 3.1.2 分页样式的定制
可以根据需要定制分页样式,例如:
```css
.pagination {
display: flex;
justify-content: center;
align-items: center;
}
.pagination li {
list-style-type: none;
margin: 0 5px;
}
.pagination li a {
display: inline-block;
padding: 5px 10px;
border: 1px solid #ccc;
text-decoration: none;
}
.pagination li.active a {
background-color: #ccc;
}
```
### 3.2 高级分页功能实现
**3.2.1 Ajax分页**
Ajax分页可以实现无刷新加载更多数据,提升用户体验。
```php
<script>
function loadMoreData(page) {
$.ajax({
url: 'ajax_pagination.php',
data: {page: page},
success: function(data) {
$('#data-container').append(data);
}
});
}
</script>
<!-- 分页导航栏 -->
<ul class="pagination">
<?php
for ($i = 1; $i <= $total_pages; $i++) {
if ($i == $page) {
echo "<li class='active'><a href='javascript:void(0);' onclick='loadMoreData($i)'>$i</a></li>";
} else {
echo "<li><a href='javascript:void(0);' onclick='loadMoreData($i)'>$i</a></li>";
}
}
?>
</ul>
```
**代码逻辑分析:**
1. 定义 `loadMoreData` 函数,用于通过 Ajax 加载更多数据。
2. 在分页导航栏中,将页码链接改为 Ajax 调用。
3. 在 `ajax_pagination.php` 中,根据传入的 `page` 参数查询并返回数据。
**3.2.2 无限滚动分页**
无限滚动分页可以自动加载更多数据,无需手动点击加载更多按钮。
```php
<script>
$(window).scroll(function() {
if ($(window).scrollTop() + $(window).height() >= $(document).height()) {
loadMoreData();
}
});
function loadMoreData() {
$.ajax({
url: 'ajax_pagination.php',
data: {page: page},
success: function(data) {
$('#data-container').append(data);
page++;
}
});
}
</script>
```
**代码逻辑分析:**
1. 定义 `loadMoreData` 函数,用于通过 Ajax 加载更多数据。
2. 监听窗口滚动事件,当滚动到页面底部时,自动加载更多数据。
3. 在 `ajax_pagination.php` 中,根据传入的 `page` 参数查询并返回数据。
# 4. PHP数据库分页的进阶应用
### 4.1 分页与搜索的结合
#### 4.1.1 分页搜索的原理
分页搜索是指在进行数据库分页查询的同时,还对查询结果进行搜索过滤。其原理是将搜索条件与分页查询条件结合,在执行分页查询时同时应用搜索条件,从而实现对分页结果的搜索过滤。
#### 4.1.2 PHP中实现分页搜索
```php
<?php
// 获取分页参数
$page = isset($_GET['page']) ? intval($_GET['page']) : 1;
$limit = isset($_GET['limit']) ? intval($_GET['limit']) : 10;
// 获取搜索参数
$search = isset($_GET['search']) ? trim($_GET['search']) : '';
// 构建查询语句
$sql = "SELECT * FROM table WHERE name LIKE '%$search%'";
// 执行分页查询
$result = $db->query($sql)->fetch_all();
// 计算总记录数
$total = count($result);
// 计算总页数
$totalPages = ceil($total / $limit);
// 获取当前页数据
$offset = ($page - 1) * $limit;
$data = array_slice($result, $offset, $limit);
// 返回分页数据
echo json_encode(['data' => $data, 'total' => $total, 'totalPages' => $totalPages]);
?>
```
**代码逻辑分析:**
1. 获取分页参数 `page` 和 `limit`,并进行类型转换和默认值设置。
2. 获取搜索参数 `search`,并进行去除前后空格处理。
3. 构建查询语句,将搜索条件与分页查询条件结合。
4. 执行分页查询,并获取所有查询结果。
5. 计算总记录数 `total`。
6. 计算总页数 `totalPages`。
7. 获取当前页数据 `data`。
8. 返回分页数据,包括当前页数据、总记录数和总页数。
### 4.2 分页与排序的结合
#### 4.2.1 分页排序的原理
分页排序是指在进行数据库分页查询的同时,还对查询结果进行排序。其原理是将排序条件与分页查询条件结合,在执行分页查询时同时应用排序条件,从而实现对分页结果的排序。
#### 4.2.2 PHP中实现分页排序
```php
<?php
// 获取分页参数
$page = isset($_GET['page']) ? intval($_GET['page']) : 1;
$limit = isset($_GET['limit']) ? intval($_GET['limit']) : 10;
// 获取排序参数
$sort = isset($_GET['sort']) ? trim($_GET['sort']) : 'id';
$order = isset($_GET['order']) ? trim($_GET['order']) : 'asc';
// 构建查询语句
$sql = "SELECT * FROM table ORDER BY $sort $order";
// 执行分页查询
$result = $db->query($sql)->fetch_all();
// 计算总记录数
$total = count($result);
// 计算总页数
$totalPages = ceil($total / $limit);
// 获取当前页数据
$offset = ($page - 1) * $limit;
$data = array_slice($result, $offset, $limit);
// 返回分页数据
echo json_encode(['data' => $data, 'total' => $total, 'totalPages' => $totalPages]);
?>
```
**代码逻辑分析:**
1. 获取分页参数 `page` 和 `limit`,并进行类型转换和默认值设置。
2. 获取排序参数 `sort` 和 `order`,并进行去除前后空格处理。
3. 构建查询语句,将排序条件与分页查询条件结合。
4. 执行分页查询,并获取所有查询结果。
5. 计算总记录数 `total`。
6. 计算总页数 `totalPages`。
7. 获取当前页数据 `data`。
8. 返回分页数据,包括当前页数据、总记录数和总页数。
# 5. PHP数据库分页的常见问题与解决方案
### 5.1 分页时数据不完整
**原因分析:**
* **数据表结构不合理:**未设置主键或唯一索引,导致分页时无法正确获取记录总数。
* **SQL查询语句错误:**查询语句未包含主键或唯一索引字段,导致无法正确分组和计数。
**解决方法:**
* **确保数据表结构合理:**为数据表设置主键或唯一索引。
* **优化SQL查询语句:**在查询语句中包含主键或唯一索引字段,并使用`GROUP BY`和`COUNT()`函数正确分组和计数。
### 5.2 分页时性能低下
**原因分析:**
* **数据量过大:**数据表中记录数过多,导致分页查询时需要扫描大量数据。
* **索引使用不当:**未在数据表中创建合适的索引,导致分页查询时无法利用索引加速查询。
* **SQL查询语句优化不当:**查询语句中包含不必要的子查询或复杂逻辑,导致查询效率低下。
**解决方法:**
* **优化数据结构:**对数据表进行分表或分区,减少单表数据量。
* **创建合适索引:**在数据表中创建主键索引和覆盖索引,加速分页查询。
* **优化SQL查询语句:**使用`LIMIT`和`OFFSET`子句正确实现分页,避免不必要的子查询和复杂逻辑。
0
0
相关推荐
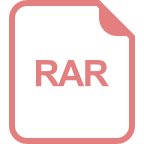
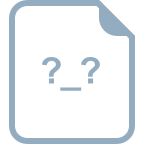
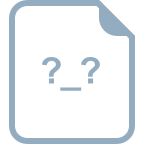
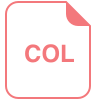
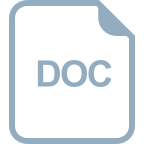
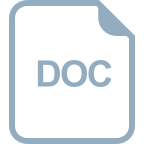
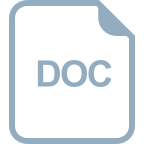
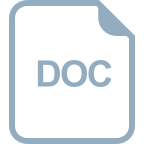
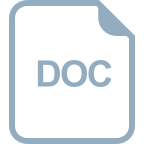