C#中的协变与逆变详解
发布时间: 2024-03-20 12:07:00 阅读量: 37 订阅数: 42 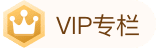
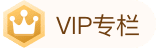
# 1. C#中的泛型和类型参数
泛型是C#中非常重要的特性,它能够让我们编写更加通用和灵活的代码,让代码更具有复用性。在使用泛型时,我们经常会接触到类型参数和泛型约束这两个概念。让我们一起来深入了解它们。
# 2. 协变与逆变的概念和原理
在C#中,协变和逆变是泛型类型参数的一种高级特性,可以使我们的代码更加灵活和可复用。接下来我们将详细介绍协变和逆变的概念和原理。
# 3. C#中的协变和逆变实践
在C#中,我们可以通过使用关键字`out`实现协变(Covariance),以及通过关键字`in`实现逆变(Contravariance)。这两个关键字可以应用于接口、委托和泛型类型参数上,让我们来看一下具体的实践案例:
#### 3.1 协变的实例化和具体应用
##### 3.1.1 接口中的协变
首先,让我们定义一个接口`IFruit`,表示水果的接口:
```csharp
public interface IFruit
{
string GetColor();
}
```
接着,我们定义一个实现接口`IFruit`的具体类`Apple`:
```csharp
public class Apple : IFruit
{
public string GetColor()
{
return "Red";
}
}
```
现在,我们创建一个协变接口`ICovariant<out T>`,其中`out T`表示`T`是一个协变类型参数:
```csharp
public interface ICovariant<out T>
{
T GetItem();
}
```
然后,我们实现`ICovariant<out T>`接口:
```csharp
public class Covariant<T> : ICovariant<T>
{
private T item;
public Covariant(T item)
{
this.item = item;
}
public T GetItem()
{
return item;
}
}
```
现在,我们来测试一下协变的具体应用:
```csharp
Apple apple = new Apple();
ICovariant<IFruit> covariantApple = new Covariant<Apple>(apple);
IFruit fruit = covariantApple.GetItem();
Console.WriteLine($"The color of the fruit is {fruit.GetColor()}");
```
##### 3.1.2 泛型委托中的协变
除了接口,我们也可以在泛型委托中使用协变。假设我们有一个泛型委托`Func<out T>`,表示返回类型为`T`的委托:
```csharp
public delegate T Func<out T>();
```
我们可以使用这个泛型委托来实现协变:
```csharp
Func<IFruit> getFruit = () => new Apple();
IFruit newFruit = getFruit();
Console.WriteLine($"The color of the new fruit is {newFruit.GetColor()}");
```
#### 3.2 逆变的实例化和具体应用
##### 3.2.1 委托中的逆变
现在,让我们来实现一个逆变的委托`Action<in T>`,其中`in T`表示`T`是一个逆
0
0
相关推荐
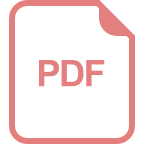
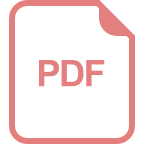
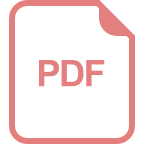
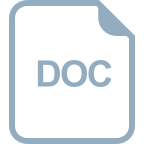
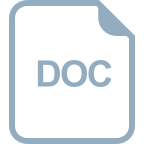
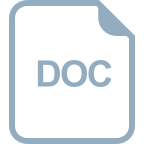
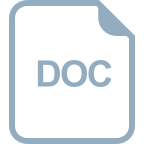
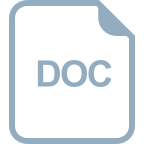