PHP连接MySQL数据库常见错误大揭秘:轻松解决,不再犯错
发布时间: 2024-07-31 08:13:11 阅读量: 18 订阅数: 14 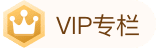
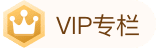
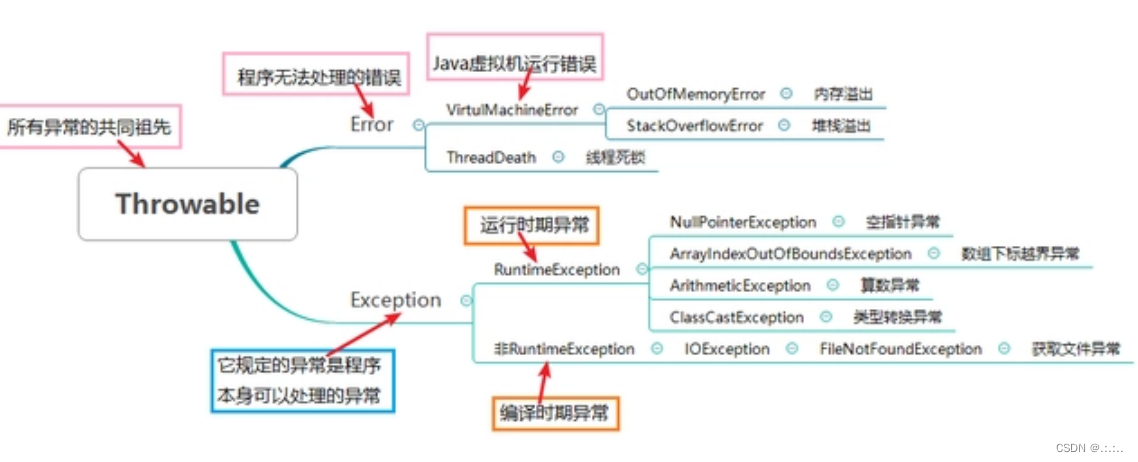
# 1. PHP连接MySQL数据库基础**
PHP连接MySQL数据库是Web开发中的常见任务。本节将介绍PHP连接MySQL数据库的基本知识,包括连接参数、连接方法和查询执行。
**连接参数**
连接MySQL数据库需要以下参数:
- 主机名或IP地址
- 用户名
- 密码
- 数据库名称
**连接方法**
PHP提供了多种连接MySQL数据库的方法,包括:
- `mysqli_connect()`:面向过程风格
- `mysqli()`:面向对象风格
- `PDO`:PHP数据对象扩展
**查询执行**
连接数据库后,可以使用SQL语句执行查询。PHP提供了以下方法执行查询:
- `mysqli_query()`:执行查询并返回结果集
- `mysqli_fetch_array()`:从结果集中获取一行数据
- `mysqli_fetch_assoc()`:从结果集中获取一行数据,键为字段名
# 2. PHP连接MySQL数据库常见错误
### 2.1 连接失败错误
#### 2.1.1 用户名或密码错误
**错误信息:**
```
mysqli_connect(): (HY000/1045): Access denied for user 'username'@'hostname' (using password: YES)
```
**原因:**
用户名或密码不正确。
**解决方案:**
* 检查用户名和密码是否正确。
* 确保用户具有连接数据库的权限。
#### 2.1.2 数据库不存在
**错误信息:**
```
mysqli_connect(): (HY000/1049): Unknown database 'database_name'
```
**原因:**
指定的数据库不存在。
**解决方案:**
* 检查数据库名称是否正确。
* 创建数据库。
#### 2.1.3 权限不足
**错误信息:**
```
mysqli_connect(): (HY000/1044): Access denied for user 'username'@'hostname' to database 'database_name'
```
**原因:**
用户没有连接数据库的权限。
**解决方案:**
* 授予用户连接数据库的权限。
* 检查用户是否具有必要的权限。
### 2.2 查询失败错误
#### 2.2.1 SQL语法错误
**错误信息:**
```
mysqli_query(): (HY000/1064): You have an error in your SQL syntax; check the manual that corresponds to your MySQL server version for the right syntax to use near 'WHERE name = 'John' at line 1
```
**原因:**
SQL语句语法错误。
**解决方案:**
* 检查SQL语句的语法是否正确。
* 使用调试工具或日志记录来识别错误。
#### 2.2.2 表或字段不存在
**错误信息:**
```
mysqli_query(): (HY000/1054): Unknown column 'name' in 'where clause'
```
**原因:**
指定的表或字段不存在。
**解决方案:**
* 检查表和字段名称是否正确。
* 确保表存在。
#### 2.2.3 数据类型不匹配
**错误信息:**
```
mysqli_query(): (HY000/1064): Data type mismatch in query
```
**原因:**
插入或更新的数据类型与表中定义的类型不匹配。
**解决方案:**
* 检查数据类型是否正确。
* 确保数据类型与表中定义的类型一致。
# 3. PHP连接MySQL数据库最佳实践
### 3.1 优化数据库连接
#### 3.1.1 使用连接池
连接池是一种缓存机制,用于存储已建立的数据库连接,以便可以根据需要重复使用它们。这可以显着提高性能,因为它消除了为每个请求建立新连接的开销。
**代码示例:**
```php
// 创建连接池
$pool = new mysqli_pool("localhost", "root", "password", "database");
// 获取连接
$conn = $pool->get();
// 使用连接
$conn->query("SELECT * FROM users");
// 释放连接
$pool->release($conn);
```
**逻辑分析:**
* `mysqli_pool` 类创建一个连接池,它将管理连接的创建和释放。
* `get()` 方法从池中获取一个可用连接。
* `query()` 方法在连接上执行 SQL 查询。
* `release()` 方法将连接释放回池中,以便可以重复使用。
#### 3.1.2 延迟加载
延迟加载是一种技术,它只在需要时才建立数据库连接。这可以减少不必要的连接开销,尤其是在应用程序不频繁访问数据库的情况下。
**代码示例:**
```php
// 创建延迟加载连接
$conn = null;
// 在需要时获取连接
if ($conn === null) {
$conn = new mysqli("localhost", "root", "password", "database");
}
// 使用连接
$conn->query("SELECT * FROM users");
```
**逻辑分析:**
* `$conn` 变量最初设置为 `null`,表示尚未建立连接。
* 当需要连接时,`if` 语句检查 `$conn` 是否为 `null`,如果是,则建立一个新的连接。
* 然后使用连接执行 SQL 查询。
### 3.2 提高查询性能
#### 3.2.1 使用索引
索引是数据库中特殊的数据结构,它允许快速查找数据。通过在经常查询的列上创建索引,可以显着提高查询性能。
**代码示例:**
```sql
CREATE INDEX idx_name ON users (name);
```
**逻辑分析:**
* `CREATE INDEX` 语句创建一个名为 `idx_name` 的索引,它基于 `users` 表中的 `name` 列。
* 当查询使用 `name` 列时,数据库将使用索引来快速查找数据,而无需扫描整个表。
#### 3.2.2 优化查询语句
优化查询语句可以减少数据库执行查询所需的时间。以下是一些优化查询的技巧:
* 使用 `WHERE` 子句过滤不必要的数据。
* 使用 `LIMIT` 子句限制返回的行数。
* 避免使用 `SELECT *`,只选择所需的列。
* 使用适当的数据类型和比较运算符。
**代码示例:**
```sql
SELECT id, name FROM users WHERE age > 18 LIMIT 10;
```
**逻辑分析:**
* 该查询只选择 `id` 和 `name` 列,而不是所有列。
* `WHERE` 子句过滤出年龄大于 18 的用户。
* `LIMIT` 子句限制返回的行数为 10。
### 3.3 确保数据安全
#### 3.3.1 预处理语句
预处理语句是一种安全机制,它防止 SQL 注入攻击。它通过将 SQL 查询和参数分开来工作,从而使攻击者无法执行恶意代码。
**代码示例:**
```php
// 创建预处理语句
$stmt = $conn->prepare("SELECT * FROM users WHERE name = ?");
// 绑定参数
$stmt->bind_param("s", $name);
// 执行查询
$stmt->execute();
// 获取结果
$result = $stmt->get_result();
```
**逻辑分析:**
* `prepare()` 方法创建一个预处理语句,它包含 SQL 查询和一个占位符 `?`。
* `bind_param()` 方法将参数(`$name`)绑定到占位符。
* `execute()` 方法执行查询。
* `get_result()` 方法获取查询结果。
#### 3.3.2 数据验证
数据验证是一种技术,它确保用户输入的数据是有效的和安全的。它可以防止恶意输入,例如 SQL 注入和跨站点脚本攻击。
**代码示例:**
```php
// 验证用户输入
if (!filter_var($input, FILTER_VALIDATE_EMAIL)) {
throw new Exception("Invalid email address");
}
```
**逻辑分析:**
* `filter_var()` 函数使用指定的过滤器(`FILTER_VALIDATE_EMAIL`)验证用户输入。
* 如果输入无效,则抛出异常。
# 4. PHP连接MySQL数据库高级应用
### 4.1 事务处理
#### 4.1.1 事务的特性
事务是一组原子性的操作,要么全部成功,要么全部失败。事务具有以下特性:
- **原子性(Atomicity):**事务中的所有操作要么全部执行成功,要么全部回滚。
- **一致性(Consistency):**事务执行前后,数据库始终处于一致的状态。
- **隔离性(Isolation):**并发事务之间相互隔离,不会相互影响。
- **持久性(Durability):**一旦事务提交,其修改将永久保存到数据库中。
#### 4.1.2 事务的实现
在PHP中,使用`mysqli::begin_transaction()`和`mysqli::commit()`或`mysqli::rollback()`来实现事务。
```php
$mysqli = new mysqli("localhost", "username", "password", "database");
// 开始事务
$mysqli->begin_transaction();
// 执行查询
$mysqli->query("UPDATE table SET name='John' WHERE id=1");
$mysqli->query("UPDATE table SET age=30 WHERE id=1");
// 提交事务
$mysqli->commit();
```
### 4.2 存储过程和函数
#### 4.2.1 存储过程的创建和调用
存储过程是预先编译和存储在数据库中的SQL语句块。它们可以接受参数,并返回结果。
```sql
CREATE PROCEDURE get_customer_orders(IN customer_id INT)
BEGIN
SELECT * FROM orders WHERE customer_id = customer_id;
END
```
```php
$mysqli = new mysqli("localhost", "username", "password", "database");
// 调用存储过程
$stmt = $mysqli->prepare("CALL get_customer_orders(?)");
$stmt->bind_param("i", $customer_id);
$stmt->execute();
// 获取结果
$result = $stmt->get_result();
while ($row = $result->fetch_assoc()) {
echo $row['order_id'] . "\n";
}
```
#### 4.2.2 函数的创建和调用
函数与存储过程类似,但不能接受参数,只能返回一个值。
```sql
CREATE FUNCTION get_customer_name(IN customer_id INT) RETURNS VARCHAR(255)
BEGIN
RETURN (SELECT name FROM customers WHERE id = customer_id);
END
```
```php
$mysqli = new mysqli("localhost", "username", "password", "database");
// 调用函数
$result = $mysqli->query("SELECT get_customer_name(1)");
$row = $result->fetch_assoc();
echo $row['get_customer_name(1)'];
```
### 4.3 数据库备份和恢复
#### 4.3.1 备份策略
定期备份数据库至关重要,以防止数据丢失。常见的备份策略包括:
- **完全备份:**备份整个数据库。
- **增量备份:**仅备份自上次备份后更改的数据。
- **差异备份:**备份自上次完全备份后更改的数据。
#### 4.3.2 恢复过程
如果数据库损坏或丢失,可以使用备份来恢复数据。恢复过程包括:
1. 停止数据库服务。
2. 删除损坏或丢失的数据库文件。
3. 从备份中恢复数据库文件。
4. 启动数据库服务。
# 5. PHP连接MySQL数据库常见问题解答
### 5.1 如何处理特殊字符?
在处理特殊字符时,需要考虑以下几点:
- **转义特殊字符:**使用 `mysqli_real_escape_string()` 函数转义特殊字符,防止 SQL 注入攻击。
- **使用预处理语句:**预处理语句可以自动转义特殊字符,更安全。
```php
$sql = "SELECT * FROM users WHERE username = ?";
$stmt = $conn->prepare($sql);
$stmt->bind_param("s", $username);
$stmt->execute();
```
### 5.2 如何解决中文乱码问题?
解决中文乱码问题需要考虑以下方面:
- **设置字符集:**在连接数据库时,设置字符集为 `utf8`。
- **使用 `mb_convert_encoding()` 函数:**将中文数据转换为 `utf8` 编码。
- **使用 `iconv()` 函数:**将中文数据转换为 `utf8` 编码。
```php
$sql = "SELECT * FROM users WHERE username = ?";
$stmt = $conn->prepare($sql);
$stmt->bind_param("s", $username);
$stmt->execute();
$result = $stmt->get_result();
while ($row = $result->fetch_assoc()) {
$username = mb_convert_encoding($row['username'], 'utf-8', 'gb2312');
}
```
### 5.3 如何提高数据库并发性能?
提高数据库并发性能可以从以下方面入手:
- **使用连接池:**连接池可以复用数据库连接,减少创建和销毁连接的开销。
- **优化查询语句:**使用索引、优化查询语句可以减少查询时间。
- **分库分表:**将数据分布到多个数据库或表中,可以减轻单个数据库的压力。
- **使用缓存:**缓存常用的数据,可以减少数据库查询次数。
- **使用读写分离:**将读写操作分离到不同的数据库服务器上,可以提高并发性能。
# 6. PHP连接MySQL数据库未来发展趋势
随着技术的不断发展,PHP连接MySQL数据库也面临着新的挑战和机遇。以下是一些PHP连接MySQL数据库的未来发展趋势:
### 6.1 云数据库服务
云数据库服务是一种基于云计算平台提供的数据库服务,它提供了弹性、可扩展、高可用和低成本的数据库解决方案。云数据库服务可以免除用户管理数据库服务器的繁琐工作,并提供自动备份、恢复和监控等功能。
**优点:**
- 弹性:可以根据业务需求动态扩展或缩减数据库资源。
- 可扩展:可以轻松地增加或减少数据库节点,以满足不断变化的负载需求。
- 高可用:采用多副本机制,确保数据库的高可用性。
- 低成本:按需付费,无需前期投资和维护成本。
**示例:**
```php
$dsn = 'mysql:dbname=my_database;host=my_host;port=3306';
$user = 'my_user';
$password = 'my_password';
try {
$conn = new PDO($dsn, $user, $password);
} catch (PDOException $e) {
echo 'Connection failed: ' . $e->getMessage();
}
```
### 6.2 NoSQL数据库
NoSQL(Not Only SQL)数据库是一种非关系型数据库,它不遵循传统的SQL结构化数据模型。NoSQL数据库提供了灵活、可扩展和高性能的存储解决方案,特别适合处理大数据和非结构化数据。
**优点:**
- 灵活:支持多种数据模型,如文档、键值对和宽表。
- 可扩展:可以轻松地扩展到数百或数千个节点。
- 高性能:提供比关系型数据库更高的吞吐量和更低的延迟。
**示例:**
```php
$client = new MongoDB\Client('mongodb://localhost:27017');
$db = $client->my_database;
$collection = $db->my_collection;
$document = [
'name' => 'John Doe',
'age' => 30,
'occupation' => 'Software Engineer'
];
$collection->insertOne($document);
```
### 6.3 数据库管理工具
数据库管理工具(DBT)提供了一套图形化界面和工具,用于管理和监控数据库。DBT可以简化数据库管理任务,如创建和修改数据库、管理用户和权限、备份和恢复数据库等。
**优点:**
- 简化管理:提供直观的图形化界面,简化数据库管理任务。
- 提高效率:自动化任务,提高数据库管理效率。
- 增强安全性:提供安全管理功能,如用户权限管理和数据加密。
**示例:**
使用phpMyAdmin管理MySQL数据库:
1. 访问phpMyAdmin网站(https://www.phpmyadmin.net/)。
2. 输入数据库服务器地址、用户名和密码。
3. 点击“登录”按钮。
4. 在phpMyAdmin界面中,可以管理数据库、表、字段和数据。
0
0
相关推荐
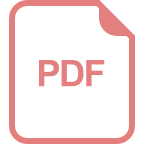
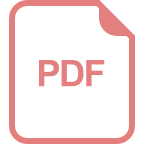
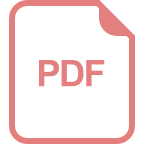





