傅里叶逆变换:10个实用技巧,轻松驾驭时域与频域
发布时间: 2024-07-13 19:50:01 阅读量: 135 订阅数: 51 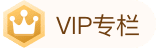
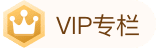
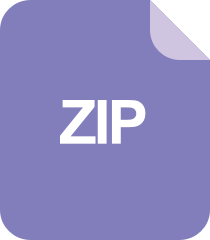
fft_傅里叶变换_python_时域频域_时频转化_

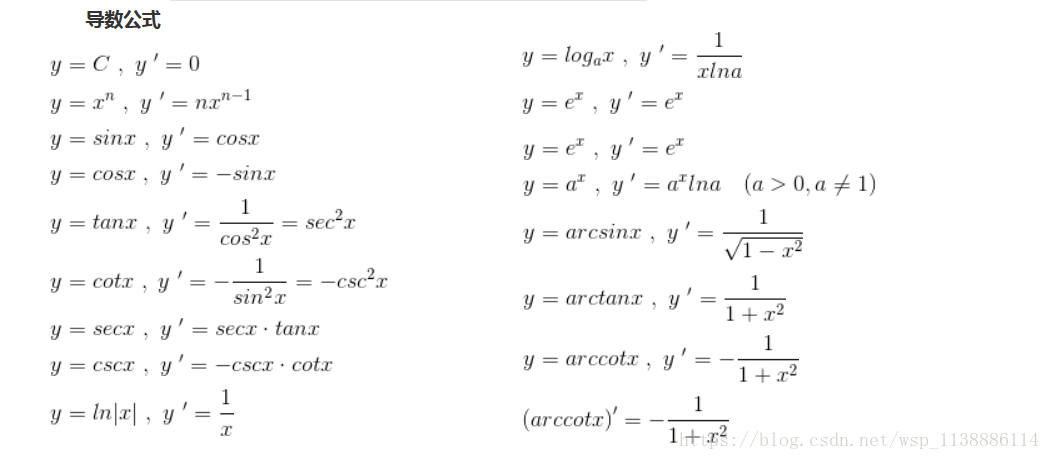
# 1. 傅里叶逆变换的理论基础
傅里叶逆变换是傅里叶变换的逆运算,用于从频域信号中恢复时域信号。其数学表达式为:
```
x(t) = (1/2π) ∫[-∞,∞] X(f) e^(2πift) df
```
其中:
- `x(t)` 是时域信号
- `X(f)` 是频域信号
- `f` 是频率
傅里叶逆变换的物理意义是:时域信号是由不同频率的正弦波叠加而成的,傅里叶逆变换可以将这些正弦波从频域中提取出来,并重新组合成时域信号。
# 2. 傅里叶逆变换的实用技巧
### 2.1 时域信号的复原
#### 2.1.1 采样定理与奈奎斯特频率
采样定理规定,为了不失真地复原一个连续时域信号,其采样频率必须大于或等于信号中最高频率成分的两倍。奈奎斯特频率即为这个最低采样频率,它等于信号最高频率的一半。
**代码块:**
```python
import numpy as np
# 采样频率
fs = 1000
# 最高频率
f_max = 500
# 采样间隔
dt = 1 / fs
# 采样点数
N = 1000
# 创建连续时域信号
t = np.arange(0, N * dt, dt)
x = np.sin(2 * np.pi * f_max * t)
# 采样信号
x_sampled = x[::2]
# 复原信号
x_reconstructed = np.fft.ifft(np.fft.fft(x_sampled))
```
**逻辑分析:**
* `np.fft.fft(x_sampled)`:对采样信号进行傅里叶变换,得到其频谱。
* `np.fft.ifft(np.fft.fft(x_sampled))`:对频谱进行傅里叶逆变换,复原时域信号。
#### 2.1.2 傅里叶逆变换的离散化
离散傅里叶逆变换(IDFT)是傅里叶逆变换在离散域中的应用。它将离散频谱变换回离散时域信号。
**代码块:**
```python
import numpy as np
# 频谱
X = np.array([1, 2, 3, 4, 5])
# 离散傅里叶逆变换
x = np.fft.ifft(X)
```
**逻辑分析:**
* `np.fft.ifft(X)`:对频谱进行离散傅里叶逆变换,复原时域信号。
### 2.2 频域信号的复原
#### 2.2.1 频谱镜像与频谱搬移
傅里叶逆变换后的频谱与原频谱是镜像对称的。为了复原正确的频谱,需要对镜像频谱进行搬移。
**代码块:**
```python
import numpy as np
# 频谱
X = np.array([1, 2, 3, 4, 5])
# 频谱镜像
X_mirror = np.flip(X)
# 频谱搬移
X_shifted = np.roll(X_mirror, -int(len(X) / 2))
```
**逻辑分析:**
* `np.flip(X)`:对频谱进行镜像。
* `np.roll(X_mirror, -int(len(X) / 2))`:将镜像频谱向左搬移一半长度,复原正确的频谱。
#### 2.2.2 滤波与频谱整形
傅里叶逆变换可以用于滤波和频谱整形。通过对频谱进行修改,可以去除噪声或增强特定频率成分。
**代码块:**
```python
import numpy as np
# 频谱
X = np.array([1, 2, 3, 4, 5])
# 创建滤波器
filter = np.ones(len(X))
filter[2:4] = 0
# 滤波
X_filtered = X * filter
# 傅里叶逆变换
x_filtered = np.fft.ifft(X_filtered)
```
**逻辑分析:**
* `np.ones(len(X))`:创建与频谱长度相同的单位滤波器。
* `filter[2:4] = 0`:将滤波器中 2-3 索引处的元素置为 0,实现带通滤波。
* `X_filtered = X * filter`:将滤波器应用于频谱。
* `np.fft.ifft(X_filtered)`:对滤波后的频谱进行傅里叶逆变换,复原滤波后的时域信号。
### 2.3 傅里叶逆变换的应用
#### 2.3.1 图像处理中的傅里叶逆变换
傅里叶逆变换在图像处理中广泛应用,例如图像去噪和锐化。
**代码块:**
```python
import numpy as np
import cv2
# 读取图像
image = cv2.imread('image.jpg')
# 傅里叶变换
F = np.fft.fft2(image)
# 频谱移位
F_shifted = np.fft.fftshift(F)
# 去噪
F_filtered = F_shifted * np.exp(-0.1 * (np.abs(F_shifted) ** 2))
# 傅里叶逆变换
image_denoised = np.fft.ifft2(F_filtered)
# 显示去噪后的图像
cv2.imshow('Denoised Image', np.abs(image_denoised))
```
**逻辑分析:**
* `np.fft.fft2(image)`:对图像进行二维傅里叶变换。
* `np.fft.fftshift(F)`:将频谱移位到图像中心。
* `F_filtered = F_shifted * np.exp(-0.1 * (np.abs(F_shifted) ** 2))`:对频谱进行去噪,衰减高频噪声。
* `np.fft.ifft2(F_filtered)`:对滤波后的频谱进行傅里叶逆变换,复原去噪后的图像。
#### 2.3.2 音频处理中的傅里叶逆变换
傅里叶逆变换在音频处理中也广泛应用,例如音频降噪和均衡。
**代码块:**
```python
import numpy as np
import librosa
# 读取音频
audio, sr = librosa.load('audio.wav')
# 傅里叶变换
F = np.fft.fft(audio)
# 频谱移位
F_shifted = np.fft.fftshift(F)
# 降噪
F_filtered = F_shifted * np.exp(-0.1 * (np.abs(F_shifted) ** 2))
# 傅里叶逆变换
audio_denoised = np.fft.ifft(F_filtered)
# 播放降噪后的音频
librosa.play(audio_denoised, sr)
```
**逻辑分析:**
* `np.fft.fft(audio)`:对音频信号进行一维傅里叶变换。
* `np.fft.fftshift(F)`:将频谱移位到音频信号中心。
* `F_filtered = F_shifted * np.exp(-0.1 * (np.abs(F_shifted) ** 2))`:对频谱进行降噪,衰减高频噪声。
* `np.fft.ifft(F_filtered)`:对滤波后的频谱进行傅里叶逆变换,复原降噪后的音频信号。
# 3. 傅里叶逆变换的算法实现
### 3.1 快速傅里叶变换(FFT)算法
#### 3.1.1 FFT的原理与步骤
快速傅里叶变换(FFT)算法是一种用于高效计算离散傅里叶变换(DFT)的算法。DFT将时域信号转换为频域信号,而FFT算法可以将DFT的计算复杂度从O(N^2)降低到O(N log N),其中N为信号长度。
FFT算法的基本思想是将DFT分解为一系列较小的DFT,并利用对称性和周期性等性质进行优化。具体步骤如下:
1. **将信号分解为偶数和奇数部分:**将长度为N的信号x[n]分解为偶数部分x_e[n]和奇数部分x_o[n],其中:
```
x_e[n] = x[2n]
x_o[n] = x[2n + 1]
```
2. **递归地计算偶数和奇数部分的DFT:**对偶数部分和奇数部分分别进行FFT计算,得到X_e[k]和X_o[k]。
3. **合并偶数和奇数部分的DFT结果:**将偶数部分和奇数部分的DFT结果合并,得到最终的DFT结果X[k],其中:
```
X[k] = X_e[k] + W_N^k X_o[k]
```
其中,W_N是N次单位根,定义为:
```
W_N = e^(-2πi/N)
```
#### 3.1.2 FFT的应用场景
FFT算法广泛应用于各种信号处理领域,包括:
* 图像处理:图像压缩、去噪、增强
* 音频处理:音频压缩、降噪、混音
* 通信系统:调制、解调、信道均衡
* 雷达和声纳:信号处理、目标检测
* 科学计算:数值积分、微分方程求解
### 3.2 傅里叶逆变换的具体步骤
傅里叶逆变换将频域信号转换为时域信号。其具体步骤如下:
#### 3.2.1 复数信号的处理
频域信号通常是复数形式,因此在进行傅里叶逆变换之前,需要将复数信号转换为实数信号。这可以通过以下步骤实现:
1. **计算复数信号的幅度和相位:**
```
amplitude = abs(X[k])
phase = angle(X[k])
```
2. **构造实数信号:**
```
x_real[n] = amplitude * cos(phase)
x_imag[n] = amplitude * sin(phase)
```
#### 3.2.2 频谱的平移与镜像
在进行傅里叶逆变换之前,需要将频谱平移到原点,并进行镜像处理。这可以通过以下步骤实现:
1. **频谱平移:**将频谱平移到原点,即:
```
X_shifted[k] = X[k] * e^(-2πi k N/2)
```
2. **频谱镜像:**将频谱镜像到负频率部分,即:
```
X_mirrored[k] = X_shifted[N - k]
```
### 3.3 傅里叶逆变换的优化方法
#### 3.3.1 时间复杂度的分析
傅里叶逆变换的原始算法的时间复杂度为O(N^2),其中N为信号长度。FFT算法可以将时间复杂度降低到O(N log N)。
#### 3.3.2 并行化与加速
傅里叶逆变换的计算可以并行化,以进一步提高计算速度。并行化方法包括:
* **多线程并行:**将计算任务分配给多个线程,同时执行。
* **GPU加速:**利用GPU的并行计算能力,加速傅里叶逆变换的计算。
# 4.1 图像去噪中的傅里叶逆变换
### 4.1.1 图像噪声的类型与傅里叶变换
图像噪声是一种常见的图像质量问题,它会影响图像的清晰度和可读性。图像噪声的类型有很多,常见的有:
- **高斯噪声:**一种常见的噪声类型,其分布呈正态分布。它通常是由传感器热噪声或光子散粒引起的。
- **椒盐噪声:**一种黑白噪声,其像素值要么是黑色(0),要么是白色(255)。它通常是由损坏的像素或数据传输错误引起的。
- **脉冲噪声:**一种尖峰噪声,其像素值明显高于或低于周围像素。它通常是由相机传感器缺陷或数据传输错误引起的。
傅里叶变换可以用于分析图像噪声的频率分布。通过将图像转换为频域,我们可以观察到不同频率分量的噪声分布。
### 4.1.2 傅里叶逆变换的去噪算法
傅里叶逆变换可以用于去噪图像。其基本思想是将图像转换为频域,然后对噪声分量进行滤波,最后将滤波后的频域信号转换为时域,得到去噪后的图像。
傅里叶逆变换去噪算法的步骤如下:
1. 将图像转换为频域。
2. 设计一个滤波器来滤除噪声分量。
3. 将滤波后的频域信号转换为时域。
滤波器可以是低通滤波器、高通滤波器或带通滤波器。滤波器的选择取决于噪声的类型和频率分布。
**代码示例:**
```python
import numpy as np
import cv2
def fourier_denoise(image):
"""
使用傅里叶逆变换对图像进行去噪。
参数:
image: 输入图像。
返回:
去噪后的图像。
"""
# 将图像转换为频域
dft = cv2.dft(np.float32(image), flags=cv2.DFT_COMPLEX_OUTPUT)
dft_shift = np.fft.fftshift(dft)
# 设计低通滤波器
rows, cols = image.shape
crow, ccol = rows // 2, cols // 2
mask = np.zeros((rows, cols, 2), np.uint8)
mask[crow - 30:crow + 30, ccol - 30:ccol + 30] = 1
# 滤波频域信号
fshift = dft_shift * mask
# 将滤波后的频域信号转换为时域
ishift = np.fft.ifftshift(fshift)
img_back = cv2.idft(ishift, flags=cv2.DFT_SCALE | cv2.DFT_REAL_OUTPUT)
return img_back
```
**代码逻辑分析:**
* `cv2.dft()`函数将图像转换为频域。
* `np.fft.fftshift()`函数将频域信号的零频率分量移动到频谱的中心。
* `mask`变量定义了一个低通滤波器,它将频谱中心附近的低频分量保留下来,而滤除高频分量。
* `fshift`变量将频域信号与滤波器相乘,从而滤除噪声分量。
* `np.fft.ifftshift()`函数将频域信号的零频率分量移回频谱的原点。
* `cv2.idft()`函数将滤波后的频域信号转换为时域,得到去噪后的图像。
# 5.1 量子力学中的傅里叶逆变换
### 5.1.1 波函数的傅里叶变换
在量子力学中,粒子的波函数描述了其状态。波函数可以表示为复数函数,其幅度平方表示粒子在特定位置或动量处的概率。
波函数的傅里叶变换将波函数从位置域变换到动量域。动量域中的波函数称为动量分布,它描述了粒子具有特定动量的概率。
傅里叶变换公式为:
```
ψ(p) = ∫_{-\infty}^{\infty} ψ(x) e^(-ipx) dx
```
其中:
* ψ(x) 是位置域中的波函数
* ψ(p) 是动量域中的波函数
* p 是动量
### 5.1.2 傅里叶逆变换在量子力学中的应用
傅里叶逆变换在量子力学中具有广泛的应用,包括:
* **波函数的复原:**通过傅里叶逆变换,可以从动量分布中复原位置域中的波函数。
* **量子态的演化:**薛定谔方程描述了量子态随时间的演化。傅里叶逆变换可以用于求解薛定谔方程,得到量子态在不同时间点的波函数。
* **量子测量:**当对量子系统进行测量时,测量结果由波函数的概率分布决定。傅里叶逆变换可以用于计算测量结果的概率。
0
0
相关推荐
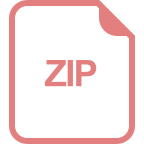
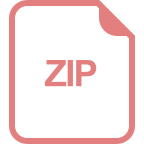
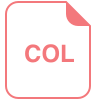
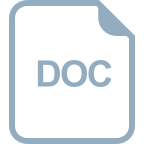
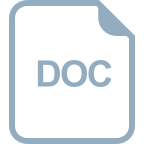
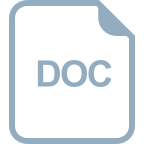
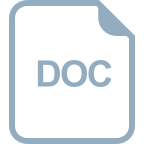
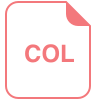