树结构时间复杂度解析:理解树形数据结构,提升算法效率
发布时间: 2024-08-25 03:26:50 阅读量: 34 订阅数: 47 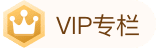
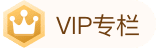
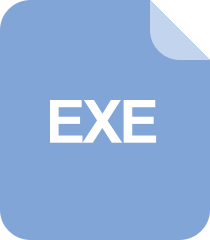
免费的防止锁屏小软件,可用于域统一管控下的锁屏机制
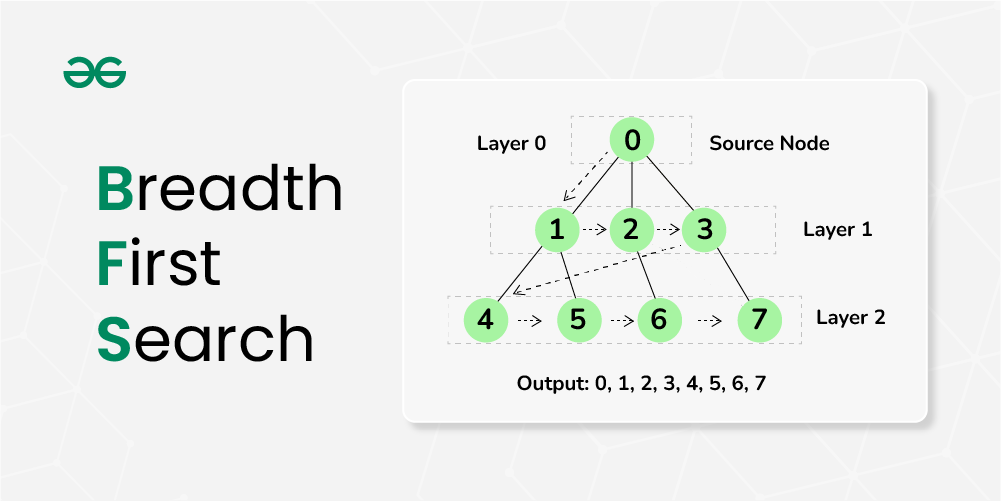
# 1. 树结构基本概念和时间复杂度
### 1.1 树结构基本概念
树结构是一种非线性数据结构,它由一个根节点和若干个子节点组成。根节点没有父节点,而子节点只有一个父节点。树结构中的节点可以进一步包含子节点,形成一个层级结构。
### 1.2 时间复杂度
时间复杂度是衡量算法效率的一个重要指标。它表示算法在最坏情况下执行所需的时间。对于树结构,时间复杂度通常与树的高度相关。树的高度是指从根节点到最深叶子节点的路径长度。
# 2. 树结构遍历算法及其时间复杂度
树结构是一种重要的数据结构,它可以用来表示具有层次关系的数据。树结构的遍历算法是访问树中所有节点的一种方法。不同的遍历算法有不同的时间复杂度,在不同的应用场景中需要选择合适的遍历算法。
### 2.1 深度优先搜索(DFS)
深度优先搜索(DFS)是一种沿着树的深度优先遍历树的算法。DFS 从根节点开始,递归地访问每个子节点,直到访问到叶节点。然后,DFS 回溯到父节点,并继续访问下一个子节点。
#### 2.1.1 先序遍历
先序遍历是一种 DFS 遍历算法,它以根节点为根的子树为单位进行遍历。先序遍历的顺序是:根节点 -> 左子树 -> 右子树。
**代码块:**
```python
def dfs_preorder(root):
if root is None:
return
print(root.data)
dfs_preorder(root.left)
dfs_preorder(root.right)
```
**逻辑分析:**
该代码块实现了 DFS 先序遍历算法。它首先检查根节点是否为空,如果是,则返回。否则,它打印根节点的数据,然后递归地调用 dfs_preorder() 函数遍历左子树和右子树。
**参数说明:**
* root:要遍历的树的根节点。
**时间复杂度:**
O(n),其中 n 是树中的节点数。
#### 2.1.2 中序遍历
中序遍历是一种 DFS 遍历算法,它以根节点为根的子树为单位进行遍历。中序遍历的顺序是:左子树 -> 根节点 -> 右子树。
**代码块:**
```python
def dfs_inorder(root):
if root is None:
return
dfs_inorder(root.left)
print(root.data)
dfs_inorder(root.right)
```
**逻辑分析:**
该代码块实现了 DFS 中序遍历算法。它首先检查根节点是否为空,如果是,则返回。否则,它递归地调用 dfs_inorder() 函数遍历左子树,然后打印根节点的数据,最后递归地调用 dfs_inorder() 函数遍历右子树。
**参数说明:**
* root:要遍历的树的根节点。
**时间复杂度:**
O(n),其中 n 是树中的节点数。
#### 2.1.3 后序遍历
后序遍历是一种 DFS 遍历算法,它以根节点为根的子树为单位进行遍历。后序遍历的顺序是:左子树 -> 右子树 -> 根节点。
**代码块:**
```python
def dfs_postorder(root):
if root is None:
return
dfs_postorder(root.left)
dfs_postorder(root.right)
print(root.data)
```
**逻辑分析:**
该代码块实现了 DFS 后序遍历算法。它首先检查根节点是否为空,如果是,则返回。否则,它递归地调用 dfs_postorder() 函数遍历左子树和右子树,然后打印根节点的数据。
**参数说明:**
* root:要遍历的树的根节点。
**时间复杂度:**
O(n),其中 n 是树中的节点数。
### 2.2 广度优先搜索(BFS)
广度优先搜索(BFS)是一种沿着树的宽度优先遍历树的算法。BFS 从根节点开始,将根节点加入队列中。然后,BFS 从队列中取出一个节点,并访问该节点。接着,BFS 将该节点的所有子节点加入队列中。BFS 重复这个过程,直到队列为空。
#### 2.2.1 层次遍历
层次遍历是一种 BFS 遍历算法,它以树的层为单位进行遍历。层次遍历的顺序是:根节点 -> 第二层节点 -> 第三层节点 -> ... -> 最后一层节点。
**代码块:**
```python
def bfs_levelorder(root):
if root is None:
return
queue = [root]
while queue:
node
```
0
0
相关推荐
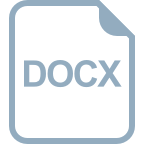
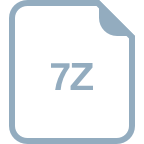
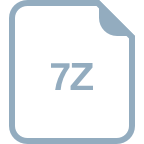
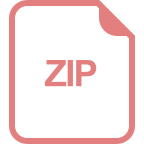
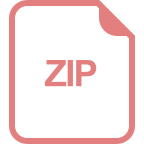