Vue.js的组件通信与状态管理
发布时间: 2024-01-21 06:11:51 阅读量: 9 订阅数: 18 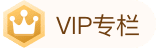
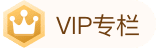
# 1. 介绍Vue.js和组件通信的重要性
## 1.1 Vue.js框架概述
Vue.js 是一套构建用户界面的渐进式框架,专注于视图层。Vue 的目标是通过尽可能简单的 API 实现响应的数据绑定和组合的视图组件。它的核心是响应的数据绑定系统,它让数据与 DOM 保持同步。Vue.js 是一个构建数据驱动的 web 界面的库。Vue.js 的设计原则是**渐进式**,所以它的核心库只关注视图层,并且非常容易学习,非常容易与其它库或已有项目整合。另一方面,Vue 也完全能够为复杂的单页应用提供驱动。
## 1.2 组件通信在前端开发中的作用
在前端开发中,组件通信是非常重要的一环。随着应用越来越复杂,不同的组件之间需要进行交互和通信,比如子组件向父组件通信、兄弟组件之间通信、祖先和后代组件之间通信等等。良好的组件通信可以使代码组织更加清晰、可维护性更高、整体结构更加健壮。
## 1.3 为什么选择Vue.js作为组件通信的解决方案
Vue.js 提供了非常便利的组件通信解决方案,包括了简单易用的 Props 和 Events 机制,以及更加强大的 provide 和 inject,$refs 和 $parent/$children 等方式。而且,Vue.js 本身集成了全局事件总线和 Vuex 状态管理,能够非常好地满足不同场景下的组件通信需求,因此在前端开发中选择 Vue.js 作为组件通信的解决方案是非常合适和高效的选择。
# 2. Vue.js组件通信的基本原则和方式
### 2.1 父子组件间通信
在Vue.js中,父子组件间通信是非常常见的场景。通常情况下,父组件通过props向子组件传递数据,子组件则通过$emit来触发事件,将数据传递给父组件。
```javascript
// ParentComponent.vue
<template>
<div>
<ChildComponent :message="parentMessage" @update="updateParentMessage" />
</div>
</template>
<script>
import ChildComponent from './ChildComponent.vue';
export default {
components: {
ChildComponent
},
data() {
return {
parentMessage: 'Hello from parent'
};
},
methods: {
updateParentMessage(newMessage) {
this.parentMessage = newMessage;
}
}
};
</script>
// ChildComponent.vue
<template>
<div>
<button @click="updateMessage">Update Message</button>
</div>
</template>
<script>
export default {
props: ['message'],
methods: {
updateMessage() {
this.$emit('update', 'Hello from child');
}
}
};
</script>
```
在上面的示例中,父组件通过props向子组件传递了一个名为message的属性,子组件可以读取并使用这个属性。子组件通过$emit('update', data)触发事件update,并将数据传递给父组件。父组件通过@update="updateParentMessage"监听子组件的update事件,然后更新自己的数据。
### 2.2 兄弟组件间通信
在Vue.js中,兄弟组件间通信可以借助于父组件作为中介来实现,或者使用事件总线或Vuex等状态管理工具。
```javascript
// ParentComponent.vue
<template>
<div>
<ChildComponent1 @update="updateData" />
<ChildComponent2 :data="sharedData" />
</div>
</template>
<script>
import ChildComponent1 from './ChildComponent1.vue';
import ChildComponent2 from './ChildComponent2.vue';
export default {
components: {
ChildComponent1,
ChildComponent2
},
data() {
return {
sharedData: ''
};
},
methods: {
updateData(newData) {
this.sharedData = newData;
}
}
};
</script>
// ChildComponent1.vue
<template>
<div>
<button @click="updateSharedData">Update Shared Data</button>
</div>
</template>
<script>
export default {
methods: {
updateSharedData() {
this.$emit('update', 'Data updated from ChildComponent1');
}
}
};
</script>
// ChildComponent2.vue
<template>
<div>
<p>{{ data }}</p>
</div>
</template>
<script>
export default {
props: ['data']
};
</script>
```
在上面的示例中,ChildComponent1通过$emit('update', data)触发update事件,将数据传递给父组件。然后父组件将数据通过props传递给ChildComponent2,实现了兄弟组件间的通信。
### 2.3 祖先/后代组件间通信
在Vue.js中,祖先/后代组件间通信可以通过provide和inject来实现。祖先组件通过provide提供数据,后代组件通过inject来注入数据。
```javascript
// AncestorComponent.vue
<template>
<div>
<DescendantComponent />
</div>
</template>
<script>
import DescendantComponent from './DescendantComponent.vue';
export default {
components: {
DescendantComponent
},
provide: {
ancestorData: 'Data provided by AncestorComponent'
}
};
</script>
// DescendantComponent.vue
<template>
<div>
<p>{{ descendantData }}</p>
</div>
</template>
<script>
export default {
inject: ['ancestorData'],
data() {
return {
descendantData: this.ancestorData
};
}
};
</script>
```
在上面的示例中,AncestorComponent通过provide向其后代组件提供了名为ancestorData的数据,而DescendantComponent通过inject注入了这个数据,实现了祖先/后代组件间的通信。
### 2.4 全局事件总线
Vue.js中可以借助一个空的Vue实例作为事件总线(事件中心),通过它来触发事件和监听事件,从而实现任意组件间的通信。
```javascript
// EventBus.js
import Vue from 'vue';
export const EventBus = new Vue();
// ComponentA.vue
<script>
import { EventBus } from './EventBus.js';
export default {
methods: {
updateData() {
EventBus.$emit('data-updated', 'Data updated from ComponentA');
}
}
};
</script>
// ComponentB.vue
<template>
<div>
<p>{{ receivedData }}</p>
</div>
</template>
<script>
import { EventBus } from './EventBus.js';
export default {
data() {
return {
receivedData: ''
};
},
created() {
EventBus.$on('data-updated', (data) => {
this.receivedData = data;
});
}
};
</script>
```
在上面的示例中,EventBus作为一个空的Vue实例被用来触发和监听事件,ComponentA通过EventBus.$emit触发事件,ComponentB通过EventBus.$on监听事件,从而实现了组件间的通信。
### 2.5 Vuex状态管理方案
Vuex是一个专门为Vue.js应用程序开发的状态管理模式,通过它可以实现组件间的集中式状态管理。状态以一个全局对象形式存储,组件通过提交mutation来改变状态,实现了组件间通信和数据共享。
```javascript
// store.js
import Vue from 'vue';
import Vuex from 'vuex';
Vue.use(Vuex);
export default new Vuex.Store({
state: {
data: ''
},
mutations
```
0
0
相关推荐
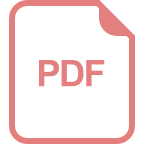
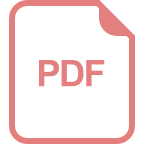
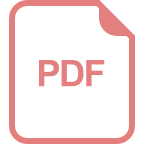
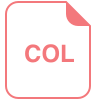
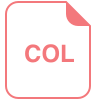
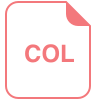
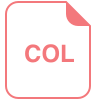

