XML布局设计与Android界面开发
发布时间: 2024-01-31 21:58:06 阅读量: 61 订阅数: 45 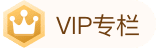
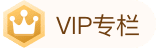
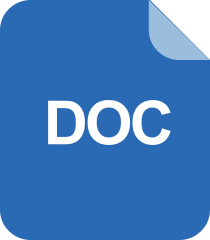
android的xml布局
# 1. 介绍
## 1.1 XML布局设计的作用和优势
XML布局设计在Android界面开发中扮演着重要的角色。它是一种将界面元素以标记语言的形式描述的技术,可以更灵活地设计和组织界面布局。
XML布局设计的优势包括:
- **可读性强**:使用XML语言描述界面元素,具有良好的可读性和可维护性,便于团队协作和代码版本管理。
- **可重用性高**:通过将相同的布局片段抽取为独立的XML文件,可以在不同的界面中重复使用,减少冗余代码。
- **灵活度高**:XML布局可以嵌套使用,灵活组合各种UI组件,满足复杂界面布局的需求。
- **可视化编辑支持**:Android Studio等开发工具提供了可视化编辑界面,方便开发者直观地设计布局。
## 1.2 Android界面开发的基本概念
在Android界面开发中,UI布局是用户界面的核心组成部分。以下是一些基本概念:
- **Activity**:Activity是Android应用程序的一个页面,它负责展示用户界面和处理用户的操作。
- **View**:View是用户界面的基本组件,如按钮、文本框、图片等。它们可以在xml布局文件中进行定义和配置。
- **Layout**:Layout是一种容器,用来组织和管理View组件的布局。常见的Layout包括LinearLayout、RelativeLayout和ConstraintLayout。
- **Widget**:Widget是UI组件的具体实现,比如Button、TextView等。
- **Event**:Event是用户操作触发的事件,如点击按钮、滑动屏幕等。通过监听和处理事件,可以实现各种交互和逻辑功能。
在后续的章节中,我们将深入了解XML布局设计和Android界面开发的相关知识和技巧。
# 2. XML布局设计基础
在Android界面开发中,XML布局设计是非常重要的一项技能。通过使用XML语言来描述界面的布局结构和属性,可以使得界面的设计更加灵活和可复用。本章将介绍XML布局设计的基础知识,包括XML语法基础、常用布局标签的介绍以及布局属性的使用技巧。
### 2.1 XML语法基础
XML(可扩展标记语言)是一种用于描述数据的标记语言,它的语法和HTML类似,但更加通用和灵活。在Android界面开发中,XML被广泛用于描述界面的布局结构和属性。
下面是一个简单的XML布局文件的示例:
```xml
<LinearLayout
xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical"
android:layout_width="match_parent"
android:layout_height="match_parent">
<TextView
android:text="Hello, World!"
android:layout_width="wrap_content"
android:layout_height="wrap_content"/>
</LinearLayout>
```
XML布局文件以根元素开始,并使用标签来描述布局的结构和属性。标签可以嵌套使用,用于表示布局的层次关系。
在上面的示例中,根元素是一个LinearLayout,它的orientation属性被设置为vertical,表示线性布局的方向为垂直方向。LinearLayout内部包含一个TextView元素,用于显示文本内容。TextView的text属性被设置为"Hello, World!",表示要显示的文本内容。
### 2.2 常用布局标签介绍
在Android界面开发中,常用的布局标签有LinearLayout、RelativeLayout和ConstraintLayout等,它们分别具有不同的布局特性和用途。
#### 2.2.1 LinearLayout
LinearLayout是一种线性布局,它可以按照水平或垂直方向排列子元素。LinearLayout的orientation属性用于设置布局的排列方向,可以取值为"horizontal"或"vertical"。
下面是一个水平方向的LinearLayout示例:
```xml
<LinearLayout
android:orientation="horizontal"
android:layout_width="match_parent"
android:layout_height="wrap_content">
<Button
android:text="Button 1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"/>
<Button
android:text="Button 2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"/>
</LinearLayout>
```
在上述示例中,两个Button元素被水平排列在LinearLayout中。
#### 2.2.2 RelativeLayout
RelativeLayout是一种相对布局,它可以根据子元素之间的相对位置来确定布局的结构。RelativeLayout使用各种属性来控制子元素的位置,包括android:layout_alignParentTop、android:layout_alignParentBottom、android:layout_alignParentLeft、android:layout_alignParentRight等。
下面是一个RelativeLayout的示例:
```xml
<RelativeLayout
android:layout_width="match_parent"
android:layout_height="match_parent">
<ImageView
android:src="@drawable/image"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_centerInParent="true"/>
<Button
android:text="Button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_below="@id/image_view"/>
</RelativeLayout>
```
在上述示例中,ImageView元素位于RelativeLayout的中心位置,并使用android:layout_centerInParent属性来实现。Button元素位于ImageView的下方,并使用android:layout_below属性来实现。
#### 2.2.3 ConstraintLayout
ConstraintLayout是一种约束布局,它使用约束关系来定义子元素之间的位置和大小。ConstraintLayout使用各种属性来定义约束关系,包括android:layout_constraintTop_toTopOf、android:layout_constraintTop_toBottomOf、android:layout_constraintStart_toEndOf、android:layout_constraintEnd_toStartOf等。
下面是一个ConstraintLayout的示例:
```xml
<androidx.constraintlayout.widget.ConstraintLayout
android:layout_width="match_parent"
android:layout_height="match_parent">
<Button
android:text="Button 1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintStart_toStartOf="parent"/>
<Button
android:text="Button 2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
app:layout_constraintTop_toBottomOf="@id/button1"
app:layout_constraintStart_toEndOf="@id/button1"/>
</androidx.constraintlayout.widget.ConstraintLayout>
```
在上述示例中,两个Button元素使用约束关系来确定它们之间的位置。第一个Button位于ConstraintLayout的顶部和左侧,并使用app:layout_constraintTop_toTopOf和app:layout_constraintStart_toStartOf属性来设置约束关系。第二个Button位于第一个Button的下方和右侧,并使用app:layout_constraintTop_toBottomOf和app:layout_constraintStart_toEndOf属性来设置约束关系。
### 2.3 布局属性的使用技巧
在XML布局文件中,每个布局标签可以使用一系列的属性来控制布局的行为和外观
0
0
相关推荐





